Proxy Design Pattern
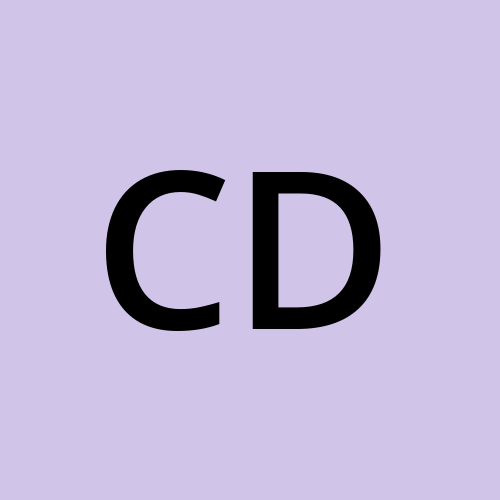
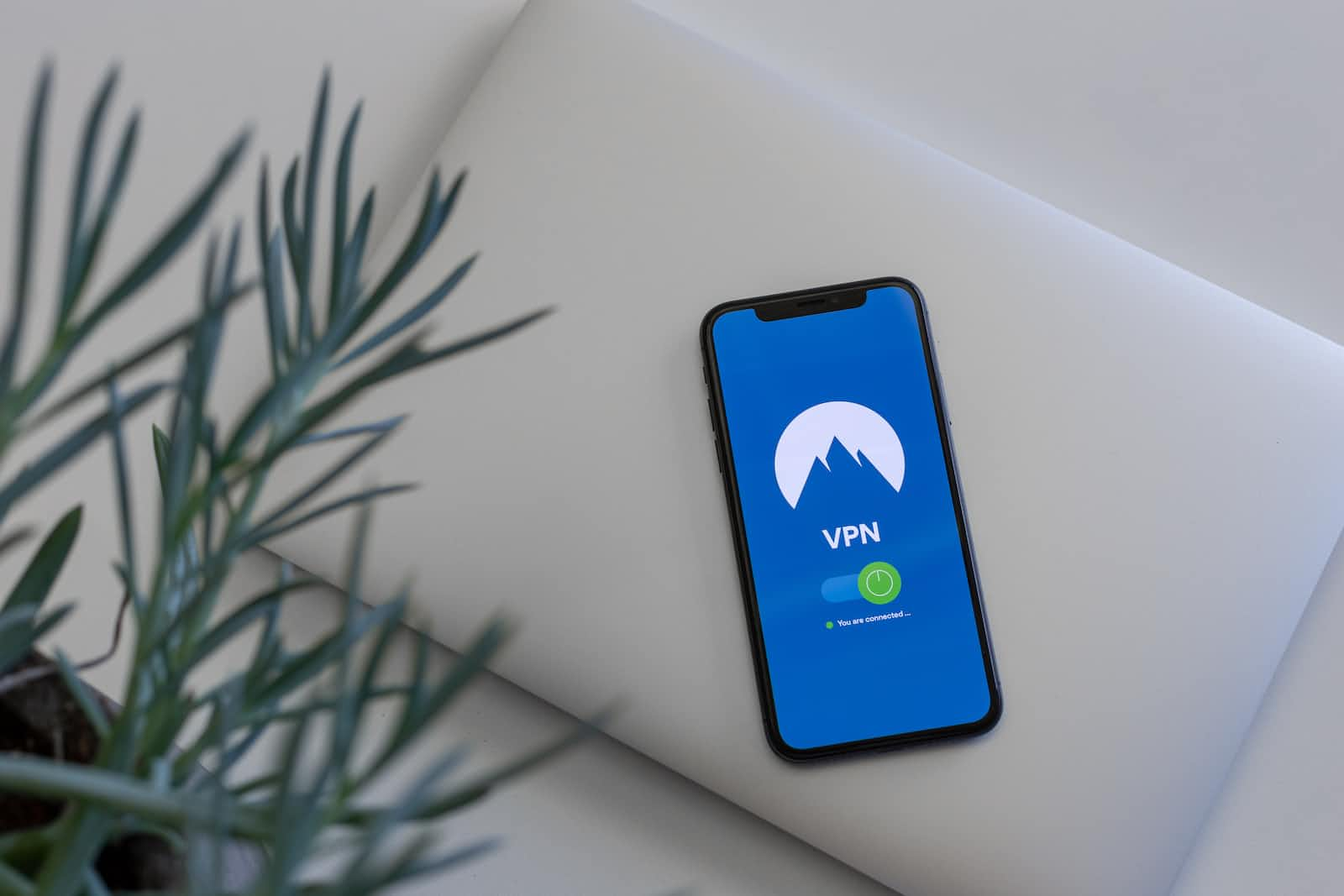
In this pattern, a middle object, generally referred to as a proxy object, will be present between every transaction involving two objects
Examples:
Access Restriction (Internet): If you are connected to the Wi-Fi network in your company, you will not be able to access blocked websites. This restriction is enforced through a proxy design pattern. Between the user and the internet, there exists a company proxy server that maintains a list of blocked websites. When you attempt to visit a website, the request is routed to the proxy server, which checks whether the requested website is on the list. If the website is not found on the list, the request is then forwarded to the main server.
