Factory Method Pattern in Python: Crafting Objects Creatively
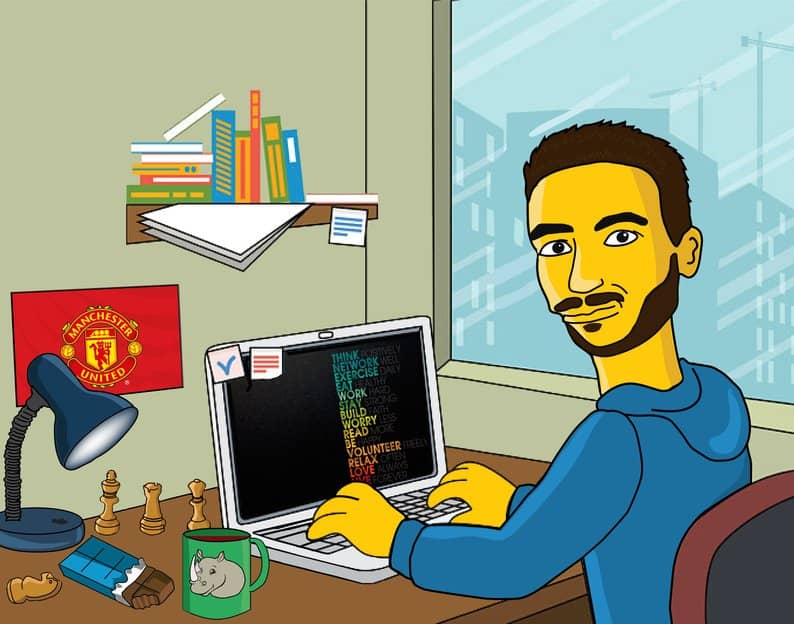
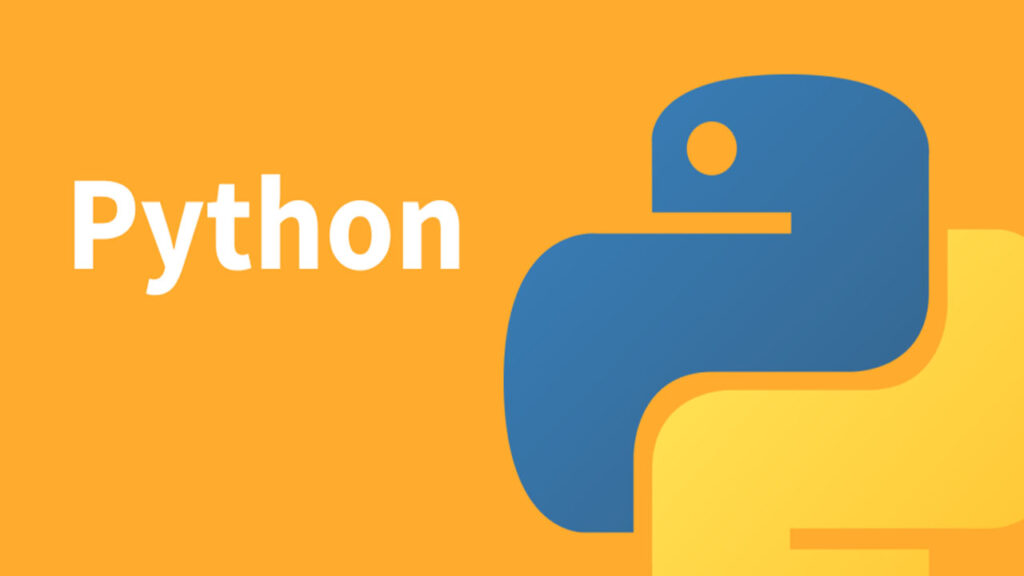
The Factory Method pattern is a creational design pattern that provides an interface for creating objects in a super class but allows subclasses to alter the type of objects that will be created. It promotes loose coupling and flexibility in object creation.
Key Components:
Creator (Superclass): This is an abstract class or interface that defines the factory method, which returns an object of the Product type. It declares the factory method but provides no implementation.
