Learning Go (& Routers) Part 1
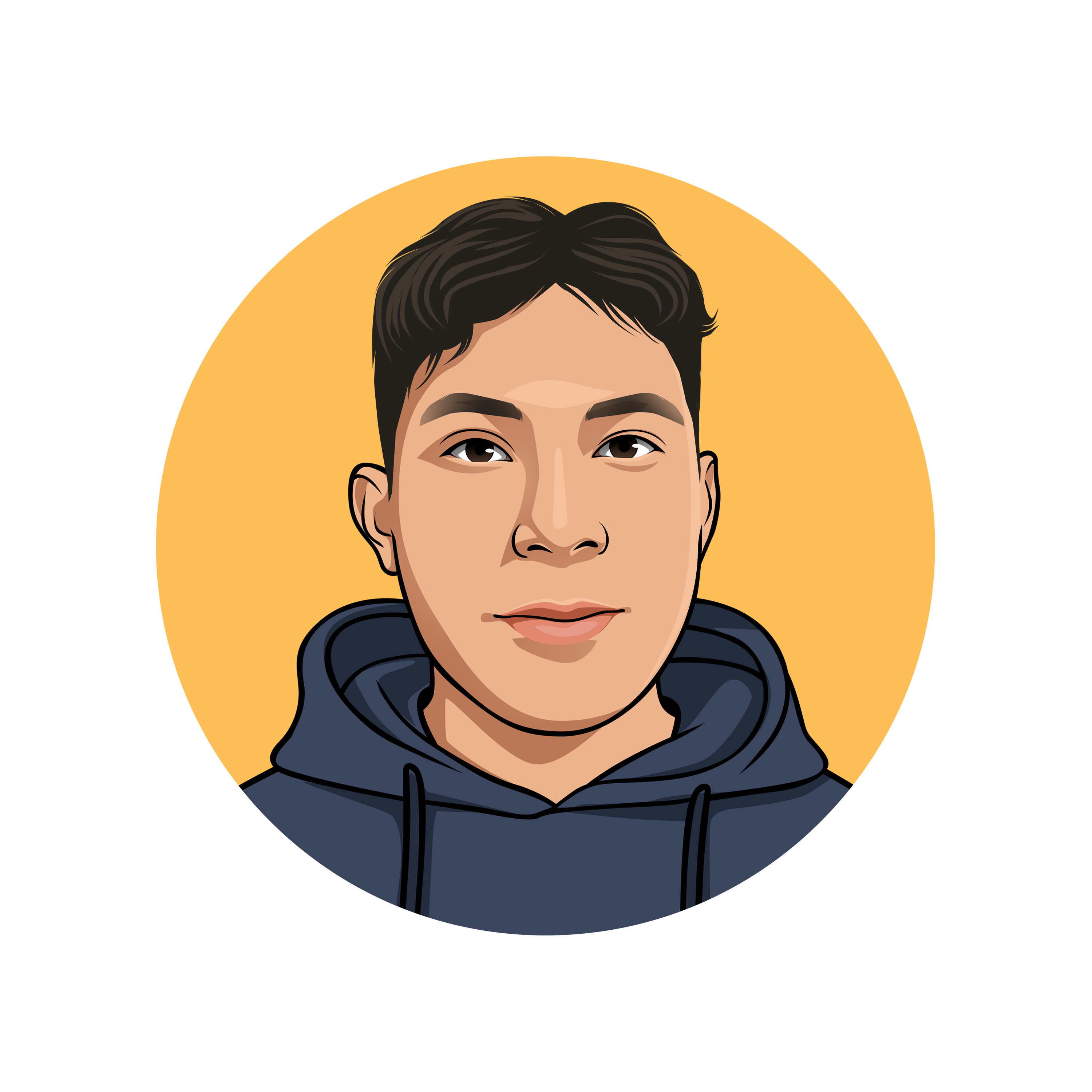
Table of contents

Introduction
Hey everyone, just began learning Go and wanted to create my first URL Shortener! It was very fun and also challenging! I learned many things along the way and would like to share a few of my learnings with you all. Hopefully, it helps someone.
Standard Lib
First off....we need a server. One of the many great things about the Go language is that it provides a great standard library with most (if not all) of the functionality you would ever need. Here is an example of how simple it is to create a server
package main
import (
"net/http"
)
func main() {
//At the route '/' run the helloWorldHandler function
http.HandleFunc("/", helloWorldHandler)
//Listen on the port 4000
http.ListenAndServe(":4000", nil)
}
func helloWorldHandler(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Hello, world!"))
