React Component Types: Functional vs. Class Components
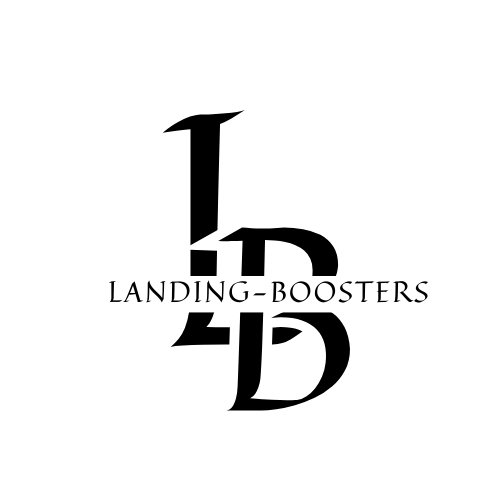
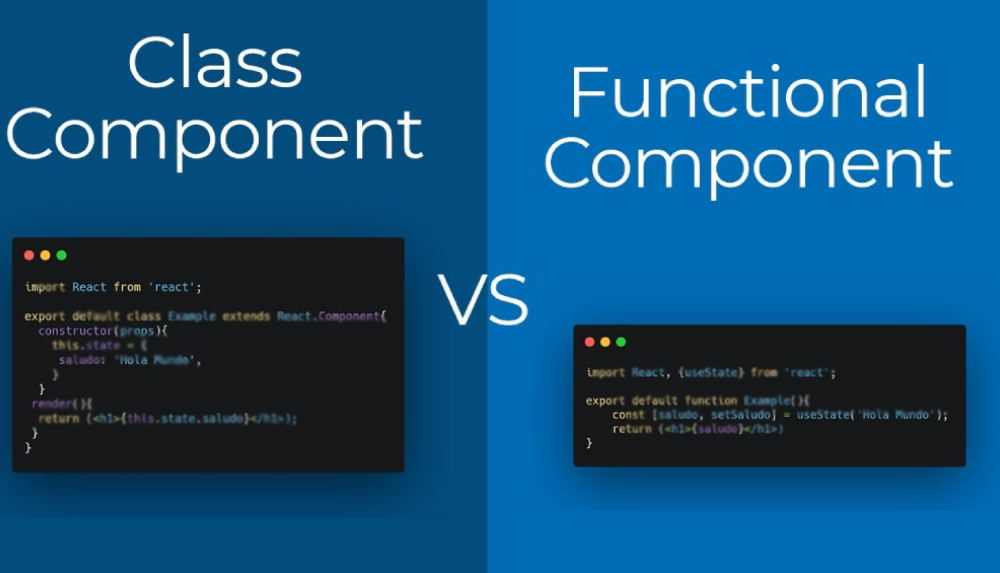
Introduction
React, the popular JavaScript library for building user interfaces, offers two primary ways to define components: functional components and class components. Understanding the differences between these two component types is essential for React developers. In this blog post, we'll explore the distinctions, advantages, and best use cases for functional and class components in React.
Table of Contents
What Are Functional Components?
What Are Class Components?
The Evolution of Functional Components
When to Use Functional Components
When to Use Class Components
Key Differences between Functional and Class Components
State and Lifecycle in Functional Components
State and Lifecycle in Class Components
React Hooks: Bridging the Gap
Performance Considerations
Migrating from Class to Functional Components
Conclusion
1. What Are Functional Components?
Functional components are JavaScript functions that take in props (properties) and return JSX to describe the component's UI. They were traditionally stateless and focused on rendering UI elements based on the props provided to them.
2. What Are Class Components?
Class components, on the other hand, are JavaScript classes that extend React's Component
class. They have the ability to manage state, lifecycle methods, and more. Class components were the primary way to handle state and complex logic in React before the introduction of hooks.
3. The Evolution of Functional Components
React's evolution introduced hooks, allowing functional components to manage state and handle lifecycle actions. This shift has made functional components more versatile and has blurred the line between the two component types.
4. When to Use Functional Components
Functional components are recommended for simpler UI elements or "dumb" components that don't require state or lifecycle management. They are concise, easy to test, and a preferred choice for many developers.
5. When to Use Class Components
Class components are still relevant for more complex components that need to manage their own state and handle lifecycle methods. They are also necessary if you're working with legacy code or third-party libraries that rely on class components.
6. Key Differences between Functional and Class Components
Explore the fundamental differences between these two component types, including syntax, lifecycle methods, and state management.
7. State and Lifecycle in Functional Components
Learn how functional components handle state and lifecycle actions using React Hooks like useState
, useEffect
, and useContext
.
8. State and Lifecycle in Class Components
Understand how class components manage state using this.state
and how they utilize lifecycle methods like componentDidMount
and componentDidUpdate
.
9. React Hooks: Bridging the Gap
Discover how React Hooks have bridged the gap between functional and class components, allowing functional components to manage state and side effects more effectively.
10. Performance Considerations
Consider the performance implications of choosing one component type over the other and how React's reconciliation process plays a role.
11. Migrating from Class to Functional Components
Learn strategies for migrating existing class components to functional components with hooks, making your codebase more modern and maintainable.
12. Conclusion
In conclusion, both functional and class components have their place in React development. The choice between them depends on your project's requirements, complexity, and personal preferences. Functional components with hooks are increasingly becoming the default choice for new React projects due to their simplicity and versatility. Understanding both component types and when to use them is a valuable skill for any React developer.
Subscribe to my newsletter
Read articles from Nitish Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
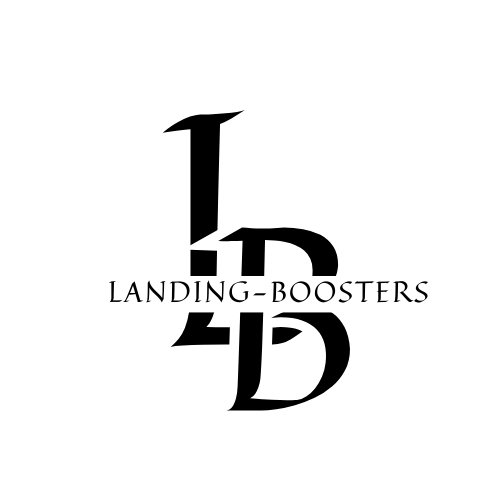
Nitish Prajapati
Nitish Prajapati
Hello & welcome to my Blog! I am Nitish Prajapati , Frontend Developer . I am an a learner, and passionate about multiple Full Stack Web Technologies.