Integrating WebView in Compose Android: A Comprehensive Guide

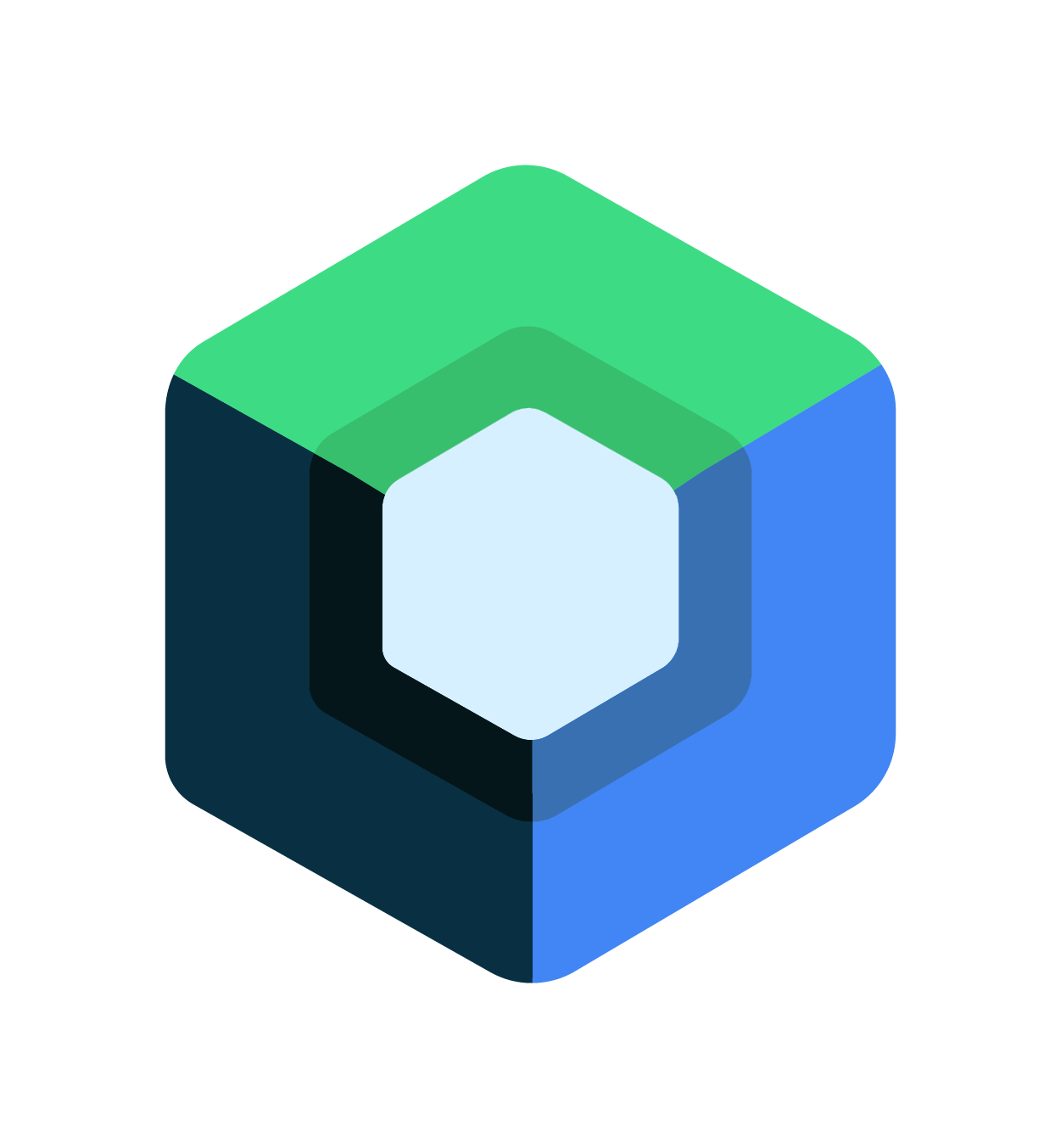
Introduction to Webview in Android Compose
Jetpack Compose is very powerful and versatile making it possible to integrate webview in your mobile application. WebView allows you to embed and interact with web resources in your mobile application without opening your regular browser such as Chrome.
Integrating webview in your mobile application enhances swift user interaction saving the stress of navigating to another app browser.
In this guide, you will understand the concept of Webview and a detailed explanation of how to integrate them into your mobile application.
Prerequisite
Kotlin programming language
Android Studio installed on your PC
Ensure you have basic knowledge of event handling in compose as well as how to create composable layouts
Add the following dependency to your build.gradle.kts(Module:app) for navigation between screens in compose
implementation("androidx.navigation:navigation-compose:")
Go to your Android Manifest XML file and add internet permission
<uses-permission android:name="android.permission.INTERNET" />
Understanding WebView
The WebView is an AndroidView Accompanist for jetpack compose. Accompanist is a set of libraries that serves as a supplement for developers for features that are not readily available. The webview extends(inherits) from the Android view class. This can be integrated or implemented by adding the dependency to your mobile app as well as the vital functions.
However, Webview is not exactly a web browser like your Chrome etc. because it lacks some functionality that a regular browser has such as a search bar, incognito tabs, desktop functionalities, etc.
