HashMaps Deconstructed: Understanding the Magic Inside
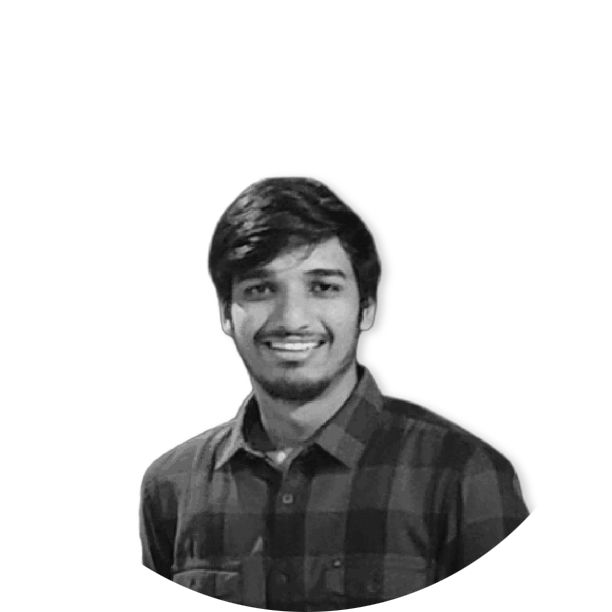
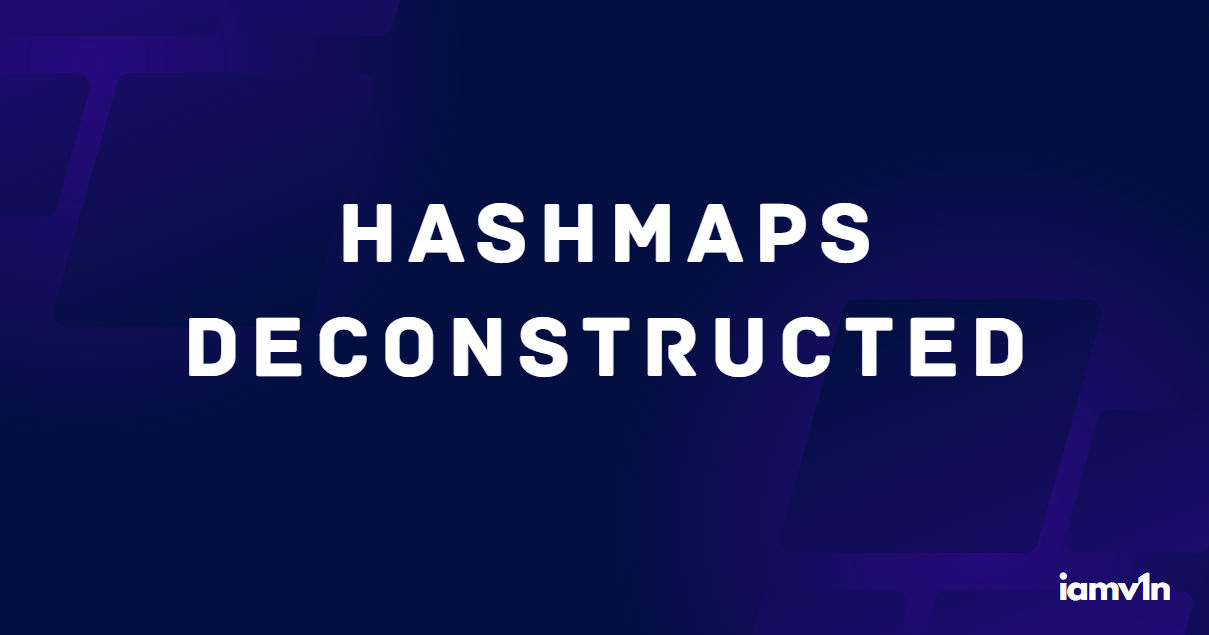
HashMaps, a staple of computer science and software engineering, are versatile data structures that facilitate efficient key-value storage and retrieval. In this comprehensive guide, we will explore the intricacies of HashMaps, covering essential topics ranging from basic concepts to low-level design. I'll provide detailed explanations and C++ code examples to deepen your understanding.
Understanding HashMap
What is a HashMap?
A HashMap is a fundamental data structure that allows you to store and retrieve key-value pairs efficiently. At its core, it offers fast access to values based on their associated keys, often achieving constant time complexity for lookups on average. This makes HashMaps invaluable for tasks like indexing, caching, and managing associations between data items.
Key Features
HashMaps boast several key features:
Fast Access: HashMaps provide swift access to values based on their keys, a fundamental requirement in many applications.
Hashing: The primary mechanism behind HashMaps is hashing, where keys are transformed into indices within the underlying array, ensuring rapid lookups.
Dynamic Sizing: HashMaps can dynamically resize to accommodate varying numbers of key-value pairs, adapting to workloads.
Collision Handling: When multiple keys hash to the same index, HashMaps employ collision resolution techniques to manage them effectively.
