Comparison of Two Objects Using equals() and hashCode() Methods in Java
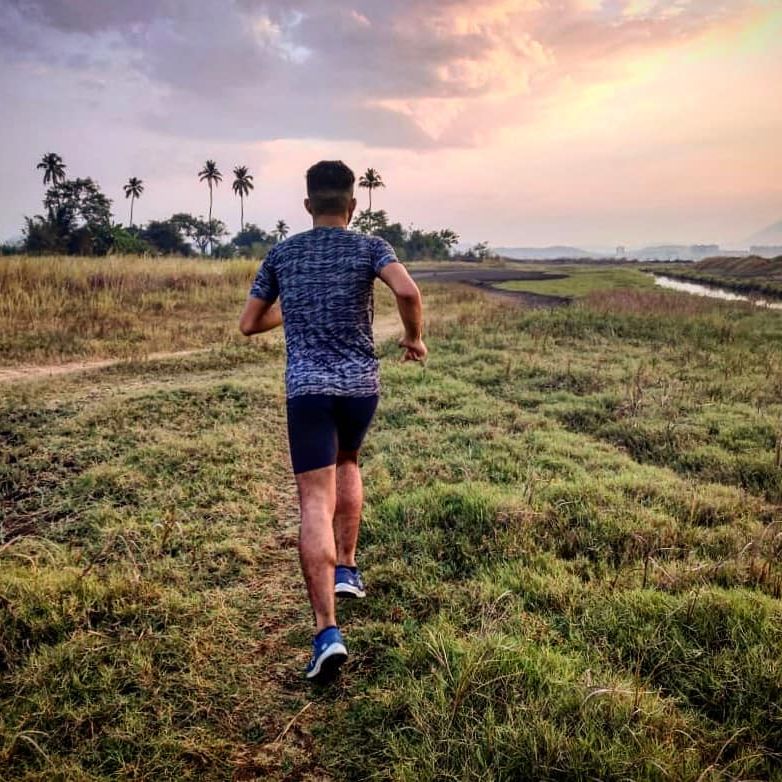
Java provides two important methods for comparing objects: equals()
and hashCode()
. These methods are fundamental when it comes to working with objects in Java. In this blog post, we'll explore the differences between these two methods and how they are used for comparing objects.
The equals()
Method
The equals()
method is used to compare the content of two objects to check if they are equal. It is defined in the java.lang.Object
class, which means that all Java classes inherit this method. However, it's common practice to override equals()
in custom classes to provide a meaningful comparison based on the object's attributes.
Overriding the equals()
Method
To properly compare two objects, you should override the equals()
method in your custom class. Here's an example of how you might override equals()
for a Person
class:
public class Person {
private String name;
private int age;
// Constructor, getters, setters...
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return age == person.age && Objects.equals(name, person.name);
}
}
In this example, we first check if the objects are the same (this == obj
). If they are, they are equal. Next, we check if obj
is null
or if it belongs to a different class. If either of these conditions is true, the objects are not equal.
Finally, if none of the above conditions apply, we cast obj
to a Person
object and compare the attributes (name
and age
) to determine equality.
Using the equals()
Method
Once the equals()
method is properly overridden, you can use it to compare objects. For example:
Person person1 = new Person("John Doe", 30);
Person person2 = new Person("John Doe", 30);
if (person1.equals(person2)) {
System.out.println("The persons are equal.");
} else {
System.out.println("The persons are not equal.");
}
This will output: "The persons are equal."
The hashCode()
Method
The hashCode()
method is used to get a unique integer value for an object. It's also defined in the java.lang.Object
class and should be overridden in custom classes when equals()
is overridden. This is important for collections like HashMap
and HashSet
which use hash codes to efficiently store and retrieve objects.
Overriding the hashCode()
Method
When you override the equals()
method, you should also override hashCode()
to ensure consistency. Here's an example:
@Override
public int hashCode() {
return Objects.hash(name, age);
}
In this example, we use the Objects.hash()
method to generate a hash code based on the name
and age
attributes. This ensures that objects with the same attributes will produce the same hash code.
Using the hashCode()
Method
Once hashCode()
is properly overridden, you can use it in conjunction with collections that rely on hash codes for efficient operations:
Set<Person> personSet = new HashSet<>();
Person person1 = new Person("John Doe", 30);
Person person2 = new Person("John Doe", 30);
personSet.add(person1);
personSet.add(person2);
System.out.println("Size of the set: " + personSet.size()); // Output: Size of the set: 1
In this example, even though person1
and person2
are equal according to the equals()
method, only one of them is added to the HashSet
due to the consistent hash codes generated by the overridden hashCode()
method.
Conclusion
In Java, the equals()
method is used to compare the content of two objects, while the hashCode()
method provides a unique identifier for an object. It's important to override both methods when you override equals()
to maintain consistency. This is especially crucial when working with collections that rely on hash codes for efficient operations.
By understanding how these methods work together, you can effectively compare and manage objects in your Java applications.
Subscribe to my newsletter
Read articles from Ronil Rodrigues directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
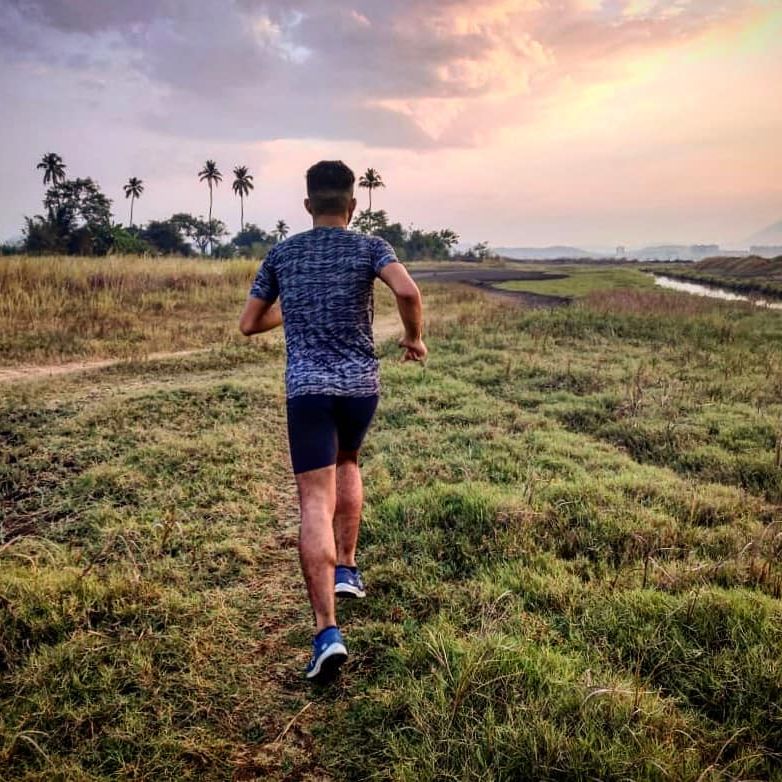
Ronil Rodrigues
Ronil Rodrigues
Cloud enthusiast who runs towards cloud!!