Array in Java
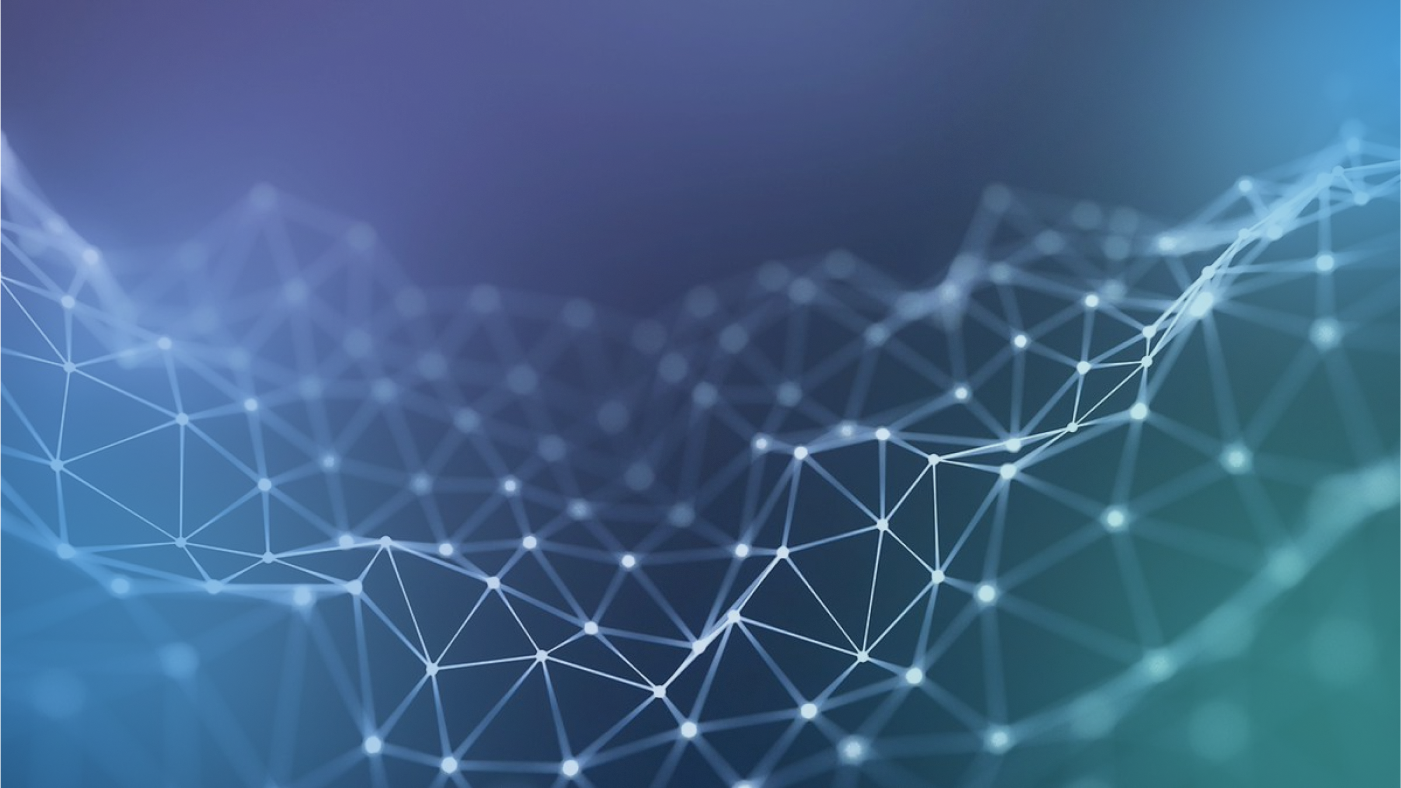
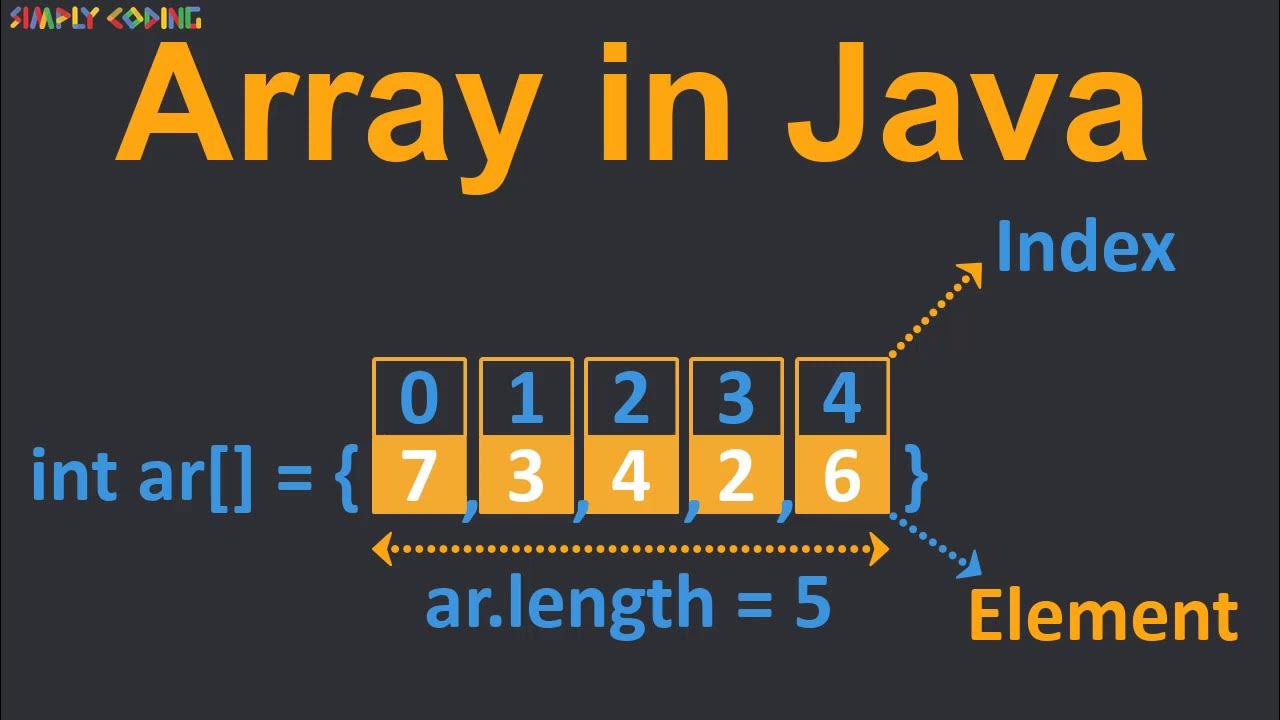
public class ArrayEx {
public static void main(String[] args){
//datatype[] reference_name = new datatype[size];
// Declaring Array
int[] rollno = new int[5];
// Directly Initializing Array
int[] rollno2 = {11,12,13,14,15};
}
}
Syntax of Array in Java
Array —
Array is a collection of data type. There are two ways to create arrays in Java. Points to remember -
1. While declaring Array ,data types represents what type of data is stored inside the Array.
2.In Array only one type of data can be stored . In above code only integers can be stored in Array not float or String or boolean values.
3.size defines total number of elements in the Array.
4.new keyword is used to create object of array at runtime .(Ignore this line if you don’t know object oriented programming)
5. rerference_name points to the array object which is stored in heap memory .
public class ArrayEx {
public static void main(String[] args){
int [] rollno; // declaration of array. rollno is getting defined int the stack.
rollno = new int [5]; // actually here object is created in the memory(heap).
Continuity of the Array in Java -
In C , C++ Array elements are stored continuously. but in Java it totally depends on the JVM whether this is going to be continuous or not. Because
Array objects are in heap. Heap objects are not continuous and also dynamic memory allocation.
Index of an Array -
Index of an Array starts from 0.
If you want to access first element of array then code is
public class ArrayEx {
public static void main(String[] args){
//datatype[] reference_name = new datatype[size];
// Directly Initializing Array
int[] rollno2 = {11,12,13,14,15};
System.out.println( rollno2[0] ); // output - 11
}
}
what is null in Java?
public class ArrayEx {
public static void main(String[] args){
//datatype[] reference_name = new datatype[size];
// Declaring Array
int[] rollno = new int[5];
System.out.println( rollno[0] );
// Directly Initializing Array
int[] rollno2 = {11,12,13,14,15};
System.out.println( rollno2[0] );
}
}
Output of above code will be
null
11
null defines any reference variable that you have by default its value going to have the null. In above ex. we did not assign any value to rollno array so the default value is null. Just remember Its not a data type or object .
