All About Variables in JavaScript
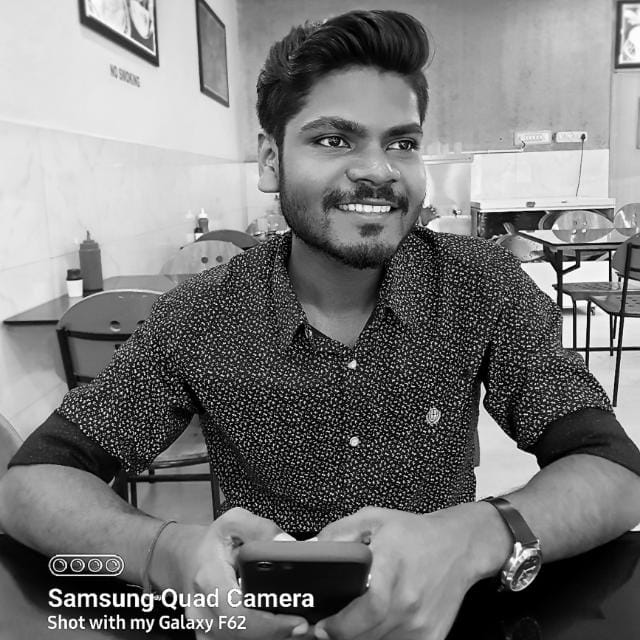
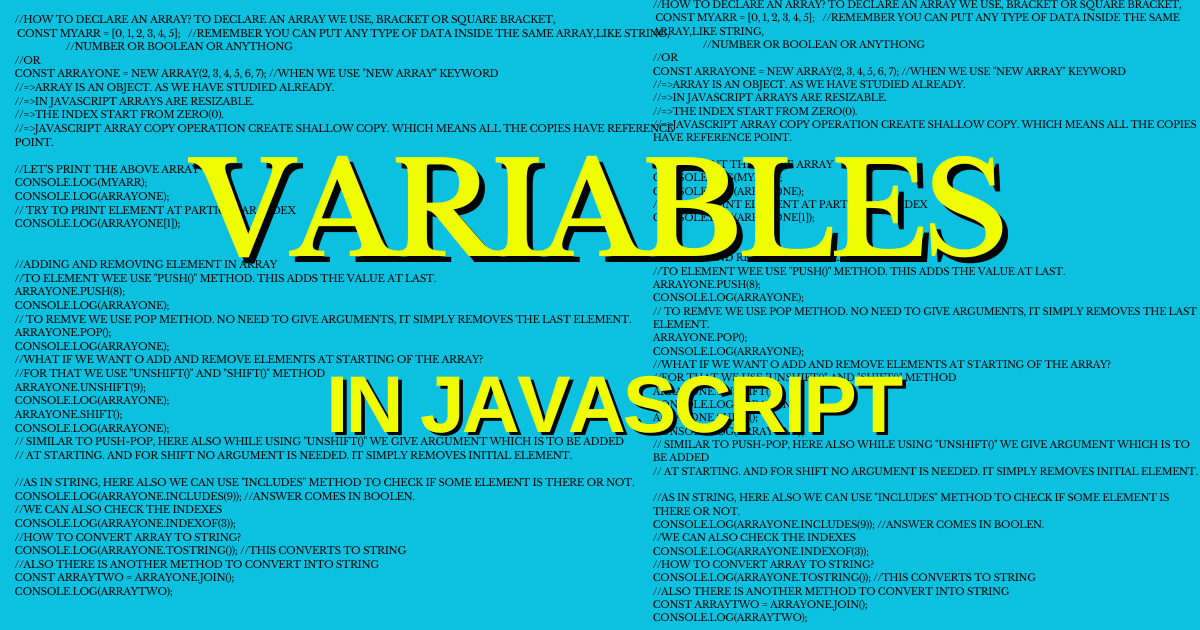
Variables are fundamental components in the world of programming. They play a crucial role in storing and managing data. In JavaScript, one of the most widely used programming languages, understanding variables is essential for building robust applications. In this blog post, we will explore the world of variables, covering variable declaration, hoisting, variable naming rules, and variable scope.
