Behind the Scenes of JavaScript: Memory Creation and Code Execution
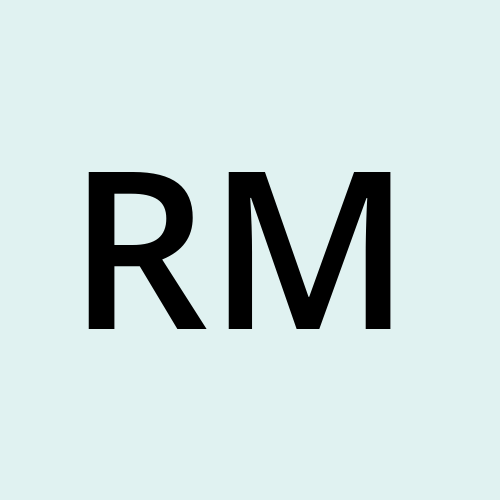
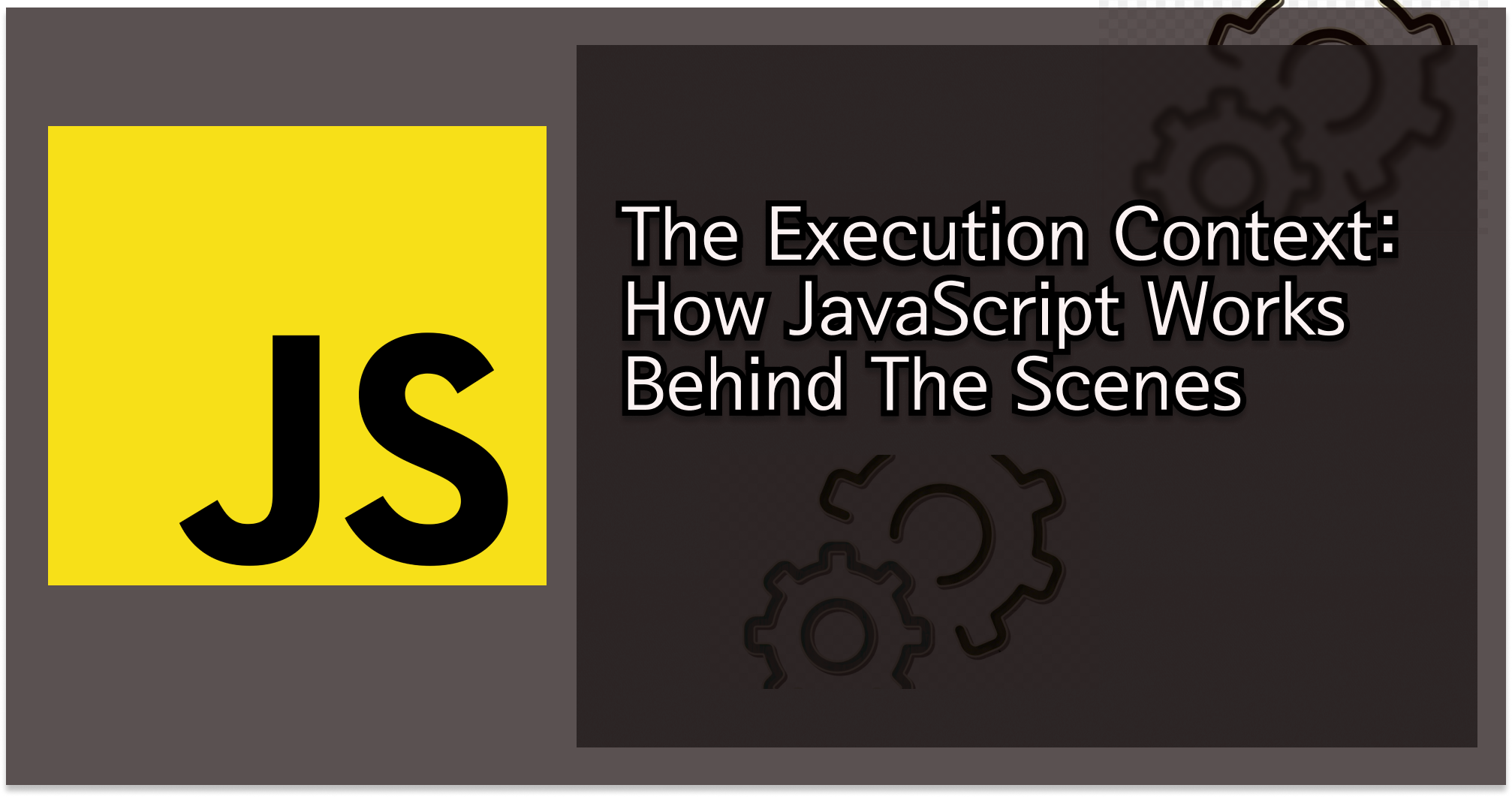
"Did you know that when you unleash a block of JavaScript code into your web page, it doesn't just run from top to bottom like a sprinter in a race? Instead, it goes through a unique two-phase dance, involving memory gymnastics and code acrobatics! Welcome to the intriguing world of how JavaScript code is executed."
