Boost Your FastAPI App's Performance with Celery
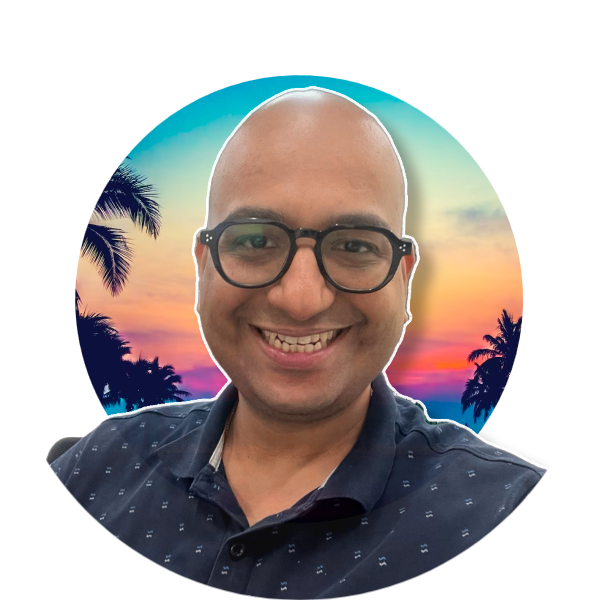
Table of contents
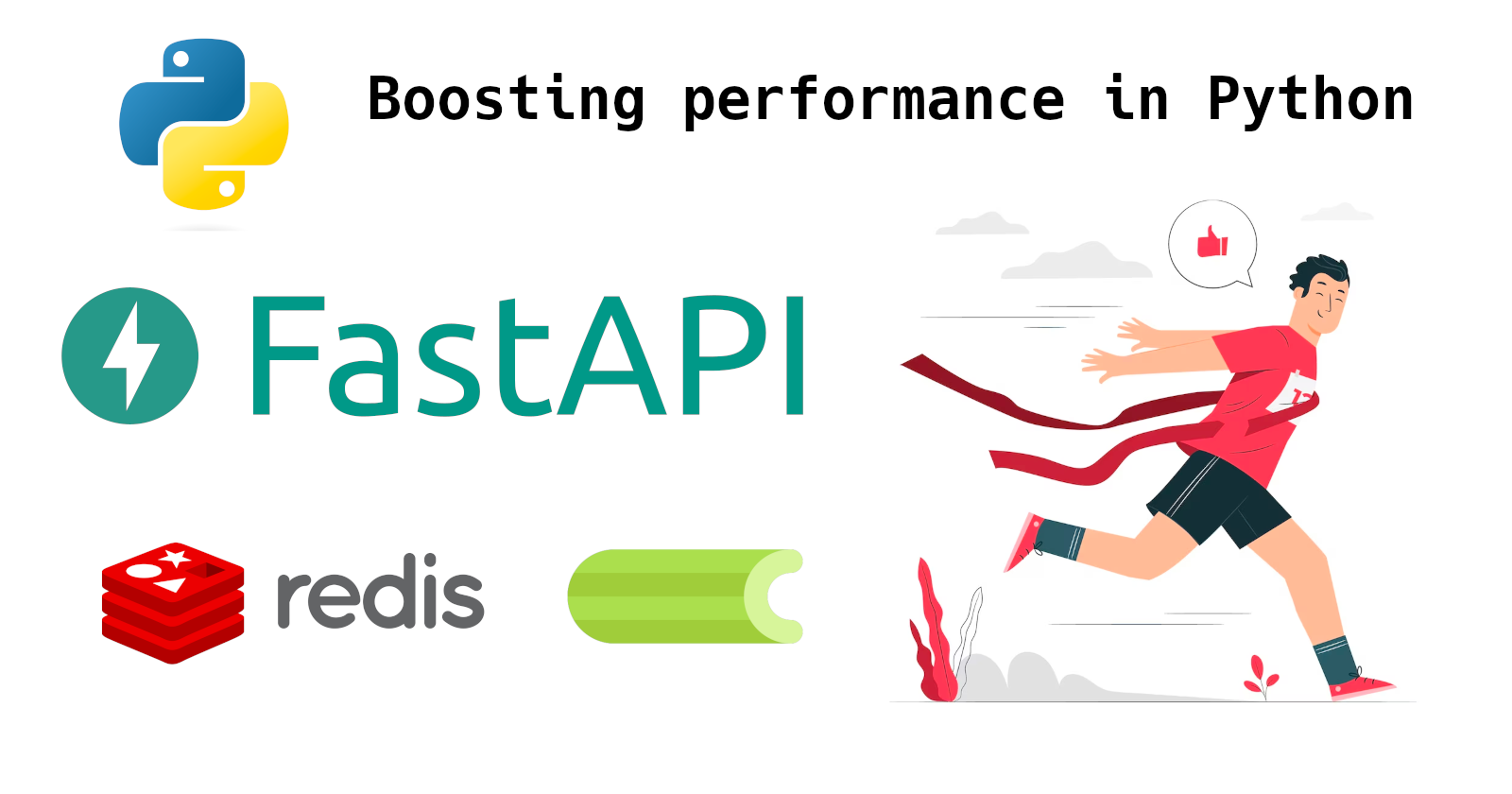
Introduction
In the world of modern web development, responsiveness and scalability are key. FastAPI, a lightning-fast web framework, has gained immense popularity for its speed and ease of use. However, when it comes to handling time-consuming tasks, asynchronous processing becomes essential. Enter Celery, a powerful distributed task queue system. In this blog, we'll explore how to seamlessly integrate Celery with your FastAPI application for efficient asynchronous task execution.
Simpler Explanation
Imagine your FastAPI app as a chef in a busy kitchen. It can only cook one dish at a time. But with Celery, it can send tasks to sous-chefs (workers) who prepare them separately, allowing the chef to keep cooking without waiting for each dish to finish.
Use-cases
Sending email notifications asynchronously.
Processing large images or file uploads in the background.
Handling time-consuming database operations without slowing down the API response.
Running periodic tasks, like data backups.
Distributing CPU-intensive calculations across multiple workers.
Basic Usage
from fastapi import FastAPI
from celery import Celery
app = FastAPI()
# Configure Celery
celery = Celery(__name__, broker='redis://localhost:6379/0')
