Introduction to functions in C
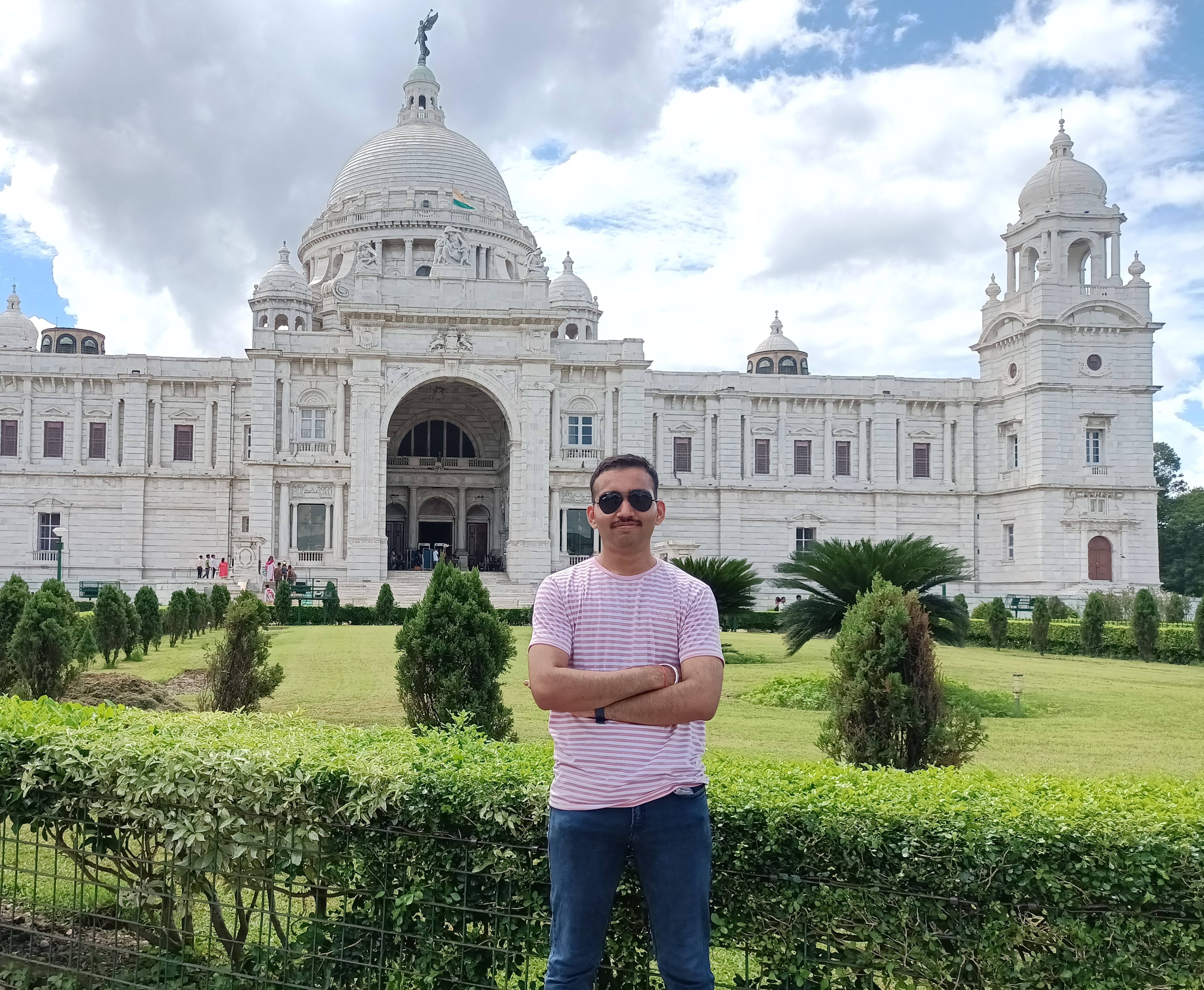
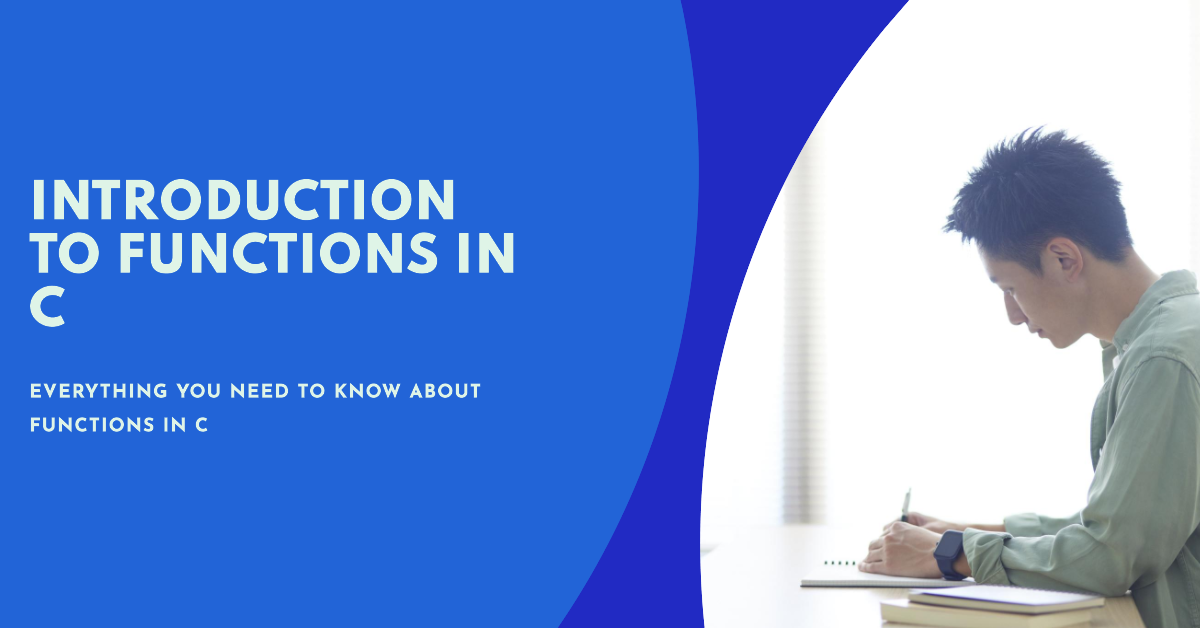
Functions are a block of code that performs a specific task. They allow us to split up our code into smaller, reusable pieces.
They allow code reusability. The same function can be called multiple times from different parts of the code.
They make our code modular and organized. Related code is bundled into a function.
They can make our code more readable and maintainable.
Library Functions
Library functions are pre-defined functions that are available in the standard library. We can use these functions in our C programs without defining them ourselves.
Some examples of library functions are:
printf() and scanf() for input/output
strlen() to find the length of a string
strcpy() to copy a string
malloc() and free() for memory allocation and deallocation
sin(), cos() and tan() for trigonometric functions
sqrt() for square root
abs() for absolute value
In order to use a library function in our code, we need to include the corresponding header file. For example:
To use printf(), we need to #include <stdio.h>
To use strlen(), we need #include <string.h>
To use malloc(), we need #include <stdlib.h>
assert.h, math.h, etc.
User-Defined Functions
User-defined functions are functions that we define ourselves in our C program. As opposed to library functions which are pre-defined in the standard library.
Some reasons to define our own functions are:
Custom functionality - We need to implement some functionality that is not provided by library functions.
Modularity - Splitting our code into functions based on functionality makes it better organized and modular.
Reusability - We can call the function whenever we need that specific functionality in our program.
Function Declaration: The function should be declared prior to its usage.
Syntax: return_type func_name(type1 var1, ...);
Function Definition: Implementation of the function.
Function Call: A function is called by giving its name and passing the required arguments.
Function returning pointers
Functions can return pointers just like any other data type. The return type of the function is simply defined as the pointer data type.
For example:
int *add(int a, int b) { ... } // Function returns an int pointer
char *concat(char *a, char *b) { .. } // Returns char pointer
Passing parameters to functions
Call by Value
Call by value is a method of passing arguments to functions where the value of an argument is copied when the function is called.
The function receives a copy of the actual argument.
Any changes made to the parameter (copy) inside the function do not affect the original argument.
The original argument remains unchanged.
For example:
void func(int a) {
a++; // Increments the local copy 'a'
}
int main() {
int x = 10;
func(x); // Passes the value 10 to func()
printf("x is %d\n", x); // Prints x is 10, since x was not changed
return 0;
}
Call by Reference
Call by reference is a method of passing arguments to a function where the argument's actual memory address is passed to the function.
