Learn Python in 10 weeks - Beginners Guide
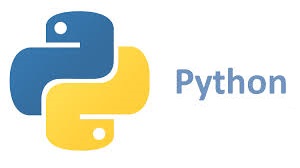
A Python syllabus for beginners typically covers fundamental programming concepts and the basics of Python programming. Here's a suggested syllabus for beginners:
Week 1: Introduction to Programming and Python
What is programming?
Setting up Python (installation)
First Python program (Hello, World!)
Variables and data types (integers, floats, strings)
Basic input and output (print and input functions)
Comments in Python
Week 2: Control Structures
Conditional statements (if, elif, else)
Boolean expressions and operators
Loops (while and for loops)
Flow control (break and continue)
Week 3: Data Structures
Lists and list operations (slicing, indexing)
Tuples
Dictionaries and Dictionary operations
Sets
List comprehensions
Week 4: Functions and Modules
Functions in Python (defining, calling, parameters, return values)
Built-in functions Creating and using modules
Importing modules
Scope and lifetime of variables
Week 5: File Handling
Opening and reading files
Writing to files
Handling exceptions (try, except, finally)
Working with text and binary files
Week 6: Object-Oriented Programming
Introduction to OOP
Classes and objects
Methods and attributes
Inheritance and polymorphism
Week 7: Error Handling and Debugging
Common types of errors
Debugging techniques
Using the Python debugger (pdb)
Week 8: Introduction to Libraries and Frameworks
Overview of popular Python libraries (e.g., NumPy, Pandas) Introduction to web frameworks (e.g., Flask, Django)
Week 9: Introduction to Data Science (Optional)
Data visualization with Matplotlib
Data Analysis with Pandas
Basic statistics with NumPy
Week 10: Final Projects and Wrap-Up
Group or individual projects to apply what has been learned
Presentation of final projects
Review and recap of key concepts
Reference:
Subscribe to my newsletter
Read articles from SQL, Python, Java, AI, ML, Data Science and Machine Learning Tips directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SQL, Python, Java, AI, ML, Data Science and Machine Learning Tips
SQL, Python, Java, AI, ML, Data Science and Machine Learning Tips
Kash is a tech enthusiast and learner with over 5+ years of experience in the tech industry.