C-Programming Operators and Keywords
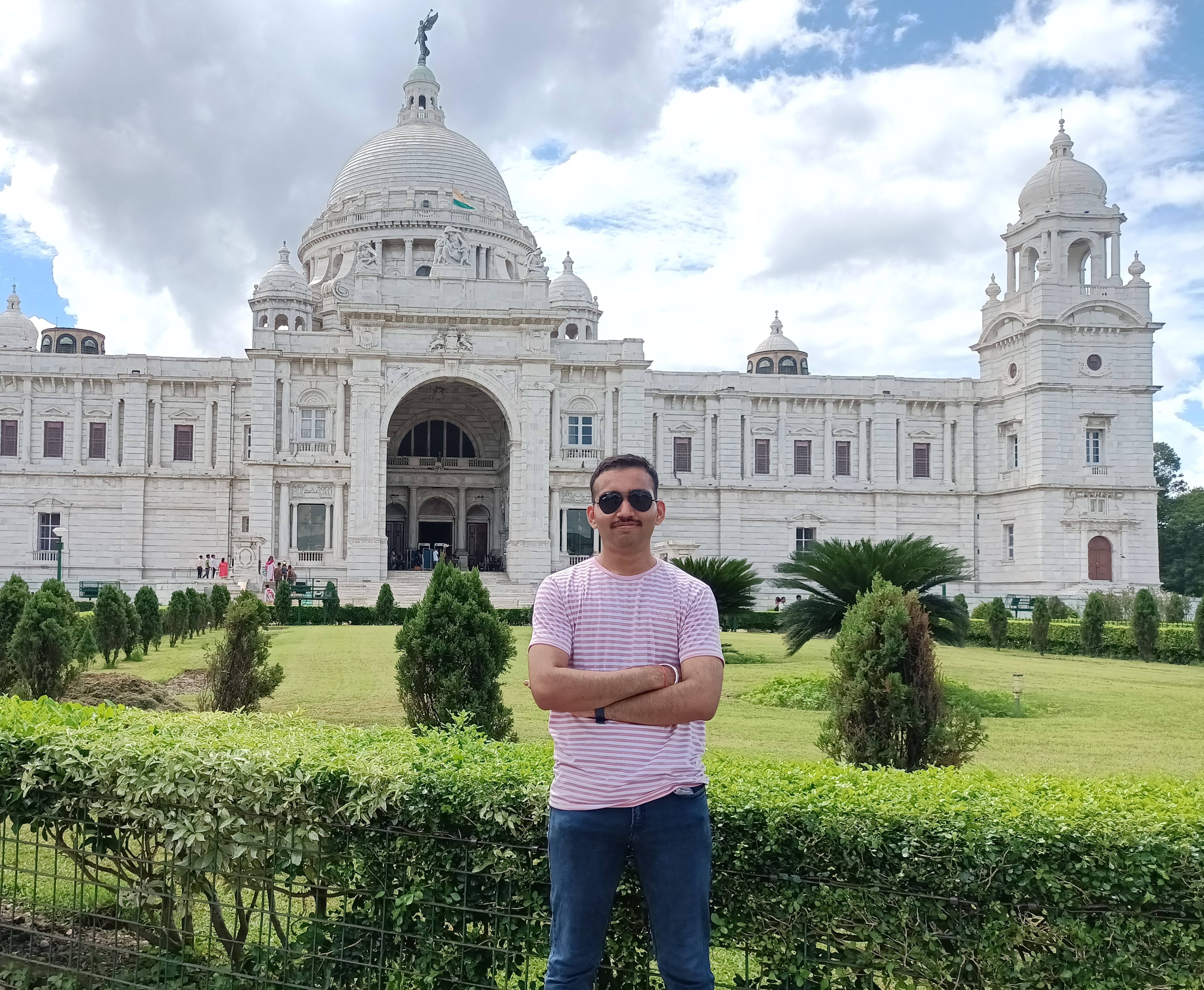
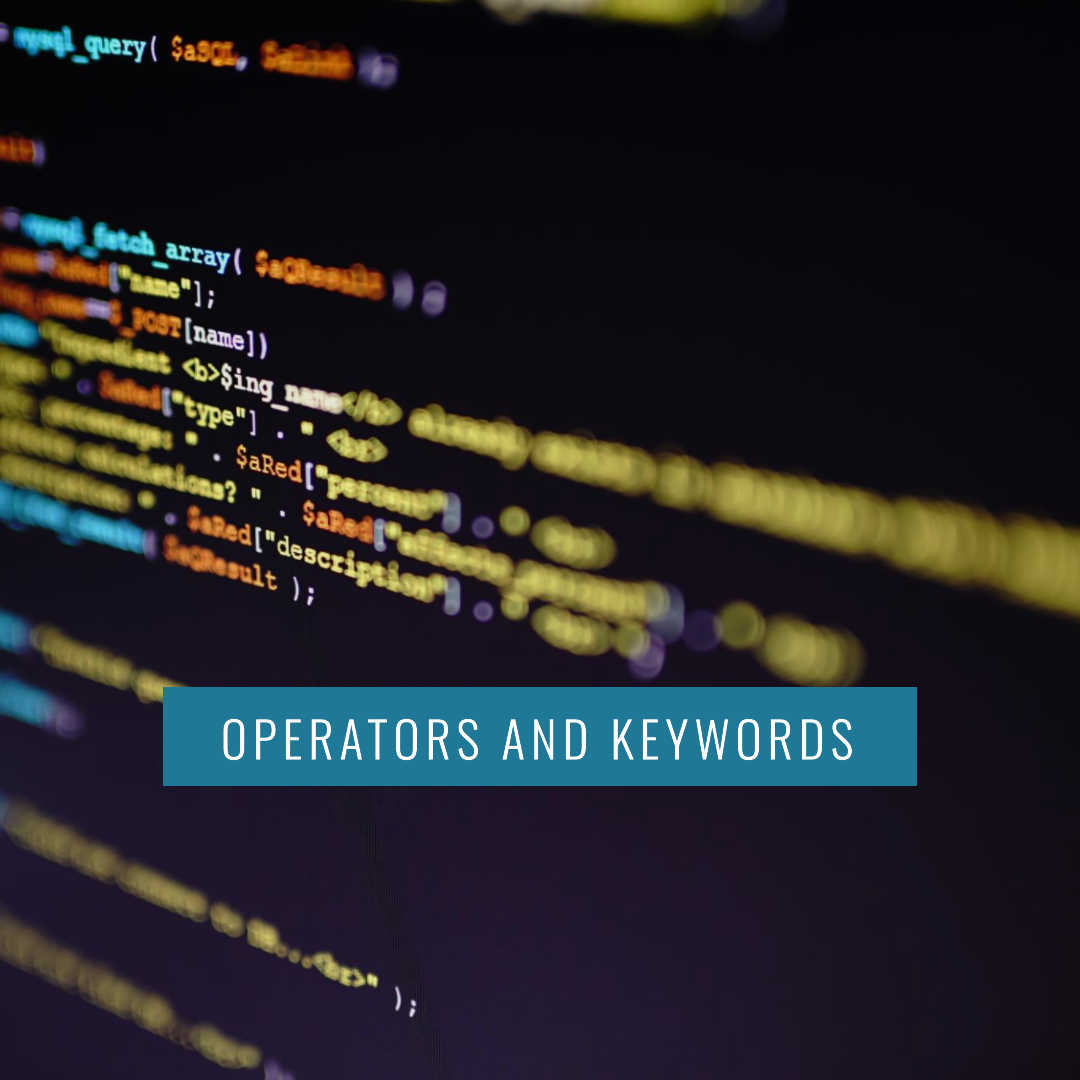
Operator
An operator is a symbol that performs some operation on one or more operands. Operators are used to assign values, compare values or perform calculations with values.
Arithmetic Operators
Relational Operators
Logical Operators
Keywords (or) Reserved Words
Keywords or reserved words are words that have a special meaning in a programming language. They are reserved by the compiler and cannot be used as variable names, function names, class names, etc.
Keywords can be classified as:
Pre-defined data types
char
int
float
double
void
Modifiers
short
long
signed
unsigned
Qualifiers
const
volatile
Storage classes
auto
static
register
extern
Control Structure
i
f
else
while
do
for
switch
goto
case
default
break
continue
return
User-defined data type
struct
union
enum
typedef
sizeof
sizeof()
Words used in C programs are either keywords or identifiers.
Identifiers
Identifiers are names given to entities in a program like variables, functions, constants, etc. They are used to identify these entities.
Some rules for identifiers in C are:
An identifier must start with the letter A-Z, a-z or underscore ( _ ).
After the first character, identifiers can contain letters, digits and underscore.
Identifiers are case-sensitive. age and Age are two different identifiers.
Keywords cannot be used as identifiers.
Identifiers cannot start with a digit.
Some valid identifiers:
age
name
salary1
firstname
Some invalid identifiers:
1age
- starts with a digit
class
- is a keyword
func#
- contains special character #
C-preprocessor
The C preprocessor is a text substitution tool that performs macro definition, macro expansion, file inclusion, and conditional compilation. It is not part of the compiler itself but rather a separate step in the compilation process.
The preprocessor uses directives that start with # to instruct the compiler to perform required preprocessing before actual compilation. Using the preprocessor has the following advantages:
It makes programs easier to develop by:
Allowing macro definitions to reduce redundant code
Allowing file inclusion to split large programs into multiple files
It makes programs easier to read and maintain by:
Using macros and #defines for constants
Giving variables and functions meaningful names
It makes programs easier to modify by:
Allowing conditional compilation to compile different versions
Allowing macro parameters to change behaviour
Preprocessor Directives | Description |
#define | Used to define a macro |
#undef | Used to undefine a macro |
#include | Used to include a file in the source code program |
#ifdef | Used to include a section of code if a certain macro is defined by #define |
#ifndef | Used to include a section of code if a certain macro is not defined by #define |
#if | Check for the specified condition |
#else | Alternate code that executes when #if fails |
#endif | Used to mark the end of #if, #ifdef, and #ifndef |
#define
Defines a macro substitution. It performs simple textual substitution of the macro name before actual compilation.
