Creating Tic Tac Toe with AI using Pygame and Minimax Algorithm
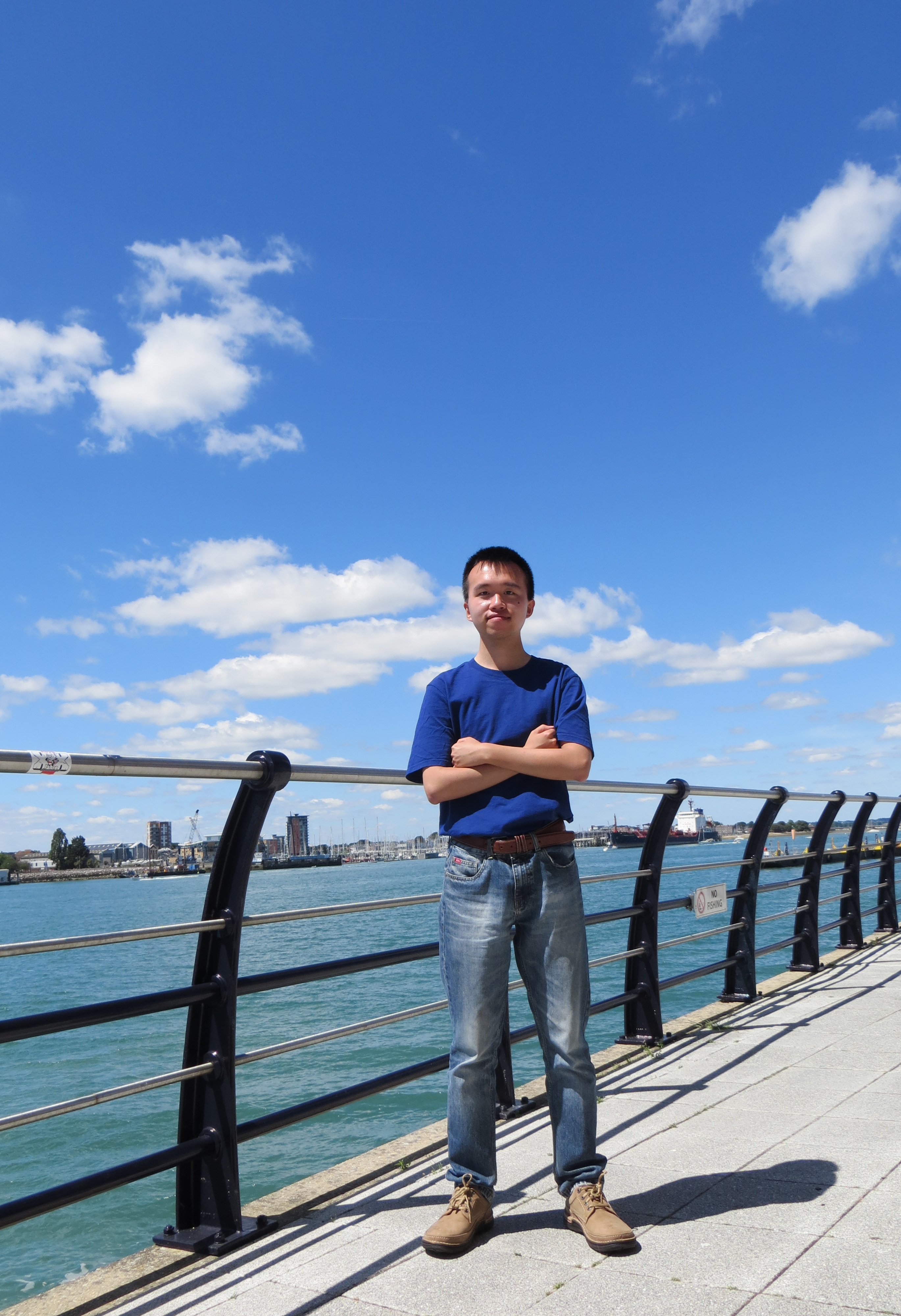
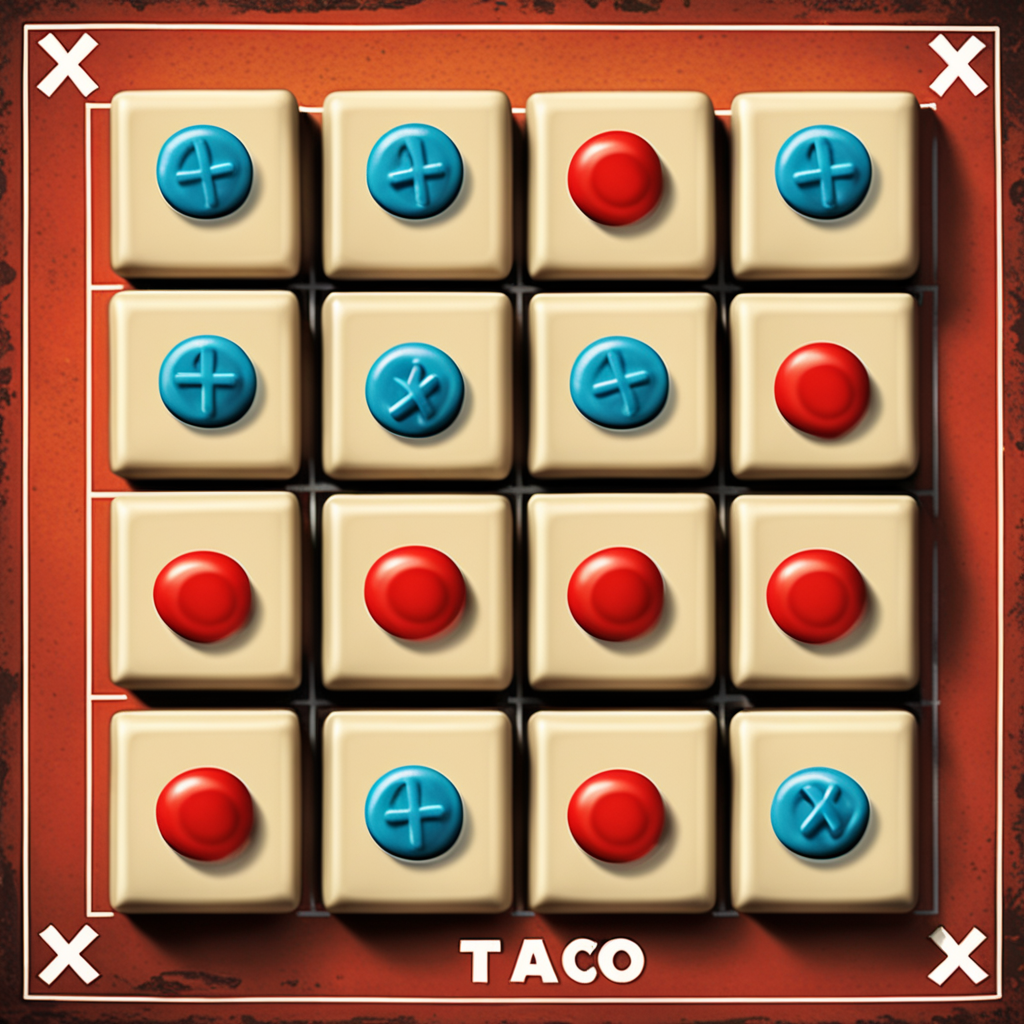
In this tutorial, we will create a game of Tic Tac Toe where you can play against a computer AI. The AI will be built using the minimax algorithm, ensuring that the AI will always make the optimal move.
Let's get started!
Setup
Firstly, make sure you have pygame
installed:
pip install pygame
Start Game
We will start with our game screen, setting up the colours, and creating a function to draw the Tic Tac Toe board.
import pygame
import sys
# initialize pygame
pygame.init()
# Colors and settings
WHITE = (255, 255, 255)
LINE_COLOR = (23, 85, 85)
CROSS_COLOR = (242, 85, 96)
CIRCLE_COLOR = (85, 170, 85)
SCREEN_WIDTH = 300
SCREEN_HEIGHT = 300
SCREEN = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Tic Tac Toe')
def draw_board():
for row in range(1, 3):
pygame.draw.line(SCREEN, LINE_COLOR, (SCREEN_WIDTH / 3 * row, 0),
(SCREEN_WIDTH / 3 * row, SCREEN_HEIGHT), 7)
pygame.draw.line(SCREEN, LINE_COLOR, (0, SCREEN_HEIGHT / 3 * row),
(SCREEN_WIDTH, SCREEN_HEIGHT / 3 * row), 7)
draw_board()
Game Logic
We will represent the game board as a 3x3 matrix. The matrix will have values 'X', 'O', or '' (empty).
board = [['' for x in range(3)] for y in range(3)]
We check for a win condition every time a move is made. Here's how we can do it:
def check_winner(board, player):
for i in range(3):
if all([cell == player for cell in board[i]]):
return True
if all([board[j][i] == player for j in range(3)]):
return True
return board[0][0] == board[1][1] == board[2][2] == player or board[0][2] == board[1][1] == board[2][0] == player
AI Logic
The AI will use the minimax algorithm. This algorithm simulates all possible moves on the board to determine the best possible strategy. We also implement alpha-beta pruning to make the algorithm faster.
def minimax(board, depth, is_maximizing, alpha, beta):
# ... [content as in provided code]
We'll also create a function to determine the AI's best move:
def best_move(board):
# ... [content as in provided code]
Game Rendering
We update our screen based on the board matrix to visualise the game.
def update_board(board):
# ... [content as in provided code]
Main Game Loop
Lastly, the main game loop listens for events and updates the game state accordingly. When a player (or AI) wins, it saves the game board as an image and exits.
while True:
# ... [content as in provided code]
Conclusion
You now have a complete Tic Tac Toe game where you can play against an unbeatable AI. This game showcases the power of the minimax algorithm in game theory. You can expand on this by adding a GUI, sound effects, or different game modes. Happy coding!
