Understanding Rest & Spread Operator in Javascript
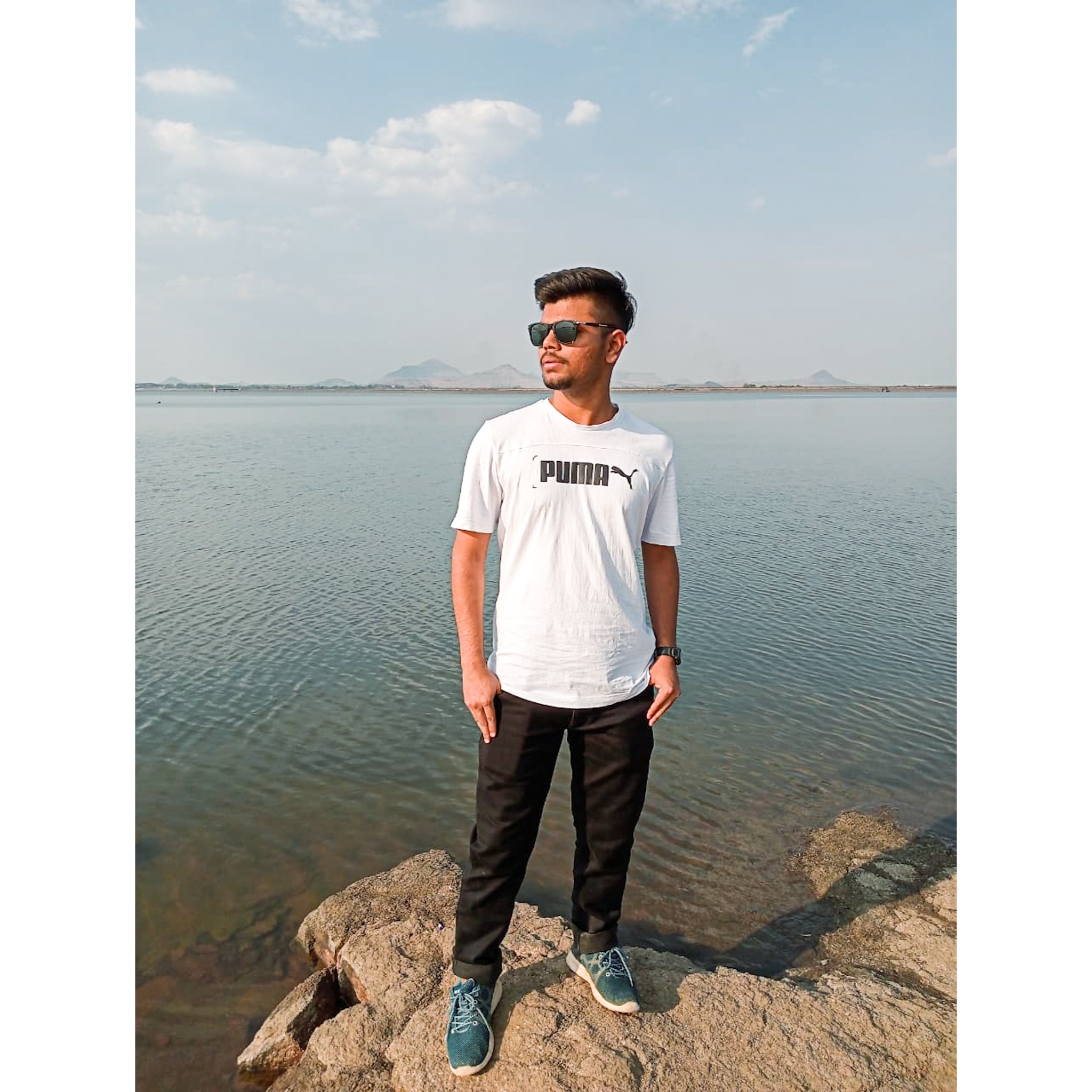
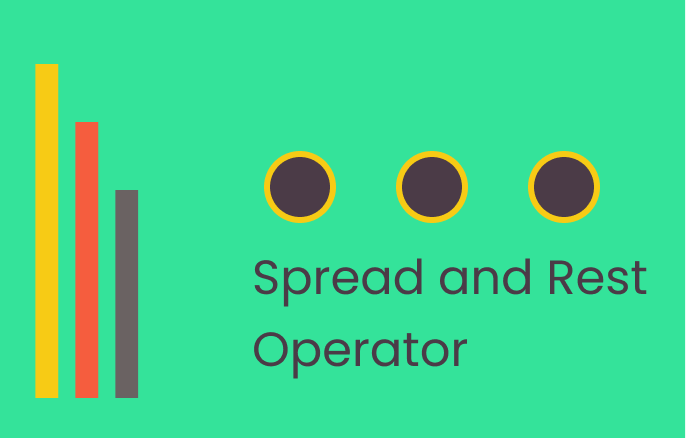
What is Rest operator in JavaScript?
Rest Operator is a feature of advanced Javascript (ES6). The rest operator in JavaScript allows a function to take an indefinite number of arguments and bundle them in an array, thus allowing us to write functions that can accept a variable number of arguments, irrespective of the number of parameters defined.
//Simple Function
function getDatas( a,b,c, ...others) {
console.log( "a is " , a)
console.log( "b is " , b)
console.log( "c is " , c)
console.log( "Others " , ...others)
}
getDatas(10,12,14,100,200,5668);
//Output of above code
a is 10
b is 12
c is 14
Others 100 200 5668
//Rest operator with object
const student = {
Name: "Vineet",
Age: 21,
Hobby: "Coding"
}
const {...rest} = student; //Destructuring the object in ...rest
console.log(rest);
console.log(rest.name);
//Output of above code
{ Name: 'Vineet', Age: 21, Hobby: 'Coding' }
"Vineet"
What is Spread operator in JavaScript?