1) Variable Declaration
In JavaScript, variables are used to store and manage data. You can think of them as containers that hold different types of information, such as numbers, text, or complex objects. To declare a variable, you use one of three keywords: var
,let
, or const
.
var
: The Legacy Keyword
var
was the original way to declare variables in JavaScript. It has global or function scope, meaning it's accessible throughout the entire function or globally if declared outside of any function. However, var
has its downsides, including hoisting and the potential for variable redeclaration, which can lead to unexpected behaviour.
var name = "John";
let
: Block-Scoped Variables
let
was introduced in ECMAScript 6 (ES6) to address some of the issues associated with var
. It has block scope, meaning it's only accessible within the block or function where it's declared. let
provides better control over variable scoping.
let age = 30;
const
: Constants
const
is another addition from ES6. It also has block scope, but unlike let
, you cannot reassign a value to a const
variable once it's declared. It's used for values that should not change during the program's execution.
const pi = 3.14;
2) Hoisting
Hoisting is a JavaScript mechanism where variable and function declarations are moved to the top of their containing scope during compilation. This means that you can use variables before they are declared in your code.
console.log(name); // undefined
var name = "Alice";
However, it's important to note that only the declarations are hoisted, not the initializations. So, the variable is hoisted with an initial value of undefined
. This behaviour is one of the reasons why let
and const
were introduced, as they are not hoisted in the same way.
3) Variable Naming Rules
When naming variables in JavaScript, there are some rules and conventions to follow:
Variable names are case-sensitive, meaning
myVar
andmyvar
are treated as two different variables.Variable names must begin with a letter, underscore (
_
), or dollar sign ($
).After the first character, variable names can also include numbers.
Variable names should be descriptive and meaningful, following a camelCase or snake_case naming convention.
4) Variable Scope
In JavaScript, variable scope determines where in your code a particular variable is accessible and where it is not. JavaScript has three main types of variable scope: block scope, function scope, and global scope. Let's explore each of these scopes in more detail:
Block Scope:
What is it?: Block scope refers to the smallest scope in JavaScript, defined by a pair of curly braces
{}
. Variables declared within a block are only accessible within that block.Example:
if (true) { let blockVar = "I'm in a block"; console.log(blockVar); // Accessible } console.log(blockVar); // Not accessible (results in an error)
Use Cases: Block scope is commonly used with
let
andconst
to create variables that are limited to a specific block of code, such as loops, conditional statements, or function bodies.
Function Scope:
What is it?: Function scope defines the scope of variables declared within a function. Variables declared with
var
are function-scoped, meaning they are accessible throughout the entire function in which they are declared.Example:
function exampleFunction() { var functionVar = "I'm in a function"; console.log(functionVar); // Accessible } console.log(functionVar); // Not accessible (results in an error)
Use Cases: Function scope is used with
var
to create variables that are specific to a particular function. These variables are not accessible outside the function.(NOTE:- Block scope and function scope are called "local scope".)
Global Scope:
What is it?: Global scope is the broadest scope in JavaScript. Variables declared outside of any function or block have global scope and can be accessed from anywhere in your code.
Example:
var globalVar = "I'm global"; function exampleFunction() { console.log(globalVar); // Accessible } console.log(globalVar); // Accessible
Use Cases: Global scope is typically used for variables that need to be accessed and modified across different parts of your application. However, it's important to use global variables sparingly to avoid unintended side effects and naming conflicts.
It's important to choose the appropriate type of scope and variable declaration (var
, let
, or const
) based on your specific use case. In modern JavaScript, it's recommended to use let
and const
for block and function scope to avoid some of the issues associated with var
. Understanding variable scope is essential for writing clean and maintainable JavaScript code.
In conclusion, variables are a fundamental concept in JavaScript and programming in general. By understanding variable declaration, hoisting, naming rules, and variable scope, you'll be better equipped to write clean, organized, and bug-free code in JavaScript.
Subscribe to my newsletter
Read articles from Manish Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
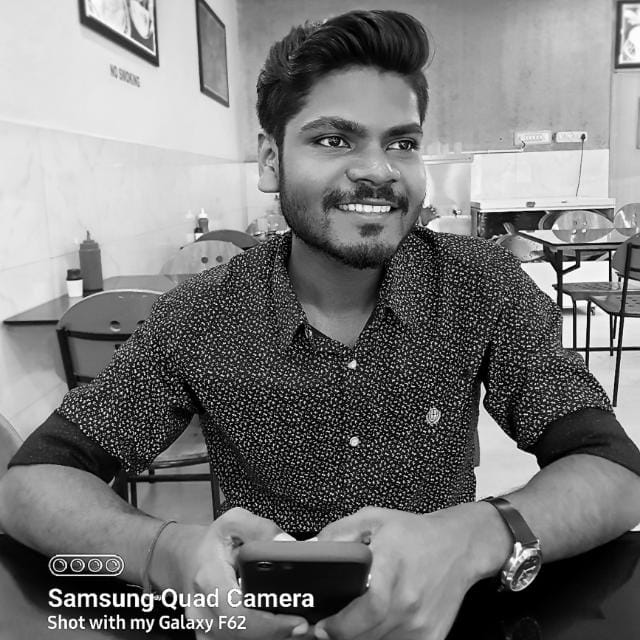
Manish Kumar
Manish Kumar
Frontend Developer