Syntax:
#define macro_name replacement_text
Example:
#define PI 3.14
#define square(x) x*x
#include
Used to insert the contents of a header file into the source code at the location of the #include statement.
Syntax:
#include <filename>
// For system header files
#include "filename"
// For user-defined header files
Example:
#include <stdio.h>
#include "functions.h"
Advantages:
Allows splitting large programs into multiple files.
Header files contain function prototypes and macro definitions that are shared across multiple source files.
Subscribe to my newsletter
Read articles from Vishesh Raghuvanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
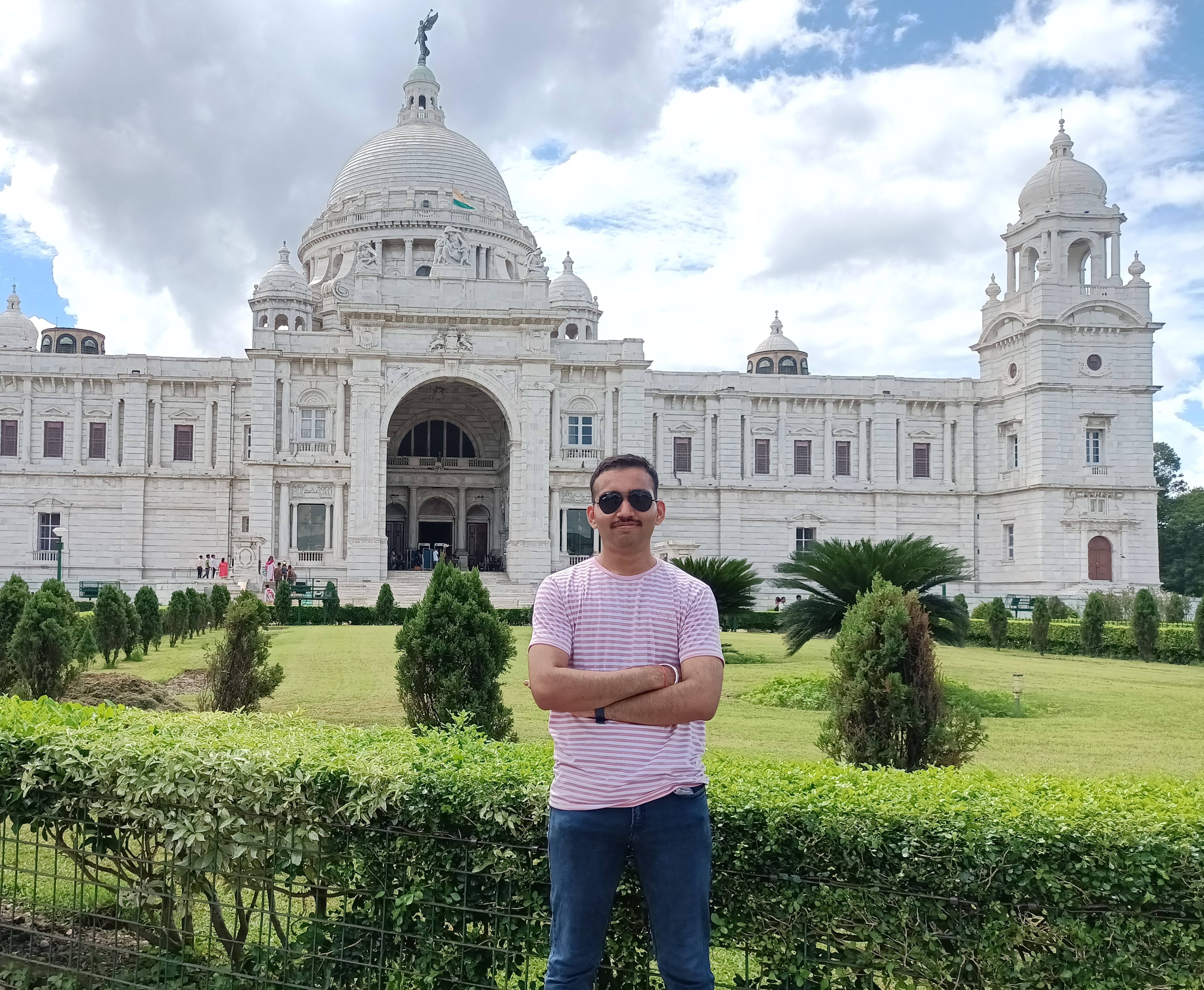