Understanding OOPS!
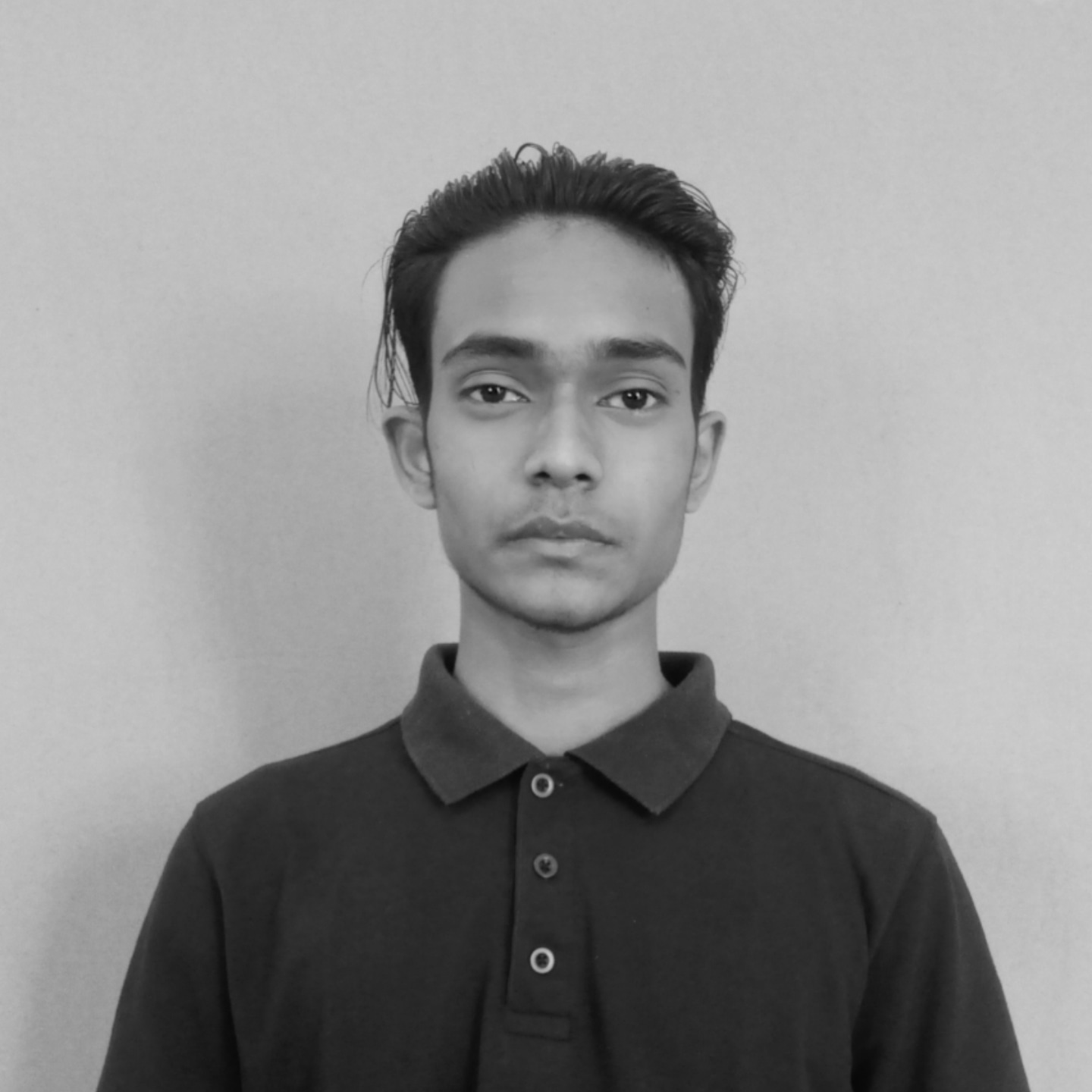
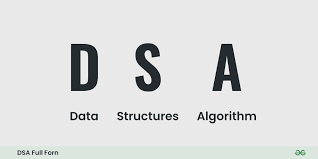
It is Day 4 in my #180ofDSA challenge. Today I will write about Object-Oriented Programming(OOP) which is a very crucial concept every programmer should understand.
When I first started in tech back in June 2019, the first programming language I was learning was C programming language. I vividly remember that summer I spent, learning something completely new. It was a mind-boggling experience for me. It was my first programming language. Every single program I would write in C would look something like the following-
#include<stdio.h>
#include<conio.h>
//main function
int main(){
int a = 20;
int b = 10;
add(a,b);
sub(a,b);
mul(a,b);
div(a,b);
}
//function 1
void add(int a, int b){
printf("%d", a+b);
}
//function 2
void sub(int a, int b){
printf("%d", a-b);
}
//function 3
void mul(int a, int b){
printf("%d", a*b);
}
//function 4
void div(int a, int b){
printf("%d", a/b);
}
Then I discovered Data Structures and Algorithm after learning C language. I read a lot of articles and watched a lot of videos to understand how to learn Data Structures and algorithms. The majority of them recommended to learn DSA in Java or C++.
