How to Display Snackbars While Listening to a ValueNotifier

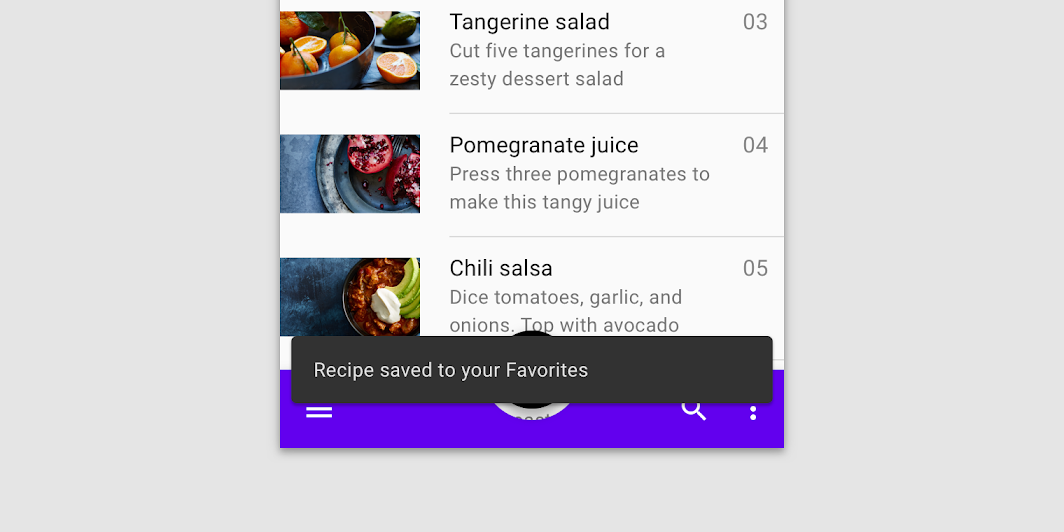
Snackbar is a widget in Flutter that displays a dismissible pop-up message on your application. It is used to show lightweight feedback on any operation to the users. For example: An e-commerce app can display a snackbar when a user adds an item to their shopping cart with a quick link to view the cart.
We can show a Snackbar like this
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: const Text('View Cart'),
action: SnackBarAction(
label: 'Go',
onPressed: () {
//code to go to cart screen
},
),
));
ValueNotifier is a class that extends ChangeNotifier and it holds a single value. ValueNotifier notifies its listeners when the identity of the value it holds changes, not when the state of that changes.
In programming, the identity of an object refers to the memory location or reference to that object and the state refers to data or properties. When we say identity changes, we mean that the object has been replaced with a new one, not that its data has changed or modified. ValueNotifier uses the '==' operator to compare the new value with the old one.
We can create a ValueNotifier like this
ValueNotifier<int> counter = ValueNotifier<int>(0);
We can update the value like this
counter.value++;
We can also listen to the ValueNotifier like this
counter.listen((){
print(counter.value);
}
There's also ValueListenableBuilder that we can use to listen to a ValueNotifier.
As we have understood the minimum knowledge of these two widgets, it's time to learn how can we show a Snackbar while listening to a ValueNotifier. We are going to keep it simple by creating a sample counter app for that.
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final ValueNotifier<int> _counter = ValueNotifier<int>(0);
@override
void initState() {
super.initState();
_counter.addListener(() {
if (_counter.value == 5) {
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar(
content: Text(
'You have reached 5',
),
),
);
}
});
}
void _incrementCounter() {
_counter.value++;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Center(
child: Text(
'Welcome',
style: Theme.of(context).textTheme.headlineMedium,
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
}
Here, we have created a ValueNotifier named _counter. To listen to that ValueNotifier we have added a listener in the initState() method. As we have added a listener to our ValueNotifier whenever the value changes it will get notified and will show the Snackbar when the value is 5.
For a complex scenario, suppose we have called an API and we got an error in response. For that error, we can show a user-friendly message using a snackbar by listening to the type of error, such as network errors, validation errors, or server errors, and then displaying a corresponding snackbar message to inform the user about the issue.
Conclusion:
In this article, I have explained the basic overview on how to show a Snackbar while listening to a ValueNotifier.
I hope this blog will provide you with sufficient information on trying to explore more on Snackbars and Valuenotifiers and how to use them both in more complex scenarios.
If I got something wrong? Let me know in the comments. I would love to improve.
Thank you reading this article. Happy Coding!!!!
Subscribe to my newsletter
Read articles from Inan Mahmud directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Inan Mahmud
Inan Mahmud
Muslim, Flutter Developer, Software Engineer, Manga Reader, Anime watcher