Exploring Recursion: Unraveling the Power of Self-Calling Functions
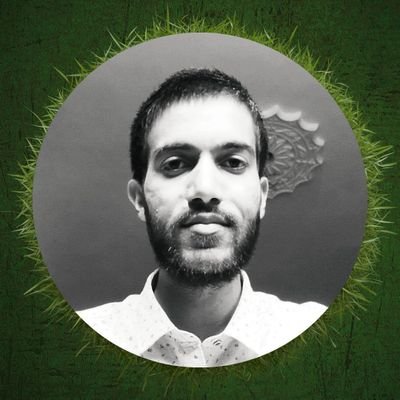
Recursion is a fascinating concept in programming and mathematics. At its core, recursion involves a function calling itself. This might sound like a peculiar idea, but it opens the door to elegant solutions for complex problems.
The Divide and Conquer Approach
Imagine you have a problem that can be divided into smaller, more manageable parts. Recursion allows you to break down these problems into tiny pieces, solve them individually, and then combine those solutions to tackle the larger problem as a whole. It's akin to a divide and conquer strategy for problem-solving.
A classic example of recursion is calculating the factorial of a number. Whether you choose an iterative or recursive approach, the underlying logic remains the same.
The Factorial Example
Let's delve into the example of finding the factorial of 4:
- 4! = 4 x 3!
- 3! = 3 x 2!
- 2! = 2 x 1!
- 1! = 1 x 0!
- 0! = 1
Each factorial calculation boils down to the product of a number and the factorial of that number minus one.
The Recursive Magic
In a recursive approach, when you calculate 4!, it expands like this:
- 4! = 4 x 3!
- 3! = 3 x 2!
- 2! = 2 x 1!
- 1! = 1 x 0!
- 0! = 1
You can observe that we keep repeatedly calculating factorials within factorials. This continues until we reach the base case.
The Crucial Base Case
Here's a vital concept: To avoid infinite recursion, you must establish a base case or an exit condition. In the case of factorial calculations, when the number reaches 1, you return 1. This base case signals the end of the recursive chain.
The Code Behind Recursion
Here's a simple example of a recursive factorial function in C:
int factorial(int n) {
if (n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
The Recursive Journey
For the factorial of 4, you start with factorial(4)
. It then calls factorial(3)
, which calls factorial(2)
, and so on, until factorial(1)
is reached.
- factorial(1)
returns 1
- factorial(2)
returns 2 * 1 = 2
- factorial(3)
returns 3 * 2 = 6
- factorial(4)
returns 4 * 6 = 24
One by one, the calls are removed from the stack memory, until you get the final result of 24.
And there you have it - the power and beauty of recursion, a fundamental concept that unlocks creative problem-solving in the world of programming.
Subscribe to my newsletter
Read articles from Aqib Javid Bhat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
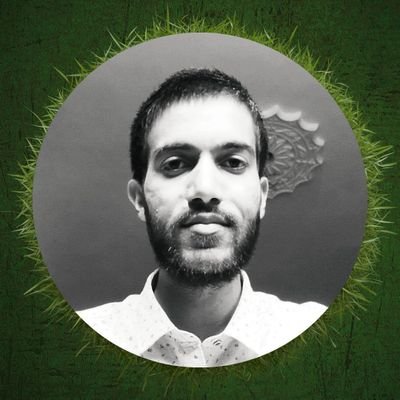
Aqib Javid Bhat
Aqib Javid Bhat
Computer Applications (Major) - Student at GDC, Anantnag In transition from Medical Science to Computer Science I love learning new technologies and I'm a curious about knowing how things work underneath the hood. I love getting involved in global communities and networking.