Implementing a model for Google authentication with Django
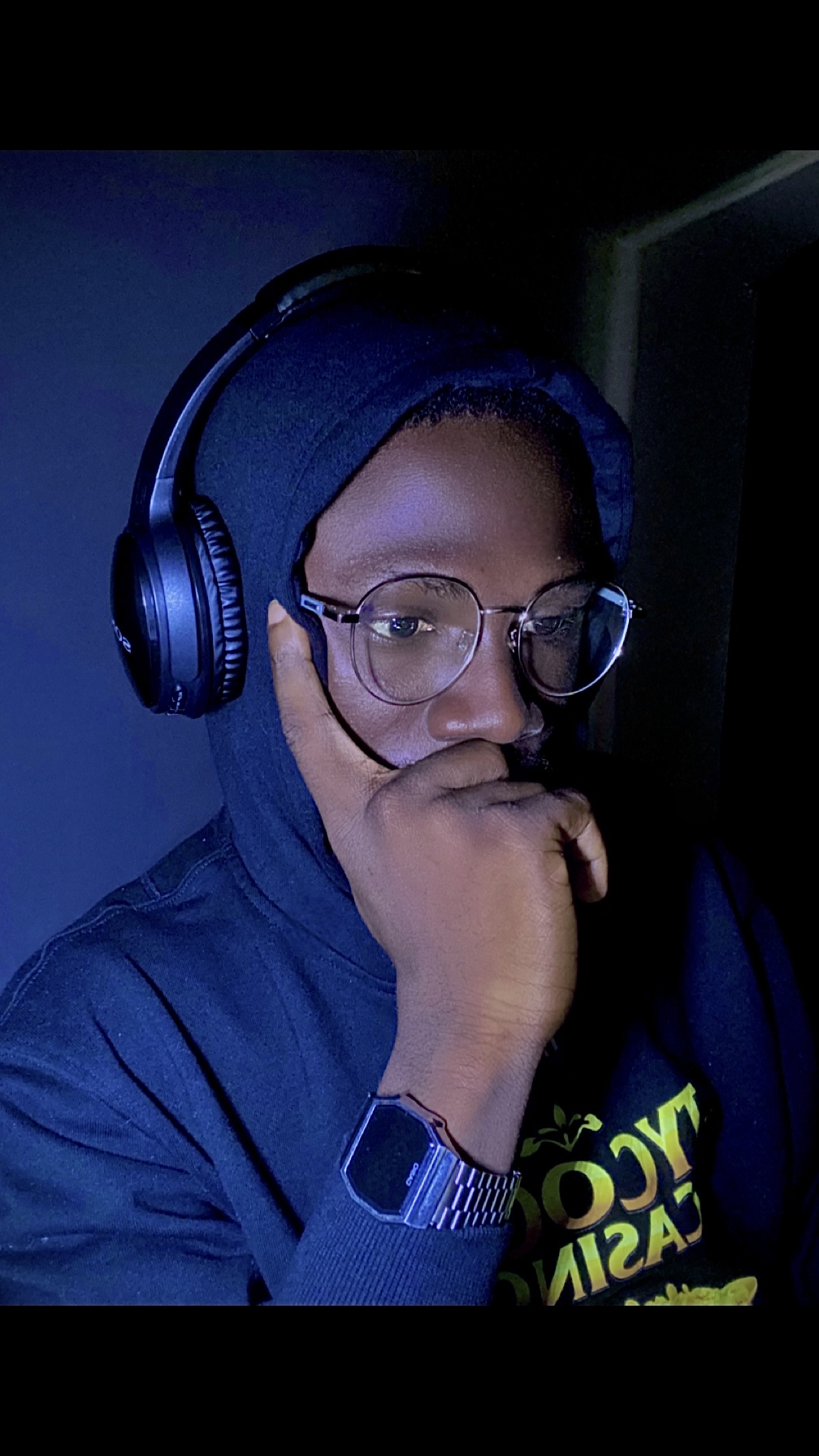
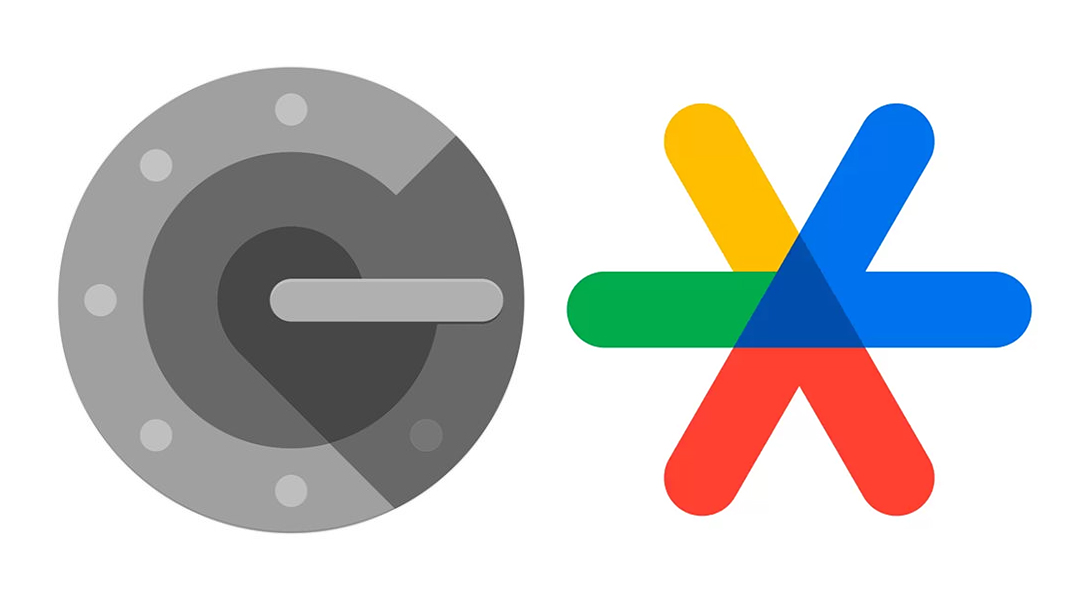
To implement a model for Google authentication with Django, you generally don't need a custom model specifically for Google authentication. Instead, you can use Django's built-in User model or a custom user model, and then use third-party packages like django-allauth
and django-rest-auth
for authentication and social authentication.
Here's a step-by-step guide on how to set up a model for Google authentication in Django:
Create a Django Project and App (if you haven't already):
django-admin startproject project_name cd project_name python manage.py startapp app_name
Configure Your Project's Settings:
In your project's
settings.py
, configure the authentication backends:AUTHENTICATION_BACKENDS = ( 'django.contrib.auth.backends.ModelBackend', 'allauth.account.auth_backends.AuthenticationBackend', # if using allauth )
Make sure you have
'django.contrib.auth'
and'allauth'
in yourINSTALLED_APPS
.User Model:
You can either use the built-in
User
model provided by Django or create a custom user model. If you choose to create a custom user model, you can do so like this:# models.py from django.contrib.auth.models import AbstractUser class CustomUser(AbstractUser): # Add any additional fields you need here pass
Then, update your project settings to use your custom user model:
AUTH_USER_MODEL = 'app_name.CustomUser'
Install and Configure django-allauth:
Install
django-allauth
using pip:pip install django-allauth
Follow the configuration steps mentioned in the previous response for configuring
django-allauth
.Create Views and URLs:
Create views and URLs for user registration, login, and profile views using
django-allauth
. You can also create views and URLs for handling Google authentication using the built-in views provided byallauth.socialaccount.providers.google
.views
. Refer to the previous response for an example of URL configuration.User Serialization:
If you're building a RESTful API, create serializers for your user model (either the default
User
model or your custom user model). You can use Django Rest Framework (DRF) serializers for this purpose. Example:# serializers.py from rest_framework import serializers from .models import CustomUser class CustomUserSerializer(serializers.ModelSerializer): class Meta: model = CustomUser fields = ['id', 'username', 'email', 'first_name', 'last_name', ...]
API Views:
Create API views for user registration, login, and profile views. You can use DRF's generic views or create custom views based on your requirements.
Testing and Deployment:
Test your Google authentication flow using tools like Postman or by building a frontend application. Ensure that your Google OAuth2 credentials are properly set up in the Google Developer Console.
Customization:
Depending on your project's requirements, you may need to customize the behavior of the authentication flow, user registration, or user data storage. django-allauth provides a lot of customization options to fit your needs.
