Exploring Functions Executed Before and After main() in C.

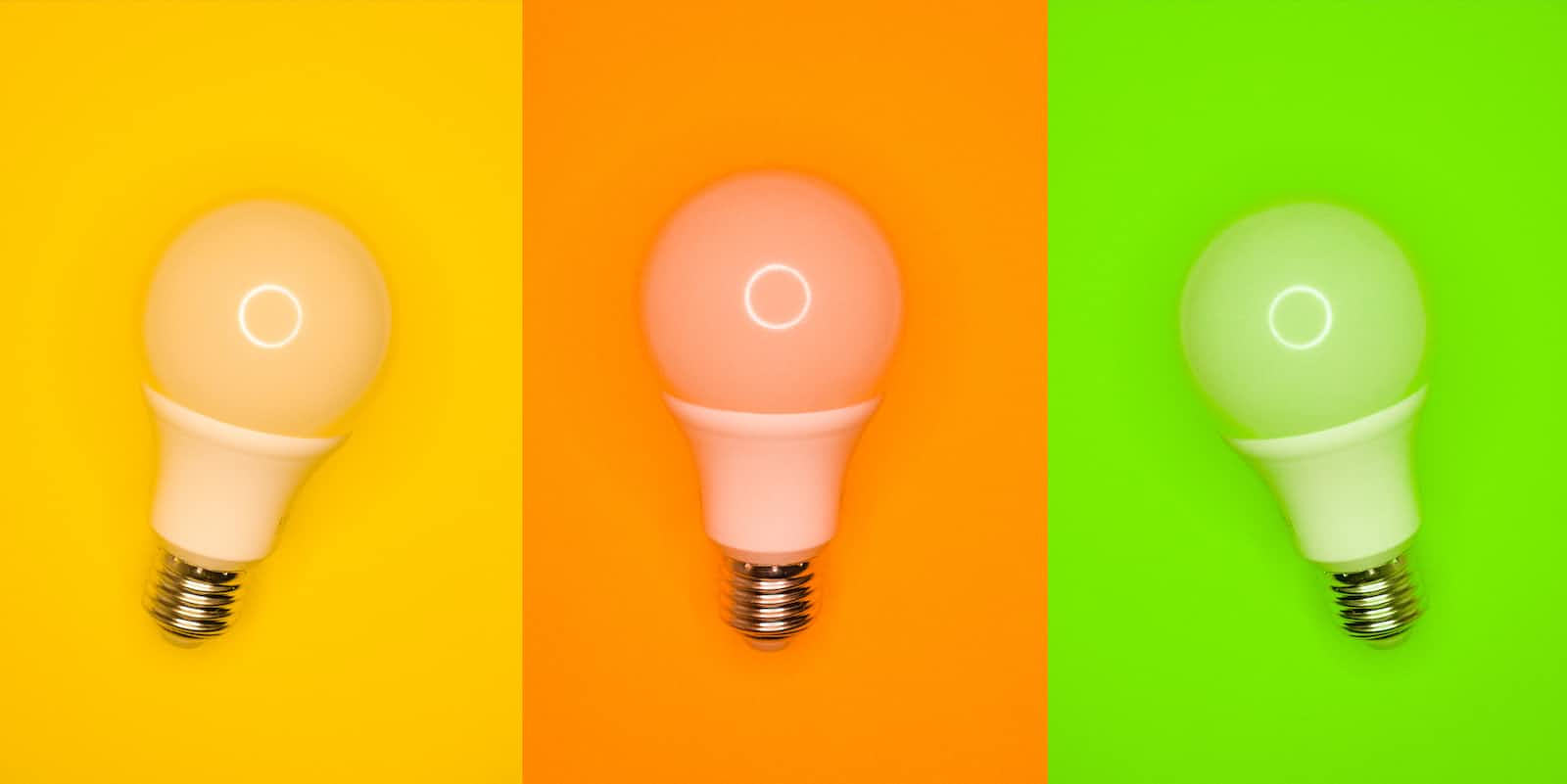
Introduction to Main Function in C
In C programming, the main function is the entry point of a program. There can be only one main function in a program. The operating system calls the main function to execute a program.
Contrary to popular opinion that functions are executed inside the main function, C provides a syntax that enables you to run a function before and after the main function.
This brings us to the concept of Constructors and Destructors.
Constructors
In many languages, constructors and destructors are Object Oriented Programming (OOP) concepts. C is not an OOP language.
Constructors are used to execute a function before the main function in C. You can try this out;
#include <stdio.h>
// Declaration of the constructor function
void before_main() __attribute__((constructor));
// Definition of the constructor function
void before_main() {
printf("Before main function\n");
}
int main() {
printf("Inside main function\n");
return 0;
}
Before main function
Inside main function
Use Cases of constructors in C?
To initialize global variables.
Establish network connections.
Initialize hardware resources.
Used for memory allocation through dynamic memory allocation
And so much more...
Destructors
It is commonly known that a C program ends with the return statement in the main function. However, you can execute functions after the main function with the help of destructors.
#include <stdio.h>
// Declaration of the destructor function
void after_main() __attribute__((destructor));
// Definition of the destructor function
void after_main() {
printf("After main function\n");
}
int main() {
printf("Inside main function\n");
return 0;
}
Inside main function
After main function
Use Cases of Destructors in C?
To clean up tasks such as closing network connections.
Used in releasing hardware resources.
Used for memory deallocation through dynamic memory allocation.
Conclusion
Even though C is not an object-oriented language, constructors and destructors still have their use cases in C.
I hope you found this article helpful. Please leave a heart ❤️ and share to encourage me to write more articles.
Subscribe to my newsletter
Read articles from Oluchukwu Ughagwu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
