Basic Shell Scripting for DevOps Engineers.

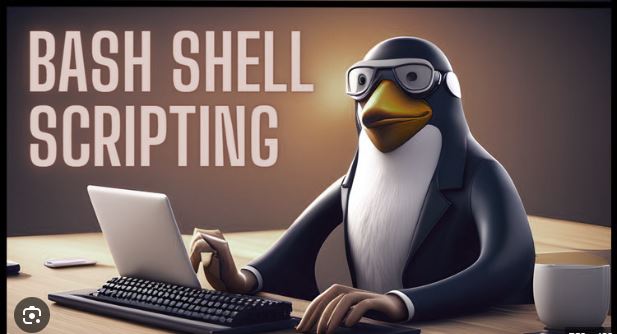
What is Kernel?
The kernel is the software that manages the communication between the hardware and the processes. It is the core component of the Linux operating system. It serves as the bridge between software and hardware, managing system resources and enabling communication between applications and the computer's hardware.
It also handles memory management, process scheduling, device drivers, system calls, and security.
The kernel is the lowest level of the operating system, and it has direct access to the hardware.
The kernel is responsible for providing a platform for the programs and services to run on top of it.
The Linux kernel is open-source, which means anyone can modify and improve it according to their needs. The Linux kernel is also modular, which means it can load and unload different components as needed.
It schedules processes, allocates CPU time, and ensures efficient multitasking by allowing processes to run concurrently.
The kernel manages system memory, allocating and deallocating memory as needed, and protecting processes from accessing each other's memory.
It interacts with the file systems, π enabling reading, writing, and accessing files on storage devices.
π The kernel is critical for the stability, security, and performance of the Linux system, as it provides a secure environment for applications to run and interact with hardware.
π‘οΈ The kernel's security is vital, as it controls access to system resources and plays a significant role in protecting the system from unauthorized access and malicious software.
π Linux's kernel is open-source software, meaning its source code is freely available, allowing users to modify and distribute it under open-source licences.
In summary, the kernel is the heart of the Linux operating system, responsible for managing resources and enabling smooth interactions between software and hardware components. Its continuous development and open-source nature have been fundamental to the success and popularity of Linux. π
π» What is Shell? π»
π Easy to understand, a shell refers to the user interface that allows interaction with an operating system, enabling users to execute commands and perform various tasks.
π Think of the shell as a "command interpreter" or a "bridge" between the user and the computer's operating system.
πββοΈ Users can communicate with the operating system by typing commands into the shell, which are then interpreted and executed by the system.
π Commands can be entered one at a time, and the shell typically displays a prompt to indicate it's ready to accept input.
π The shell allows users to navigate through the file system, list files, create directories, and move or copy files.
π Different operating systems often provide various shell options, with some popular examples being Bash (Bourne-Again Shell), PowerShell (for Windows), and Zsh (Z Shell).
π Beyond interactive use, shells are also used for scripting, allowing users to automate tasks by writing scripts consisting of multiple commands.
π Shells are powerful tools that can boost productivity by providing quick access to system utilities and enabling complex operations through simple commands.
π€ While some familiarity with commands is helpful, modern shells often provide auto-completion and command history features to ease the learning curve.
In summary, a shell is a user-friendly interface that facilitates communication between users and the operating system. By entering commands, users can perform various tasks, navigate the file system, and run applications efficiently. It plays a crucial role in interacting with the computer in a textual and command-based manner. π
π What is Linux Shell Scripting? π
π Linux Shell Scripting refers to the process of writing and executing scripts using a shell in the Linux operating system. It involves creating a series of commands in a text file that can be executed as a single program.
ποΈ Linux shell scripts are written in plain text using a text editor, containing a sequence of commands that would otherwise be executed individually in the shell.
βοΈ When the script is run, it is interpreted by the specified shell
(e.g., Bash
#!/bin/bash?
), executing the commands one after the other.π Shell scripting is used to automate repetitive tasks, perform system administration tasks, and execute a sequence of commands efficiently.
π By writing shell scripts, users can automate complex tasks, saving time and effort in executing repetitive commands.
π οΈ Shell scripts are commonly used in system administration tasks, such as backup operations, log analysis and software installation.
π Shell scripts can include loops and conditional statements, allowing for decision-making and repeating actions based on certain conditions.
