(*, /) Beyond Multiplication and Division - Part1

Table of contents
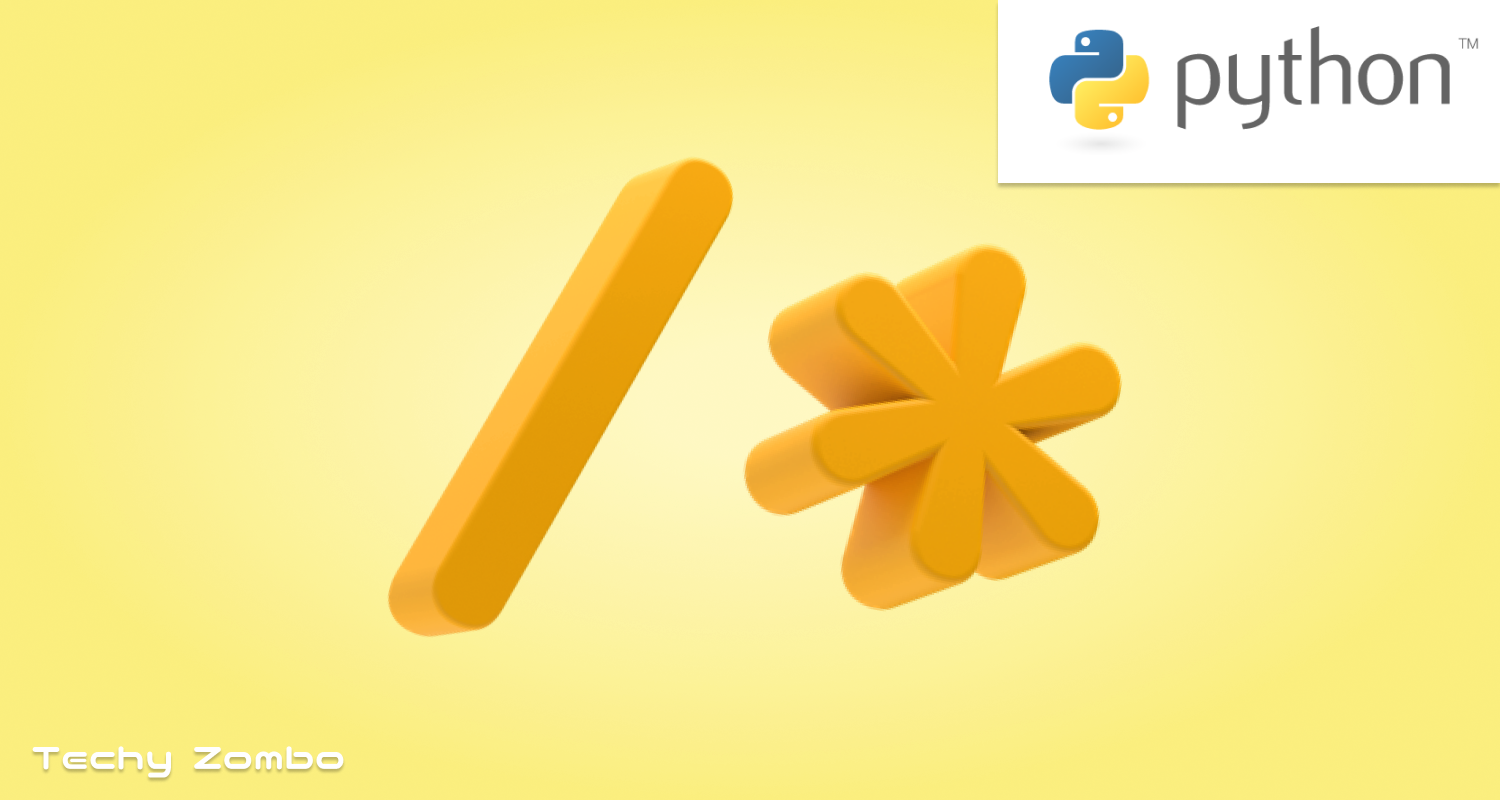
It is commonly known that the asterisk "*" is used for multiplication, while the forward slash "/" is used for division in Python. This is true, but many don't know that these operators can do more.