Execution Context: Where the Magic Happens:
Before we dive into the intricacies of JavaScript execution, let's understand the concept of the execution context. An Execution context is like a bubble or a container where all the magic happens in JavaScript. It includes everything needed to manage and execute your code effectively. Execution context manages JavaScript code in two phases:
Memory creation phase.
Code execution phase.
Let's Discuss both phases in detail.
Memory creation phase:
The first phase of an execution context is the memory creation phase. During this stage, JavaScript prepares the groundwork by allocating memory for variables and functions, scanning for declarations, and setting up the stage for the code execution. In the memory creation phase, the execution context stores the values of variables and functions in key-value pairs.
Let's take an example of how the memory is allocated to the variables and functions.
var n = 4;
function sum(num) {
var ans = num + num;
return ans;
}
var answer = sum(n);
console.log(answer);
Now as I mentioned execution context is created for this code and memory is allocated for this code. Basically what will happen is JavaScript engine assign the value to each of the variable and function like this:
Memory Creation->
key | value |
---|---|
n | undefined (special keyword in JavaScript) |
sum |
|
answer | undefined |
This is how memory is allocated. The variable gets assigned a special keyword in JavaScript: 'Undefined'. We will talk about it in the next articles until then you can consider Undefined as a special placeholder (not null). In the case of a function, it stores the whole code as you can see in the above table.
Code Execution Phase: The Grand Performance
With the memory allocation complete, JavaScript moves on to the code execution phase. This is where the real performance takes place. JavaScript executes your code line by line, following the order in which it was written.
During the code execution phase, variable values are initialized according to their assignments. As you can see in the first line of the code in memory allocation phase n was declared as undefined. But now 4 is placed in the memory of n.
Now as the code moves to the next line and as you can also see there is nothing to execute here so the code moves to line number 6 and there you can see there is a function invocation or a function is getting called. Functions in JavaScript behave differently than in any other language. Basically whenever there is a function invoked or called a brand new execution context is created. This execution context has also two phases one is memory creation and the other is code execution. Let's see what memory allocation looks like in this:
num | undefined |
ans | undefined |
As you can see we pass the value of undefined to both the variables. Now in the code execution phase. Num gets the value of 4 from the parameter and 4 is stored in the memory of the num variable after this code moves to the next line does its calculation and puts the value of Num + Num in the variable ans. Now the ans has a value of 8 in its memory. So basically in the code execution phase, it will look like this:
num | 4 |
ans | 8 |
Now after this calculation code moves to the next line of the program and as there is a return it will give the whole control back to the previous execution context.
After returning the flow of the code to the previous execution context this execution context is deleted and the code move to the next line and it will print the answer in the console as you can see.
Now you might be wondering:
How does JavaScript manage this?
Now, you might be wondering how javaScript manages all of this you know creating the execution context and creating another and then deleting it. And for large code, it will be very difficult. The answer is it is not difficult to handle as JavaScript uses something called Program stack also known as Call stack. Program stack uses the Stack Data structure to manage all this.
So basically, whenever the JavaScript program is executed, the first execution context, also known as the Global execution context, is pushed onto the stack and then if there is any other execution context is created it will also be pushed into the stack as you can see in the picture.
After these execution contexts finish their work, they will be popped out of the call stack and the call stack will be empty. That's how JavaScript code executes behind the scenes.
Thanks for joining us on this journey through the inner workings of JavaScript execution! We hope you found this exploration enlightening and that it sheds light on how this dynamic language brings your web projects to life.
In my next article, I will dive even deeper into JavaScript's fascinating world by demystifying the concept of "Hoisting".
To stay up to date with our latest insights and updates, be sure to follow me on
Twitter -> Twitter
LinkedIn -> LinkedIn
Github -> Github
I look forward to sharing more exciting discoveries with you soon!
Subscribe to my newsletter
Read articles from Rana MS directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
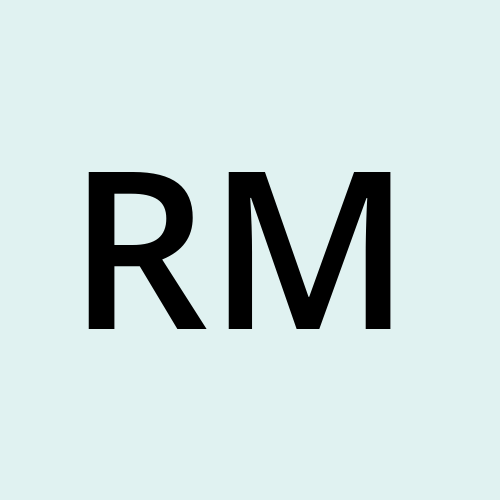