Creating a 9x9 Tic Tac Toe Game with Pygame
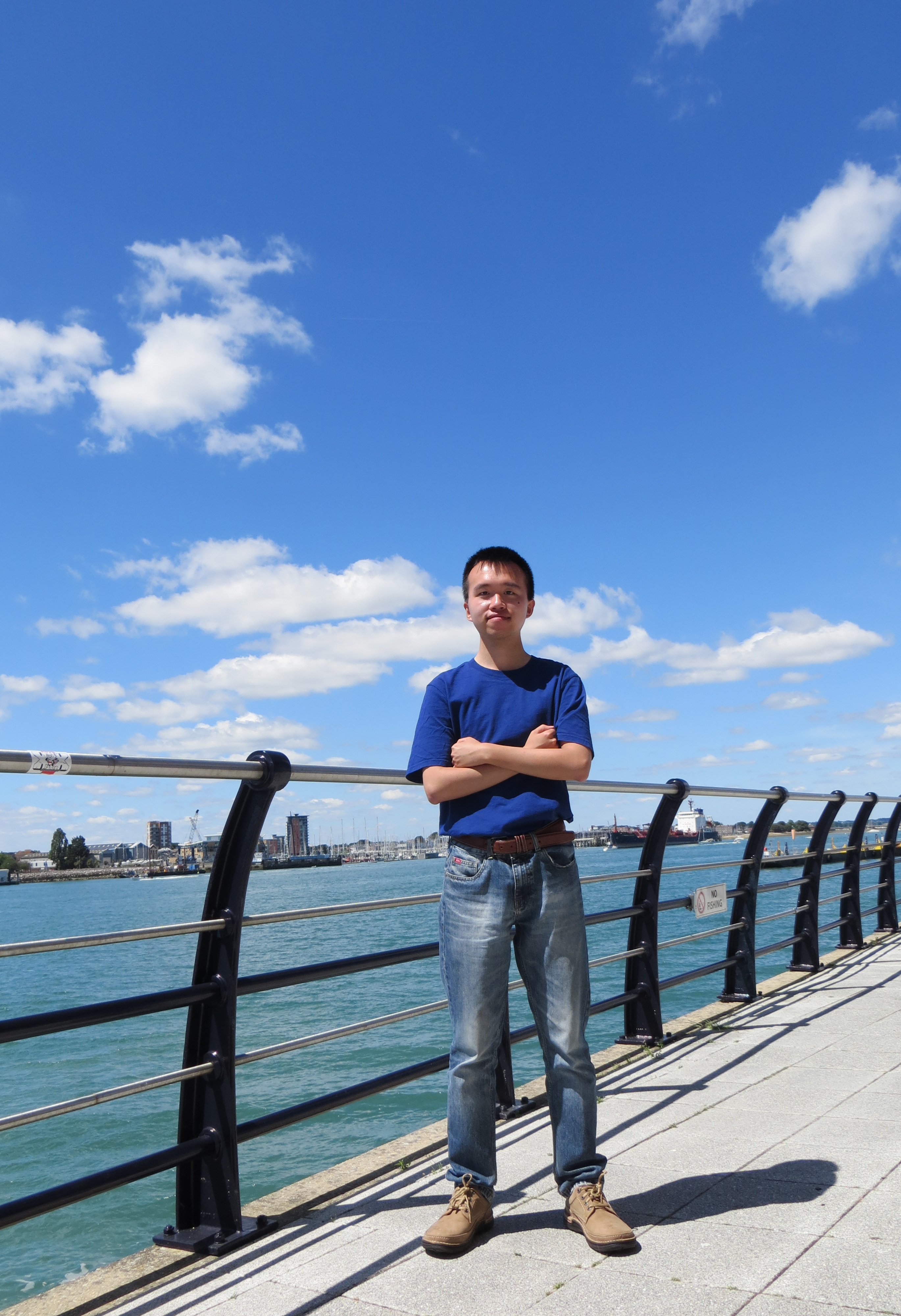
Table of contents
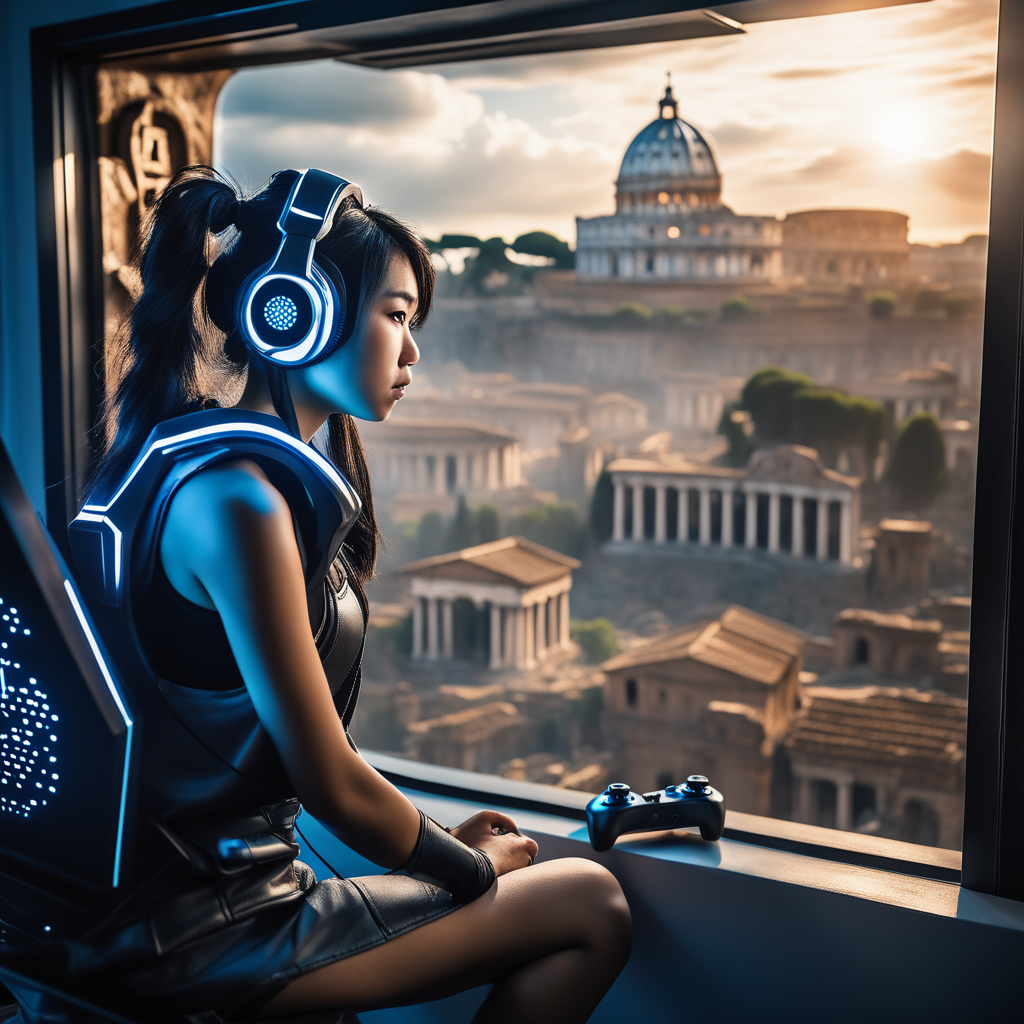
Welcome to this tutorial!
Today, we learn how to create an enhanced version of the classic Tic Tac Toe game. Instead of the traditional 3x3 grid, we will design a more challenging 9x9 version and use Pygame, a library in Python for game design.
Prerequisites:
Basic knowledge of Python.
Familiarity with Pygame would be beneficial but not necessary.
Steps to follow:
Setting Up Your Environment: Before you start coding, make sure you have installed
pygame
. You can install it using pip:pip install pygame
Initialize the Game: Start by importing the necessary modules and defining the constants for the game settings.
import pygame # Constants BOARD_SIZE = 9 CELL_SIZE = 45 WIN_LENGTH = 3 WIDTH, HEIGHT = CELL_SIZE * (BOARD_SIZE + 3), CELL_SIZE * BOARD_SIZE
Create the TicTacToe Class: Our game will be encapsulated within a class called
TicTacToe
. The main functions in this class will be:__init__
Initializes the game settings.draw_main
Draws the main game screen.get_mouse_pos
Gets the position of the mouse.check_win
Checks if there's a winner.switch_turn
Alternates between players.play
Handles the game loop.
Drawing the Board: The
draw_main
