Maximizing Performance: Optimization Techniques for React Applications
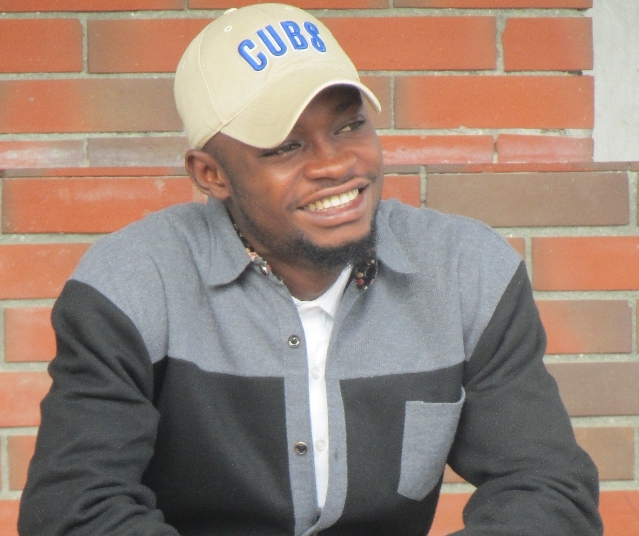
As a software engineer, It is important to maximize the performance of your application. The concept of "code now, improve later" is often referred to as the minimum viable product (MVP) approach in software development. This involves developing the basic features required to meet the initial goals. After these requirements have been reached, the team needs to iterate and improve the software over time. However, developers should also consider that while working on the MVP, it is important to strike a balance between delivering a functional product quickly and ensuring a reasonable level of performance. In this article, I will discuss the importance of maximizing performance, optimization techniques, and the benefits of maximizing performance either at the beginning or the end of software development.
In this digital age, users have come to expect fast, responsive, and efficient web experiences. Slow-loading websites and applications is not only frustrating but can also drive users away. Here are some reasons you should consider optimization as a top priority.
Improved user experience: This translates to a smoother and more enjoyable experience for your visitors leading to increased user satisfaction and engagement. Technically, this can mean increasing the load time of the webpage, caching, accessibility, image optimization, etc.
Competitive advantage: A good user experience can attract new users to your platform and retain existing ones which can set you apart from your competitors with slower and less optimized offerings.
Cost efficiency: This is very crucial for your product as it involves the reduction of expenses associated with hosting and operating your application. Some of the ways to reduce cost may include optimizing the utilization of resources such as efficient data fetching, memory consumption, the use of content delivery networks (CDNs) for faster load times, and lower data transfer expenses.
Better Search Engine Optimization Ranking: An optimized application has an advantage in the world of SEO as search engines such as Google consider page load time and performance when ranking websites which improves the discoverability of your product.
Scalability and Maintenance: As your application grows bigger with the addition of more features, optimization becomes the backbone of maintaining performance. A well-optimized codebase and architecture provide the flexibility needed to scale your application without sacrificing user experience.
Optimization techniques
There are several ways to improve performance in your React application. we'll explore various optimization techniques to enhance the performance of your application.
Code optimization: It is very important to write efficient and high-performance code. The aim is to make the code run faster, consume fewer resources, and be easier to understand and maintain. One fundamental principle of code optimization is to ensure that components render only when necessary by utilizing techniques like
React.memo
,useCallback
orPureComponent
which prevents components from re-rendering when there are changes in props.React Memo: This is a higher-order component(HOC) used to optimize functional components in cases where a component receives the same props but doesn't need to re-render.
import React from 'react'; // Functional component const MyComponent = ({ name }) => { return <div>Hello, {name}!</div>; }; // Memoize the component const MemoizedMyComponent = React.memo(MyComponent); // App component function App() { const [count, setCount] = React.useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <button onClick={increment}>Increment Count</button> <MemoizedMyComponent name="Augustine" /> </div> ); } export default App;
React useCallback: This is a React hook that is used to memoize a callback function to prevent it from being recreated on each render.
import React, { useState, useCallback } from 'react'; function ParentComponent() { const [count, setCount] = useState(0); // A callback function using useCallback const handleClick = useCallback(() => { setCount(count + 1); }, [count]); // The second argument is an array of dependencies return ( <div> <p>Count: {count}</p> <ChildComponent onClick={handleClick} /> </div> ); } function ChildComponent({ onClick }) { return <button onClick={onClick}>Increment Count</button>; } export default ParentComponent;
PureComponent:: This is a base class that is similar to a regular
Component
but it comes with built-in shallow prop and state comparison to prevent unnecessary re-renders. It automatically implements theshouldComponentUpdate
method by performing a shallow comparison of the current and next props and state.import React, { PureComponent } from 'react'; class MyPureComponent extends PureComponent { render() { return <div>Hello, {this.props.name}!</div>; } } class App extends React.Component { constructor(props) { super(props); this.state = { count: 0, }; } increment = () => { this.setState({ count: this.state.count + 1 }); }; render() { return ( <div> <button onClick={this.increment}>Increment Count</button> <MyPureComponent name="Augustine" /> </div> ); } } export default App;
Code splitting: This is the breaking down of your application into smaller, more manageable modules which can significantly reduce the initial load time. This requires you to load components, images, and other assets lazily as needed rather than all at once during the initial load asynchronously.
