JavaScript - An Introduction
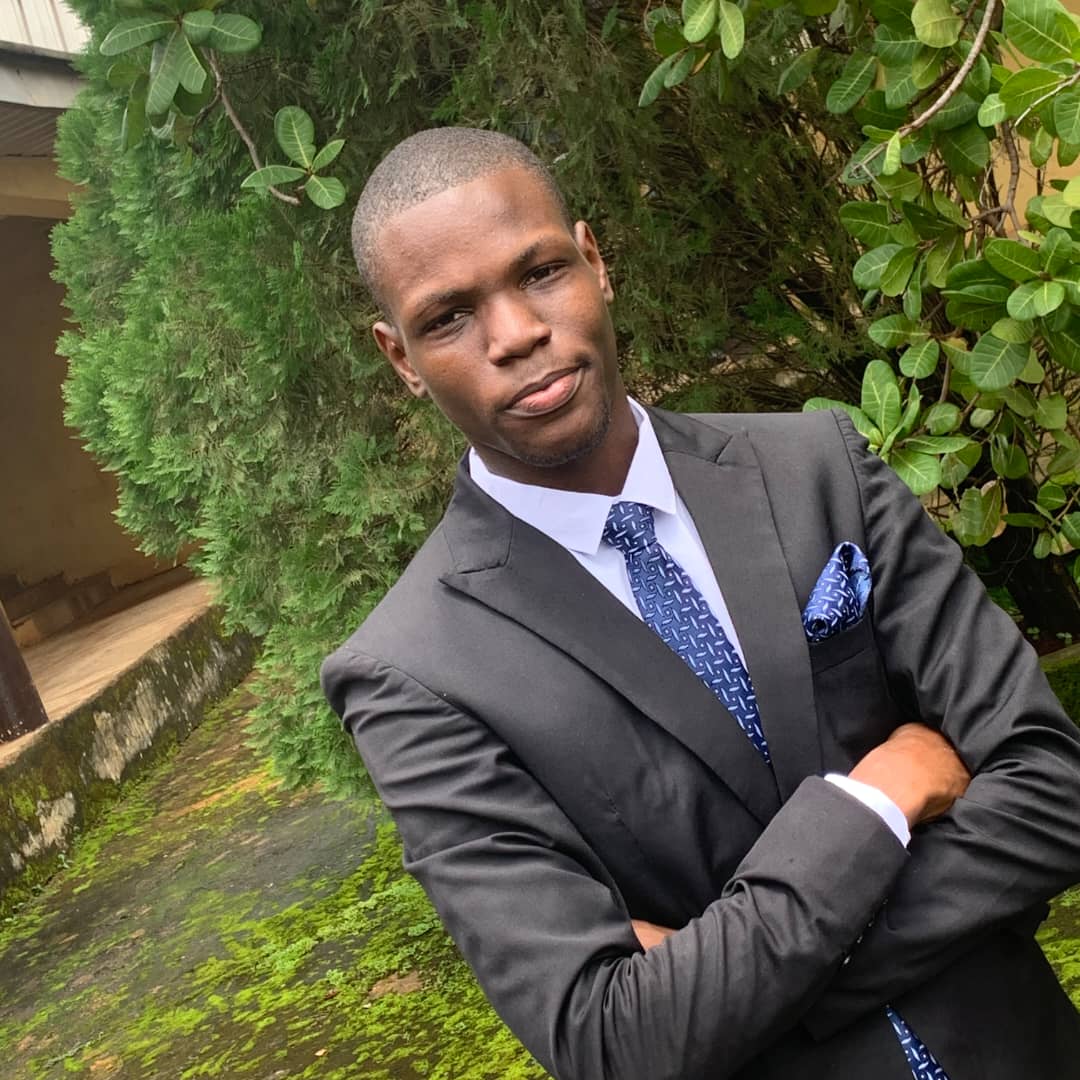
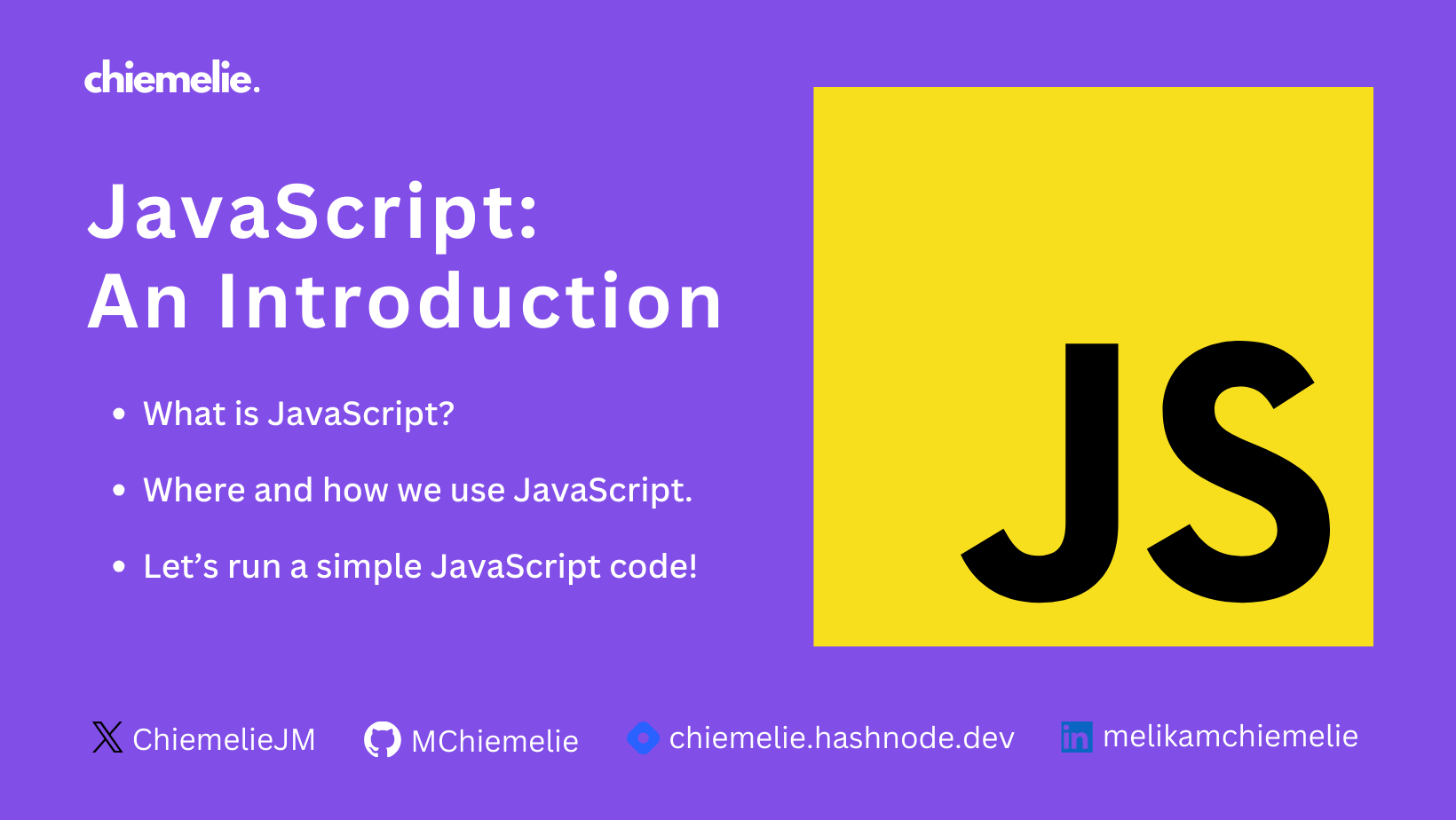
What is JavaScript?
JavaScript is a programming language for creating dynamic and interactive web pages and web-based applications. It plays a critical role as a primary component of modern web applications and is widely supported by all major web browsers, making it a universal language on the web.
JavaScript is a programming language that is one of the core technologies of the World Wide Web, alongside HTML and CSS. As of 2023, 98.7% of websites use JavaScript on the client side for webpage behavior, often incorporating third-party libraries. All major web browsers have a dedicated JavaScript engine to execute the code on users' devices.
HTML provides structure and content, such as text, images, buttons, and links, CSS improves the appearance of these elements and the webpage layout. JavaScript adds functionality to elements like buttons and links, making them interactive and dynamic. It also aids in styling and layout enhancements while enabling elements to perform tasks and calculations.
