DataTypes of Python
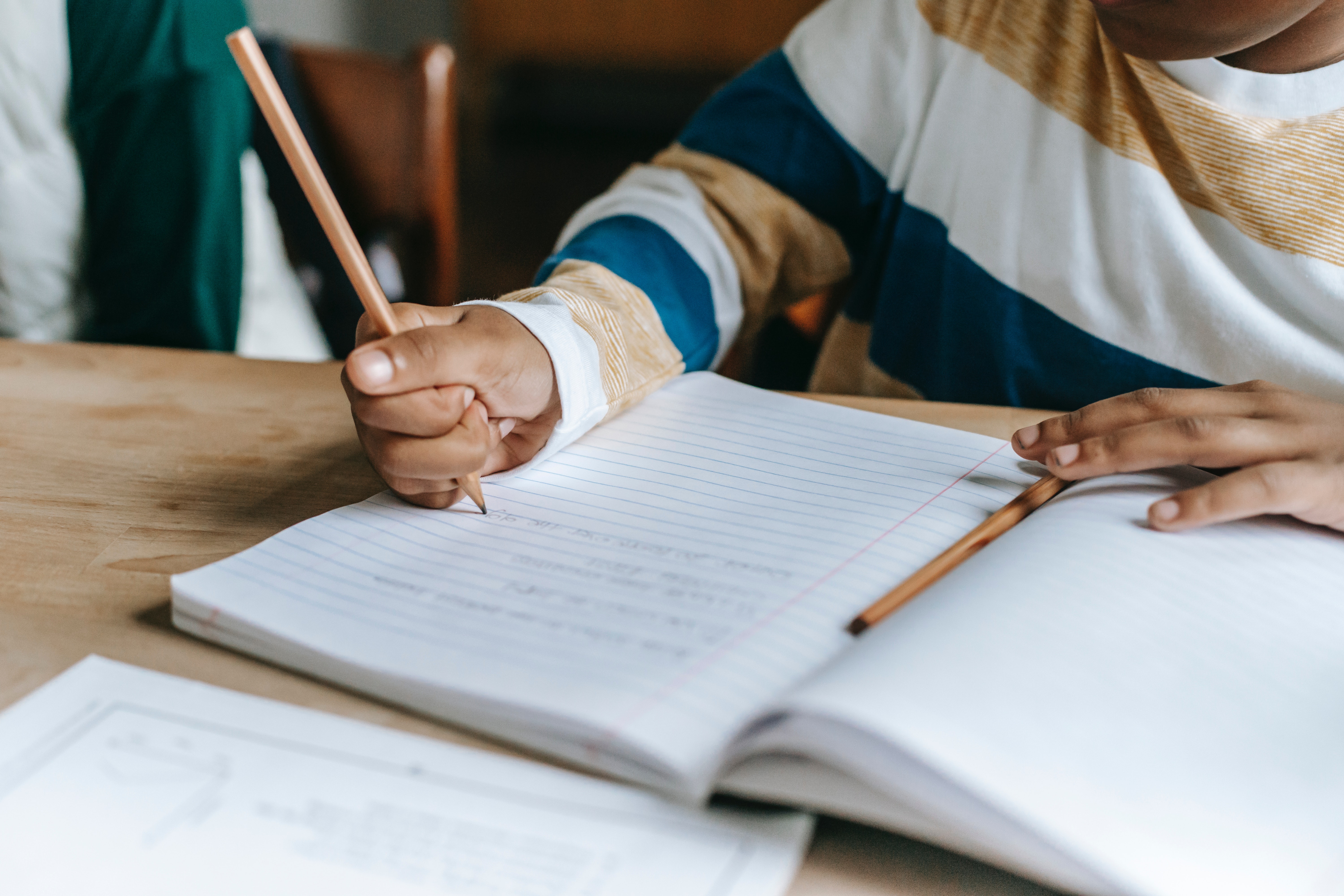
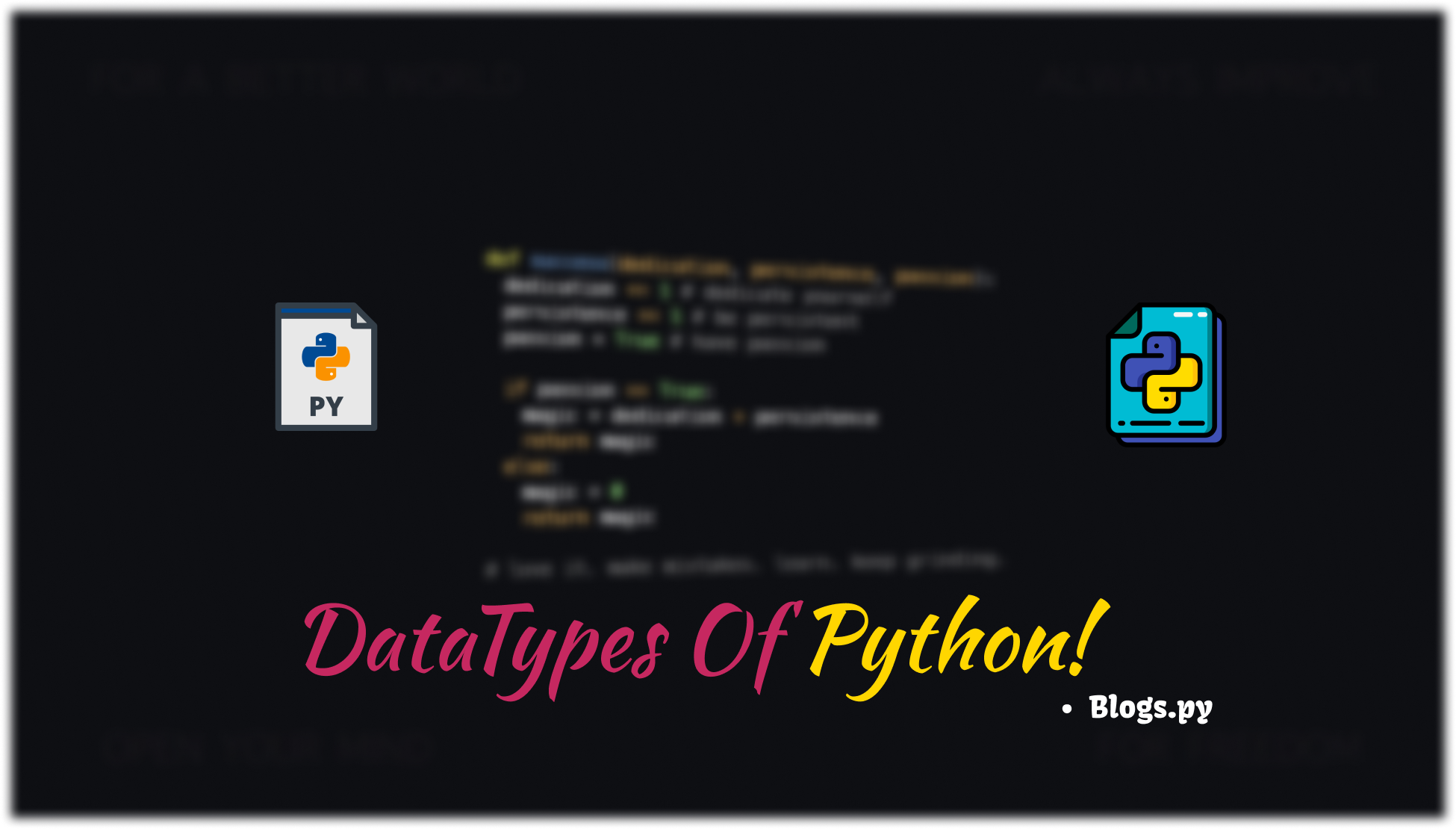
We can understand different datatypes in Python and their usage. Before that, we should understand what are tokens and their types.
Tokens in Python are the basic building blocks of a Python program. There are five types of tokens in Python:
Keywords: Reserved words that have special meanings in Python and cannot be used as identifiers. Examples include
if
,else
for
,while
,def
,import
, etc.Identifiers: Names given to various program elements such as variables, functions, classes, modules, etc. An identifier must start with a letter (a-z, A-Z) or an underscore (_) and can be followed by letters, digits (0-9), or underscores.
Operators: Operators perform operations on variables and values Examples include arithmetic operators (
+
,-
,*
,/
), comparison operators (==
,!=
,<
,>
, etc.), assignment operators (=
,+=
,-=
), etc.Literals: Literals are raw data given in a variable or constant. Examples include numeric literals (e.g.,
42
,3.14
), string literals (e.g.,"hello"
), and boolean literals (True
,False
).Comments: Comments are used to annotate the code for readability and understanding. In Python, comments start with the
#
symbol and continue until the end of the line.
In Python, data types are classifications that categorize the types of values a particular expression can yield. Here are some common data types in Python:
Numeric Types
int: Integer type, representing whole numbers
e.g.,
-3
,0
,42
float: represents decimal numbers
e.g.,
-3.14
,0.0
,3.14159
Sequence Types
str: represents a sequence of characters (String type),enclosed in single or double quotes.
e.g.,
"Hello"
,'Python'
list: represents an ordered collection of items, enclosed in square brackets [] (List type)
e.g.,
[1, 2, 3]
tuple: represents an ordered, immutable collection of items, enclosed in parenthesis. ()
e.g.,
(1, 2, 3)
Boolean Type
- bool: represents truth values
True
orFalse
.
