Deciphering the LCP Matrix to Construct the Lexicographically Smallest String
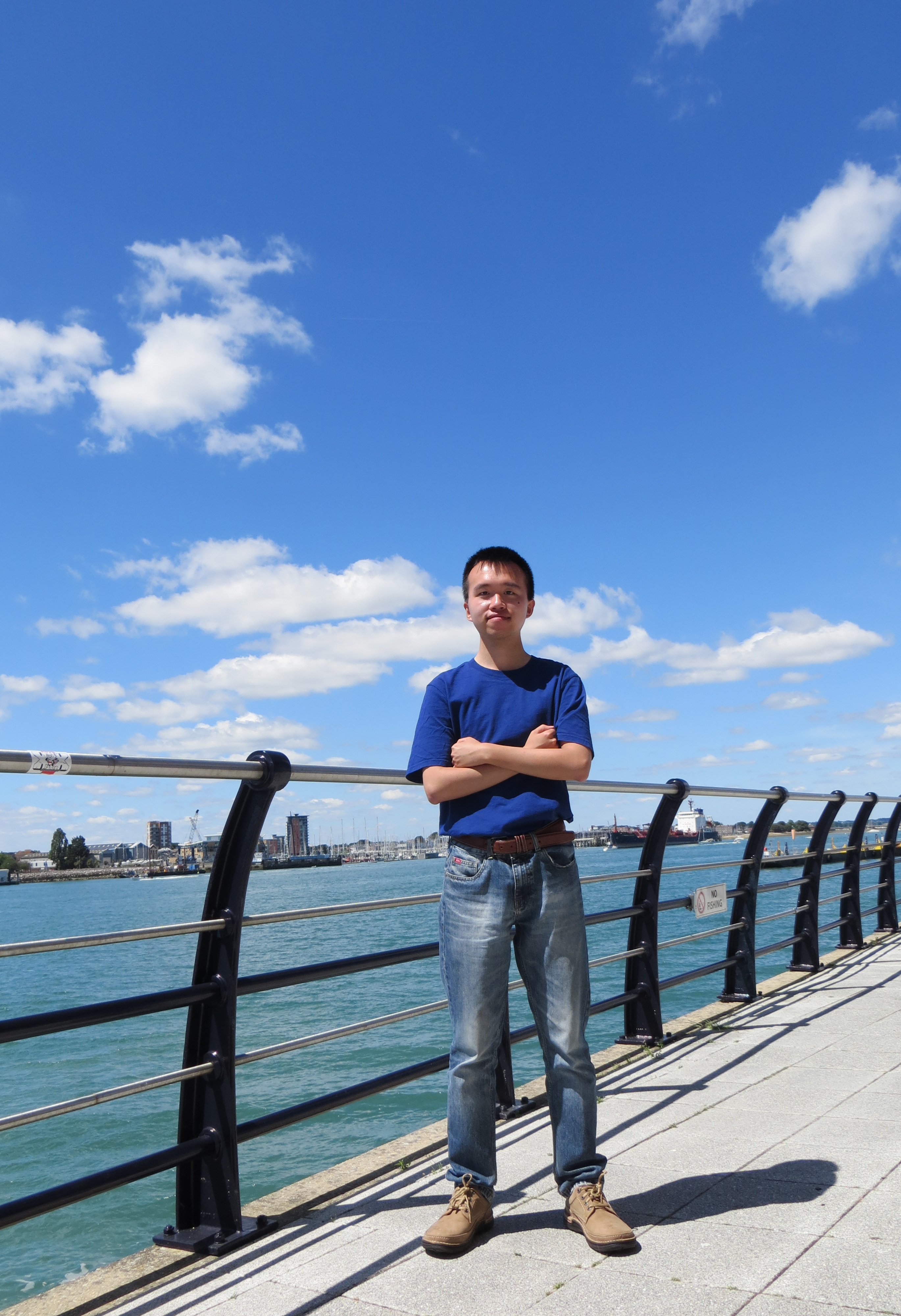
Table of contents
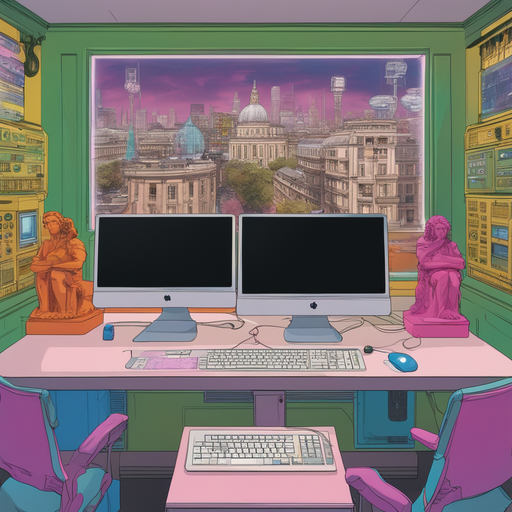
Hey, computer scientists!
