Writing reusable Components in your Next JS or React JS app

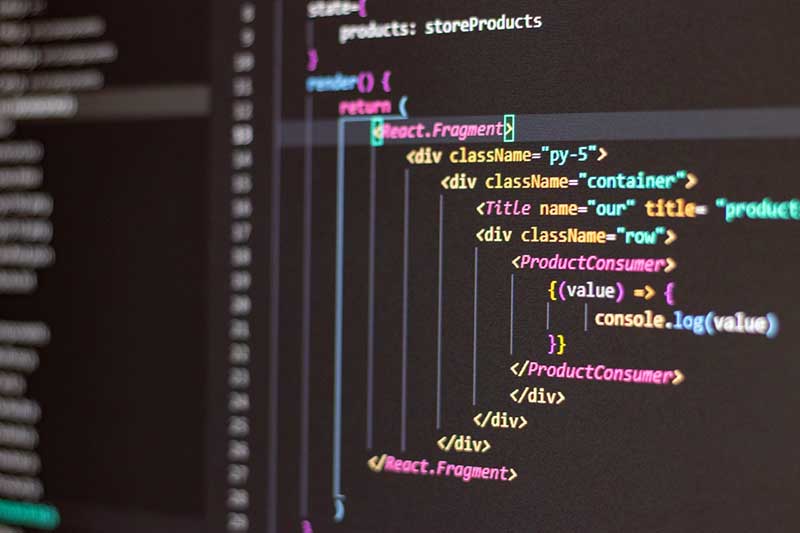
As a React js developer, one of the most important skills that you need to master is building reusable components that you can use later in your entire App, These reusable component will help you to write clean and less code which make optimizing your app easier in the future.
In this post, We are going to talk about why reusable components are essential in every react or next js application, how you can write these reusable components and what are the best practices to follow to enhance the performance of your components in your App
Why reusable components are essential in a React JS or Next JS APP
Code Maintainability: Reusable components promote a solid code structure. When you need to make updates or fix bugs, you only have to do it in one place.
Consistency: Reusable components ensure a consistent look and behavior throughout your application. For example, you can build two buttons, a primary button and a secondary button and you can use these two buttons in your entire application, by using only these two buttons in your entire application, you are satisfying one of the most important concepts in web design which is consistency, this will definitely enhace the UI and UX of your App
Development Speed: Building an application with reusable components accelerates development. Instead of reinventing the wheel for every UI element, developers can leverage existing components, saving time and effort.
Scalability: The big challenge of maintaining a consistent UI comes when your app grows and becomes larger, Reusable components scale with your application, making it easier to manage and extend as new features are added.
Team Collaboration: When multiple developers work on a project, reusable components act as a shared language. Team members can understand and use these components, fostering collaboration and reducing misunderstandings.
How to write reusable components
Before you write a reusable component, you have to keep in mind that you are going to use this component in your entire application, not just in one or two pages, so you may want to create a folder called "components" This folder, You want to add all your reusable components
Another thing you have to worry about when creating reusable components is the name of the component, The name should not be related to a specific page or section, For example, if you want to create a title component for your dashboard page, and you want the title component to be reusable, you should not name it DashboardTitle
instead you want to name it Title
To make your reusable components customizable, you have to use different props, You can also use callbacks for user interactions, and you can invoke the component with different props according to your needs, It is always a good idea to use typescript to validate your props, this will enhance your developer experience and you will prevent many development errors
In some cases, you don't want to provide props for your component when using it, so you should always provide default props for your reusable component
Use conditional logic and props to conditionally render parts of a component based on specific props or states. This makes your components dynamic and adaptive.
It is also a good idea to use other reusable components to build new reusable components, this way you ensure the component composition in your app
You should always try to make your components pure, Pure components are components whose outputs depend only on their props, which means that the pure components will return the same results for the same props, You can make your component pure by:
not using side effects such as a state or a variable that is outside the component
not making any API calls inside your component
making sure that your component returns the same outputs for the same inputs
A Great Example of a reusable component:
import { CSSProperties, HTMLAttributes } from "react";
import { H2, H4, P } from "./typography";
import { cn } from "@/lib/tailwind";
type TitleProps = {
title : string |JSX.Element ,
descreption? : string | JSX.Element ,
titleStyle? : CSSProperties ,
titleClassName? : string ,
className? : string,
descreptionStyle? : CSSProperties ,
descreptionClassName? :string ,
} & HTMLAttributes<HTMLDivElement>
export const Title : React.FC<TitleProps> = ({title , descreption , className , titleStyle , descreptionClassName , titleClassName , descreptionStyle , ...props })=>{
return <div className={cn( "w-full items-center flex flex-col" , className )} {...props} >
{ typeof title === "string" ? <H2 style={titleStyle} className={cn("text-primary text-center font-bold" , titleClassName ) } >{title}</H2> : <>{title}</> }
{ typeof descreption === "string" ? <P className={cn("text-center" , descreptionClassName ) } style={descreptionStyle} >{descreption}</P> : <>{descreption}</> }
</div>
}
This is a great example of a component I have used in my SaaS
It is basically a Title component that I can use in my entire application to ensure consistency
Underneath the title there is a conditional description, and in the Title component I am getting the titleStyle
, titleClassName
to ensure that I can restyle the title when I use the component, I am also getting the descreptionClassName
and the descreptionStyle
In the Title component, I made sure that the component is customizable by allowing restyling the title and the description by the props,
I am also using conditional rendering by setting the description and the title conditionally (according to the props input)
You can also remark that the component is a pure component, which means that it will return the same outputs for the same props and it does not include any side effects such as an API call
Conclusion
Reusable components are the building blocks that empower developers to create efficient, adaptable, and cohesive user interfaces. By following the best practices mentioned in this article, you set the stage for a robust and flexible codebase.
Subscribe to my newsletter
Read articles from Rahim directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
