Code Quality Analysis

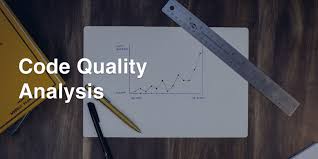
Introduction
The two most popular tools that resonate with code quality check are SonarQube and SonarLint.
SonarQube is a powerful code quality management platform designed for teams and organizations. It conducts static code analysis to detect and report issues like bugs, security vulnerabilities, and code smells in codebases. It offers customizable coding rules, technical debt tracking, and quality gates, making it an essential tool for maintaining high code quality standards. SonarQube integrates seamlessly into CI/CD pipelines, providing a centralized view of code quality metrics and trends, and facilitating informed decision-making for software development projects.
SonarLint, on the other hand, is a lightweight code analysis tool tailored for individual developers. It operates directly within integrated development environments (IDEs) like IntelliJ IDEA and Visual Studio, offering real-time feedback on code issues as developers write and modify code. SonarLint highlights issues, provides explanations, and suggests fixes, making it an invaluable companion for developers striving to write cleaner, more maintainable code. Developers can customize rules to match project-specific coding standards, ultimately helping teams improve code quality from the ground up.
Steps to integrate SonarQube in java-gradle project
Install SonarQube:
- Ensure that you have SonarQube installed and running. You can download and set up SonarQube from the official website (https://www.sonarqube.org/downloads/).
Configure SonarQube in Gradle:
Open your
build.gradle
file and add the following dependencies:You can generate an authentication token in SonarQube by navigating to "My Account" -> "Security" -> "Generate Tokens."
Run SonarQube Analysis:
In your terminal, navigate to your project's root directory and run the following Gradle command to perform the SonarQube analysis:
Replace
./gradlew
withgradlew.bat
on Windows.
View SonarQube Results:
Open your web browser and access the SonarQube dashboard at the URL you specified in the Gradle configuration (e.g., http://localhost:9000).
Log in with your credentials, and you'll see the code analysis results for your Gradle project.
Analyze and Address Issues:
- SonarQube will provide a detailed report of code quality issues, code smells, and other metrics. Review these findings and address the identified issues in your code.
Continuous Integration:
- To integrate SonarQube into your CI/CD pipeline, you can automate the analysis step by adding the
sonarqube
task to your build scripts or CI configuration.
- To integrate SonarQube into your CI/CD pipeline, you can automate the analysis step by adding the
Integrate SonarLint to Intellij IDEA
Install IntelliJ IDEA: Ensure you have IntelliJ IDEA installed on your system. If not, you can download it from the JetBrains website (https://www.jetbrains.com/idea/download/).
Install SonarLint Plugin: Open IntelliJ IDEA and go to "File" -> "Settings" (or "IntelliJ IDEA" -> "Preferences" on macOS).
In the settings window, select "Plugins" from the left sidebar.
In the "Plugins" window, click on the "Marketplace" tab.
Search for "SonarLint" in the marketplace search bar.
Click the "Install" button next to the "SonarLint" plugin.
After installation, you may need to restart IntelliJ IDEA to activate the plugin.
Configure SonarLint:
Once IntelliJ IDEA has restarted, open your project.
In the bottom-right corner of the IntelliJ IDEA window, you will see a "SonarLint" tab. Click on it.
Click the "Connect to SonarQube or SonarCloud" button to configure SonarLint with your SonarQube server or SonarCloud account. You'll need to provide the server URL, authentication token, and project key.
Enable SonarLint in Your Project:
- After configuration, you can enable SonarLint for your project by clicking the "Enable in this Project" button in the SonarLint tab.
Code Analysis:
As you write or modify code, SonarLint will perform real-time analysis and highlight code issues.
You can click on the highlighted issues to see more details and recommended fixes.
Customize Rules (optional):
SonarLint comes with a set of predefined rules, but you can customize the rule set to match your project's coding standards.
To customize rules, go to "File" -> "Settings" (or "IntelliJ IDEA" -> "Preferences" on macOS) -> "Editor" -> "Inspections" -> "SonarLint," and adjust the rule settings there.
