Exploring the Stream API in Java
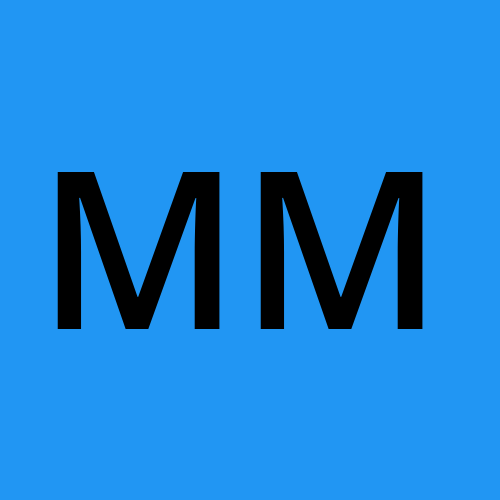
Java's Stream API, introduced in Java 8, brought a significant shift in the way developers handle collections of data. Streams provide a powerful and expressive way to work with data in a functional, declarative style. This article explores the Stream API in Java, explaining its key concepts, benefits, and how to use it effectively.
What is the Stream API?
A stream, in the context of Java, is a sequence of elements that can be processed sequentially or in parallel. It's not a data structure; instead, it's a higher-level abstraction that allows you to perform operations on data, such as filtering, mapping, and reducing, in a concise and readable way.
Key Concepts
Before diving into how to use streams, let's understand some fundamental concepts:
Source: A stream originates from a data source, which can be a collection (e.g., a List or Set), an array, an I/O channel, or even a generator function.
Intermediate Operations: These operations transform one stream into another, allowing you to filter, map, sort, or modify the elements in the stream. Common intermediate operations include
filter
,map
,flatMap
,distinct
, andsorted
.Terminal Operations: A terminal operation produces a result or a side effect and consumes the elements in the stream. It triggers the processing of the stream. Common terminal operations include
forEach
,collect
,reduce
,min
,max
, andcount
.Lazy Evaluation: Streams are evaluated lazily, which means intermediate operations don't execute until a terminal operation is invoked. This allows for efficient processing, especially with large datasets.
Short-Circuiting: Certain operations, like
findFirst
,findAny
, andanyMatch
, can terminate the stream early if a condition is met, improving performance for large streams.
Benefits of Using Streams
The Stream API provides several advantages for Java developers:
Readability: Streams enable you to express data transformations in a more declarative and concise manner, making your code easier to understand.
Immutability: Streams do not modify the source data; they produce new streams with the transformed data, promoting immutability and reducing bugs related to mutable state.
Parallelism: Java streams offer parallel processing with minimal effort, making it easier to take advantage of multi-core processors for improved performance.
Error Handling: Streams handle exceptions gracefully. You can use try-catch blocks within stream operations to handle exceptions without affecting the entire stream.
Working with Streams
Let's look at some common use cases for working with streams.
Stream Creation
Creating a stream from a collection:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
Stream<String> stream = names.stream();
Creating a stream of numbers:
IntStream numbers = IntStream.rangeClosed(1, 10); // Creates a stream of integers from 1 to 10.
Intermediate Operations
Filtering elements:
List<Integer> evenNumbers = numbers
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
Mapping elements:
List<String> upperCaseNames = names
.stream()
.map(String::toUpperCase)
.collect(Collectors.toList());
Terminal Operations
Counting elements:
long count = names.stream().count();
Collecting elements into a list:
List<String> collectedNames = names
.stream()
.collect(Collectors.toList());
Parallel Streams
Processing in parallel:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
long sum = numbers.parallelStream().reduce(0, Integer::sum);
Conclusion
The Stream API in Java is a powerful addition to the language, providing a functional and expressive way to process data. It encourages writing cleaner, more readable code and enables efficient parallel processing. By understanding the key concepts of streams and practicing their use, you can significantly improve your Java programming skills and write more maintainable code.
Happy learning!
Subscribe to my newsletter
Read articles from Minal Madhur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
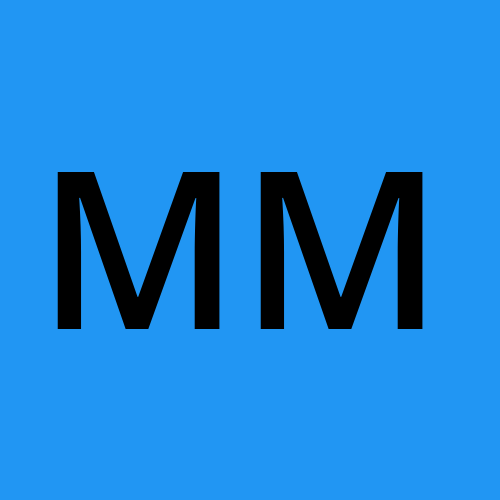