Use of Javascript Closures in React App
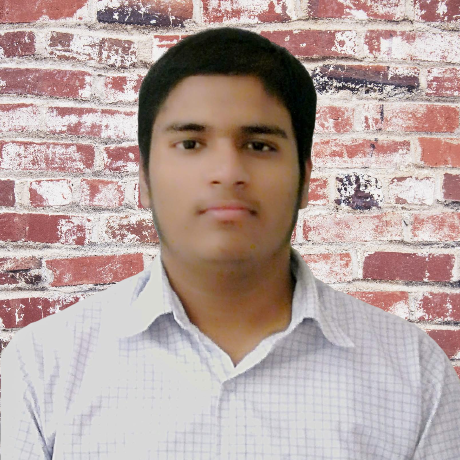
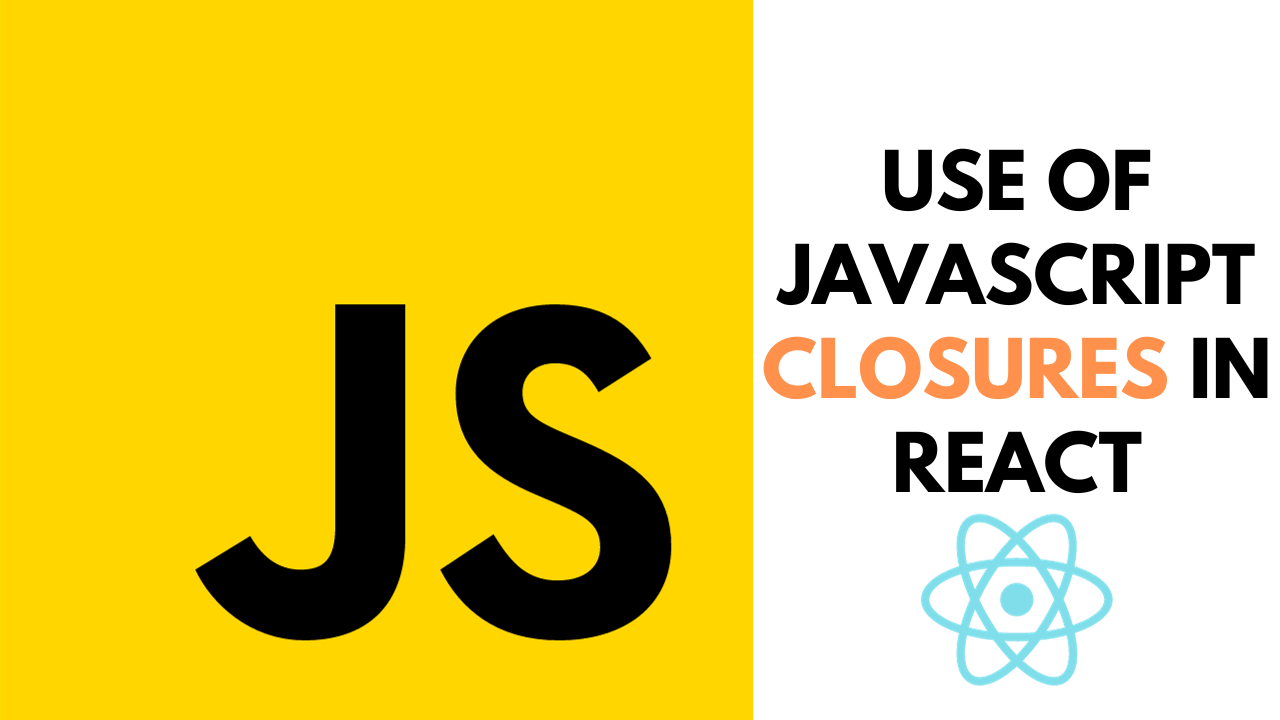
Introduction
Picture yourself enjoying your favourite latte at a coffee shop. Sitting at a corner table, you overhear two friends having a conversation. One of them shares a confidential secret with the other and asks them to keep it to themselves. Later, when you talk to a friend who was also present during the conversation, they still remember the secret and keep it private. This scenario is similar to how closures work in JavaScript. Like how the friend remembers the secret even after the conversation ends, closures allow functions to access variables from their surrounding scope, even after that scope has finished executing. This powerful concept is essential in many JavaScript and React applications, enabling them to efficiently manage data, handle events, and create encapsulated functionality.
