Solidity Basics: Data-Types
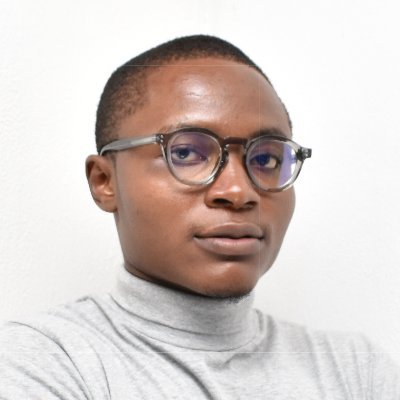
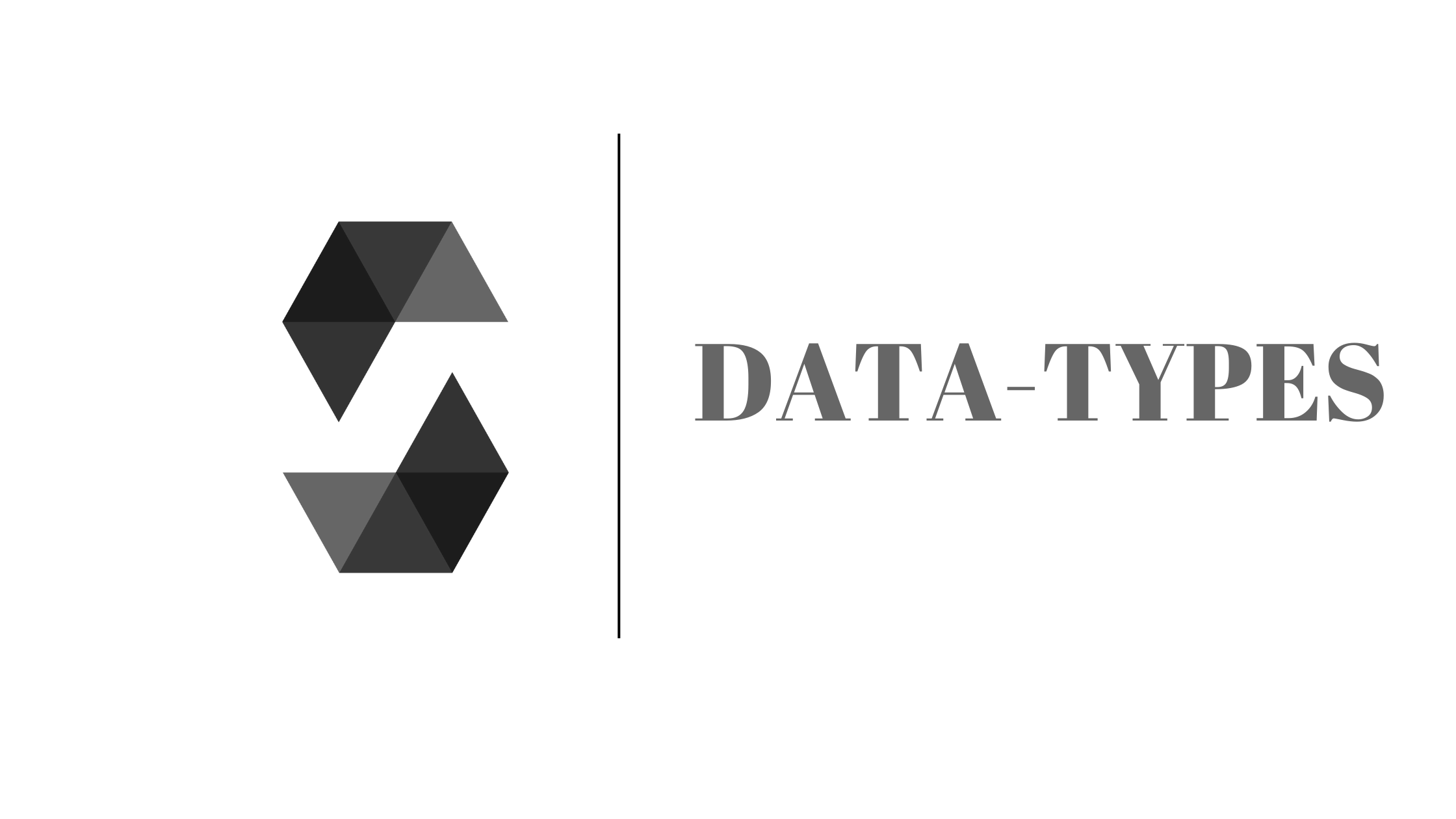
Table of Content
Introduction
Value Types
Boolean
Integer
Address
Enums
Reference Types
Bytes
String
Array
Struct
Mappings
Summary
Introduction
Solidity is a statically typed language, which means the type of each variable has to be specified at compile time, the declared types have default values called Zero State. There are two types of data types in solidity.