function will render our 9x9 grid:def draw_main(self): self.screen.fill((255, 255, 255)) # Filling screen with white for x in range(1, BOARD_SIZE): pygame.draw.line(self.screen, (128, 128, 128), (x * CELL_SIZE, 0), (x * CELL_SIZE, HEIGHT), 1) pygame.draw.line(self.screen, (128, 128, 128), (0, x * CELL_SIZE), (WIDTH - 3*CELL_SIZE, x * CELL_SIZE), 1)
Game Mechanics: Inside
play
, we'll handle user input and game mechanics. When a player clicks on a grid cell, their symbol ("X" or "O") is drawn on the board. After every move, the game checks for a win condition or switches turns.Checking for a Win:
check_win
checks for a winning line of three identical symbols by iterating through the grid horizontally, vertically, and diagonally.Main Game Loop: Our game is managed by the main loop inside the
play
function. This loop constantly checks for events, updates the game state, and redraws the screen.Executing the Game: Wrap up by initializing Pygame and running the game loop:
if __name__ == "__main__": pygame.init() game = TicTacToe() game.play() pygame.quit()
Conclusion:
In this tutorial, we dove deep into creating a 9x9 Tic Tac Toe game using Pygame. We explored how to draw the game board, handle player input, check for win conditions, and manage the game loop. This version provides a fresh take on the classic game and offers a higher level of challenge.