ConcreteCreator (Subclass): Subclasses of the Creator implement the factory method. Each subclass can produce objects of a different Product type.
Product: This is the abstract class or interface that defines the object created by the factory method.
ConcreteProduct: Subclasses of Product are the actual objects created by the factory method in ConcreteCreators.
Example Implementation:
Here's a simplified example of the Factory Method pattern in Python:
from abc import ABC, abstractmethod
# Product interface
class Animal(ABC):
@abstractmethod
def speak(self):
pass
# Concrete Products
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
# Creator interface
class AnimalFactory(ABC):
@abstractmethod
def create_animal(self):
pass
# Concrete Creators
class DogFactory(AnimalFactory):
def create_animal(self):
return Dog()
class CatFactory(AnimalFactory):
def create_animal(self):
return Cat()
# Usage
def get_pet(factory):
pet = factory.create_animal()
return pet
dog_factory = DogFactory()
cat_factory = CatFactory()
dog = get_pet(dog_factory)
cat = get_pet(cat_factory)
print(dog.speak()) # Output: Woof!
print(cat.speak()) # Output: Meow!
In this example, AnimalFactory
is the Creator interface with the factory method create_animal()
. Concrete creators like DogFactory
and CatFactory
implement this method to produce specific products (Dog
and Cat
).
Why It Matters:
The Factory Method pattern provides a way to create objects without specifying their exact classes. This flexibility allows you to introduce new products (ConcreteProducts) without modifying existing client code (which relies on the Creator interface). It promotes code reusability, maintainability, and scalability by adhering to the open-closed principleβopen for extension but closed for modification.
Subscribe to my newsletter
Read articles from Karun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
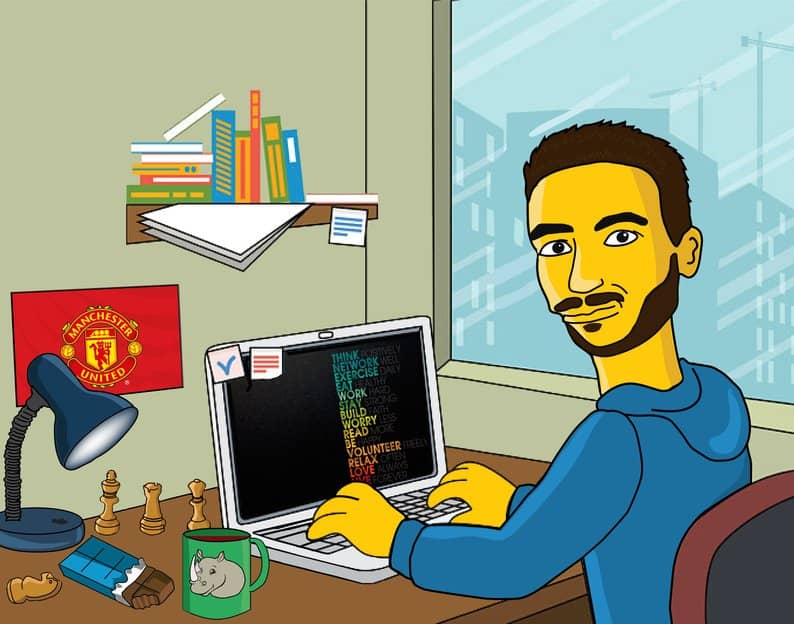
Karun
Karun
π Passionate Python Enthusiast | Educator | Blogger π Welcome to my Python playground! π I'm here to share my love for Python programming and help you master this versatile language. Whether you're just starting your coding journey or looking to level up your Python skills, you're in the right place. π Dive into my tutorials and learn Python from scratch, one step at a time. From basic syntax to complex projects, I've got you covered. π As an educator, I believe in the power of sharing knowledge. Let's learn, grow, and conquer coding challenges together. π Got a question or a cool project idea? Don't hesitate to reach out. Remember, in the world of programming, a little indentation goes a long way. Happy coding, Pythonistas! ππ» π karun.hashnode.dev πΈ https://twitter.com/karunakarhv