JavaScript is highly recognized and extends beyond the web, with applications in mobile development, desktop applications, server-side programming, games, blockchain and cryptocurrencies, and even cloud functions and serverless computing. Though there are other platforms as well, these are some of the most common areas where JavaScript is used regularly.
JavaScript is a scripting or programming language that allows you to implement complex features on web pages โ every time a web page does more than just sit there and display static information for you to look at โ displaying timely content updates, interactive maps, animated 2D/3D graphics, scrolling video jukeboxes, etc. โ you can bet that JavaScript is probably involved. It is the third layer of the layer cake of standard web technologies, two of which (HTML and CSS).
Where and how we use JavaScript.
Initially, JavaScript was created to function solely within web browsers. However, it has proven to be much more versatile than originally intended. JavaScript can now be executed in a multitude of environments, such as the browser's console, Node.js runtime, and more. In this article, we will delve into the various applications and platforms where JavaScript can be utilized.
Web Browsers: JavaScript primarily operates within web browsers, empowering developers to elevate the functionality and interactivity of websites. This versatile language facilitates the creation of dynamic web pages, enables the seamless handling of user input, and facilitates communication with web servers.
๐กIn this example, index.html contains a heading, a paragraph, and a button; styles.css refines the layout of the webpage and improves the visual appeal of the text and buttons. However, the real magic happens with the script.js file, each time you click the button, the displayed quote undergoes a delightful transformation.<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="/styles.css"> <title>Quotes ๐ญ</title> </head> <body> <h1>Qoutable Quotes ๐ญ</h1> <p id="quote"></p> <button id="generate">Generate Quote</button> <script src="/script.js"></script> </body> </html>
@import url("https://fonts.googleapis.com/css2?family=IBM+Plex+Sans&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; } body { font-family: "IBM Plex Sans", sans-serif !important; display: flex; flex-direction: column; justify-content: center; align-items: center; height: 100vh; gap: 2%; text-align: center; background: #e7e7eb; } button { padding: 0.5rem; border: none; border-radius: 0.15rem; color: white; background: rgb(115, 63, 193); }
// A list of quotes const quotes = [ "The only way to do great work is to love what you do. - Steve Jobs", "Life is what happens when you're busy making other plans. - John Lennon", "In the middle of every difficulty lies opportunity. - Albert Einstein", "Success is not final, failure is not fatal: It is the courage to continue that counts. - Winston Churchill" ]; // Function to generate a random quote function generateQuote() { const randomIndex = Math.floor(Math.random() * quotes.length); const randomQuote = quotes[randomIndex]; document.getElementById("quote").textContent = randomQuote; } // Event listener for the button click document.getElementById("generate").addEventListener("click", generateQuote); // Initial quote generation generateQuote();
Browser Console: The browser console serves as a useful tool within our web browser, enabling the execution of JavaScript code directly. Its primary functions include testing and debugging scripts, inspecting web page elements, and experimenting with JavaScript commands.
For example, in my browser console, I recently performed a mathematical calculation involving two numbers, denoted as 'x' (with a value of 3) and 'y' (with a value of 5). The calculation was straightforward: 'sum = x + y'.
To demonstrate this, I employed a JavaScript function explicitly designed to add two numbers. Here's the code I used:
//The below JavaScript function adds two numbers function sum (a, b){ console.log(a + b); // Adds a and b and logs the output } sum(3, 5); // pass in the actual values for a and b
Upon running this code in the console, it successfully performed the addition operation and returned the value, which can be seen in the image below:
You can see the output as labelled A on the console. This concise example illustrates how the browser console facilitates JavaScript code execution and how it aids in performing tasks like mathematical calculations, making it an invaluable tool for web development and debugging.
Node.js Runtime: Node.js is a server-side runtime environment that empowers JavaScript to operate on web servers. This capability enables the development of robust and high-performance server applications, APIs, and real-time web applications.
