Exploring the Power of ES6 JavaScript Features.
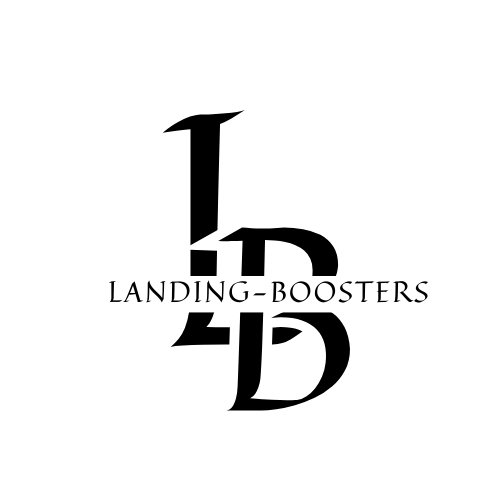
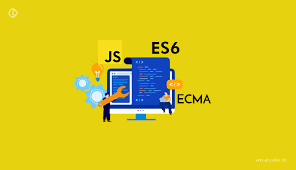
Introduction
JavaScript has come a long way since its inception, and one of the most significant updates to the language was the introduction of ECMAScript 2015, commonly referred to as ES6 (ECMAScript 6). ES6 brought a plethora of new features and enhancements to the language, making it more powerful, concise, and developer-friendly. In this blog post, we'll explore some of the key ES6 features and how they can improve your JavaScript coding experience.
- Let and Const Declarations
ES6 introduced two new ways to declare variables: let
and const
. These declarations are block-scoped, which means they have a more predictable behavior than the old var
keyword. let
allows variable reassignment, while const
creates read-only constants. This improves code predictability and reduces bugs caused by variable hoisting.
javascriptCopy codelet myVar = 10;
const PI = 3.14159;
- Arrow Functions
Arrow functions provide a more concise syntax for defining functions, especially for one-liners. They also capture the surrounding this
value automatically, making them useful for callback functions and event handling.
javascriptCopy codeconst add = (a, b) => a + b;
- Template Literals
Template literals allow you to embed expressions inside strings using backticks ( ), making string concatenation cleaner and more readable.
javascriptCopy codeconst name = "John";
const greeting = `Hello, ${name}!`;
- Destructuring Assignment
Destructuring assignment provides an elegant way to extract values from objects and arrays into individual variables, making code more concise.
javascriptCopy codeconst person = { firstName: "John", lastName: "Doe" };
const { firstName, lastName } = person;
- Spread and Rest Operators
The spread operator (...
) allows you to spread elements of an iterable (e.g., an array) into another array or object. The rest operator does the opposite, collecting multiple values into a single array.
javascriptCopy codeconst arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5];
- Classes
ES6 introduced a more structured way to define classes in JavaScript. Classes provide a blueprint for creating objects, making code organization and inheritance more straightforward.
javascriptCopy codeclass Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
- Promises
Promises simplify asynchronous code, making it more readable and maintainable. They represent the eventual completion or failure of an asynchronous operation.
javascriptCopy codeconst fetchData = () => {
return new Promise((resolve, reject) => {
// Async operation
if (success) {
resolve(data);
} else {
reject(error);
}
});
};
- Modules
ES6 introduced a native module system, allowing you to organize your code into reusable modules. This feature simplifies dependency management and promotes code encapsulation.
javascriptCopy code// In a separate module
export const myFunction = () => {
// Function logic
};
// In another module
import { myFunction } from "./module";
Conclusion
ES6 brought a significant evolution to JavaScript, enhancing its readability, maintainability, and overall developer experience. These features are now widely supported by modern browsers, making them essential tools for any JavaScript developer. By incorporating these ES6 features into your code, you can write cleaner, more efficient, and more maintainable JavaScript applications. So, don't hesitate to embrace the power of ES6 and take your JavaScript skills to the next level!
Subscribe to my newsletter
Read articles from Nitish Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
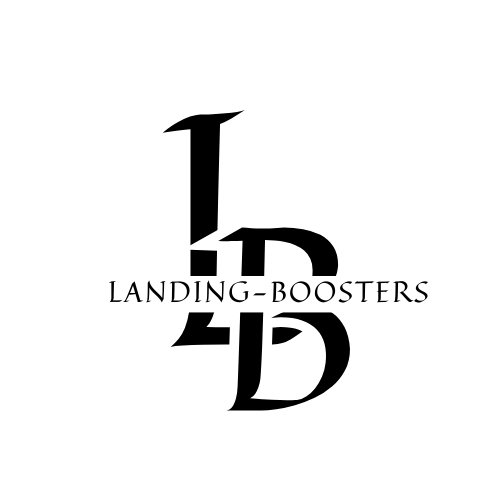
Nitish Prajapati
Nitish Prajapati
Hello & welcome to my Blog! I am Nitish Prajapati , Frontend Developer . I am an a learner, and passionate about multiple Full Stack Web Technologies.