π Shell scripting is often used for file management, including creating, copying, moving, and deleting files and directories.
π Shell scripts provide a convenient way to streamline processes and perform multiple operations with a single command.
π Shell scripting is not limited to Linux but can also be used on other Unix-like systems, including macOS and various BSD distributions.
π Care should be taken when writing and executing shell scripts, as they have the potential to modify system files and settings.
In summary, Linux Shell Scripting involves writing a series of commands in a text file that can be executed as a single program. It is a powerful tool for automating tasks, managing files, and performing system administration duties, making it an essential skill for Linux users and system administrators. π
TASKπ
Explain in your own words and examples, what is Shell Scripting for DevOps.
Shell scripting in DevOps involves creating automated sequences of commands for managing and streamlining software development and operations tasks. ππ§ These scripts use shell languages like Bash to interact with operating systems, configure environments, deploy applications, and perform routine tasks. For instance, a shell script could automate the deployment of a web application by pulling code from a repository, setting up the environment, and starting the server. π This enables DevOps teams to save time, reduce errors, and ensure consistency in their workflows. π€πΌ
What is
#!/bin/bash
? Can we write#!/bin/sh
as well?#!/bin/bash
is called a "shebang" and appears at the beginning of a shell script. It tells the operating system which interpreter to use for executing the script, in this case, the Bash shell. ππ Bash provides more advanced features compared to the basic/bin/sh
, offering powerful scripting capabilities and better compatibility with modern systems.Yes, you can use
#!/bin/sh
as well. It refers to the system's default shell interpreter. However, keep in mind that the functionalities might be limited compared to Bash. So, choosing between them depends on your script's requirements and desired features. ππ§Write a Shell Script which prints "This is my first script"
Steps and commands
sudo apt install vim
vim print.sh
press i to insert and paste below content
#!/bin/bash echo "This is my first script"
save file !wq or (Shift+zz)
bash print.sh
Notes:-
To create and run this script, follow these steps:
Open a text editor (e.g.,
nano
,vim
, or a code editor like VS Code).Copy and paste the script into the editor.
Save the file with a
.sh
extension, for example,print.sh
.Open your terminal and navigate to the directory where the script is saved.
Run the script using the command:
bash print.sh
.
Write a Shell Script to take user input, input from arguments and print the variables.
Certainly! Here's a shell script that takes user input and input from command-line arguments, then prints the variables:
#!/bin/bash # Taking user input echo -n "Enter your name:" read username # Printing the User input using variable echo "Your name is: $username" # Taking input from command line arguments arg1=$1 arg2=$2 # To print the command line arguments echo "Below are the command line arguments: " echo "Argument-1: $arg1" echo "Argument-2: $arg2"
To use this script:
Save it in a
.sh
file, for example,script2-user-input.sh
Open your terminal and navigate to the directory where the script is saved.
Run the script using the command:
bash script2-user-input.sh
It will prompt you for a name.Run the script with an argument:
bash script2-user-input.sh ArgumentValue
.
The script will display the provided user input and argument value:
Write an Example of If else in Shell Scripting by comparing 2 numbers
Certainly! Here's an example of using if-else statements in a shell script to compare two numbers:
#!/bin/bash # To take inputs from usrs echo -n "You are using script to compaire two numbers" echo -n "Enter your first number:- " read num1 echo -n "Enter your second number:- " read num2 if (($num1 == $num2)) then echo "number $num1 and number $num2 Both numbers are equal " elif (($num1 > $num2)) then echo "number $num1 is greater than number $num2" else echo "number $num1 is less than number $num2" fi
In this example, the script compares
num1
andnum2
using if-else statements. It checks whether they are equal or which one is greater. The==
flag is used for equality and>
for greater than. The script then prints the appropriate message based on the comparison.When you run the script, it will output:
I hope you enjoy the blog post!
If you do, please show your support by giving it a like ππ», leaving a comment π¬, and spreading the word π’ to your friends and colleagues π
Subscribe to my newsletter
Read articles from Vinayak Salunkhe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinayak Salunkhe
Vinayak Salunkhe
DevOps Engineer with 8+ Years of Experience | AWS, Azure DevOps, Linux | CKA & RHCSA Certified | Docker+K8S Expert