What is a decorator in Python?
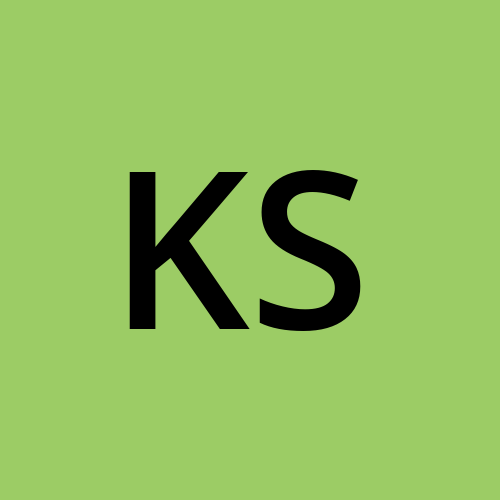
In Python, a decorator is a powerful and flexible tool used for modifying or enhancing the behavior of functions or methods without changing their actual code. Decorators are often employed to add functionality such as logging, authentication, caching, or validation to functions or methods, making them a key feature in Python's metaprogramming capabilities.
Decorators are typically implemented as functions themselves, and they take another function as their input and return a new function as output. This new function usually extends or wraps the original function, allowing you to insert custom code before and/or after the original function's execution. This mechanism is commonly referred to as "wrapping" or "decorating" the function. APart from it by obtaining Python Training Course, you can advance your career in Python. With this course, you can demonstrate your expertise as an as Sequences and File Operations, Conditional statements, Functions, Loops, OOPs, Modules and Handling Exceptions, various libraries such as NumPy, Pandas, Matplotlib, many more fundamental concepts, and many more critical concepts among others.
Here's a simple example of a decorator in Python:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
In this example, my_decorator
is a decorator function that takes the say_hello
function as an argument and returns a new function called wrapper
. When we decorate the say_hello
function using @my_decorator
, it effectively becomes equivalent to calling say_hello = my_decorator(say_hello)
. Now, when we call say_hello()
, it executes the code defined in both wrapper
and the original say_hello
function, producing the following output:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
Decorators are commonly used in Python frameworks like Flask and Django to handle various tasks such as routing, authentication, and request/response manipulation. They provide a clean and reusable way to extend the functionality of functions or methods, making code more modular and maintainable.
Additionally, Python has some built-in decorators, such as @staticmethod
and @classmethod
, used in object-oriented programming to define static and class methods, respectively. You can also create custom decorators tailored to your specific application needs, allowing for greater flexibility and code organization in your Python projects.
Subscribe to my newsletter
Read articles from Kamla Sri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
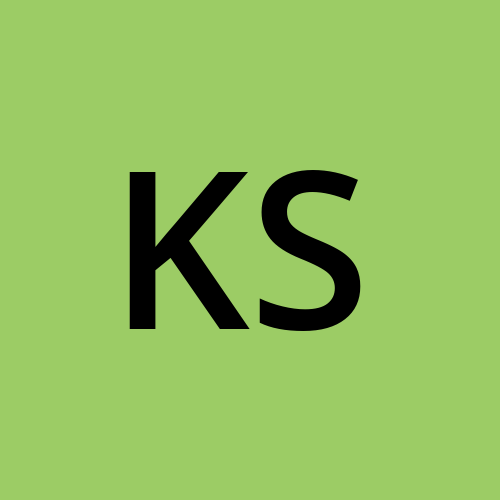