The Complete Guide to For Loops in JavaScript
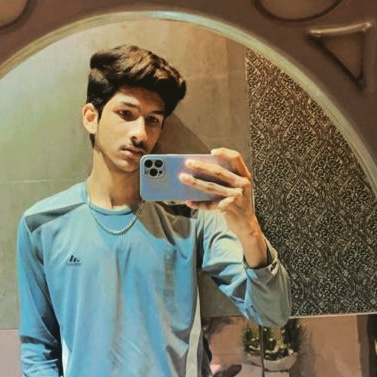
For loops are often the workhorse of JavaScript code, but they have many advanced features beyond basic iterating. In this epic deep dive, we'll explore all the ins and outs of JavaScript loops so you can level up your skills.
Grab some coffee and get comfortable - this will be quite the journey!
For Loop Basics
Let's start with a quick primer on basic for loop syntax:
for (let i = 0; i < 5; i++) {
// Executes 5 times
}
This loop contains:
Initialization -
let i = 0
Condition check -
i < 5
Iteration -
i++
With this structure, you can repeat a block of code a set number of times.
While simple, for loops enable techniques like:
Displaying numbers
Iterating arrays
Building countdown timers
Let's look at some basic examples...
Displaying Numbers
To display the numbers 1-10 in the console:
for (let i = 1; i <= 10; i++) {
console.log(i);
}
This shows the basics of using the counter to print an incrementing value.
Iterating Array Values
We can loop through array elements like so:
let fruits = ['Apple', 'Orange', 'Banana'];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
The length
property provides the total count.
This prints each fruit name one by one.
There are even easier ways to loop arrays we'll cover soon!
Building a Countdown Timer
Let's use a for loop to build a simple countdown timer that displays in the browser:
let timeLeft = 10;
for (let i = 0; i <= 10; i++) {
setTimeout(() => {
timeLeft--;
document.body.textContent = timeLeft;
}, 1000)
}
This gradually decrements timeLeft
and updates the page each second.
After 10 seconds, the timer reaches 0! Our first mini-app using a basic for loop.
These examples provide a solid base upon which to build. Now let's level up our looping skills!
Advanced Looping Constructs
JavaScript provides many other ways to iterate besides a standard for loop:
forEach()
The .forEach()
method built into arrays iterates each element:
let fruits = ['Apple', 'Orange', 'Banana'];
fruits.forEach(fruit => {
console.log(fruit);
});
This offers a more concise syntax for array iteration.
for...of
The for...of
loop iterates over iterable objects like arrays:
let fruits = ['Apple', 'Orange', 'Banana'];
for (let fruit of fruits) {
console.log(fruit);
}
This is handy when you need both the value and index.
for...in
The for...in
loop iterates object properties:
let car = {
make: 'Ford',
model: 'Mustang',
year: 2022
};
for (let key in car) {
console.log(key);
}
This prints each key rather than the values.
There are many more advanced constructs we'll cover soon!
Avoiding Infinite Loops
One common pitfall is accidentally creating an infinite loop that crashes your program.
For example:
// Infinite loop!
for (let i = 0; ; i++) {
console.log(i);
}
We "forgot" to include a condition check, so this will repeat forever!
To avoid:
Double-check your condition checks
Use break statements
Handle errors properly
Careful loop construction prevents hanging your program.
Common Loop Errors:
Infinite Loops: These occur when the loop's condition is never met, causing the loop to run indefinitely. To troubleshoot, ensure that your condition check will eventually evaluate to
false
. Also, consider using a break statement in appropriate cases.Off-by-One Errors: These occur when you iterate one element too many or too few times. Double-check your loop boundaries and the condition to avoid this.
Uninitialized Variables: If you forget to initialize your loop counter or iterator variable, it can lead to unexpected behavior. Always initialize variables before using them in loops.
Troubleshooting Tips:
Console Logging: Use
console.log()
statements strategically to print variables' values and conditions inside the loop. This can help you identify where the issue lies.Debugging Tools: Take advantage of browser developer tools or integrated development environments (IDEs) with debugging capabilities to step through your code and pinpoint errors.
Var vs. Let vs. Const
Declaring the loop counter variable properly ensures correct scoping:
jsCopy codefor (var i = 0; i < 5; i++) {
// Don't use var!
}
// i is still accessible here
var
is function scoped, so i
leak outside the loop.
Instead, use let
or const
for block scoping:
for (let i = 0; i < 5; i++) {
// Use let
}
// i is not accessible here
Block scoping contains the variable within the loop.
Looping Performance
In some cases, the standard for loops can offer better performance than array methods like .forEach()
.