Remember, game development is a vast field, and this is just a stepping stone. You can expand upon this game by adding features, such as player vs. computer mode, enhanced graphics, or even an AI opponent!
Happy coding!
Subscribe to my newsletter
Read articles from Haocheng Lin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
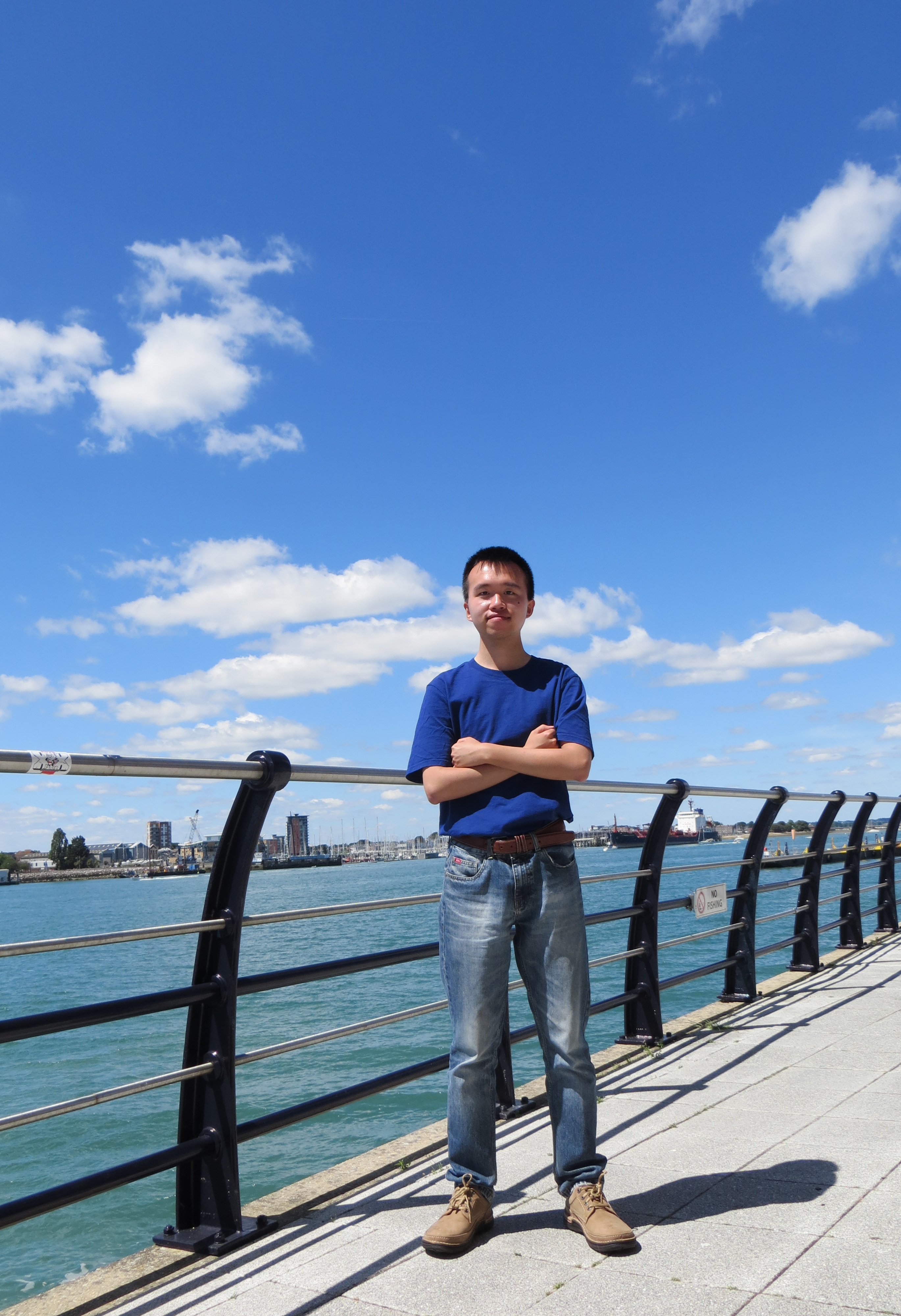
Haocheng Lin
Haocheng Lin
๐ป AI Developer & Researcher @ Geologix ๐๏ธ UCL MEng Computer Science (2019 - 23). ๐๏ธ UCL MSc AI for Sustainable Development (2023 - 24) ๐ฅ Microsoft Golden Global Ambassador (2023 - 24)๐