Text Preprocessing for Effective Natural Language Processing (NLP) Tasks
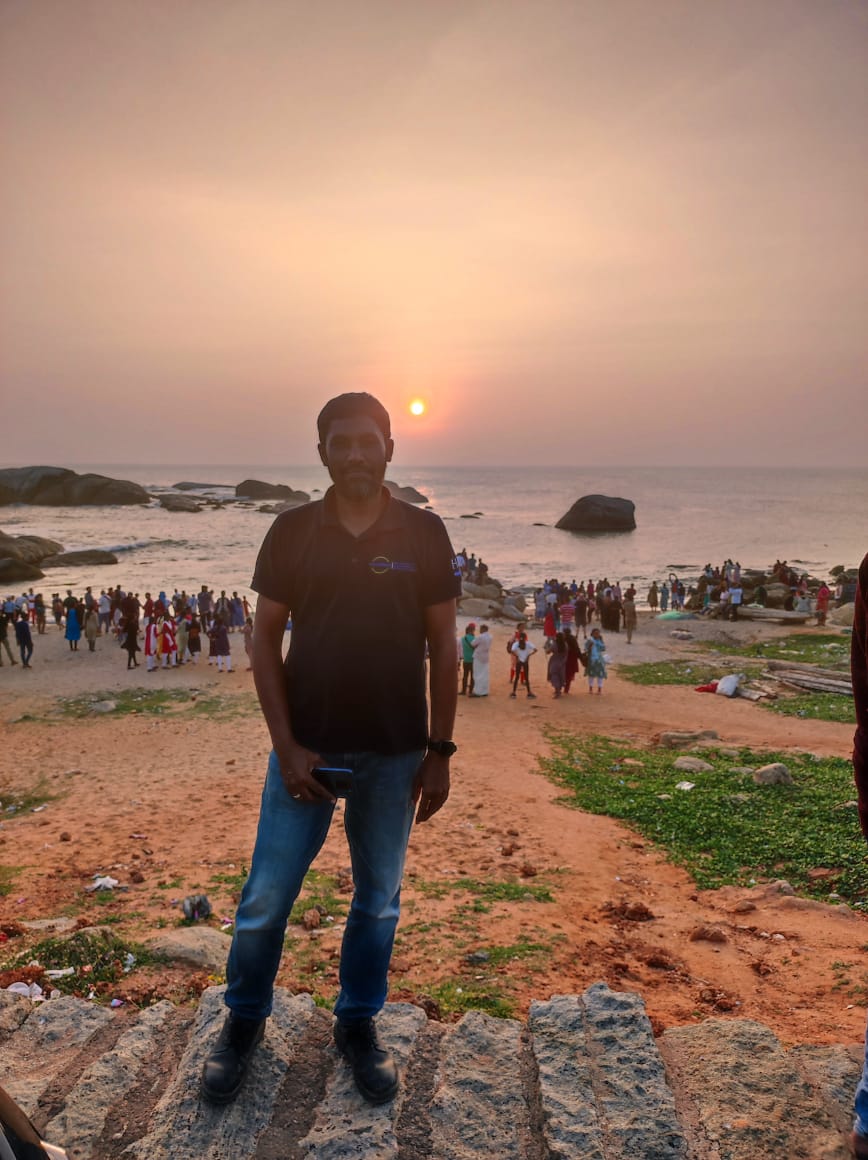
When working with text data for Natural Language Processing (NLP) tasks like sentiment analysis or text classification, it's essential to perform text preprocessing. It involves cleaning and transforming raw text data into a format that can be effectively used for various NLP tasks.
Preprocessing steps often include:
Tokenization:
Splitting text into individual words or tokens.
Lowercasing:
Converting all text to lowercase to ensure consistent matching.
Removing Punctuation:
Eliminating punctuation marks.
Stopword Removal:
Removing common words like "and," "the," "in," which may not carry significant meaning.
Stemming or Lemmatization:
Reducing words to their base or root form (e.g., "running" to "run").
Handling Numerical Values:
Decide whether to treat numbers as-is, replace them with a special token, or remove them.
Handling Special Characters:
Address special characters and symbols appropriately.
Here's a sample code snippet to perform these preprocessing steps using Python and the NLTK library:
import nltk
nltk.download('punkt')
nltk.download('stopwords')
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
from nltk.stem import PorterStemmer
import string
# Sample text
text = "Natural language processing is a computational analysis of human language. It allows computers to respond to context clues in the same way a human would."