Subscribe to my newsletter
Read articles from Srushti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
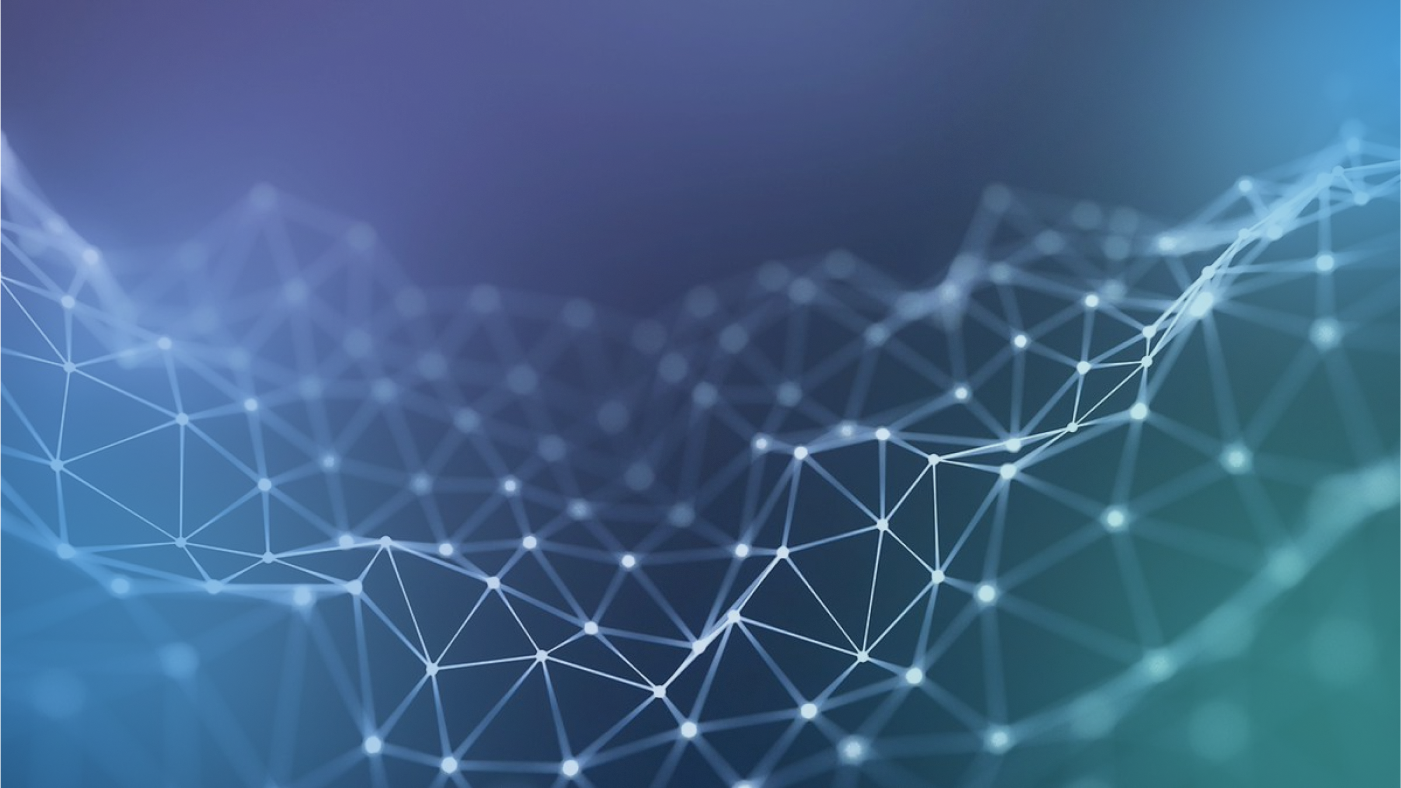