Value Types
Reference Types
Value Types
These are data types that simply store value. Let's look at various value types in solidity and their examples.
Boolean
The boolean type stores the value true
or false
. By default its value is false.
Example:
bool defaultValue; // false
bool declaredValue = true;
Integer
The uint
(unsigned integer) and int
(integer) are both integer types. uint
can only store positive integers(i.e The number has to be greater than or equal to zero), while int
can store both negative and positive integers. Both can be used to store integers ranging from uint8/int8
to uint256/int256
in steps of 8— uint/int
are aliases for uint256/int256
.
Example:
uint defaultValue; // 0
int defaultValue; // 0
uint256 a = 9805111;
int8 b = -12;
int32 c = 100;
Address
The address
data type is unique to solidity, it stores an Ethereum address.
Example:
address defaultValue; // 0x000000
address owner = 0x235eE805F962690254e9a440E01574376136ecb1
Enums
This is a user-defined type for enumerating discrete values.
Example:
enum Colors {
Green,
Red,
Blue
}
Colors public myColors;
Reference Types
These are data types that store the reference to a value in memory, not the value itself.
Bytes
Bytes is used to hold binary data, it is a reference type(it stores the reference to a location in memory). The fixed-size bytes32
type can hold a series of arbitrary binary data not more than 32 bytes, while the variable-sized bytes
type also holds binary data but it does not have a predefined length.
Example:
// byte1 = 8bit [01010101]
bytes32 fixedData = "0x235eE805F962690254e9a440E01574376136ecb1";
bytes dynamicData = "0x235eE805F962690254e9a440E01574376136ecb1";
Strings
Strings represent a sequence of characters encoded in the utf-8 format. Like bytes, a string can be fixed or variable-sized. It is quite difficult to work with strings in solidity(since it is mostly used for computation), which is why bytes are mostly used to store and manipulate strings(it is more gas efficient).
Example:
string name = "John";
// Converting string to byte
bytes nameA = bytes(name) // 0x00...
Arrays, Mappings, Structs
Arrays
Arrays in solidity can either be fixed or dynamic and it can contain only one value type.
// fixed array of 3 unsigned integers
uint[3] nums = [1,2,3]
// dynamic array
uint[] nums = [4,5,6,7]
Mappings
Mappings are simply hash lookup tables for key-value pairs: mapping(KEY_TYPE => VALUE_TYPE)
.
mapping(address => uint) balances;
// Add to the map
balances[msg.sender] = 100;
// - Read from the map
balances[msg.sender];
// - Update the map
balances[msg.sender] = 200;
// - delete a map value
delete balances[msg.sender];
// - Set a default value
balances[someAddressThatDoNotExist] => 0
Structs
Structs are user-defined containers for grouping variables: struct NAME {TYPE1 VARIABLE1, TYPE2 VARIABLE2;... }
struct User {
address addr;
uint score;
string name;
}
Summary
In this article, you learned about the different data types in Solidity and their use cases. However, It is important to remember that Solidity is a static-typed language, which means data types are crucial for variable declarations.
Subscribe to my newsletter
Read articles from Onyedikachi Eni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
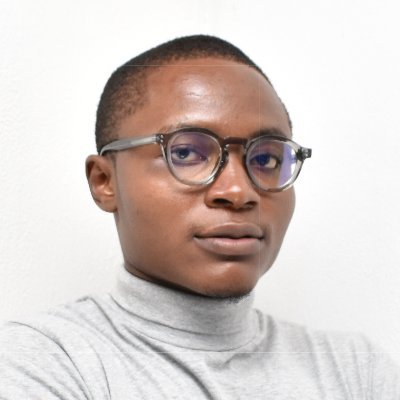
Onyedikachi Eni
Onyedikachi Eni
Dev. | Creator. | Writer.