For simple iterations, a plain for loop is faster:
// Faster
for (let i = 0; i < arr.length; i++) {
// ...
}
// Slower
arr.forEach(item => {
// ...
})
However, methods like .map()
and .reduce()
utilize optimized implementations under the hood.
Balance readability with performance for your use case.
Looping Backwards
We can count backward by decrementing the counter:
for (let i = 10; i >= 0; i--) {
console.log(i); // 10 to 1
}
Helpful for showing countdown timers and reverse animations.
Skipping Iterations with Continue
The continue
statement skips the current loop iteration:
for (let i = 1; i <= 10; i++) {
if (i === 3) {
continue; // Skip ahead
}
console.log(i);
}
// 1, 2, 4, 5, ...10
When i
was 3, we hit continue
to jump ahead and resume after 3. Handy for leap years!
Breaking Early with Break
To exit a loop immediately, use break
:
for (let i = 1; i <= 10; i++) {
if (i > 5) {
break; // Stop after 5
}
console.log(i);
}
// Prints just 1, 2, 3, 4, 5
Once i
exceed 5, we trigger break
to bail out early. Useful for timeouts or limits!
Short-Circuiting a Loop
We can use break
and continue
to interrupt loop execution:
for (let i = 0; i < 10; i++) {
if (i === 5) {
continue; // Skip to next iteration
}
if (i > 7) {
break; // Stop entirely
}
console.log(i);
}
The continue
skips ahead, while break
exits early.
This allows precise control over iteration flow.
Multiple Operations with the Comma Operator
The comma operator runs multiple operations in each iteration:
for (let i = 0, j = 10; i < 5; i++, j--) {
// Use i and j
}
Now i
increments up while j
decrements down. Very powerful!
These pro techniques give you precise control over complex loops. Now let's dive further...
Advanced Looping Concepts
Let's dive deeper into some more advanced looping techniques and how they can be applied in real-world situations.
For loops can do way more than simple numerical iterating. Let's look at how to loop arrays, objects, and async code like a pro.
Looping Arrays Backwards
We can reverse array iteration with a decrementing index:
let fruits = ['Apple','Orange','Banana'];
for (let i = fruits.length - 1; i >= 0; i--) {
console.log(fruits[i]);
}
// Banana, Orange, Apple
Looping backwards is a handy trick for stacks and queues!
Randomizing Array Order
To loop an array randomly, we can utilize the splice()
method:
let shuffledFruits = [];
let fruits = ['Apple','Orange','Banana'];
while (fruits.length > 0) {
// Pick random index
let randIndex = Math.floor(Math.random() * fruits.length);
// Remove from fruits array
let randFruit = fruits.splice(randIndex, 1)[0];
// Add to shuffled array
shuffledFruits.push(randFruit);
}
// Shuffled random order!
This results in a crazy shuffled-up array order. Awesome for shuffling card decks!
Iterating Multidimensional Arrays
We can loop a 2D array with nested loops:
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log(matrix[i][j]);
}
}
The outer loop handles rows, and the inner handles iterating columns.
This allows the processing of 2D data like grids and game boards.
Object Property Iteration
To loop object properties, use the for...in
syntax:
jsCopy codelet car = {
make: 'Ford',
model: 'Mustang',
year: 2022
};
for (let key in car) {
console.log(key); // 'make', 'model', 'year'
}
This prints each key rather than the values.
Async Loops with Async/Await
To loop async code like promises sequentially, use async/await
:
async function fetchFruits() {
let fruits = ['apple.com','orange.com','banana.com'];
for (let i = 0; i < fruits.length; i++) {
let resp = await fetch(url); // Wait for each
let html = await resp.text();
console.log(html);
}
}
The await
keyword pauses the loop to wait for each promise before fetching the next fruit. Much cleaner than promised!
Looping Over Async Code
We can iterate asynchronously using async/await:
async function getUsers() {
const urls = [
'https://jsonplaceholder.typicode.com/users/1',
'https://jsonplaceholder.typicode.com/users/2',
'https://jsonplaceholder.typicode.com/users/3'
];
for (let url of urls) {
const response = await fetch(url);
const user = await response.json();
console.log(user);
}
}
This allows us to await each call before fetching the next user. Much better than nesting callbacks!
You might use this in an e-commerce app to load user data on the server side.
Looping Over Maps and Sets
We can iterate Map and Set data structures:
const pets = new Set(['cat', 'dog', 'fish']);
for (let pet of pets) {
console.log(pet);
}
const ages = new Map();
ages.set('john', 20);
ages.set('mary', 21);
for (let [name, age] of ages) {
console.log(name, age);
}
This provides a way to loop key/value pairs in a Map, similar to objects.
