Data Types
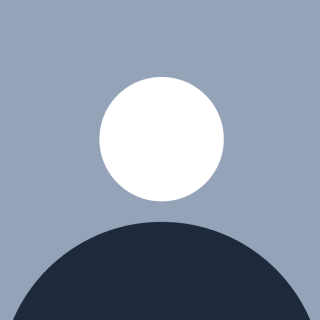
A data type is a way to store and manipulate data using variables. There are two types of programming languages based on data types:
Weakly Typed
In weakly typed languages like Python, JavaScript, Perl etc. same variable can store different data types. You don’t need to specify the data type of a variable.
var a = 3;
a = "Adam";
Strongly Typed
In strongly typed languages like C++, C#, Java, TypeScript etc. you have to specify the data type of a variable e.g., you can’t store a char in an integer variable.
int a = 13;
a = "Adam"; ❌
Categories of Data Types
Data types can be classified as built-in and user-defined data types as well as some other categories.
Built-in data types
int | Integer type used to store numbers e.g., 0, ±1, ±2 . . . |
float | for floating-point values e.g., 3.8, 3.14 etc. |
double | also for floating-point values but with more precision. |
char | Character type used to store alphabets, symbols etc. |
bool | Boolean type for true & false. |
signed | can store both +ve and -ve integers e.g., ±1, ±2 etc. |
unsigned | can only store positive integers e.g., 1, 2, 3 . . . |
User-defined types
class | a data type to encapsulate multiple data members and functions, blueprint for creating objects. |
structure | to define a custom data type that holds multiple variables of different types. |
Derived data types
array | collection of elements on the same data type |
pointer | used to store the memory address of another variable |
Modifier data types
long | increases the range of values that an integer can hold. |
short | decreases the range of values that an integer can hold. |
long long | further increases the range of values that an integer variable can hold beyond what long provides. |
Memory Occupation
You can find memory occupied by a data type using sizeof( ) function in C++.
| BYTES |
| BYTES |
int | 4 | long int | 4 |
float | 4 | long long int | 8 |
char | 1 | short | 2 |
double | 8 | bool | 1 |
long double | 12 |
|
|
Limitations of Data Types
Each data type has some limitations according to memory e.g., in the case of an integer, it can only store 231-1 values.
The size of int is 4 bytes i.e., 4 * 8 = 32 bits. So, using 32 bits we can represent 231 values and 1 bit is for signed values i.e., + or -.
The formula to calculate the number of values that can be represented by 'n' bits is 2n.
bits | values |
1 | 2 |
2 | 4 |
4 | 16 |
8 | 256 |
16 | 65,536 |
32 | 4,294,967,296 |
31 | 2,147,483,648 |
So, 32 bits can represent 4,294,967,296 values but a simple integer also stores negative values, so we can store ±2,147,483,647 (231-1).
- Other data types also have some limitations, try to Google that stuff.
Subscribe to my newsletter
Read articles from Adam Rofayel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Adam Rofayel
Adam Rofayel
Hello world! I'm a Computer Science student who is always craving for new coding skills and share them with you. In starting, I thought coding would be easy, but I was wrong. It's hard af, but it's also fun. It's like being a wizard 🧙♂️, creating things out of thin air. And it's incredibly rewarding to see your code come to life and do something useful. So, if you're thinking about learning to code, don't be discouraged. It's hard, but it's also worth it. And who knows, you might even have some fun along the way.