The function receives the memory address of the argument.
Any changes made to the parameter (which points to the same memory location) inside the function, reflect outside the function.
The original argument is changed.
For example:
void func(int *a) {
(*a)++; // Increments the value at the address *a
}
int main() {
int x = 10;
func(&x); // Pass the address of x
printf("x is %d\n", x); // Prints x is 11
return 0;
}
Subscribe to my newsletter
Read articles from Vishesh Raghuvanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
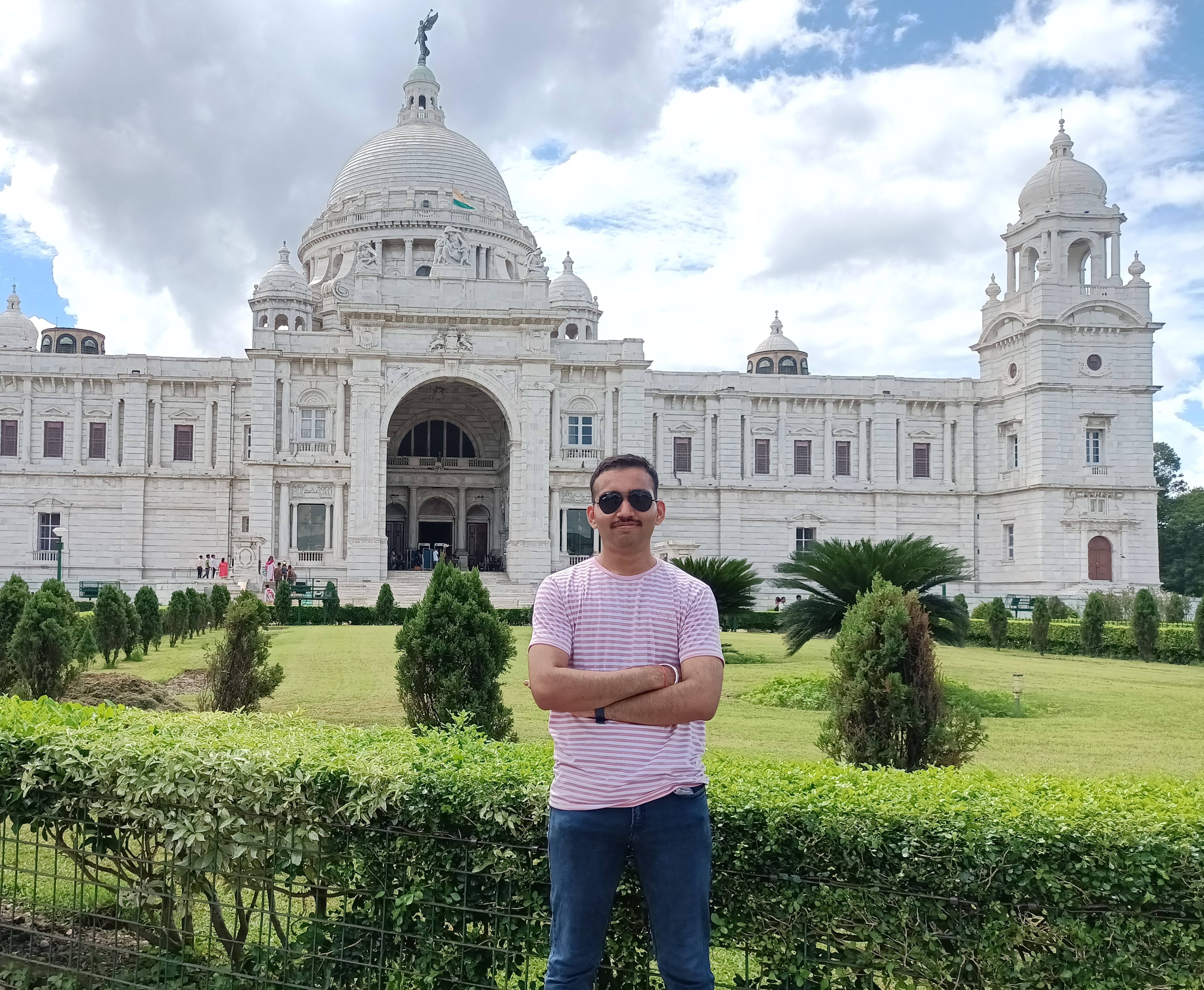