Today, we are diving into an intriguing topic: the Longest Common Prefix (LCP) matrix for strings and how it can be harnessed to construct strings with specific characteristics. LCP is a classic problem that challenges your understanding of strings and their lexicographical properties. By the end of this article, you will have a solid grasp of how to solve it, its implications, and its underlying principles.
Understanding the LCP Matrix: The LCP matrix of a string provides a compact representation of how different substrings within that string relate to each other in terms of common prefixes. In simple terms, for a string word
, the entry lcp[i][j]
tells us the length of the longest common prefix between the substrings word[i,n-1]
and word[j,n-1]
.
Problem Statement
From the given LCP matrix, our objective is to derive the lexicographically smallest string in this matrix. The catch is that an empty string is returned if we can not produce one using the LCP matrix.
Solution Approach
Our approach is intuitive yet systematic:
Identifying Unique Characters: We first identify positions in our resultant string that should have the same characters using the LCP matrix. Two positions with a common prefix are assigned the same character.
Constructing the String: Having identified these character positions, we construct our resultant string using 26 unique characters.
Validation: Once our string is formed, we validate it against the LCP matrix. If our constructed string's LCP matrix matches the given one, we are successful! If not, our string is invalid, and we return an empty string.
Code Breakdown
Let's break down the provided code step-by-step:
vector<int> A(n);
: a representation of our resultant string in numeric form. Each entry will eventually correspond to a character.The outer loop (
for (int i = 0; i < n; i++)
) iterates through each position in our string.Within this loop, we identify locations to assign the same character (
if (lcp[i][j] > 0)
). We give them a number, incrementing with each unique character
we discover (++c
).
The check
if (++c > 26)
ensures that we are using at most 26 unique characters. If constructing such a string is impossible, we return an empty string.After identifying characters for each position, we validate our constructed string against the given LCP matrix. The nested loops (
for (int i = 0; i < n; i++)
andfor (int j = 0; j < n; j++)
) help in this validation process.While inside the nested loops, we calculate the LCP value for our constructed string and compare it with the given LCP matrix. If any discrepancy is found (
if (lcp[i][j] != v)
), we know our string is invalid, and we return an empty string.If the constructed string passes the validation, we then transform our numeric representation (
vector<int> A
) into an actual string (res
) using the lineres += 'a' + c - 1
.
class Solution {
public:
string findTheString(vector<vector<int>>& lcp) {
int n = lcp.size(), c = 0;
vector<int> A(n);
for (int i = 0; i < n; i++){
if (A[i] > 0){
continue;
}
if (++c > 26){
return "";
}
for (int j = i; j < n; j++){
if (lcp[i][j] > 0){
A[j] = c;
}
}
}
for (int i = 0; i < n; i++){
for (int j = 0; j < n; j++){
int v = i + 1 < n && j + 1 < n ? lcp[i + 1][j + 1] : 0;
v = A[i] == A[j] ? v + 1 : 0;
if (lcp[i][j] != v){
return "";
}
}
}
string res = "";
for (int c : A)
res += 'a' + c - 1;
return res;
}
};
Complexity Analysis
Time Complexity: The solution runs in O(n^2) time. This is primarily due to the nested loops used for validation against the given LCP matrix.
Space Complexity: The space requirement is O(n) since we are maintaining a single-dimensional vector
A
to represent our resultant string.
Takeaways: Constructing a lexicographically smallest string from a given LCP matrix can be a great exercise in understanding string manipulation, prefix relationships, and lexicographical ordering. The solution offers an intuitive approach to tackle this problem, combining the power of representation using vectors, validation using matrix properties, and finally, string construction.
By understanding this problem and solution, computer science undergraduates can develop a solid foundation in string algorithms, matrix operations, and the principles of lexicographical order. It is a neat trick to impress at coding interviews or challenge discussions!
