Installing and Using Click with SQLAlchemy in Python
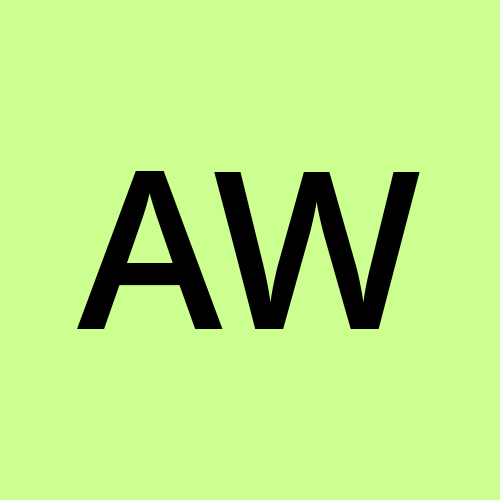
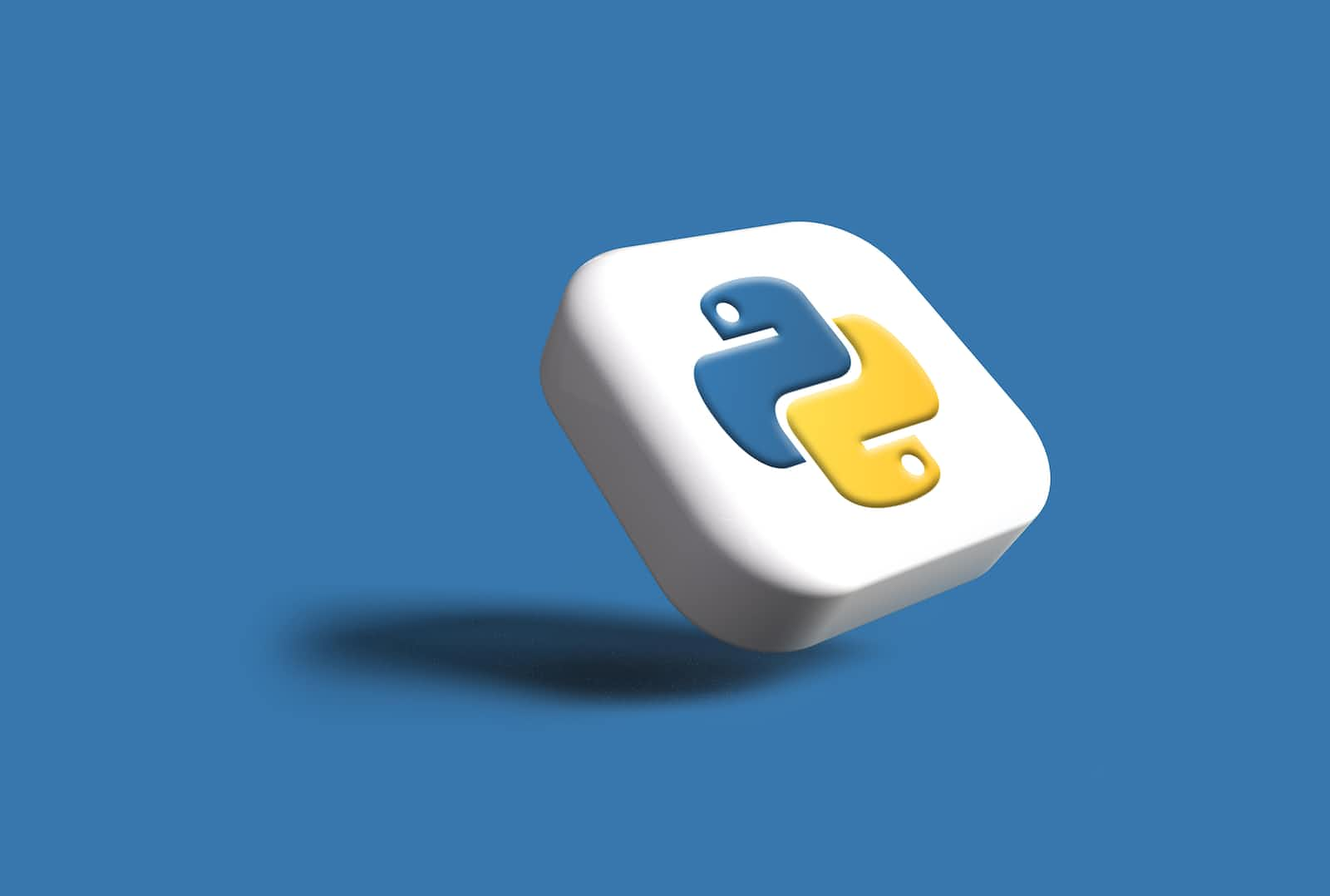
Command-line interfaces (CLIs) are an essential part of many software applications, providing a convenient way to interact with and manage your application from the terminal. Python's Click library is a powerful tool for building CLIs, and when combined with SQLAlchemy, a popular Object-Relational Mapping (ORM) library, you can create robust command-line tools for managing your database-driven applications. Last week, I incorporated Click on my Inventory Management App built using Python and SQLAlchemy (you can check the repo here: Github Repo. In this article, I will explore how to install and use Click with SQLAlchemy in Python, with practical examples.