In the world of JavaScript and React, numerous concepts and techniques can make your code more efficient and flexible. One such concept that often puzzles developers but proves to be incredibly useful is JavaScript closures. In this blog post, we'll demystify closures, explore their real-world use cases in React, and illustrate their importance through practical examples.
Understanding JavaScript Closures
To fully understand closures, it is crucial to comprehend the idea of lexical scope. In JavaScript, functions possess access to variables from their surrounding scope. If a function is defined within another function, it creates a closure, allowing the inner function to access the outer function's variables and parameters, even after the outer function has finished executing.
Here's a simple example:
function outer() {
const outerVar = 10;
function inner() {
console.log(outerVar); // Accesses outerVar from the outer function
}
return inner;
}
const closureFunc = outer();
closureFunc(); // Outputs 10
In this example, inner
is a closure because it maintains access to the outerVar
variable even after outer
has finished executing. This is incredibly useful in React for various scenarios.
Use Cases of Closures in React
1. Event Handlers with Closures
Closures can be used effectively in React to handle events with dynamic data. Consider a scenario where you want to create a list of buttons, each with its unique value:
function ButtonList() {
const values = [1, 2, 3, 4, 5];
return (
<div>
{values.map((value) => (
<button key={value} onClick={() => handleButtonClick(value)}>
Button {value}
</button>
))}
</div>
);
}
In this example, the onClick
handler creates a closure around value
, ensuring that each button knows its corresponding value when clicked.
2. Private Data and Encapsulation
JavaScript closures are also useful for creating private variables and encapsulating data within React components. For instance, consider building a counter component with a private counter variable:
function Counter() {
let count = 0;
return {
increment: () => {
count++;
console.log(`Count: ${count}`);
},
decrement: () => {
count--;
console.log(`Count: ${count}`);
},
};
}
const myCounter = Counter();
myCounter.increment(); // Outputs: Count: 1
myCounter.decrement(); // Outputs: Count: 0
In React, you can achieve similar encapsulation by using closures to maintain the component state and hide it from the outside world.
3. Memoization for Performance Optimization
Caching expensive function results using memoization is made possible with closures. In React, you can use closures within functional components to significantly improve performance.
function ExpensiveCalculation() {
const cache = {};
return (input) => {
if (input in cache) {
return cache[input];
} else {
const result = /* Perform expensive calculation */;
cache[input] = result;
return result;
}
};
}
Conclusion
In React development, JavaScript closures can be a valuable asset. With closures, you can efficiently create encapsulated and maintainable code for a variety of real-world scenarios. This includes handling dynamic data in event handlers, encapsulating private data, and optimizing performance through memoization. By embracing closures, you can unlock their potential and enhance your React projects.
Subscribe to my newsletter
Read articles from Rishikesh Shinde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
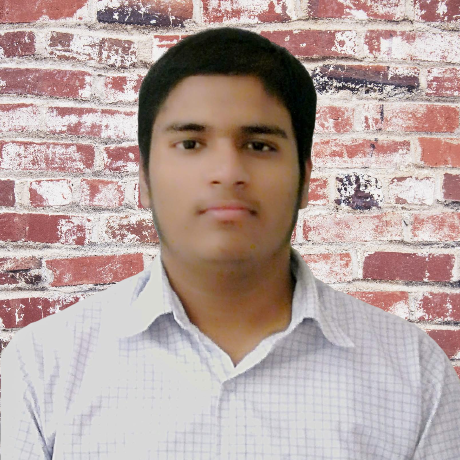
Rishikesh Shinde
Rishikesh Shinde
A person who is attracted by the world of computer science and very much passionate to learn development.