You could use this in a user profile page to display custom user data.
Recursive Looping
Recursion is an alternative to looping that calls a function repeatedly:
function countdown(n) {
if (n < 1) {
console.log('Done!');
return;
}
console.log(n);
countdown(n - 1); // Call again
}
countdown(5);
The function keeps calling itself until the base case is reached.
You might use recursion in advanced algorithms like sorting binary trees.
We've covered a ton of advanced loop techniques! Let's recap what we learned...
Real-World Projects:
Project 1: Simple Task List
Description: Create a web-based task list application where users can add, edit, and delete tasks. Use loops to dynamically display and manage tasks.
basic code :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Task List</title>
<style>
/* Add your CSS styles here for task list appearance */
</style>
</head>
<body>
<h1>Task List</h1>
<input type="text" id="taskInput" placeholder="Add a task">
<button onclick="addTask()">Add Task</button>
<ul id="taskList">
<!-- Tasks will be dynamically added here -->
</ul>
<script>
// JavaScript code for the Task List
const taskInput = document.getElementById('taskInput');
const taskList = document.getElementById('taskList');
function addTask() {
const taskText = taskInput.value.trim();
if (taskText === '') return;
// Create a new list item
const listItem = document.createElement('li');
listItem.textContent = taskText;
// Add delete button to the list item
const deleteButton = document.createElement('button');
deleteButton.textContent = 'Delete';
deleteButton.onclick = function () {
taskList.removeChild(listItem);
};
listItem.appendChild(deleteButton);
// Add the list item to the task list
taskList.appendChild(listItem);
// Clear the input field
taskInput.value = '';
}
</script>
</body>
</html>
Project 2: Number Guessing Game
Description: Develop a number guessing game where the computer generates a random number, and the user has to guess it. Loops can be used to allow multiple guesses until the correct number is guessed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Number Guessing Game</title>
</head>
<body>
<h1>Number Guessing Game</h1>
<p>Guess a number between 1 and 100:</p>
<input type="number" id="guessInput">
<button onclick="checkGuess()">Submit Guess</button>
<p id="message"></p>
<script>
// JavaScript code for the Number Guessing Game
const secretNumber = Math.floor(Math.random() * 100) + 1;
let attempts = 0;
function checkGuess() {
const guessInput = document.getElementById('guessInput');
const message = document.getElementById('message');
const guess = parseInt(guessInput.value);
if (isNaN(guess) || guess < 1 || guess > 100) {
message.textContent = 'Please enter a valid number between 1 and 100.';
return;
}
attempts++;
if (guess === secretNumber) {
message.textContent = `Congratulations! You guessed the number ${secretNumber} in ${attempts} attempts.`;
guessInput.disabled = true;
} else if (guess < secretNumber) {
message.textContent = 'Try a higher number.';
} else {
message.textContent = 'Try a lower number.';
}
guessInput.value = '';
}
</script>
</body>
</html>
For Loop Recap and Key Takeaways
We covered a lot of ground, so let's recap the key looping concepts:
for - The standard numerical iterator
forEach - Iterator built into arrays
for... in-Loop object properties
for...of - Loop iterable values
async/await - Write async code like it's sync
Break and continue - Pause or skip iterations
Reverse - Count backward with a decrementing index
Random - Shuffle array order randomly
Multidimensional - Nested loops for 2D arrays
Multiple iterators - Use commas to declare multiple variables
I hope these pro tips help you write better, cleaner loops and fully unlock the powers of for loops in your own projects!
Here are some recommendations:
MDN Web Docs: JavaScript Loops: Link to the Mozilla Developer Network's comprehensive guide on loops and iteration in JavaScript.
W3Schools: JavaScript Loops: W3Schools offers interactive tutorials and examples on JavaScript loops.
JavaScript.info: Loops: Link to JavaScript.info's section on loops, which provides in-depth explanations and examples.
Subscribe to my newsletter
Read articles from Mikey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
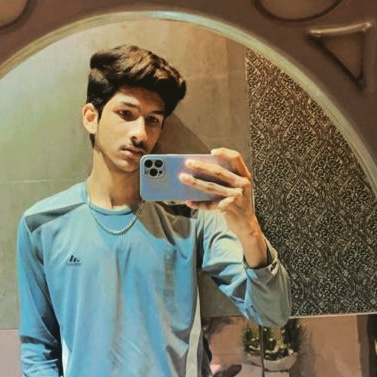
Mikey
Mikey
Undergrad Student in domain of Web developer at Chandigarh University.