Here's an example of a JavaScript function that calculates a person's grade based on their score:
function calculateGrade(score) { if (score >= 90 && score <= 100) { console.log("Grade A+"); } else if (score >= 80 && score < 90) { console.log("Grade A"); } else if (score >= 70 && score < 80) { console.log("Grade B"); } else if (score >= 60 && score < 70) { console.log("Grade C"); } else if (score >= 50 && score < 60) { console.log("Grade D"); } else if (score >= 40 && score < 50) { console.log("Grade E"); } else if (score >= 0 && score < 40) { console.log("Grade F"); } else if (score < 0) { console.log("Grade cannot be less than 0!"); } else if (score > 100) { console.log("Grade cannot be greater than 100!"); } else { console.log("Invalid!"); } } calculateGrade(74); // Provide the actual score to determine the grade
๐กTo execute the above code in your terminal, ensure that Node is installed.Open your terminal in your preferred Integrated Development Environment (IDE) such as VSCode and execute the following command:
node index.js
In the image below, you can observe the results displayed in the terminal. "A" signifies the command used to run the script using Node, while "B" represents the script's output.
This demonstrates how Node.js extends JavaScript's capabilities beyond web browsers to enable server-side scripting, making it a valuable tool for various applications, including web development and server applications.
Mobile App Development: JavaScript plays a pivotal role in the domain of mobile app development. Through frameworks such as React Native, developers gain the ability to craft cross-platform mobile applications using JavaScript and React. This approach significantly streamlines development processes, cutting down both time and effort.
The above image was extracted from the React Native documentation. It showcases prominent software companies leveraging React Native to construct mobile applications.
Desktop Applications: JavaScript, in conjunction with HTML and CSS, serves as a versatile tool for crafting cross-platform desktop applications through the utilization of frameworks such as Electron. This methodology empowers developers to seamlessly generate native desktop applications compatible with diverse operating systems.
The above image below was extracted directly from the official Electron documentation. It shows very popular software that uses Electron for building their desktop applications.
Internet of Things (IoT): JavaScript finds practical applications in robotics, empowering us to program IoT devices and craft applications that seamlessly interface with sensors and actuators. Platforms such as Johnny-Five play a pivotal role in facilitating JavaScript-based robotics and IoT development.
Game Development: Game developers harness JavaScript's capabilities through libraries and engines like Babylon.js, Three.js and Phaser. These tools facilitate the creation of both 2D and 3D games that can run in web browsers.
Blockchain and Cryptocurrency: JavaScript plays a role in blockchain application development and smart contract creation. Ethereum, for example, employs Solidity, a JavaScript-like language, for writing smart contracts.
Augmented Reality (AR) and Virtual Reality (VR): JavaScript can be used in the development of AR and VR experiences. Frameworks like A-Frame and AR.js empower developers to build web-based AR and VR applications.
Cloud Functions and Serverless Computing: JavaScript is compatible with serverless computing platforms such as AWS Lambda, Google Cloud Functions, and Azure Functions. It enables the creation of serverless functions and microservices, facilitating scalable and event-driven applications.
JavaScript's adaptability and extensive ecosystem make it a versatile language suitable for a multitude of applications and platforms. Its ubiquity in the world of programming ensures its continued relevance and utility in various domains beyond web development.
Let's run a simple JavaScript code!
Follow the below set of instructions,
Open your web browser (e.g., Google Chrome, Mozilla Firefox, or Safari).
Navigate to any website or simply open a new tab.
Right-click anywhere on the web page or press
Ctrl + Shift + J
orCmd + Option + J
on macOS to open the browser's developer tools. Click onInspect
.This will open the browser console, then click on
Console
.In the console, paste the below JavaScript code:
// JavaScript says Hi๐๐ฝ
function greet (name) {
console.log(`Hi ๐๐ฝ, ${name}.`);
}
greet("Chiemelie"); // You should replace Chiemelie with your name.
After pasting the code, you can press the
Enter
to execute it.You should see the output in the console, which will be: "Hi ๐๐ฝ, Chiemelie.".
Chiemelie
with your name to see the greeting with your name in the console.Conclusion
JavaScript is a dynamic and very powerful language that can be used to build a wide range of applications for different platforms. It has a very large ecosystem of libraries and frameworks and a robust community of millions of developers who build and maintain applications written in JavaScript. Proficiency in JavaScript is a lucrative skill and valuable asset as JavaScript developers are in high demand in the Tech industry.
If you found this blog post helpful, please consider sharing it with others who might benefit. You can also follow me for more content on Javascript, React.js, Next.js and other web development topics.
To sponsor my work, please visit: Chiemelie's Sponsor Page and explore the various sponsorship options.
