Ant, Maven, and Gradle in DevOps: A Powerful Trio
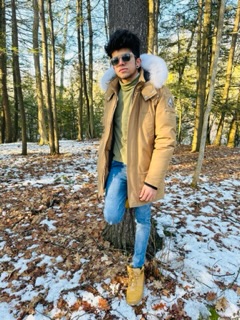
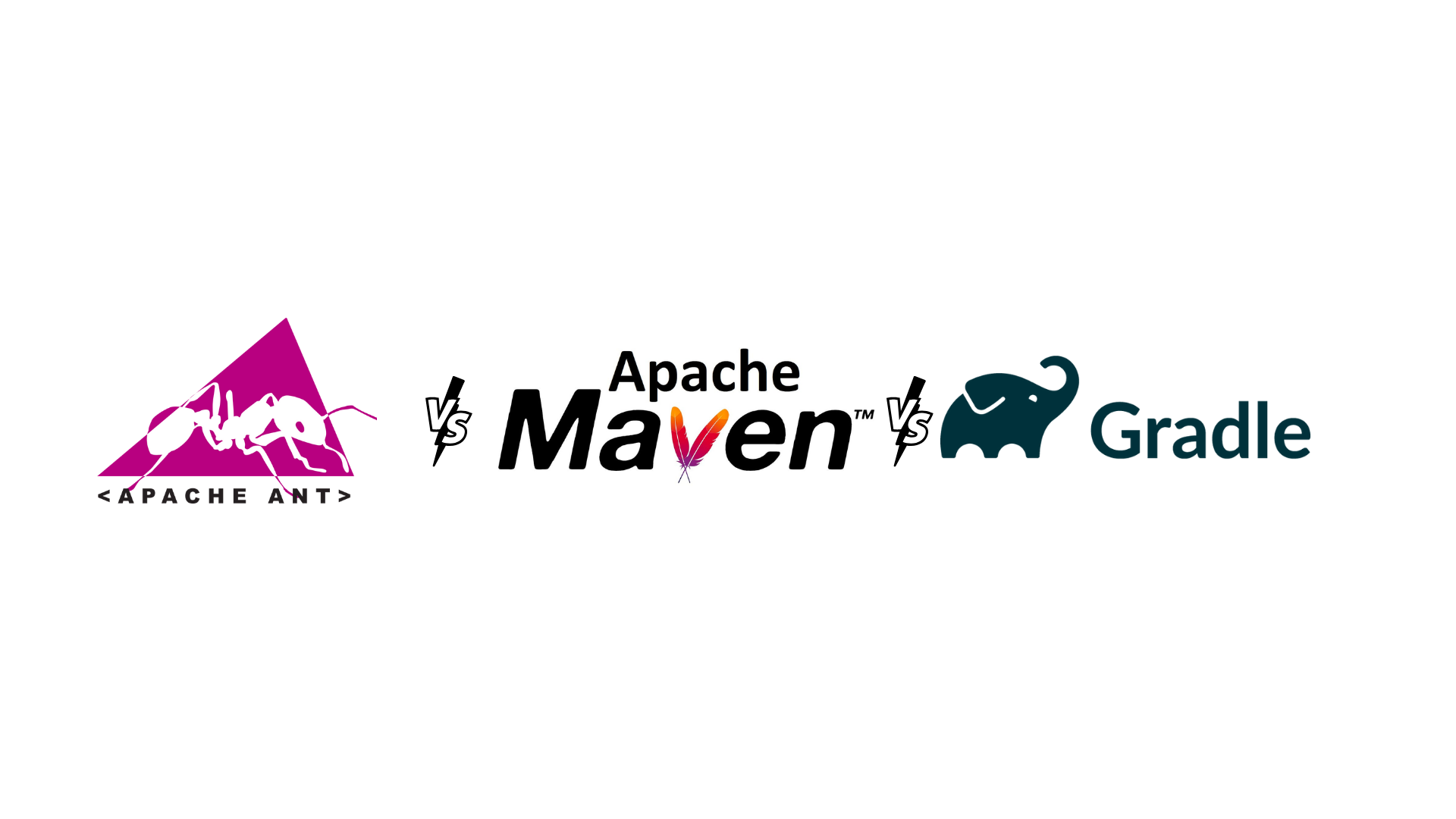
In the ever-evolving world of DevOps, efficient build and deployment processes are essential for delivering high-quality software rapidly. Three of the most popular build automation tools in the DevOps landscape are Apache Ant, Apache Maven, and Gradle. In this blog post, we will explore these tools from a DevOps perspective and showcase some code examples to help you understand their power and versatility.
Apache Ant:
Apache Ant is one of the oldest build automation tools that has stood the test of time. It uses XML-based configuration files known as build.xml to define build tasks and dependencies. Ant is known for its flexibility and is widely used for building Java applications, but it can be used for any project requiring automation.
DevOps Perspective:
Ant's flexibility makes it a valuable tool for customizing build and deployment pipelines to meet specific project requirements.
It integrates well with version control systems and continuous integration (CI) tools.
Code Example: Here's a simple Ant build.xml file to compile and package a Java application:
<project name="MyApp" default="build">
<target name="build">
<javac srcdir="src" destdir="build"/>
<jar destfile="dist/MyApp.jar" basedir="build"/>
</target>
</project>
Apache Maven:
Apache Maven is known for its "convention over configuration" approach. It uses a project object model (POM) file, typically named pom.xml
, to manage project dependencies and plugins. Maven follows a standardized directory structure and provides a wide range of built-in plugins.
DevOps Perspective:
Maven simplifies dependency management and ensures consistency across projects by enforcing conventions.
It integrates seamlessly with popular CI/CD systems like Jenkins and GitLab CI/CD.
Code Example: A basic pom.xml
file for a Java project:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<!-- Define your project dependencies here -->
</dependencies>
</project>
Gradle: The Modern Build Automation
Gradle combines the best of both Ant and Maven, offering flexibility and convention. It uses a Groovy or Kotlin DSL to define build scripts, allowing for concise and readable code. Gradle's dependency management is robust, and it's highly extensible.
DevOps Perspective:
Gradle's build.gradle files are highly maintainable and easy to read, making them an excellent choice for complex projects.
It is compatible with CI/CD platforms like Travis CI and CircleCI.
Code Example: A basic build.gradle file for a Java project:
plugins {
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'com.example:my-library:1.0'
}
task buildJar(type: Jar) {
from sourceSets.main.output
archiveFileName = "my-app.jar"
destinationDirectory = file("build/libs")
}
In conclusion, the choice between Ant, Maven, and Gradle depends on your project's requirements and your team's preferences. Apache Ant offers flexibility, Maven enforces conventions, and Gradle combines both approaches. Regardless of your choice, mastering these build automation tools is a crucial step toward achieving DevOps excellence, streamlining your development workflow, and delivering high-quality software efficiently.
Subscribe to my newsletter
Read articles from Edvin Dsouza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
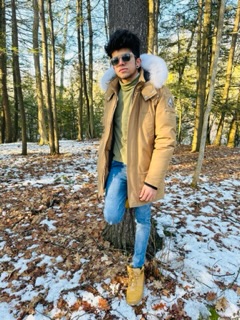
Edvin Dsouza
Edvin Dsouza
๐ฉโ๐ป DevOps engineer ๐ | Automation enthusiast โ๏ธ | Infrastructure as code | CI/CD ๐ Let's build awesome things together!