Prerequisites
Before diving into the installation and usage of Click and SQLAlchemy, ensure that you have the following prerequisites installed:
Python (version 3.6 or higher)
pip (Python package manager)
Installing Click and SQLAlchemy
Let's start by installing Click and SQLAlchemy using pip:
pip install click sqlalchemy
If you have any troubles with the installation, you can troubleshoot using this documentation:
Creating a SQLAlchemy Model
To interact with a database using SQLAlchemy, you need to define a database model. In this example, we'll create a simple SQLite database model for a fictional "Tasks" application. We'll define a Task
model with fields for the task's ID, title, and status.
from sqlalchemy import create_engine, Column, Integer, String, Boolean
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class Task(Base):
__tablename__ = 'tasks'
id = Column(Integer, primary_key=True)
title = Column(String, nullable=False)
done = Column(Boolean, default=False)
# Create a SQLite database file named 'tasks.db'
engine = create_engine('sqlite:///tasks.db')
# Create the 'tasks' table in the database
Base.metadata.create_all(engine)
# Create a Session class for interacting with the database
Session = sessionmaker(bind=engine)
Building a Click CLI
Now that we have our database model set up, we can create a Click CLI to interact with it. Click provides decorators for defining CLI commands and options easily.
import click
@click.group()
def cli():
"""A CLI for managing tasks."""
pass
@cli.command()
@click.argument('title')
def add(title):
"""Add a new task."""
session = Session()
task = Task(title=title)
session.add(task)
session.commit()
session.close()
click.echo(f"Task '{title}' added successfully.")
@cli.command()
def list():
"""List all tasks."""
session = Session()
tasks = session.query(Task).all()
session.close()
if not tasks:
click.echo("No tasks found.")
else:
click.echo("Tasks:")
for task in tasks:
status = "✓" if task.done else "✗"
click.echo(f"{task.id}. [{status}] {task.title}")
@cli.command()
@click.argument('task_id', type=int)
def complete(task_id):
"""Mark a task as completed."""
session = Session()
task = session.query(Task).filter_by(id=task_id).first()
if task:
task.done = True
session.commit()
session.close()
click.echo(f"Task '{task.title}' marked as completed.")
else:
session.close()
click.echo(f"Task with ID {task_id} not found.")
if __name__ == '__main__':
cli()
In this example, we've defined three CLI commands: add
, list
, and complete
, each with its functionality to interact with the database. The click.argument
decorator is used to define command arguments, and the click.echo
function is used to print messages to the console.
Running the CLI
Save the script with a .py
extension (e.g., tasks_cli.py
) and run it using the Python interpreter (in my case, I was using python3):
python3 tasks_cli.py --help
This will display the available CLI commands and their descriptions.
You can now add, list, or mark your task as complete using the cli commands. Here is how you can go about it.
Adding a Task
To add a task, use the add
command:
python3 tasks_cli.py add "Buy groceries"
Listing Tasks
To list all tasks, use the list
command:
python3 tasks_cli.py list
Completing a Task
To mark a task as completed, use the complete
command:
python3 tasks_cli.py complete 1
**
Installing and Using Click with SQLAlchemy in Python**
Command-line interfaces (CLIs) are an essential part of many software applications, providing a convenient way to interact with and manage your application from the terminal. Python's Click library is a powerful tool for building CLIs, and when combined with SQLAlchemy, a popular Object-Relational Mapping (ORM) library, you can create robust command-line tools for managing your database-driven applications. In this article, we will explore how to install and use Click with SQLAlchemy in Python, with practical examples.