What's so special about them?
When these operators are used in function definition as parameters, then they get to be special. They are used to show or define the types of arguments that are to be passed to a function. By types, I mean whether the arguments should be keyword arguments, positional arguments, or even both.
Positional arguments are arguments whose values are assigned to parameters based on their position or order in which they are provided.
Keyword arguments are arguments whose values are assigned to parameters, by explicitly specifying or naming the parameters.
The table below shows how each operator determines the type of argument(s) to be passed to a function.
On its left | Operator | On its right |
both positional and keyword arguments | * | only keyword arguments |
only positional arguments | / | both positional and keyword arguments |
\The Asterisk \
Let me explain what the table illustrates starting with the asterisk. When you put an asterisk in a function definition like this:
def display(a, *, b):
"""a function that prints arguments passed to it"""
print(a, b)
...it sets all parameters on its left to be passed as either keyword or positional arguments and those on its right as keyword arguments only. This means that when we want to use our "display" function above, we have to pass "a" and "b" like this:
# Either this way
display("letterA", b="letterB")
# or this way
display(a="letterA", b="letterB")
As you can see here, while we passed "a" by position or keyword, "b" was passed by keyword only. The output of our code will be:
letterA letterB
letterA letterB
And yes, the asterisk is not used when calling the function, it's only for the function definition.
If you try to pass "b" as a positional argument you will get an error.
display("letterA", "letterB")
Traceback (most recent call last):
File "<pyshell#19>", line 1, in <module>
display("letterA", "letterB")
TypeError: display() takes 1 positional argument but 2 were given
Because as far as the Python interpreter is concerned you must pass "b" as a keyword argument and "a" either way.
Now what if you do something like this:
display(b="letterB", "letterA")
"b" and "a" seem to be correctly passed, right? Well, you will get this or similar:
SyntaxError: positional argument follows keyword argument
This is because, by default in Python keyword arguments should always come after positional arguments. But you can do something like this:
display(b="letterB", a="letterA")
Since they are both passed as keyword arguments this code will work. Try it and see!
/ The Slash /
Now let's look at the forward slash. The forward slash in a function definition causes all the parameters on its left to be passed as only positional arguments, and those on its right can be either positional or keyword arguments. Meaning if we define our function like this:
def display(x, /, y):
"""a function that prints arguments passed to it"""
print(x, y)
...the forward slash causes "x" to be passed by position only, whereas "y" can be passed either way. Thus we can call our function like this:
# Either this way
display("this is X", y="this is Y")
# or this way
display("this is X", "this is Y")
Running our code will give us this result:
this is X this is Y
this is X this is Y
If you try to pass "x" as a keyword argument you will get an error.
display(x="this is X", "this is Y")
SyntaxError: positional argument follows keyword argument
So what if you do something like this:
display("this is Y", x="this is X")
It seems as if it will work, but it will raise an error, like this:
Traceback (most recent call last):
File "<pyshell#16>", line 1, in <module>
display("this is Y", x="this is X")
TypeError: display() got some positional-only arguments passed as keyword arguments: 'x'
But how? The thing is "x" was already set to be a positional argument, not a keyword argument.
Bye Bye
I will stop here for now, as I do not want to bore you with a lot of details in one go. In my next article, we will look into using the slash and asterisk together and I will show you our new friend, "\args"*. Until then have fun and take care❤️
Kindly like this article if you learned something new and follow me for more technical articles like this.
Subscribe to my newsletter
Read articles from M'mah Zombo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

M'mah Zombo
M'mah Zombo
I am a born-again Christian and a software engineer at Korlie Limited. I'm an ALX graduate and I'm studying software engineering at Limkokwing University. I like chess ❤️