Subscribe to my newsletter
Read articles from Haocheng Lin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
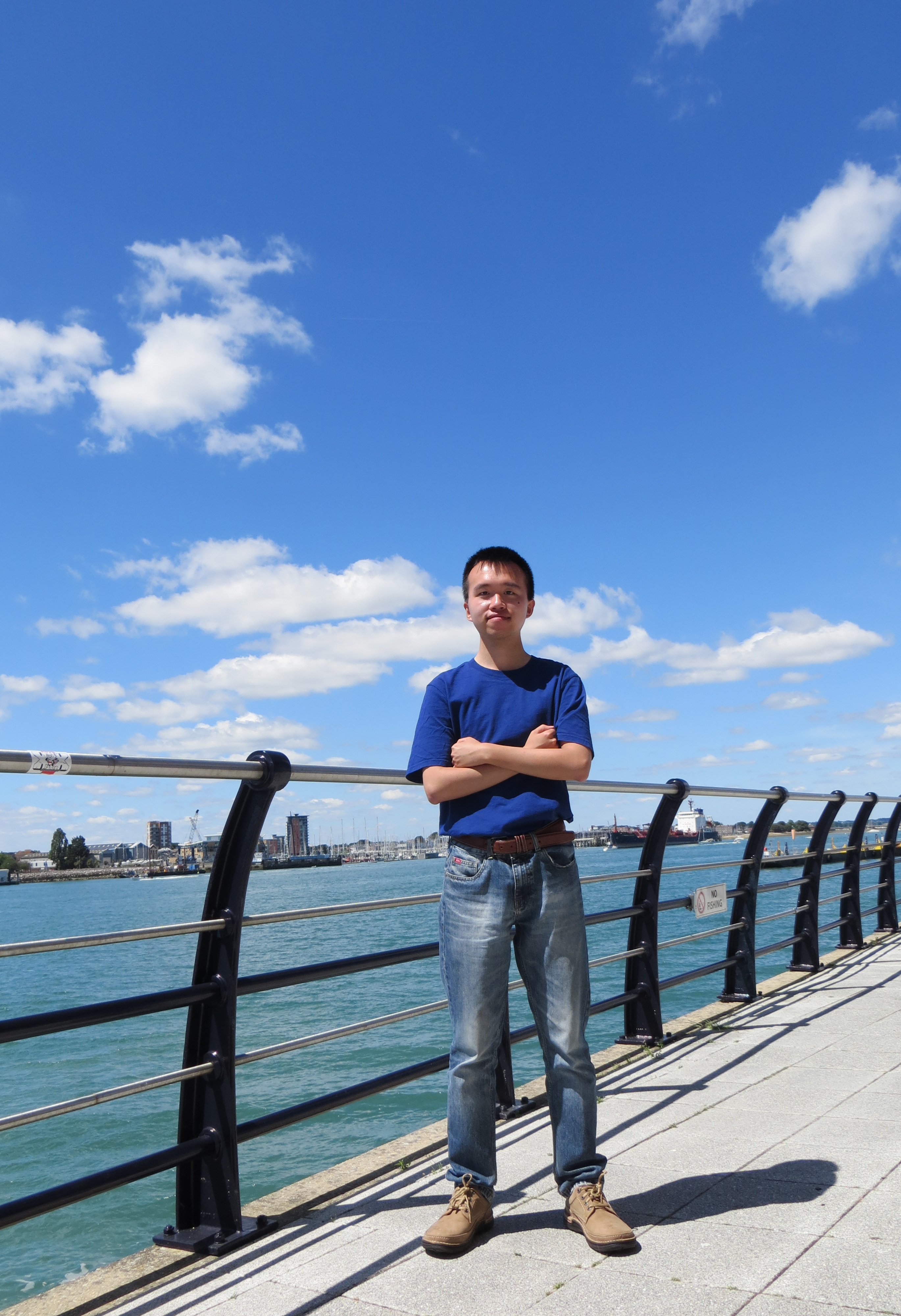
Haocheng Lin
Haocheng Lin
I am a full-stack developer from London 💻 An MEng computer science graduate 🎓 from UCL 🏛️ (2019 - 23). 🏛️UCL MSc AI for Sustainable Development (2023 - 24) 🥇Microsoft Golden Global Ambassador (2023 - 24)🏆