Connect with me on X (Twitter), LinkedIn, and GitHub.
Thank you for reading and see you at the next one :)
Subscribe to my newsletter
Read articles from Chiemelie Melikam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
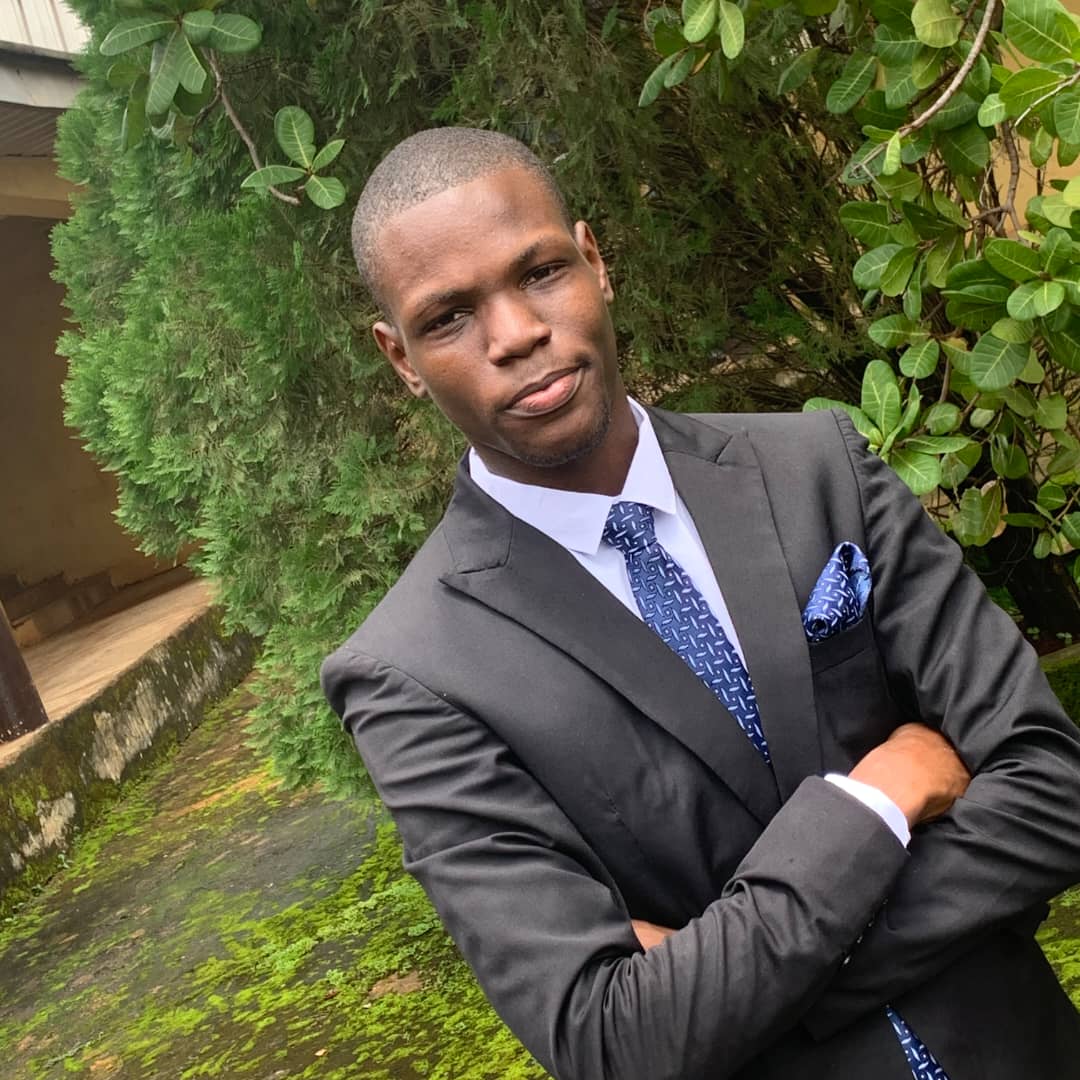
Chiemelie Melikam
Chiemelie Melikam
A proficient front-end developer and prospective software engineer. I am into building experiences & applications on the web. I love writing and talking about web development, technical writing, open source. I think JavaScript is awesome and love writing about it and building fanastic applications with it.