Resolve Issues:
To fix issues reported by SonarLint, you can apply recommended fixes directly from the IDE.
Right-click on an issue and choose "Show Quick Fixes" to see available solutions.
By following these steps, you can integrate SonarLint into IntelliJ IDEA and leverage its real-time code analysis capabilities to improve the quality of your code as you write it. This integration helps you catch and address code quality issues early in the development process.
Best Practices
Early Integration: Integrate SonarQube into your development process as early as possible, ideally during the CI/CD pipeline. This allows you to catch and address code quality issues before they become deeply ingrained in your project.
Set Quality Gates: Define quality gates with strict criteria for code quality and enforce them in your CI/CD pipeline. This ensures that only code meeting certain quality standards is allowed to be merged or deployed.
Customize Rules: SonarQube comes with a wide range of built-in rules, but not all may be applicable to your project. Customize the rule set to fit your specific coding standards and requirements. Opinions on which rules to enable or disable can vary based on the project, but it's essential to strike a balance between catching real issues and avoiding noise.
Regular Analysis: Run code analysis regularly, preferably after every code commit. This helps in identifying and addressing issues early, preventing technical debt from piling up.
Prioritize Technical Debt: Pay attention to the "Technical Debt" metric in SonarQube. It quantifies the effort required to fix code issues. Prioritize addressing high-impact technical debt items that have a significant impact on code quality and maintainability.
Involve Developers: Developers should actively participate in addressing code quality issues. Make it a part of the development process to fix issues as they arise. Encourage a culture of code ownership and responsibility.
Monitor Trends: Use SonarQube to monitor code quality trends over time. Opinions may vary on specific thresholds, but it's generally essential to track whether code quality is improving or deteriorating and take action accordingly.
Use Pull Requests: If possible, integrate SonarQube with your code review process. It can provide feedback on code quality in pull requests, making it easier for reviewers to catch issues early.
Educate the Team: Ensure that the development team understands the importance of code quality and how to interpret SonarQube reports. Training and knowledge sharing can go a long way in maintaining code quality.
Balance Automation and Human Review: While automation through SonarQube is valuable, remember that it's not a substitute for human code review. Combine automated checks with manual code reviews to catch issues that automated tools might miss.
Regularly Update SonarQube: Keep your SonarQube installation up to date with the latest version and plugins. Updates often include bug fixes, performance improvements, and new rules that can enhance its effectiveness.
Consider False Positives: Acknowledge that automated tools like SonarQube can sometimes produce false positives. It's crucial to review and validate reported issues to avoid unnecessary code changes.
Common SonarQube issues for java code
Null Pointer Dereference:
Fix: Check for null before accessing an object's methods or properties. Unused Variables or Methods: Fix: Remove or refactor unused variables and methods to declutter the codebase. Code Duplication: Fix: Identify and consolidate duplicated code into reusable functions or classes.
Cognitive Complexity:
Fix: Break down complex functions into smaller, more manageable parts, and use proper abstraction and encapsulation.
Inefficient Resource Management:
Fix: Ensure proper resource management, such as closing files, database connections, and streams, using try-with-resources or finally blocks.
Security Vulnerabilities (e.g., SQL Injection):
Fix: Use prepared statements or parameterized queries to prevent SQL injection attacks. Sanitize user inputs before using them in queries.
Unused Imports:
Fix: Remove unused import statements to keep the codebase clean and improve readability.
Inefficient Data Structures and Algorithms:
Fix: Choose appropriate data structures and algorithms for the task to improve performance and resource usage.
Unchecked Exceptions:
Fix: Handle or propagate checked exceptions appropriately, or document why an exception is intentionally left unchecked.
Complex Conditional Statements:
Fix: Simplify complex if-else or switch statements by refactoring or using more straightforward logic
Conclusion In conclusion, SonarQube is a valuable tool for improving code quality, but its effectiveness depends on how well it's integrated into your development process and how seriously the team takes code quality. The best practices and opinions outlined above should help you get the most out of SonarQube while adapting to your specific project's needs.
Subscribe to my newsletter
Read articles from Debajyoti Dutta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