The Spread operator allows an iterable to expand in places where 0+ arguments are expected. It is mostly used in the variable array where there is more than 1 value is expected. It allows us the privilege to obtain a list of parameters from an array.
//Spread with Arrays
var Code = ["C", "C++", "Javascript", "Python"];
function print(...others)
{
console.log(...others) //returns
}
print(...Code); //Sprad make single copy of an array
//Output of above code
C C++ Javascript Python
//Spread With Objects
const student = {
Name: "Vineet",
Age: 21,
Hobby: "Coding",
}
const newStud = {
...student,
Hobby: "Chilling",
}
console.log(newStud);
//Output of above code
{ Name: 'Vineet', Age: 21, Hobby: 'Chilling' }
I hope you understand the Rest and Spread operator if you reach till here.
Please Like and Follow Vineet Jadhav
for more amazing content
Thanks for Reading... ❤️
Subscribe to my newsletter
Read articles from Vineet Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
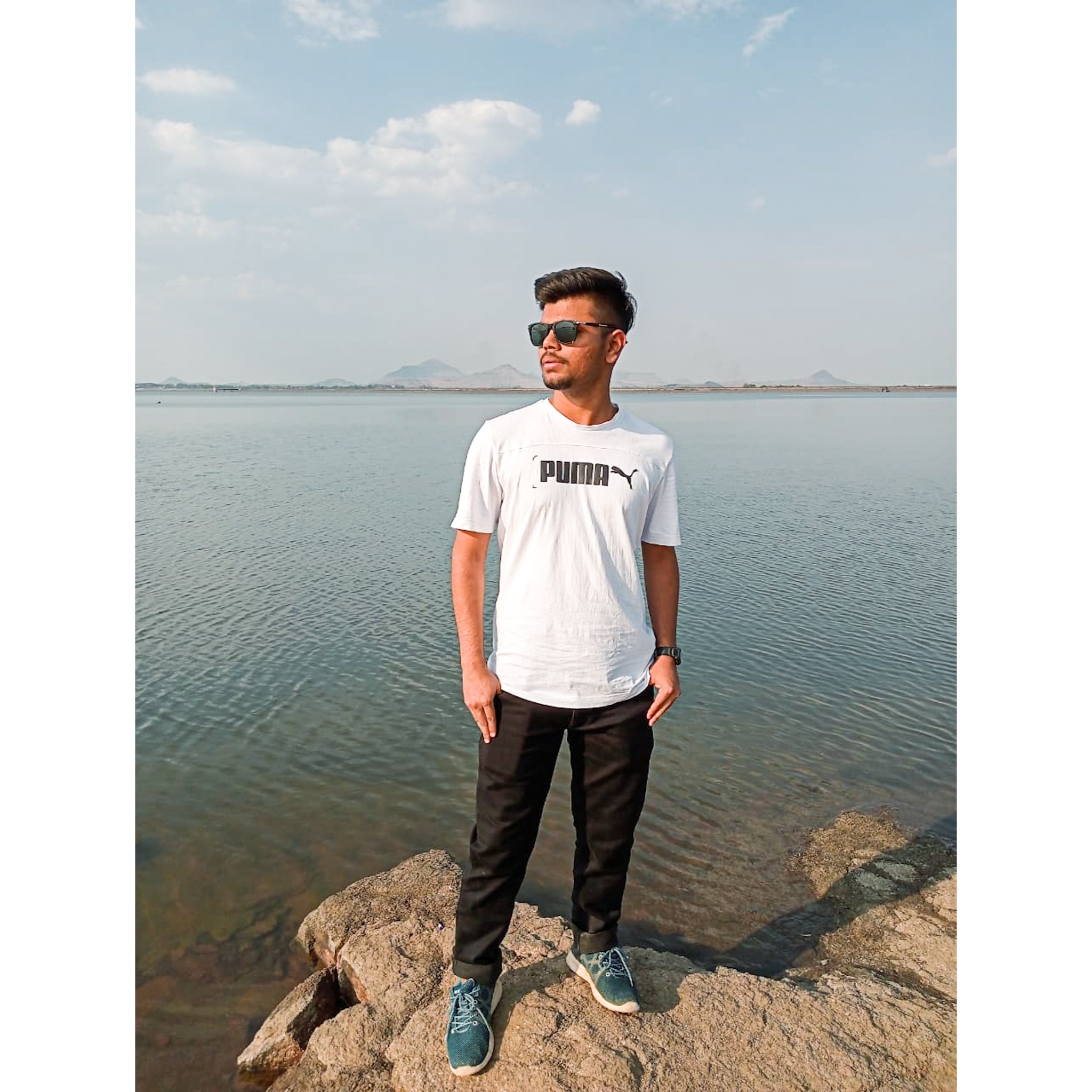
Vineet Jadhav
Vineet Jadhav
"Tech Enthusiast | Frontend Developer | Writer ✍️ Welcome to my corner of the digital realm! I'm a passionate full-stack developer with love for crafting elegant code and building immersive web applications. With expertise in a wide range of technologies including HTML, CSS, JavaScript, and various frameworks like React.js and Node.js, I strive to create seamless and user-centric experiences. Beyond coding, I'm an avid writer who enjoys sharing insights, tips, and tutorials about the fascinating world of web development. My blog posts aim to simplify complex concepts, inspire fellow developers, and foster a vibrant community of learners. Join me on this journey as we delve into the latest trends, discuss best practices, and explore the limitless possibilities of technology. Let's connect, learn, and grow together!"