Set Type
set: represents an unordered collection of unique items, enclosed in curly brackets. {}
e.g.,
{1, 2, 3}
Mapping Type
dict: Dictionary type, represents a collection of key-value pairs, enclosed in curly brackets.
e.g.,
{'key': 'value'}
None Type
- None: Represents the absence of a value or a null value,
None
Complex Type
complex: Represents complex number types.
e.g.,
3 + 4j
Understanding and utilizing these data types is essential for effectively working with data and building robust Python programs.
In the following blogs, we can start coding using the print
and input
statement in Python.
Be ready to code !!!
Subscribe to my newsletter
Read articles from Vismaya Prasad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
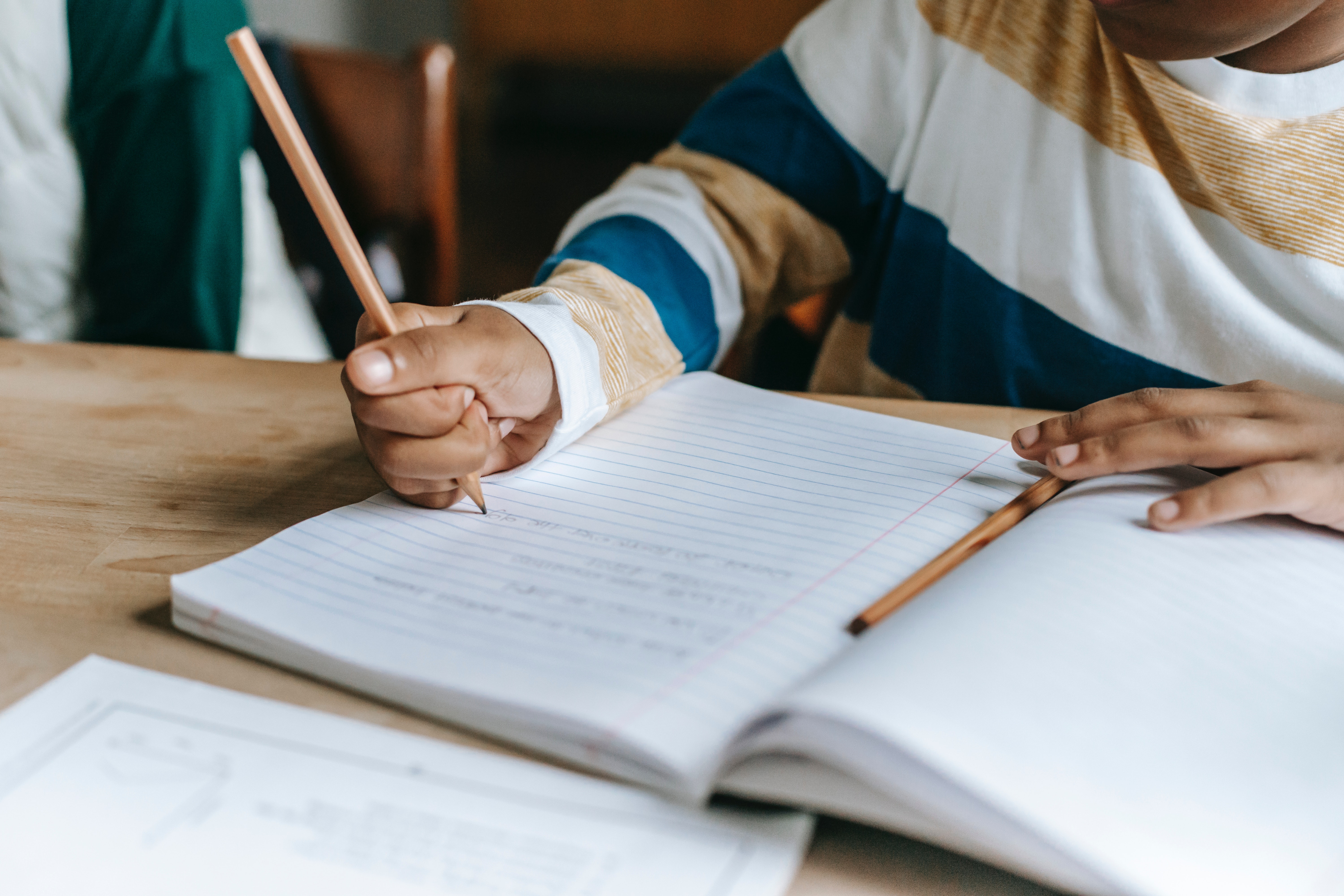
Vismaya Prasad
Vismaya Prasad
I am currently a Computer Science Engineering student focusing on backend development using Django. I usually code in Python. I am also interested technical writing and blogging.