Prerequisites
Before we dive into the installation and usage of Click and SQLAlchemy, ensure that you have the following prerequisites installed:
Python (version 3.6 or higher)
pip (Python package manager)
Installing Click and SQLAlchemy
Let's start by installing Click and SQLAlchemy using pip:
bashCopy codepip install click sqlalchemy
Save to grepper
Creating a SQLAlchemy Model
To interact with a database using SQLAlchemy, you need to define a database model. In this example, we'll create a simple SQLite database model for a fictional "Tasks" application. We'll define a Task
model with fields for the task's ID, title, and status.
pythonCopy codefrom sqlalchemy import create_engine, Column, Integer, String, Boolean
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class Task(Base):
__tablename__ = 'tasks'
id = Column(Integer, primary_key=True)
title = Column(String, nullable=False)
done = Column(Boolean, default=False)
# Create a SQLite database file named 'tasks.db'
engine = create_engine('sqlite:///tasks.db')
# Create the 'tasks' table in the database
Base.metadata.create_all(engine)
# Create a Session class for interacting with the database
Session = sessionmaker(bind=engine)
Save to grepper
Building a Click CLI
Now that we have our database model set up, we can create a Click CLI to interact with it. Click provides decorators for defining CLI commands and options easily.
pythonCopy codeimport click
@click.group()
def cli():
"""A CLI for managing tasks."""
pass
@cli.command()
@click.argument('title')
def add(title):
"""Add a new task."""
session = Session()
task = Task(title=title)
session.add(task)
session.commit()
session.close()
click.echo(f"Task '{title}' added successfully.")
@cli.command()
def list():
"""List all tasks."""
session = Session()
tasks = session.query(Task).all()
session.close()
if not tasks:
click.echo("No tasks found.")
else:
click.echo("Tasks:")
for task in tasks:
status = "✓" if task.done else "✗"
click.echo(f"{task.id}. [{status}] {task.title}")
@cli.command()
@click.argument('task_id', type=int)
def complete(task_id):
"""Mark a task as completed."""
session = Session()
task = session.query(Task).filter_by(id=task_id).first()
if task:
task.done = True
session.commit()
session.close()
click.echo(f"Task '{task.title}' marked as completed.")
else:
session.close()
click.echo(f"Task with ID {task_id} not found.")
if __name__ == '__main__':
cli()
Save to grepper
In this example, we've defined three CLI commands: add
, list
, and complete
, each with its functionality to interact with the database. The click.argument
decorator is used to define command arguments, and the click.echo
function is used to print messages to the console.
Running the CLI
Save the script with a .py
extension (e.g., tasks_cli.py
) and run it using the Python interpreter:
bashCopy codepython tasks_cli.py --help
Save to grepper
This will display the available CLI commands and their descriptions.
Adding a Task
To add a task, use the add
command:
bashCopy codepython tasks_cli.py add "Buy groceries"
Save to grepper
Listing Tasks
To list all tasks, use the list
command:
bashCopy codepython tasks_cli.py list
Save to grepper
Completing a Task
To mark a task as completed, use the complete
command:
bashCopy codepython tasks_cli.py complete 1
Save to grepper
Conclusion
In this article, I've explored how to install and use Click with SQLAlchemy in Python to create a command-line interface for managing a simple tasks database. Click's simplicity and SQLAlchemy's power make them an excellent combination for building CLI tools for your database-driven applications. You can extend this example to add more features and options to your CLI as needed.
Happy coding!
Subscribe to my newsletter
Read articles from Austine Jack Were directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
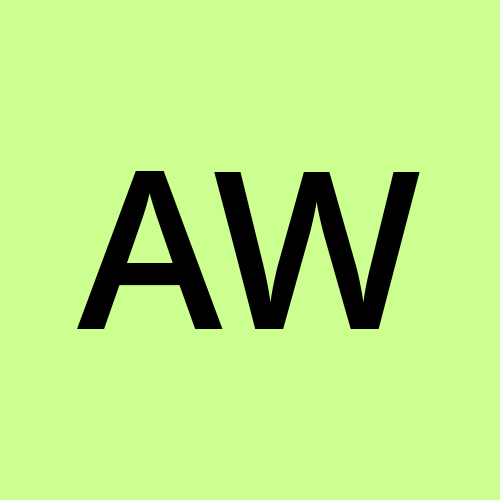
Austine Jack Were
Austine Jack Were
I am Austine Jack Were from Kenya, and I am a full-stack developer! I have a strong background in data structures, Python, C, JavaScript, ReactJS, NodeJS, and Django. I have experience building web applications using JavaScript, HTML, and CSS and creating engaging web interfaces using ReactJS and Tailwind CSS. I also develop efficient backend systems using NodeJS or Python with Django. If you're looking for a skilled developer to bring your ideas to life, please don't hesitate to reach out.