Mastering Promises, Async/Await, and Try/Catch in ES6 JavaScript ๐
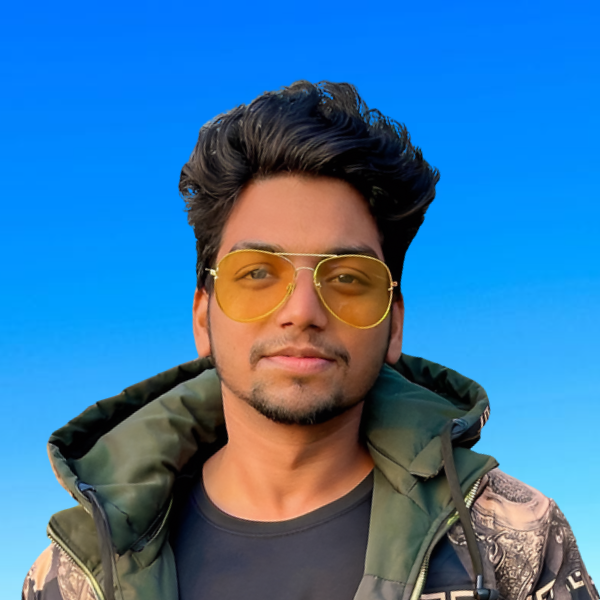
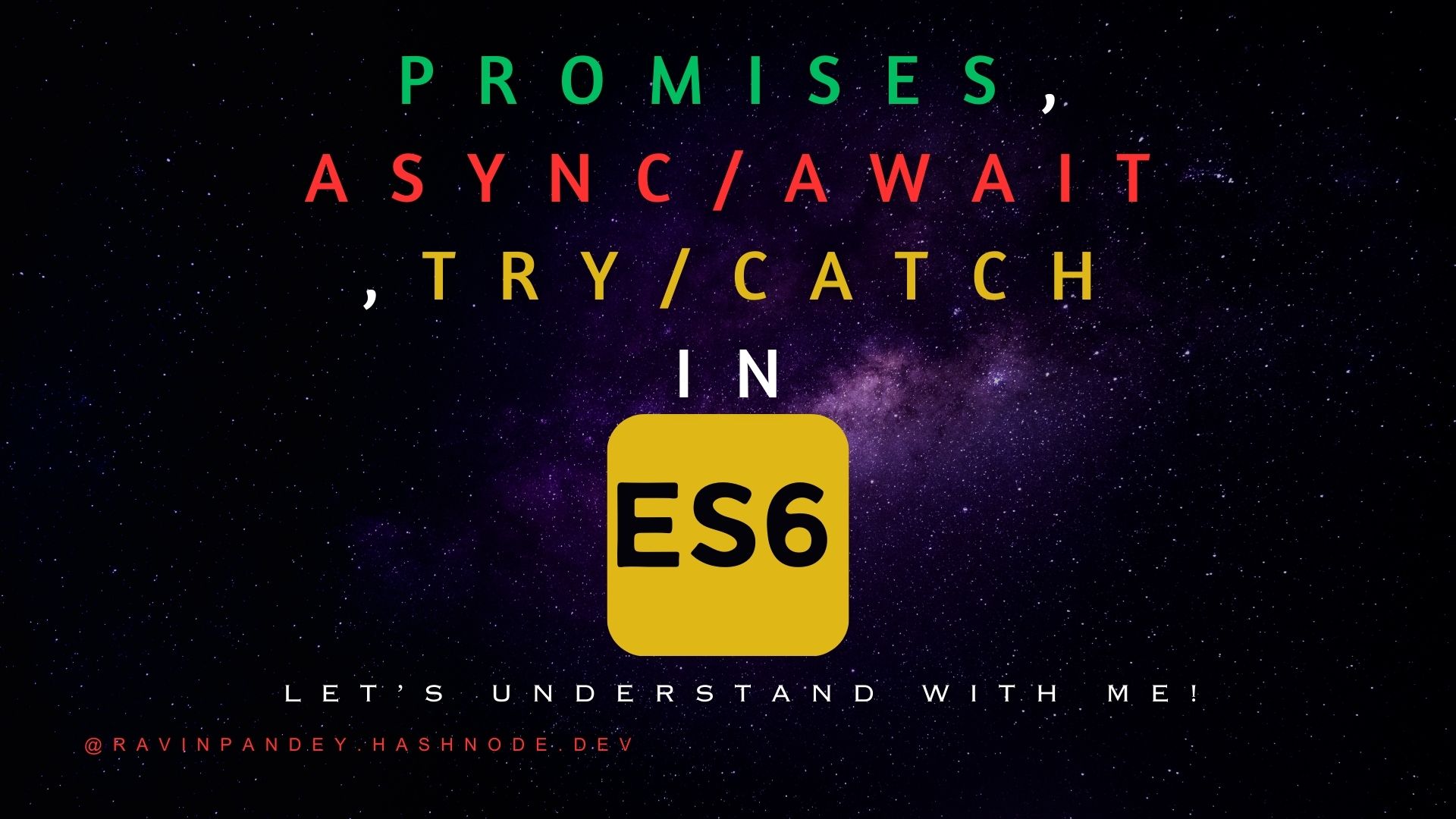
Hello, JavaScript enthusiast! ๐ In the ever-evolving world of JavaScript, ES6 (ECMAScript 2015) introduced some powerful features that have forever changed the way we handle asynchronous code. Today, we're going to dive deep into three of these features: Promises, Async/Await, and Try/Catch. These tools allow you to write cleaner and more maintainable asynchronous code. By the end of this blog, you'll be equipped to conquer complex asynchronous tasks with ease. Let's get started! ๐
Promises: A Better Way to Deal with Asynchronous Operations ๐
Promises are a way to manage asynchronous operations in a more structured and readable manner. They provide a clear syntax for handling success and error cases.