Without much consideration, I picked up Java and started learning it. Then I realized the same code that I wrote earlier in C would look the following in Java
import java.util.*;
class Add {
Add(int a, int b){
return a+b;
}
}
class Sub {
Sub(int a, int b){
return a-b;;
}
}
class Mul {
Sub(int a, int b){
return a*b;
}
}
class Div {
Sub(int a, int b){
return a/b;
}
}
class Arithmetic {
public static void main(String args[]){
Add addition = new Add(10,20);
System.out.println(addition);
Sub subtraction = new Sub(10,20);
System.out.println(subtraction);
Mul multiplication = new Mul(10,20);
System.out.println(subtraction);
Div division = new Div(10,20);
System.out.println(division);
}
}
I started wondering why is the case that we are not writing code just like we did in the C program with functions in Java. Then I came across programming paradigms.
I discovered C programming uses the Procedural Programming Paradigm and Java Programming uses the Object-Oriented Programming Paradigm.
Also, I discovered many other programming paradigms namely;
Functional Programming Paradigm
Logic Programming Paradigm
Imperative Programming Paradigm
Declarative Programming Paradigm
It was a eureka moment for me😅
Fun Fact: We can implement multiple programming paradigm while writing code in a particular language. It is best practise to use the one language suggests.
Java says, "We are a object-oriented programming language". When you write code using our language please implement OOP. Similarly, C says that we are a procedural programmng language. Please implement procedural paradigm when you write code in C.
The moral of the story is that first we have to understand programming paradigms in order to understand OOPS.
Therefore, I will write another blog where I will continue from here. Until then happy coding.
Subscribe to my newsletter
Read articles from Grut directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
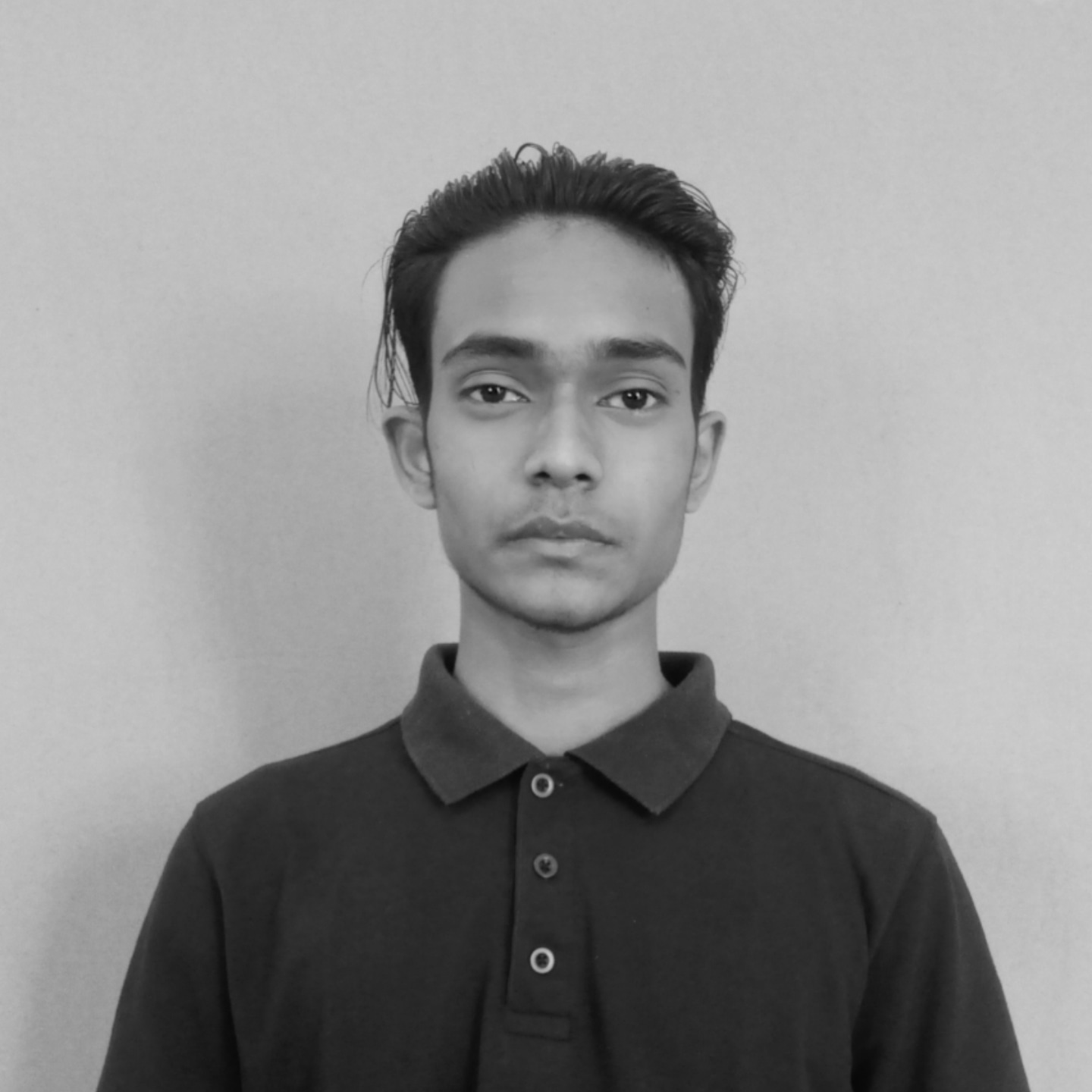
Grut
Grut
I am a CS Student from Nepal interested in Competitive Programming, Web Development, and Blockchain Development.