Caching: The cache itself functions as a proxy object. When we request something from the database, the initial request is directed to the cached data. If the requested data is found in the cache, it will be returned directly without requiring a database call.
Preprocessing and post-processing: proxy is also used in pre-processing and post-processing, such as logging and publishing events or messages, or for tracking purposes
Spring Boot bean creation: Every bean has a proxy object.
Note: There can be any number of proxy objects between the client and the real object.
Sample working example:
Assume that there is an EmployeeDao
class is responsible for creating or deleting an employee from the table. For these operations, specific roles are required. Therefore, we introduce a proxy object that handles role validation. If the validation is successful, it will then call the actual Dao
class code. This approach allows us to separate all validations from the core logic.
Proxy Object:
package Dao;
import dto.EmployeeDo;
public class EmployeeDaoProxy implements EmployeeDao {
EmployeeDao employeeDaoObj;
public EmployeeDaoProxy() {
employeeDaoObj = new EmployeeDaoImpl();
}
@Override
public void create(String client, EmployeeDo obj) throws Exception {
if (client.equals("ADMIN")){
employeeDaoObj.create(client, obj);
return;
}
throw new Exception("Access Denied");
}
@Override
public void delete(String client, int employeeId) throws Exception {
if (client.equals("ADMIN")){
employeeDaoObj.delete(client,employeeId);
return;
}
throw new Exception("Access Denied");
}
@Override
public EmployeeDo get(String client, int employeeId) throws Exception {
if (client.equals("ADMIN") || client.equals("USER")) {
return employeeDaoObj.get(client,employeeId);
}
throw new Exception("Access Denied");
}
}
usage
import Dao.EmployeeDao;
import Dao.EmployeeDaoProxy;
import dto.EmployeeDo;
public class ProxyPattern {
public static void main(String[] args) {
try{
EmployeeDao employeeDao = new EmployeeDaoProxy();
employeeDao.create("USER", new EmployeeDo());
System.out.println("Operation successful");
}catch (Exception e){
System.out.println(e.getMessage());
}
}
}
Source code - link
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
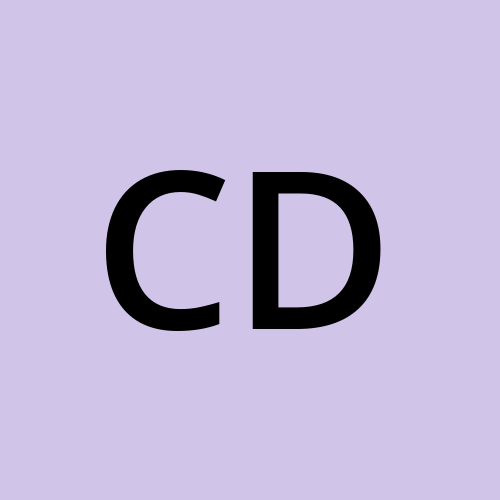
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.