}
this is a great start, however, we are going to need much more than this, for our URL shortener we will be creating two routes
Routes
Method - Route | Description |
GET /url/* | The route used to match the shortened URL and redirect to the original URL |
POST /url/create | This route will be used to create a mapping to the shortened URL and the original URL |
while we do love the standard library, in this project I decided to use the Chi library to make our lives a little easier in working with routers and HTTP requests, as the standard librarys net/http package does not have support for HTTP methods and some other niceties. It can simply be installed using the command
go get -u
github.com/go-chi/chi/v5
Our code will now look something like this
package main
import (
"net/http"
"os"
"time"
"github.com/go-chi/chi/middleware"
"github.com/go-chi/chi/v5"
)
func main() {
r := chi.NewRouter()
// A good base middleware stack
r.Use(middleware.RequestID)
r.Use(middleware.RealIP)
r.Use(middleware.Logger)
r.Use(middleware.Recoverer)
// Set a timeout value on the request context (ctx), that will signal
// through ctx.Done() that the request has timed out and further
// processing should be stopped.
r.Use(middleware.Timeout(60 * time.Second))
//Our routes we defined earlier
r.Get("/url/shorten/*", urlFind)
r.Post("/url/create", urlCreatePost)
http.ListenAndServe(":4000", r)
}
func urlFind(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Finding a url and redirecting..."))
}
func urlCreatePost(w http.ResponseWriter, r *http.Request) {
w.Write([]byte("Creating a url data object..."))
}
Whats Next..
Now we're looking good! The next step is to set up our database connection and implement our handlers...you can use any database but I decided to go with MongoDB, we will start with that in Part 2.
Subscribe to my newsletter
Read articles from Frankie Murillo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
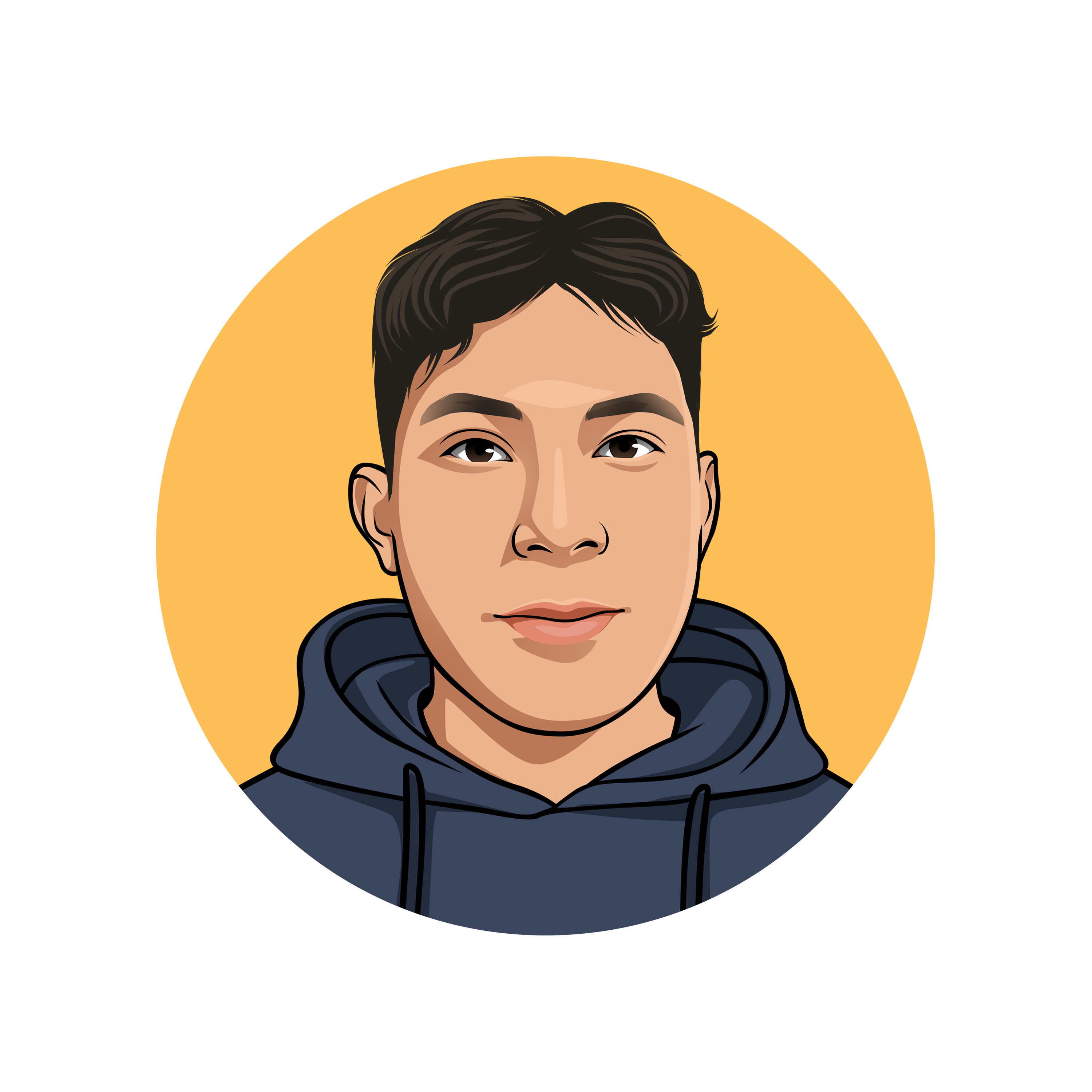