Basic Promise Syntax ๐ฉ
const fetchData = () => {
return new Promise((resolve, reject) => {
// Simulating an asynchronous operation
setTimeout(() => {
const data = { message: 'Hello, Promises!' };
resolve(data); // Success
// reject(new Error('Something went wrong!')); // Error
}, 1000);
});
};
fetchData()
.then((result) => {
console.log(result.message); // Outputs: "Hello, Promises!"
})
.catch((error) => {
console.error(error.message); // Outputs: "Something went wrong!"
});
In this example, fetchData
returns a Promise that resolves with data after a simulated asynchronous operation.
Async/Await: Making Asynchronous Code Readable ๐
Async/Await is a syntax sugar built on top of Promises. It allows you to write asynchronous code in a more synchronous, readable style.
Async Function and Await Keyword ๐๏ธโโ๏ธ
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = { message: 'Hello, Async/Await!' };
resolve(data);
}, 1000);
});
};
const fetchAndDisplayData = async () => {
try {
const result = await fetchData();
console.log(result.message); // Outputs: "Hello, Async/Await!"
} catch (error) {
console.error(error.message);
}
};
fetchAndDisplayData();
In this example, fetchAndDisplayData
is an async function that uses the await
keyword to wait for the fetchData
Promise to resolve.
Try/Catch: Handling Errors Gracefully ๐งก
Try/Catch is a fundamental JavaScript construct for handling errors. When combined with Promises and Async/Await, it provides a robust way to manage exceptions.
Error Handling with Try/Catch ๐ช
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
const randomNumber = Math.random();
if (randomNumber < 0.5) {
resolve(randomNumber);
} else {
reject(new Error('Random number is too high!'));
}
}, 1000);
});
};
const fetchAndHandleData = async () => {
try {
const result = await fetchData();
console.log(`Random number: ${result}`);
} catch (error) {
console.error(error.message); // Outputs: "Random number is too high!"
}
};
fetchAndHandleData();
In this example, fetchData
generates a random number and rejects the Promise if it's too high. The error is caught and handled gracefully with Try/Catch.
Use Cases and Examples ๐
Let's explore some practical use cases for Promises, Async/Await, and Try/Catch:
1. Fetching Data from an API ๐
const fetchUserData = async (userId) => {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
if (!response.ok) {
throw new Error('Failed to fetch user data');
}
const user = await response.json();
return user;
} catch (error) {
console.error(error.message);
}
};
fetchUserData(123)
.then((user) => {
console.log(`User: ${user.name}`);
})
.catch((error) => {
console.error(error.message);
});
Promises and Async/Await are perfect for making API requests and handling responses gracefully.
2. Loading Resources in a Game ๐ฎ
const loadResource = async (resourceName) => {
try {
const resource = await loadResourceFromFile(resourceName);
return resource;
} catch (error) {
console.error(`Failed to load ${resourceName}: ${error.message}`);
}
};
const gameResources = ['playerModel', 'terrainMap', 'soundtrack'];
gameResources.forEach(async (resource) => {
const loadedResource = await loadResource(resource);
console.log(`Resource loaded: ${loadedResource}`);
});
Async/Await can simplify loading resources in a game or application.
Wrapping Up
Promises, Async/Await, and Try/Catch are essential tools for handling asynchronous code in a more readable and robust way. Whether you're making API requests, loading resources, or dealing with any other asynchronous operation, these features will help you write cleaner and more maintainable code.
Feel free to share your experiences and ask questions in the comments below. Happy coding๐ช
Subscribe to my newsletter
Read articles from Ravinder Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
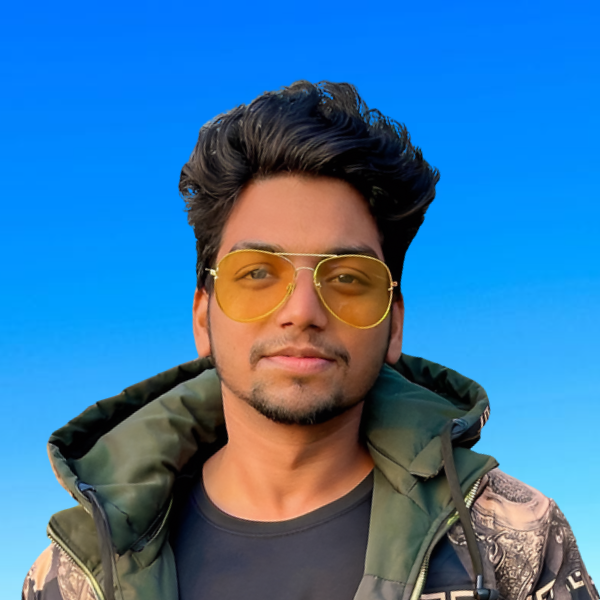
Ravinder Pandey
Ravinder Pandey
Self taught Web Developer ๐ป.