Happy coding!
Subscribe to my newsletter
Read articles from Haocheng Lin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
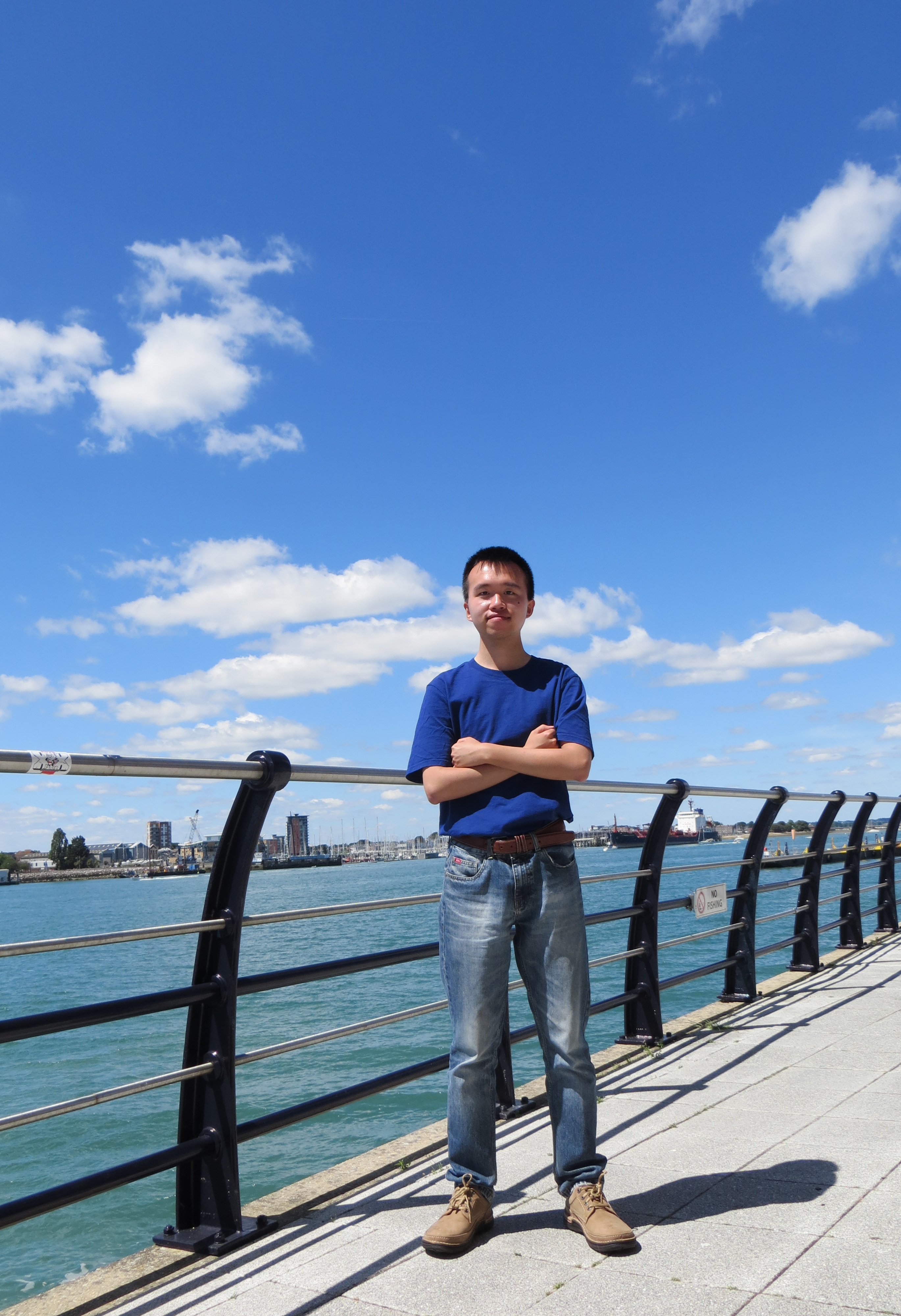
Haocheng Lin
Haocheng Lin
๐ป AI Developer & Researcher @ Geologix ๐๏ธ UCL MEng Computer Science (2019 - 23). ๐๏ธ UCL MSc AI for Sustainable Development (2023 - 24) ๐ฅ Microsoft Golden Global Ambassador (2023 - 24)๐