Creating JAR Libraries for Android: From Scratch (Part 2)
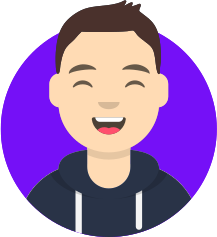
Table of contents
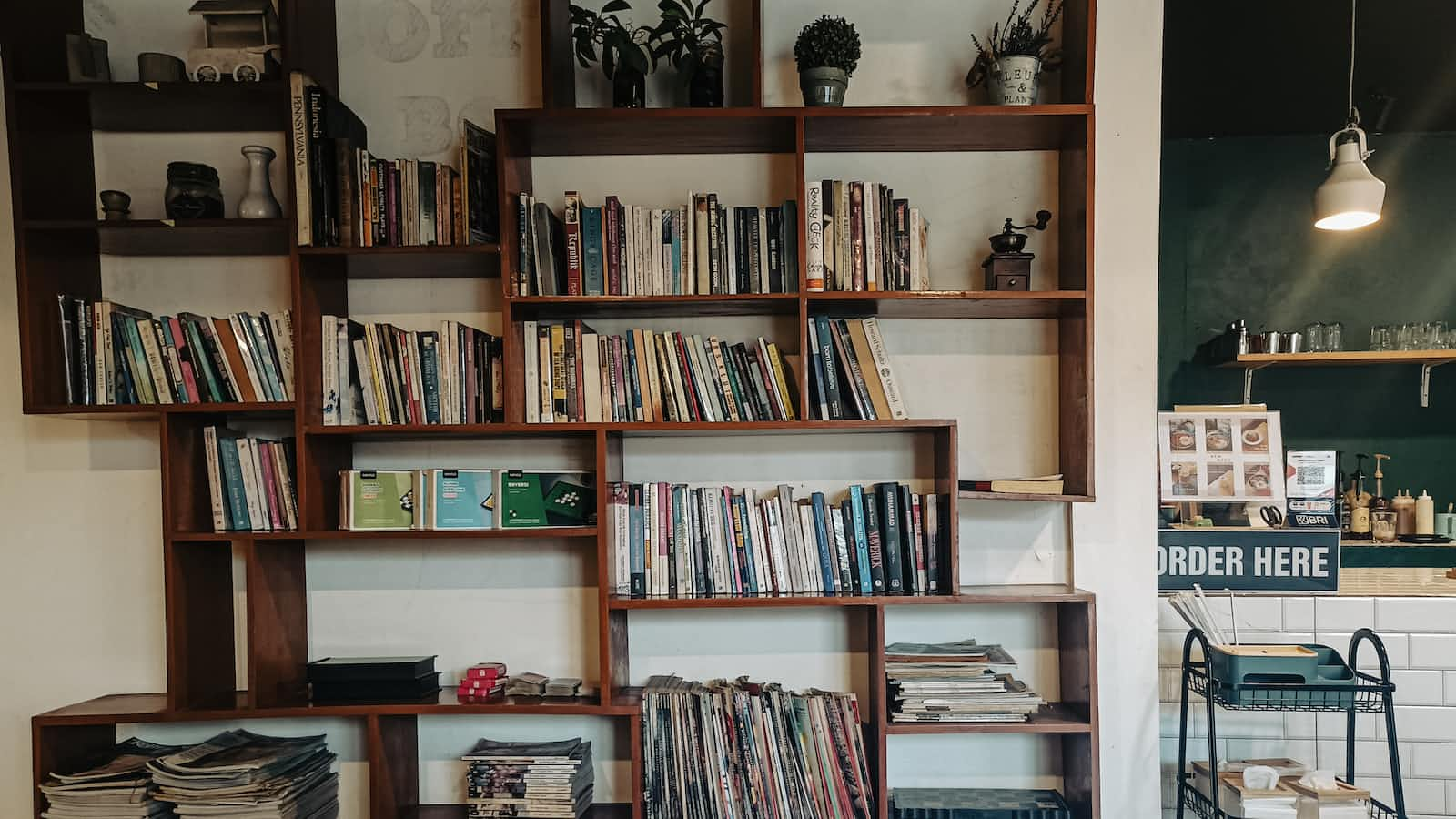
Introduction
In the first part of our series, we embarked on the explorative journey of understanding the pivotal role of dependencies in Android development, laying a strong foundation on the necessity and benefits of creating libraries. As we venture into the second part, we are geared up to take a step further — diving into the practical realm of creating and testing a simple library in Android. This segment promises to be a hands-on guide, steering you meticulously through the nuances of library creation, and setting you on the path to enhancing the efficiency and functionality of your Android projects.
