Add and Format Shapes in PowerPoint in C#
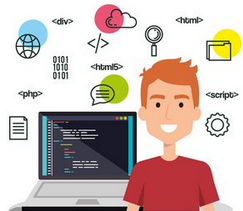
In PowerPoint, shapes are versatile graphical elements that allow you to visually communicate ideas, highlight key points, create diagrams, and add a touch of creativity to your slides. This article is going to share how to add and format shapes in a PowerPoint presentation in C# using a free .NET library.
Install the Free Library
First, it’s necessary to download the free library - Free Spire.Presentation for .NET via the link below or install it directly via Nuget.
https://www.e-iceblue.com/Download/download-presentation-for-net-free.html
Add and Format Shapes in PowerPoint Using C#.
With the free .NET PowerPoint API, you can insert various shapes such as rectangles, hexagons, ellipses, triangles, arrows, etc. at a specified location in a PowerPoint slide through the ISlide.Shapes.AppendShape(ShapeType shapeType, RectangleF rectangle) method. Below is an example of adding 6 different shapes and setting their fill effects.
Sample C# code.
using Spire.Presentation;
using Spire.Presentation.Drawing;
using System.Drawing;
namespace AddShapes
{
internal class Program
{
static void Main(string[] args)
{
//Create a Presentation instance
Presentation presentation = new Presentation();
//Get the first slide
ISlide slide = presentation.Slides[0];
//Append a triangle shape to the slide
IAutoShape shape = slide.Shapes.AppendShape(ShapeType.Triangle, new RectangleF(100, 130, 100, 100));
//Fill the shape with solid color
shape.Fill.FillType = FillFormatType.Solid;
shape.Fill.SolidColor.Color = Color.Cyan;
//Set the outline color for the shape
shape.ShapeStyle.LineColor.Color = Color.White;
//Append an ellipse shape to the slide
shape = slide.Shapes.AppendShape(ShapeType.Ellipse, new RectangleF(280, 130, 150, 100));
//Fill the shape with picture
string picUrl = "bg.jpg";
shape.Fill.FillType = FillFormatType.Picture;
shape.Fill.PictureFill.Picture.Url = picUrl;
shape.Fill.PictureFill.FillType = PictureFillType.Stretch;
//Set the outline color for the shape
shape.ShapeStyle.LineColor.Color = Color.CornflowerBlue;
//Append a heart shape to the slide
shape = slide.Shapes.AppendShape(ShapeType.Heart, new RectangleF(500, 130, 130, 100));
//Add text to the shape
shape.TextFrame.Text = "Heart";
//Fill the shape with solid color
shape.Fill.FillType = FillFormatType.Solid;
shape.Fill.SolidColor.Color = Color.Pink;
//Set the outline color for the shape
shape.ShapeStyle.LineColor.Color = Color.LightGray;
//Append a five-pointed star shape to the slide
shape = slide.Shapes.AppendShape(ShapeType.FivePointedStar, new RectangleF(100, 270, 150, 150));
//Fill the shape with gradient color
shape.Fill.FillType = FillFormatType.Gradient;
shape.Fill.SolidColor.Color = Color.Gold;
//Set the outline color for the shape
shape.ShapeStyle.LineColor.Color = Color.White;
//Append a rectangle shape to the slide
shape = slide.Shapes.AppendShape(ShapeType.Rectangle, new RectangleF(310, 290, 100, 120));
//Fill the shape with solid color
shape.Fill.FillType = FillFormatType.Solid;
shape.Fill.SolidColor.Color = Color.LightGreen;
//Set the outline color for the shape
shape.ShapeStyle.LineColor.Color = Color.Gray;
//Append an arrow shape to the slide
shape = slide.Shapes.AppendShape(ShapeType.BentUpArrow, new RectangleF(500, 300, 150, 100));
//Fill the shape with gradient color
shape.Fill.FillType = FillFormatType.Gradient;
shape.Fill.Gradient.GradientStops.Append(1f, KnownColors.Red);
shape.Fill.Gradient.GradientStops.Append(0, KnownColors.PowderBlue);
//Set the outline color for the shape
shape.ShapeStyle.LineColor.Color = Color.White;
//Save the result document
presentation.SaveToFile("AddShapes.pptx", FileFormat.Pptx2010);
presentation.Dispose();
}
}
}
Using Free Spire.Presentation for .NET, you can also add other elements in PowerPoint presentations, such as inserting tables, creating smart art, and adding charts, images and comments.
Subscribe to my newsletter
Read articles from Jonathon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
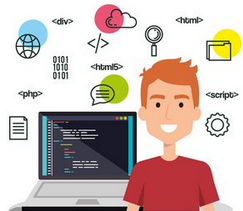