React.lazy
was introduced in React 16.6 and can be used with React Router as shown below.import React, { lazy, Suspense } from 'react'; import { BrowserRouter as Router, Route, Routes } from 'react-router-dom'; const Home = lazy(() => import('./Home')); const About = lazy(() => import('./About')); function App() { return ( <Router> <Suspense fallback={<div>Loading...</div>}> <Routes> <Route path="/" element={<Home />} /> <Route path="/about" element={<About />} /> </Routes> </Suspense> </Router> ); } export default App;
In the code above, the
Home
andAbout
components are loaded on demand only when the user navigates to the respective routes. This optimization technique is powerful when your React application grows larger with many components and assets.Catching Strategies and Progressive Web Apps(PWAs): These play a significant role in optimizing React applications for performance, offline access, and a better user experience. This can be done by implementing a service worker in your application which is a core part of PWAs to enable caching of assets for offline access.
Server-Side Rendering (SSR): This is a powerful technique that offers significant benefits such as SEO-friendliness, faster initial page loads, and better user experience. Unlike traditional Single Page Applications (SPAs), which render content on the client side, SSR involves rendering the initial HTML on the server and sending it to the client's browser. Most of the heavy duties are been done on the server hereby increasing the performance on the client side.
The server side can implement server-side caching to store the rendered HTML of frequently requested pages using tools like Redis server.
List virtualization: This is an optimized technique used to efficiently render larger lists or grids by rendering only the items that are currently visible in the viewpoint rather than rendering the entire list. There are so many approaches to this using popular libraries such as react-virtualized, react-infinite-scroll-component, and react-window. However here is an example of a custom list virtualization component in a React application that allows you to have complete control over the rendering and behavior of your list:
import React, { useState, useRef, useEffect } from 'react'; const CustomVirtualizedList = ({ items, itemHeight }) => { const [visibleItems, setVisibleItems] = useState([]); const containerRef = useRef(null); // Calculate the number of items that fit in the viewport const itemsInView = Math.ceil(window.innerHeight / itemHeight); // Calculate the total container height const containerHeight = items.length * itemHeight; // Function to update the visible items based on scroll position const updateVisibleItems = () => { if (containerRef.current) { const scrollTop = containerRef.current.scrollTop; const startIndex = Math.floor(scrollTop / itemHeight); const endIndex = Math.min( startIndex + itemsInView, items.length - 1 ); setVisibleItems(items.slice(startIndex, endIndex + 1)); } }; // Attach the scroll event listener and initial visibility update useEffect(() => { if (containerRef.current) { containerRef.current.addEventListener('scroll', updateVisibleItems); updateVisibleItems(); // Initial update } return () => { // Remove the event listener when the component unmounts if (containerRef.current) { containerRef.current.removeEventListener('scroll', updateVisibleItems); } }; }, []); return ( <div ref={containerRef} style={{ height: '100vh', overflowY: 'scroll', position: 'relative', }} > <div style={{ height: containerHeight }}> {visibleItems.map((item, index) => ( <div key={index} style={{ height: itemHeight, borderBottom: '1px solid #ccc', display: 'flex', alignItems: 'center', justifyContent: 'center', }} > {item} </div> ))} </div> </div> ); }; export default CustomVirtualizedList;
Ultimately, the decision on when to maximize performance either at the beginning or at the end of software development varies depending on the project, its requirements, and the development process. Here are considerations on both approaches:
Early Optimization: This approach prioritizes writing efficient code and making performance-conscious architectural decisions right from the start. This will ensure a better user experience, and a more responsive application leading to improved user satisfaction and retention. The disadvantages of this approach can be slower initial development where developers spend more time optimizing code instead of delivering features, over-engineering, and uncleared priorities.
Late Optimization: This approach comes later in the development process typically after the core functionalities of the application have been validated and implemented. This helps in faster initial development and real-world testing that allows you to gather real-world usage data and identify genuine pain points experienced by users. The disadvantage of this approach may lead to a loss of users at the beginning of the project due to performance-related issues and refactoring of code and architecture which can be time-consuming, costly, and potentially the introduction of new bugs.
In conclusion, the striking balance between early and late optimization is essential as it makes sense to deliver a minimum viable product (MVP) quickly without extensive performance optimization.
Subscribe to my newsletter
Read articles from Augustine Igwe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
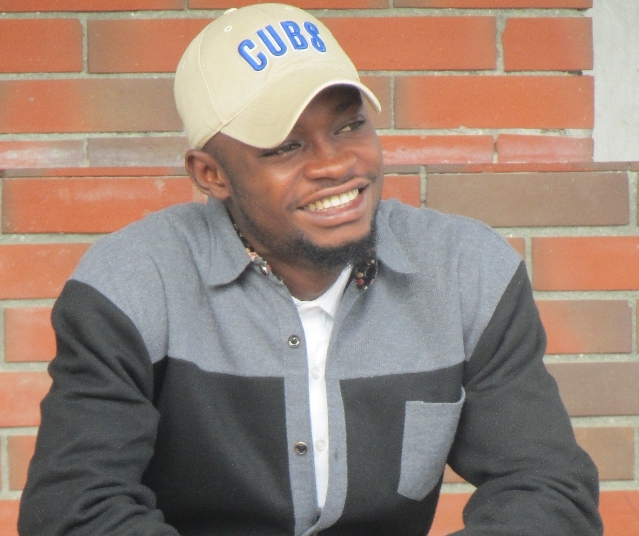