Understanding Type, Reference, and Undefined Errors in JavaScript
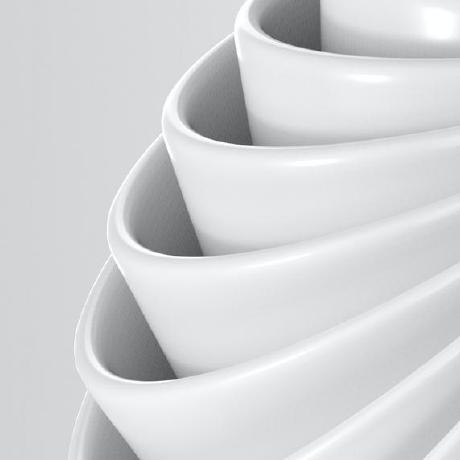
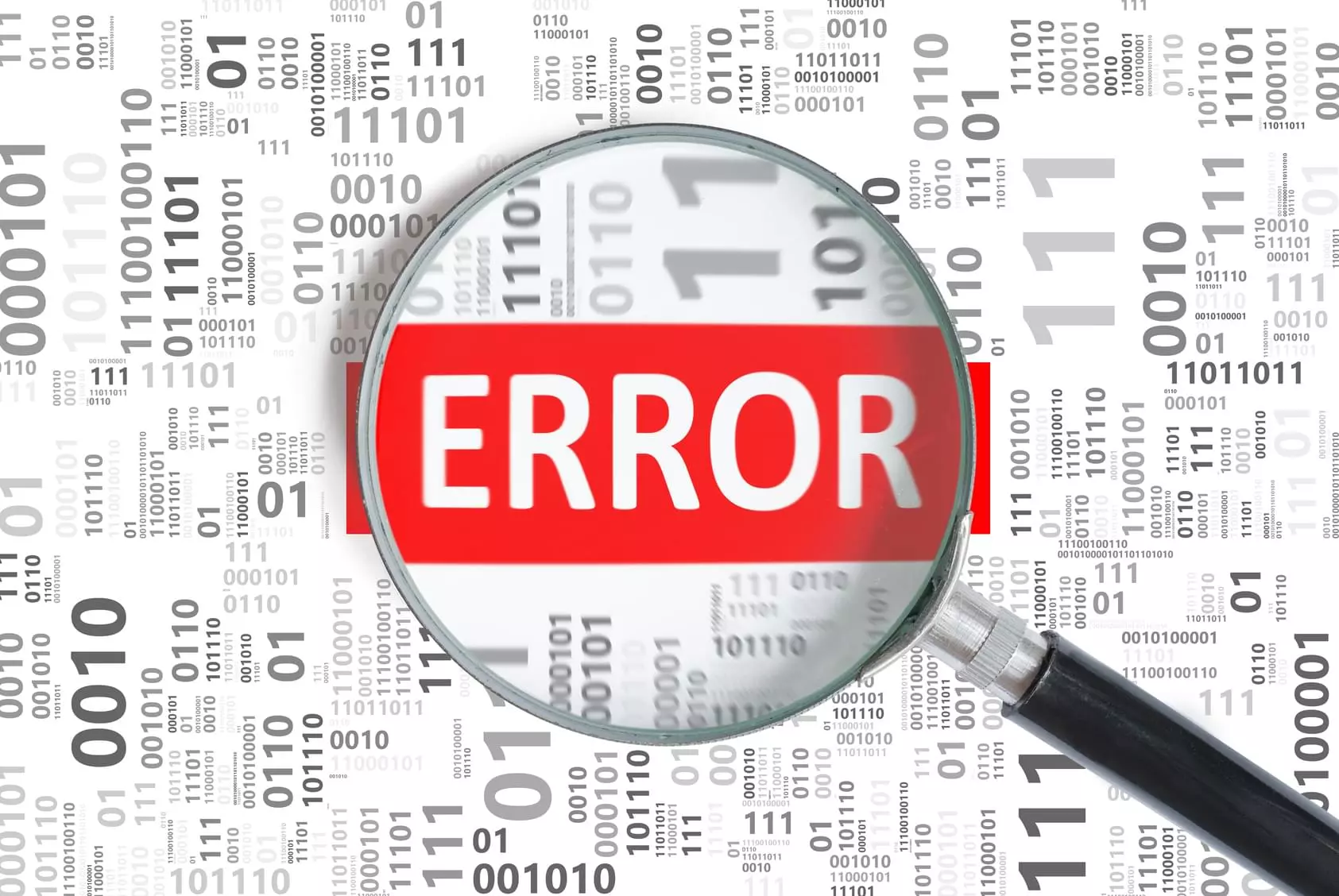
JavaScript is a versatile and widely-used programming language, known for its flexibility and ability to run in web browsers. However, like any programming language, it has its own set of quirks and pitfalls. One common source of frustration for developers are errors, and three of the most prevalent types of errors in JavaScript are Type Errors, Reference Errors, and Undefined Errors. Let's take a closer look at each of these and how to deal with them.