By following these steps, you can implement Google authentication in your Django project using the existing User model or a custom user model along with third-party packages like django-allauth
and django-rest-auth
.
Subscribe to my newsletter
Read articles from Micheal David directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
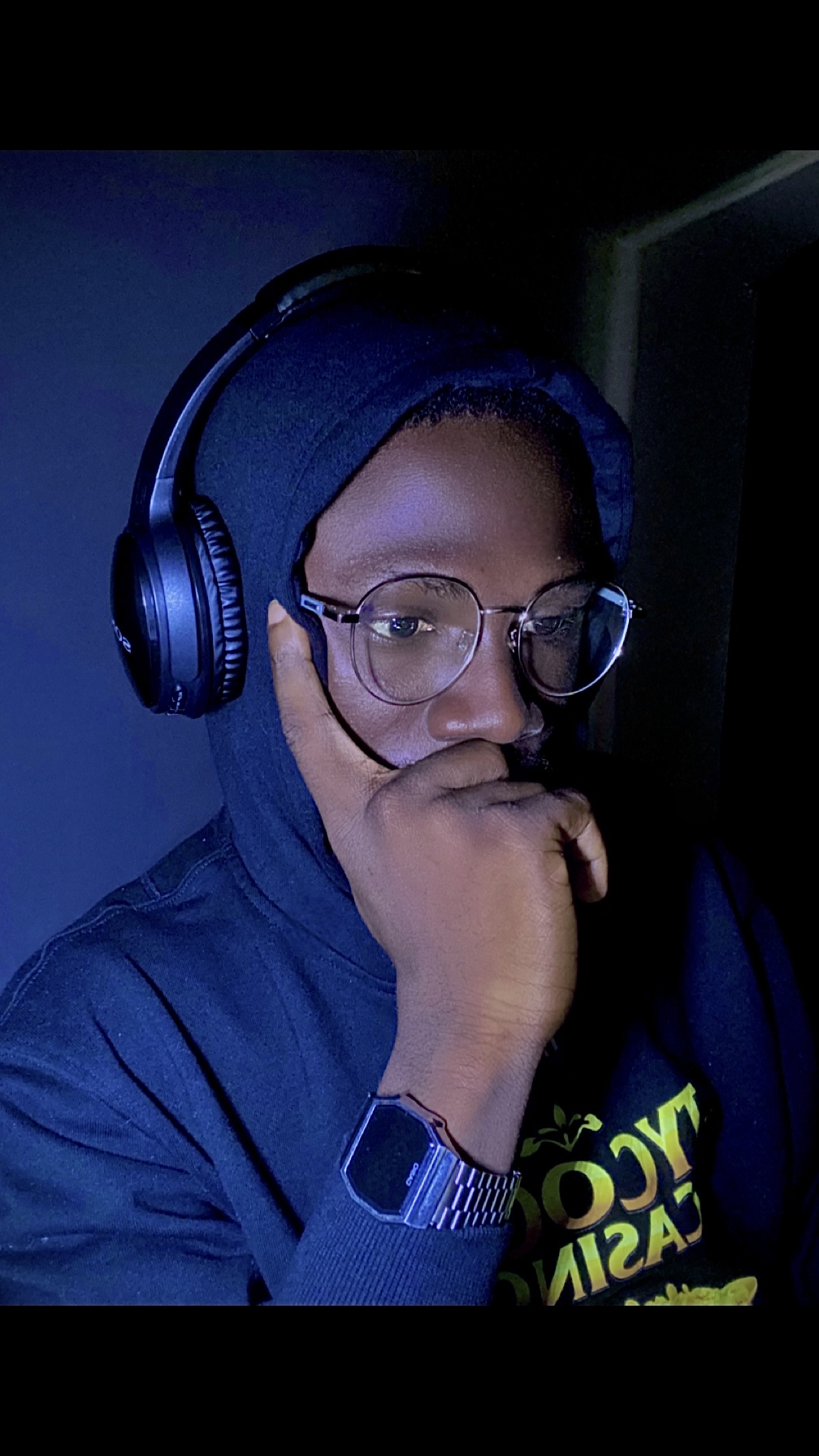
Micheal David
Micheal David
I’m a Software & Machine Learning Engineer, I write technical articles and I play chess.