You can find the code to this section here
The idea
We know that there are two types of libraries that we can create for Android apps i.e pure Java libraries (JAR) and android libraries(AAR). Let's go ahead and first try to create a simple Java library that will do basic calculations like add, subtract, and multiply.
Step 1
Create a new project, and inside that project go to file and create a new module.
Step 2
Now, select Java/Kotlin library as the module type, and name the module whatever you'd like the library to be called, I've named it as calculator
Step 3
Let's add the following code to our MyCalculator
class
class MyCalculator {
fun addNumbers(a: Int, b: Int): Int{
return a+b
}
fun subtractNumbers(a: Int, b: Int): Int{
return a-b
}
fun multiplyNumbers(a: Int, b: Int): Int{
return a*b
}
}
Step 4
Now, let's go ahead and build our project and check what the build generates as output.
If everything worked perfectly, you should now be able to see a JAR
file created in the output build/libs folder (change the view to Project from Android).
Step 5
At this point, there are 2 ways to use this jar file in our project.
Manually transferring the JAR file to the new project
The first method is to simply copy this file and paste it inside the libs folder of any app and then import it from the dependencies section as shown below.
While this method works, it will still be cumbersome to create a library for every change and then paste it into our project every time we make a change. A second method is to use a hosted service like jitpack.io which remotely builds and stores these JAR/AAR files and provides it to our app when needed.
Using
Jitpack.io
for our project.To implement this, we need to create a GitHub repo and push our code first. Since this step is relatively straightforward, I won't be adding the steps to it. If you are not familiar with this step, consider checking any online tutorial or ChatGPT.
Now, we need to create a release on Git Hub. To do this, click on the tags tab on the repo home screen
Then go to Releases and click on draft a new release
At this point, you can give any random version name to your project, but it'd be better if you followed the standard conventions of versioning. Now, add any other details like release changelogs or titles and hit publish release
Go to
jitpack.io
Now, all you need to do is add the link to your repository into the search bar and hit enter.
Here, you should now be able to see the version details of the newly added release.
Adding the JitPack repository to your build file.
NOTE: Add the following changes in a new repository that does not have any reference to
calculator
library at all, DO NOT MAKE THESE CHANGES IN THE SAME REPO WHERE YOU ARE CREATING THE LIBRARIES.dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url 'https://jitpack.io' } //make sure this is present in the file } }
Make sure that a reference to the jitpack.io link is available under
dependencyResolutionManagement
.Now add the dependency to the
app.gradle
file as shown on the jitpack.io website and sync everything.dependencies { //other dependencies implementation 'com.github.zuhayr123.From-Scratch:calculator:v1.0.2' }
And that's it!
You should now be able to use the MyCalculator
class anywhere in your project as shown below
class MainActivity : AppCompatActivity() {
var TAG = this.javaClass.canonicalName
override fun onCreate(savedInstanceState: Bundle?) {
var result = MyCalculator().addNumbers(1,1)
}
Okay, now that we have a basic JAR
library implemented, let's try to add a Toast to this library that will be called whenever we call one of its methods. To do this we need to pass context from our MainActivity
to the MyCalculator
class as follows.
import android.content.Context
class MyCalculator(context: Context) {
//rest of the code here to generate the toast
}
But, you must have noticed that now we are not able to access any Android-based classes in our calculator library!
This is because calculator
is a pure Java library that is not supposed to have any references to Android-specific classes.
This is where Android Libraries or AARs come into play, Using AARs we can create libraries that have references to even Android-specific classes like Context.
Conclusion
In today's post, we saw how we can create, use, and host pure Java libraries in Android. In our next post, we'll explore how and when we should consider creating an Android Library instead of a simple Java library.
Till then, happy coding!
Subscribe to my newsletter
Read articles from Abou Zuhayr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
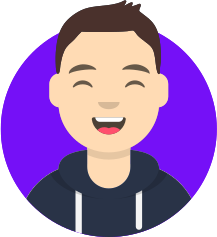
Abou Zuhayr
Abou Zuhayr
Hey, I’m Abou Zuhayr, Android developer by day, wannabe rockstar by night. With 6 years of Android wizardry under my belt and currently working at Gather.ai, I've successfully convinced multiple devices to do my bidding while pretending I'm not secretly just turning them off and on again. I’m also deeply embedded (pun intended) in systems that make you question if they’re alive. When I'm not fixing bugs that aren’t my fault (I swear!), I’m serenading my code with guitar riffs or drumming away the frustration of yet another NullPointerException. Oh, and I write sometimes – mostly about how Android development feels like extreme sport mixed with jazz improvisation. Looking for new challenges in tech that don’t involve my devices plotting against me!