Routing in Angular

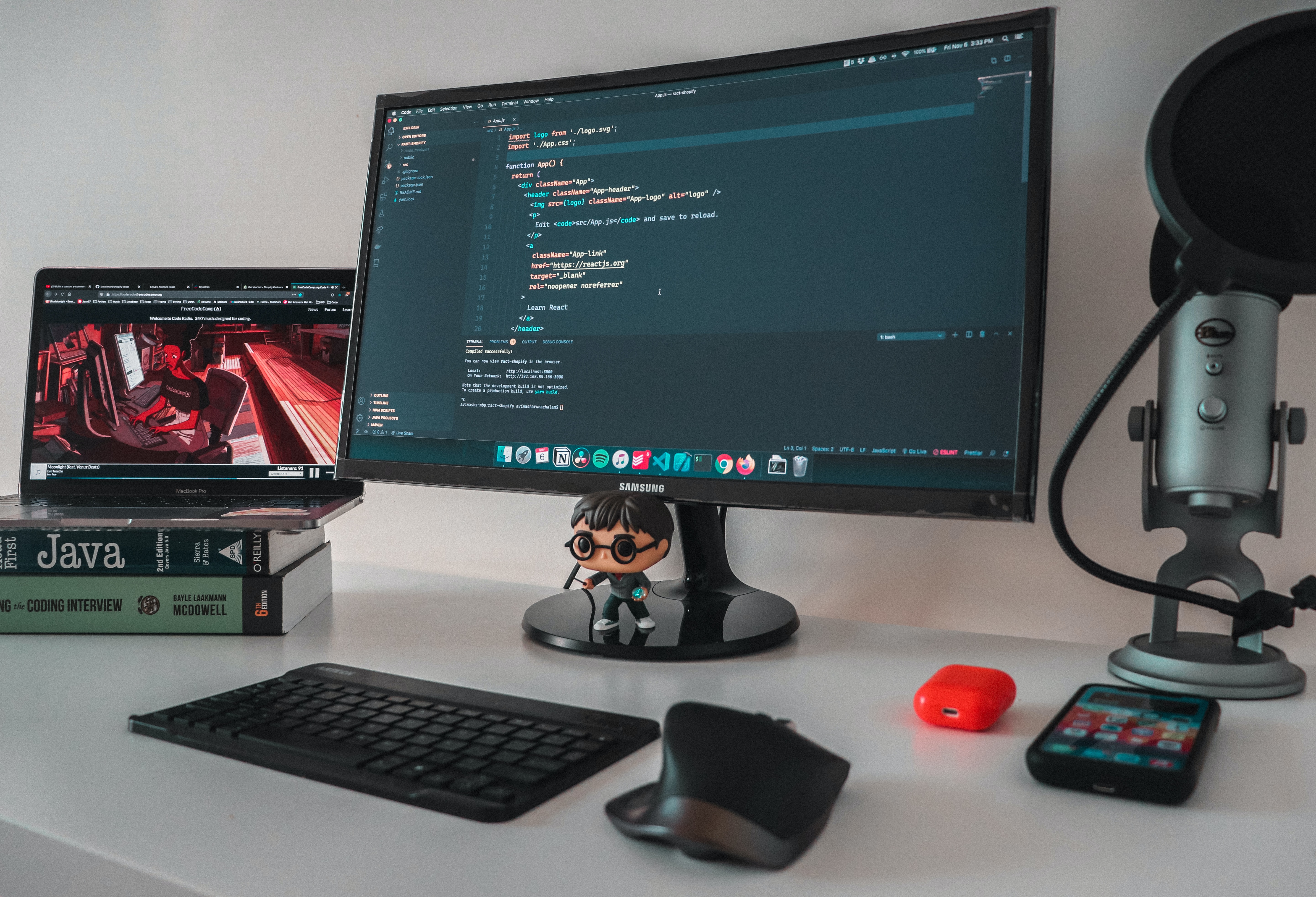
Routing in Angular is a crucial feature that allows you to build single-page applications (SPAs) by defining how different views or components of your application should be displayed based on the URL or user interactions. It enables you to create a seamless and dynamic user experience without requiring full-page refreshes.
By the end of this article, you will learn how to configure routing in Angular.
Prerequisites
For you to follow along with this article, you will need:
TypeScript
A GitHub account
VS Code
Step 1 - Create a new Angular Project
ng new routing-in-angular --routing --defaults
This will create a new project titled ‘RoutingInAngular’.
The ‘ -- routing’ flag takes care of the Angular routing imports into the app.module.ts file:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppRoutingModule } from './app-routing.module'; // CLI imports AppRoutingModule
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule // CLI adds AppRoutingModule to the AppModule's imports array
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Step 2 - Create two components
ng generate component first // or 'ng g c first'
ng generate component second // or 'ng g c second'
Angular CLI will handle the importation of these files into app.module.ts:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { FirstComponent } from './first/first.component';
import { SecondComponent } from './second/second.component';
@NgModule({
declarations: [
AppComponent,
FirstComponent,
SecondComponent
],
imports: [
BrowserModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Step 3 - Update app.component.html
Delete the code inside of the above file and replace it with:
<nav>
<ul>
<li><a routerLink="/first-component" routerLinkActive="active" ariaCurrentWhenActive="page">click to see the first component</a></li>
<li><a routerLink="/second-component" routerLinkActive="active" ariaCurrentWhenActive="page">click to see the second component</a></li>
</ul>
</nav>
Step 4 - Configure app-routing.ts file
Import the two components like this:
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { FirstComponent } from './first/first.component'; //your component can be named whatever you want
import { SecondComponent } from './second/second.component';
const routes: Routes = [
{ path:'first-component', component: FirstComponent },
{ path:'second-component', component: SecondComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Step 5 - Add <router-outlet>
Update the app.component.html file with the <router-outlet> element. This informs Angular to update the view to show the selected component. Something worth noting is that the string url assigned to the routerLink attribute is preceded with a forward slash. Without this element, the client view will not be updated.
<nav>
<ul>
<li><a routerLink="/first-component" routerLinkActive="active" ariaCurrentWhenActive="page">click to see the first component</a></li>
<li><a routerLink="/second-component" routerLinkActive="active" ariaCurrentWhenActive="page">click to see the second component</a></li>
</ul>
</nav>
<router-outlet></router-outlet>
Conclusion
Routing in Angular is a powerful tool for building dynamic, client-side applications with multiple views and navigation between them. It helps create a more interactive and user-friendly experience by handling the rendering of components based on URL changes and user interactions. You can find this code on my GitHub.
Hope this article was helpful.
Happy coding!
Subscribe to my newsletter
Read articles from Kiiru Ryan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Kiiru Ryan
Kiiru Ryan
Full stack Dev