Day 03

2 min read
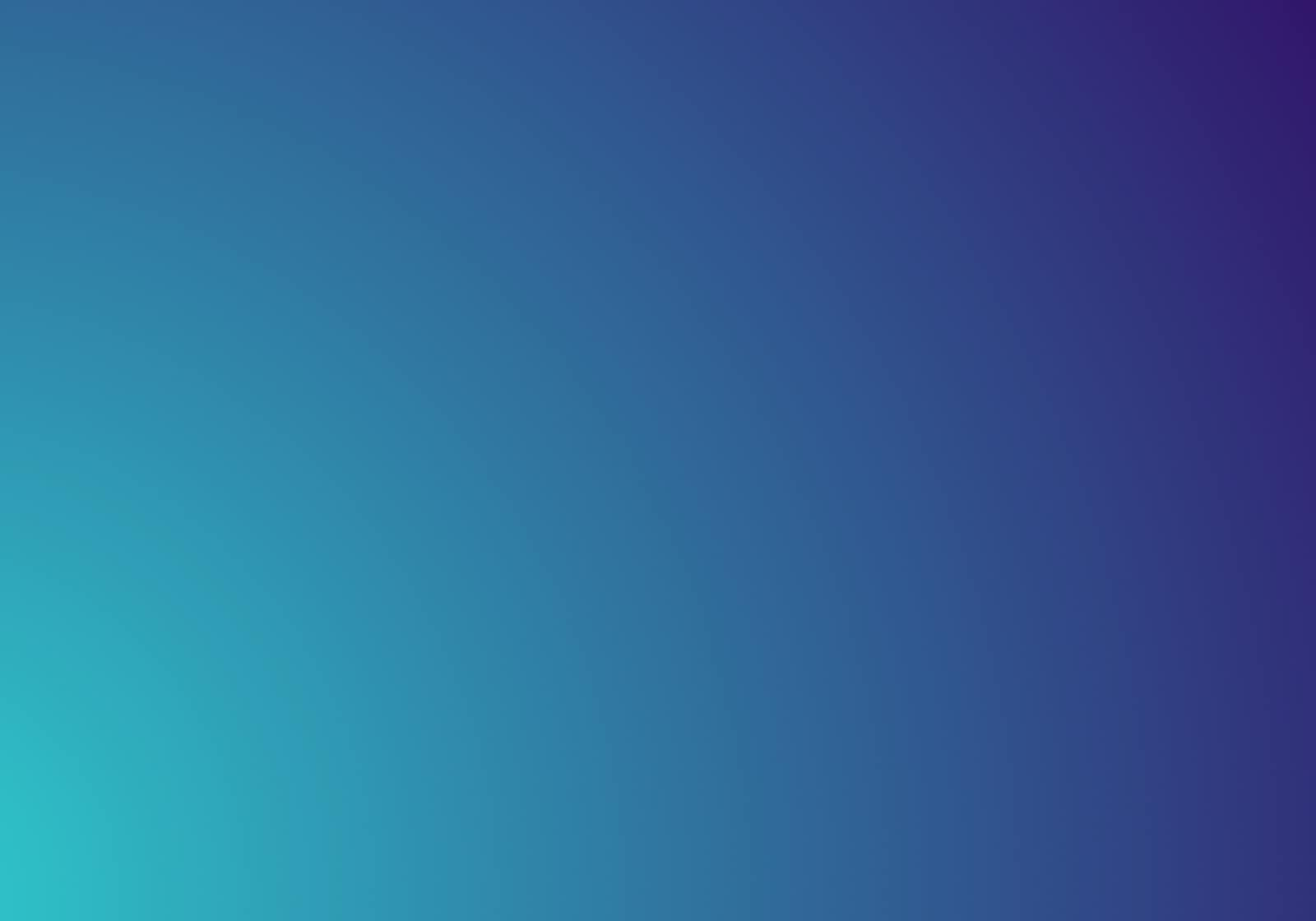
Made a number guessing game in Python. At first, I tried it myself then later I followed the instructions. The second method was a bit more complicated than the first one.
This is my method...
import random
print("Welcome to the guessing number game.")
i = random.randrange(1, 101)
chances = input("Do you want 'easy' or 'hard'?\nType the respective difficulty : ")
x = 0
if chances == 'hard':
x = 5
print("You have 5 attempts.")
elif chances == 'easy':
x = 10
print("You have 10 attempts.")
while x > 0:
question = int(input("Guess the number : "))
if question > i:
print("Too high")
x -= 1
print(f"You have {x} attempts left")
elif question < i:
print("Too low")
x -= 1
print(f"You have {x} attempts left")
else:
print("You guessed right")
break
This is the other method...
import random
print("Welcome to the guessing number game.")
answer = random.randint(1, 100)
turns = 0
easy_turns = 10
hard_turns = 5
def difficulty():
permission = input("Type 'hard' or 'easy' to set the difficulty : ")
if permission == 'hard':
return hard_turns
elif permission == 'easy':
return easy_turns
def guess_number(guess, answer, turns):
if guess > answer:
print("Too high")
return turns - 1
elif guess < answer:
print("Too low")
return turns - 1
else:
print("You are right")
def game():
turns = difficulty()
print(f"You have {turns} attempts left.")
guess = 0
while guess != answer:
guess = int(input("Guess the number : "))
turns = guess_number(guess, answer, turns)
print(f"You have {turns} attempts left.")
if turns == 0:
print("You lose")
return
game()
0
Subscribe to my newsletter
Read articles from Shubham Tomar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