# Tokenization
tokens = word_tokenize(text)
# Lowercasing and removing punctuation
tokens = [word.lower() for word in tokens if word.isalnum()]
# Stopword removal
stop_words = set(stopwords.words('english'))
filtered_tokens = [word for word in tokens if word not in stop_words]
# Stemming
stemmer = PorterStemmer()
stemmed_tokens = [stemmer.stem(word) for word in filtered_tokens]
print(stemmed_tokens)
Output
['natur', 'languag', 'process', 'comput', 'analysi', 'human', 'languag', 'allow', 'comput', 'respond', 'context', 'clue', 'way', 'human', 'would']
Proper text preprocessing can significantly improve the performance of your NLP models by reducing noise and ensuring consistent representations of text data.
#NLP #TextPreprocessing
Subscribe to my newsletter
Read articles from K Ahamed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
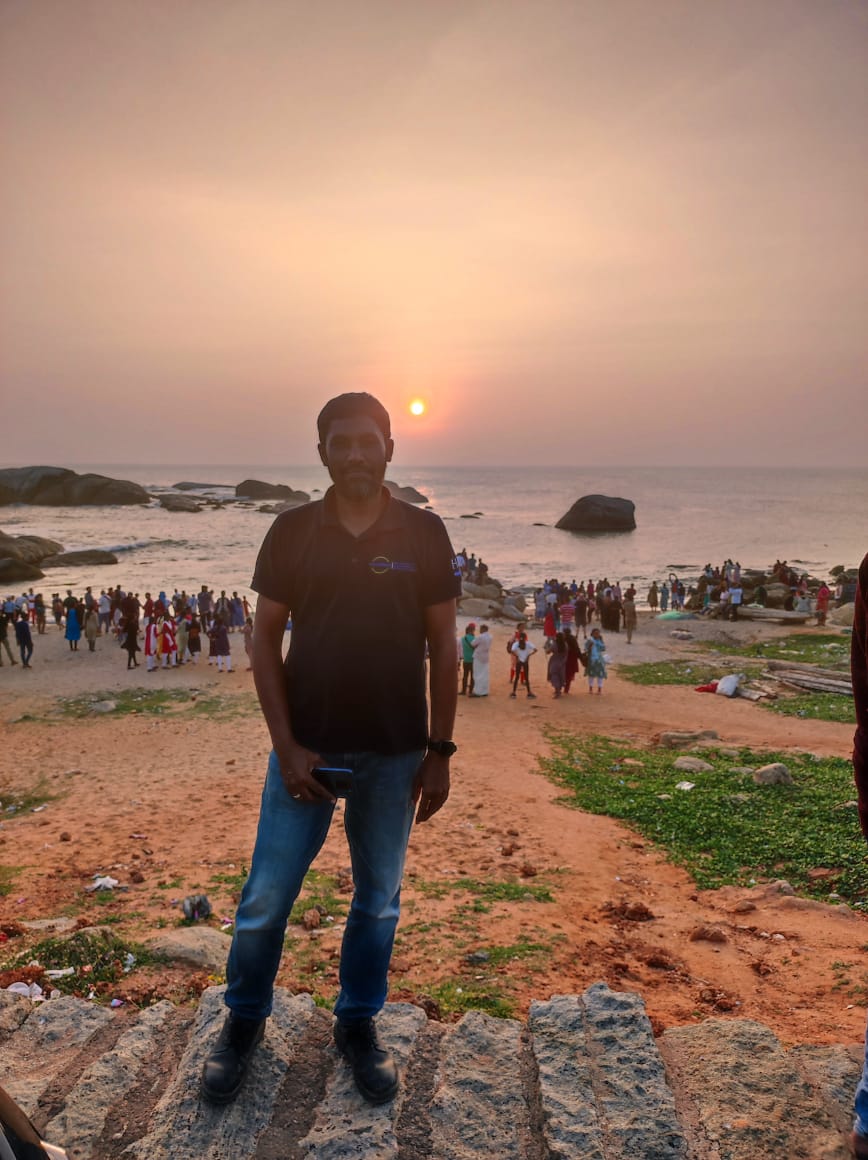
K Ahamed
K Ahamed
A skilled construction professional specializing in MEP projects. Armed with a Master's degree in Data Science, seamlessly combines hands-on expertise in construction with a passion for Python, NLP, Deep Learning, and Data Visualization. While currently at a basic level, dedicated to enhancing data skills, envisioning a future where insights derived from data reshape the landscape of construction practices. With a forward-thinking mindset, building structures but also shaping the future at the intersection of construction and data.