Internal Working and Low-Level Design
Hashing Function
The core of a HashMap's functionality lies in its hashing function. This function takes a key, computes a hash code (typically an integer), and maps it to an index within the underlying array. An effective hashing function ensures an even distribution of keys across the array, minimizing collisions.
Hash Code Calculation in C++
size_t hash(const KeyType& key) {
// Custom hash code calculation based on key properties
// Ensure the result is within the array bounds
return hash_code % array_size;
}
Buckets and Linked Lists
HashMaps are typically implemented as an array of buckets, where each bucket can hold multiple key-value pairs. When two keys hash to the same index (a collision occurs), they are stored in a linked list within the bucket. This linked list serves as a chain to store multiple entries at the same index.
Bucket Structure in C++
struct Node {
KeyType key;
ValueType value;
Node* next; // Reference to the next node in the linked list
};
Load Factor and Resizing
To maintain efficient operations, HashMaps dynamically resize when the number of key-value pairs crosses a certain threshold, known as the load factor. Resizing involves creating a new, larger array and rehashing all existing key-value pairs into it.
Resizing Algorithm in C++
if (size >= loadFactor * capacity) {
// Double the capacity and rehash all key-value pairs
resize(2 * capacity);
}
Collision Resolution
One of the key challenges in designing a HashMap is handling collisions gracefully. Collisions occur when two or more keys hash to the same index. HashMaps use collision resolution techniques to manage these situations effectively. One common approach is separate chaining, where linked lists within buckets store colliding entries.
Collision Resolution in C++
void put(const KeyType& key, const ValueType& value) {
size_t index = hash(key);
// Check if there's already an entry at this index
if (buckets[index] == nullptr) {
buckets[index] = new Node(key, value);
} else {
// Handle collision by appending to the linked list
Node* current = buckets[index];
while (current->next != nullptr) {
current = current->next;
}
current->next = new Node(key, value);
}
}
Code Examples
Creating a HashMap in C++
Let's create a basic HashMap in C++ to understand its usage.
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> hashMap;
hashMap["Alice"] = 25;
hashMap["Bob"] = 30;
std::cout << "Alice's age: " << hashMap["Alice"] << std::endl;
return 0;
}
Custom HashMap Implementation in C++
Now, let's build a custom HashMap implementation in C++ to delve deeper into its mechanics.
#include <iostream>
#include <vector>
template <typename KeyType, typename ValueType>
class CustomHashMap {
private:
struct Node {
KeyType key;
ValueType value;
Node* next;
Node(const KeyType& k, const ValueType& v) : key(k), value(v), next(nullptr) {}
};
std::vector<Node*> buckets;
size_t size;
size_t capacity;
const double loadFactor;
void resize(size_t newCapacity) {
// Implement the resizing logic here
}
public:
CustomHashMap() : capacity(16), size(0), loadFactor(0.75) {
buckets.resize(capacity, nullptr);
}
// Implement other methods like put, get, and more
};
int main() {
CustomHashMap<int, std::string> customHashMap;
customHashMap.put(1, "Alice");
customHashMap.put(2, "Bob");
std::cout << customHashMap.get(2) << std::endl; // Outputs "Bob"
return 0;
}
Conclusion
HashMaps are indispensable data structures with a myriad of applications. By comprehending their inner workings, including hashing functions, bucket structures, collision resolution, and resizing algorithms, you can harness the full potential of HashMaps in your software development projects. Whether you are using a standard library implementation or constructing your custom HashMap, these concepts are pivotal for optimizing performance and efficiency.
In particular, addressing collisions and efficiently handling them is a crucial aspect of HashMap design. Techniques like separate chaining with linked lists ensure that HashMaps can accommodate a large number of key-value pairs while maintaining efficient access times. Understanding these intricacies will empower you to design and use HashMaps effectively in your algorithms and data structures.
Subscribe to my newsletter
Read articles from Vineet Anil Likhitkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
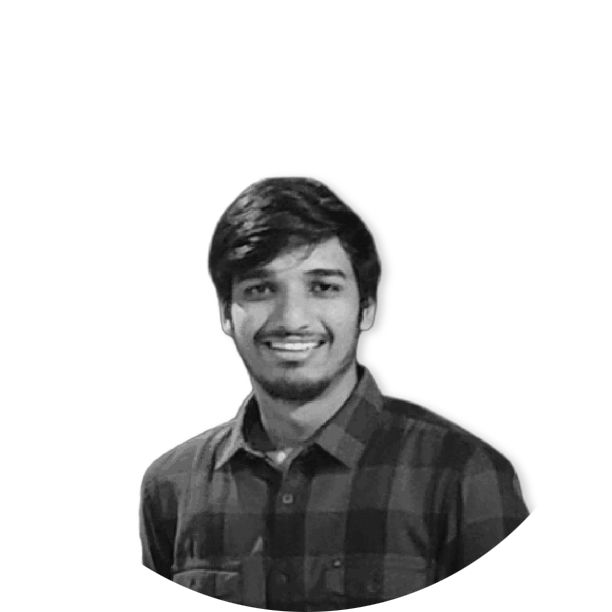