@celery.task
def async_task(param):
# Your asynchronous task logic here
return f"Processed: {param}"
@app.post("/process")
async def process(param: str):
# Trigger the asynchronous task
result = async_task.delay(param)
return {"message": "Task started", "task_id": result.id}
Advanced Usage - Chaining Tasks and Error Handling
from celery import group, chain
@app.post("/process")
async def process(params: List[str]):
# Create a group of asynchronous tasks
task_group = group(async_task.s(param) for param in params)
# Chain the group with a final task
final_task = task_group | process_result.s()
result = final_task.apply_async()
return {"message": "Task group started", "task_id": result.id}
Further Reading
Conclusion
Integrating Celery with FastAPI can significantly enhance your application's performance and responsiveness. Whether you need to handle background tasks, process data asynchronously, or distribute computational load, this combination empowers you to build robust and efficient web applications. By following the examples and resources provided, you'll be well-equipped to take your FastAPI projects to the next level.
Happy coding!
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
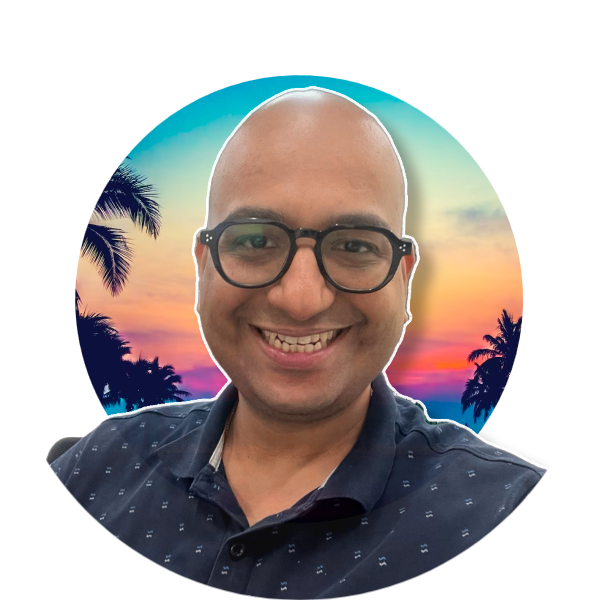
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.