Type Errors
Type errors occur when you try to perform an operation on a value that is of the wrong data type. JavaScript is a dynamically typed language, meaning that variables can change types during runtime, which can lead to type errors if not handled properly. For example:
javascriptCopy codelet number = 42;
let text = "Hello, World!";
let result = number + text; // This will throw a Type Error
In this example, JavaScript doesn't allow you to add a number and a string together directly. To avoid type errors, you should always ensure that you are working with values of compatible types or explicitly convert them if needed.
Reference Errors
Reference errors occur when you try to access a variable or function that doesn't exist. This can happen for a variety of reasons, such as misspelling a variable or trying to use a variable before it's declared. Here's an example:
javascriptCopy codeconsole.log(nonExistentVariable); // This will throw a Reference Error
To avoid reference errors, double-check your variable and function names for typos, and make sure you've declared them before using them.
Undefined Errors
Undefined errors are closely related to reference errors and occur when you try to access a property or method of an object that doesn't exist. JavaScript will return undefined
in such cases, and if you try to perform an operation on an undefined value, it may lead to unexpected behavior or errors. For instance:
javascriptCopy codelet person = { name: "John", age: 30 };
console.log(person.address.city); // This will not throw a Reference Error but will result in an Undefined Error
In this example, the address
property does not exist in the person
object, so trying to access the city
property of address
will result in undefined
. To prevent undefined errors, it's essential to check if the properties and methods you want to use actually exist before trying to access them.
Handling Errors
To write robust JavaScript code, it's crucial to handle these types of errors gracefully. You can use techniques like try...catch
blocks to catch and handle exceptions, or you can use conditional checks to ensure that your code only runs when variables and properties are in the expected states.
javascriptCopy codetry {
// Code that might throw errors
} catch (error) {
// Handle the error
}
// Or, use conditional checks
if (typeof someVariable !== "undefined") {
// Code that uses someVariable
} else {
// Handle the case where someVariable is undefined
}
Understanding and effectively dealing with type, reference, and undefined errors are essential skills for any JavaScript developer. By being proactive in your code and implementing error-handling strategies, you can write more robust and reliable JavaScript applications.
Subscribe to my newsletter
Read articles from Shaki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
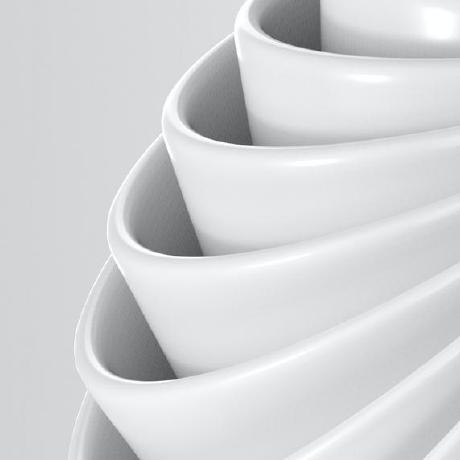
Shaki
Shaki
A Full-stack Developer based in Nairobi Kenya , with a focus on creating robust and user friendly web applications. With strong foundation in both front-end and backend development, I specialize in building responsive and scalable solutions that deliver exceptional user experiences.