Webview is mostly used in cases where developers integrate functionalities like using social media accounts to log into an application. Other areas where it is applied is in the privacy policies of our mobile applications which is very common in almost every mobile application including websites.
For example, if you are familiar with the popular Twitter mobile application now called X, when you scroll down to the privacy policy section, and click on it you will notice that it will navigate you to another screen inside the application with the option at the top to open in the default browser. That is a good integration of the Webview library.
Implementation of Webview in Jetpack Compose
To implement the webview in Jetpack compose you have to understand the following functions in the Android View library webview class.
In creating a web view in compose you have to create a composable function. The major functions you will use and come across while integrating your Webview in Jetpack Compose are listed:
1. URL: Which stands for uniform resource locator, it is the URL of the webpage to be loaded in your webView which takes a string as a parameter
2. LocalContext: Mainly used for initializing and instantiating the Webview that represents the current Android context.
3. Webviewclient: Sets the WebViewClient that will receive various notifications and requests. This will replace the current handler.
4. settings.javaScriptEnabled = true: Tells the Web view to enable javascript the default is false. It is set to true if you want your Webview to enable javascript
Note: Before introducing this function you must consider it as it may expose your application to certain security threats.
5. Android View: This is an extension of the view class that allows you to modify your Webview in terms of appearance as well as load the URL of your webpage
6. Factory: This block is responsible for creating the view to be composed
7. modifier: This function is applied to the layout to give the Webview screen a customized view, such as sizing the width, or height.
8. Update: The update function is invoked after the layout has been inflated. It updates the information and state of view upon recomposition.
Adding a Basic Webview to a Compose Layout
Adding a basic Webview is very simple using the following code :
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
WebViewPage(url = "https://developer.android.com/studio")
}
}
}
@Composable
fun WebViewPage(url: String) {
val context = LocalContext.current
val webView = remember { WebView(context).apply {
webViewClient = WebViewClient()
settings.javaScriptEnabled = true
settings.cacheMode = WebSettings.LOAD_NO_CACHE
}
}
AndroidView(
modifier = Modifier
.fillMaxSize(),
factory = { webView },
update = {
it.loadUrl(url)
})
}
In the above code, a simple web view was added, this will open upon launching your application, you have to create a composable function inside your main activity or preferably in another file. And call that function inside the main activity to display your Webview
Below is a screenshot of the output
Using a button Composable to open WebView within the Application
This is very easy to implement, You start by creating two screen composable functions. The main screen which is the homepage should contain a button that will enhance navigation to the webview screen. The second screen will contain the Webview page. You will implement the nav controller that will help you navigate the two screens.
- Create another package for navigation: This will help you control the navigation on both screens.
- Create a package for the UI which is the home screen and web view screen composable.
3. Creating your composable files here is a screenshot below
HomePageScreen
@Composable
fun OpenWebView(navController: NavController){
Column(modifier = Modifier
.fillMaxSize(),
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.Center
) {
Button(onClick = {
navController.navigate("webviewscreen")
},
shape = RoundedCornerShape(12.dp),
colors = ButtonDefaults.buttonColors(MaterialTheme.colorScheme.onPrimaryContainer),
modifier = Modifier
.width(200.dp)
.height(50.dp)
) {
Text(text = "Open WebView")
}
}
}
Explanation
This is the Homepage screen composable function which contains a button composable that will help you navigate to the webview screen on action click. The navcontroller.navigate plays the action of navigating the user to the Webview screen. Little customization was added to the button by adding a background color.
WebviewPageScreen
@Composable
fun WebViewPage(url: String, navController: NavController) {
val context = LocalContext.current
val webView = remember { WebView(context).apply {
webViewClient = WebViewClient()
settings.javaScriptEnabled = true
settings.cacheMode = WebSettings.LOAD_NO_CACHE
}
}
AndroidView(
modifier = Modifier
.fillMaxSize(),
factory = { webView },
update = {
it.loadUrl(url)
})
}
This is the WebViewPage composable function that will display your webpage, the URL is your webpage link to be loaded, and the Android view enables properties for modification of the layout. When the button in the homePage is clicked the web view of your website launches.
Navigation
@Composable
fun AppNavigation(){
val navController = rememberNavController()
NavHost(navController = navController, startDestination = "homepage" ){
composable("homepage"){
OpenWebView(navController = navController)
}
composable("webviewscreen"){
WebViewPage("https://developer.android.com/studio", navController)
}
}
}
The navigation composable function helps in navigating through screens by stating your destination and managing the back stack. From the above code upon launching the application the first destination which is the home page displays first. The destination takes a string as a parameter. Then it navigates to the Webview page. The remebernav controller creates an instance of the NavController and remembers it for the lifetime of the composable function or the associated screen.
MainActivity
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
AppNavigation()
}
}
}
The main activity that I assume you know as the entry point of your mobile application calls the app navigation composable function.
Conclusion
Integrating Webview in Compose is not as complex as you think, in this article, I have comprehensively explained all you need to know about Webview and its integration in Jetpack Compose.
With this knowledge, you can display web resources in your mobile application user interface and enhance smooth user experience.
Subscribe to my newsletter
Read articles from Etugbo Judith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Etugbo Judith
Etugbo Judith
I'm a Technical writer passionate about breaking down complex concepts into clear, concise and engaging content.