Building map based apps and websites.
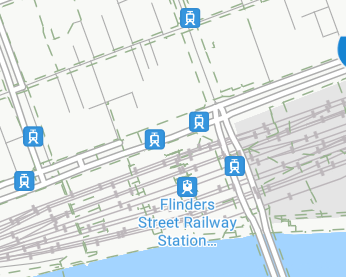
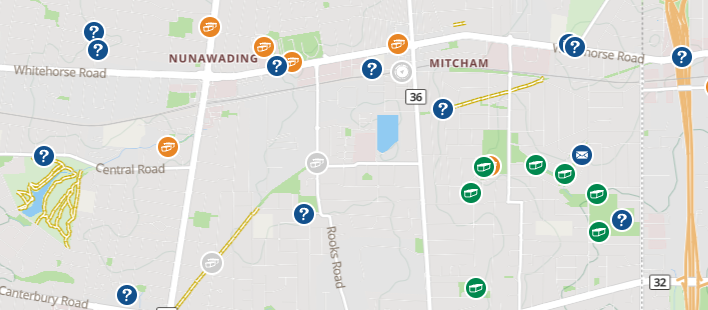
I've always had an affinity for maps, mapping and associated activities (Orienteering, Rogaining, Geocaching). There is just something about distilling so much real-world information down to a few lines and squiggles on a page.
At work this meant that customer queries, demos and tender responses for Azure and Bing maps tended to come to me. I found over the years that there was significant confusion over which ecosystem to use, whether to buy vs. build, and the relationship (or not) between the map visuals in the browser and the backend infrastructure and data that powers it.
The goal of this series of posts is to talk through these choices and architectures, build some apps and see where the road leads :-)
Why is this needed?
The major mapping platforms all have fantastic demos and documentation detailing how to display the maps and draw icons, lines and layers on them.
For example: Bing Maps Samples (bingmapsportal.com) has hundreds of working code snippets to completely control look of the the front end. The other platforms have similar samples available.
However, in nearly all these examples, the data that is being displayed on the map is static and hardcoded into the demo or pulled from a simple CSV file. Full end-to-end examples where geospatial data is captured, stored, processed and then displayed are few.
Build vs Buy
I have had many customer conversations that went along the lines of :
The answer to nearly every question is "yes" - because it is a development platform that you can code up to do nearly anything. The dark side of that answer is that you have to code up everything. Out of the box, all you get is an empty map on a screen. So the discussion becomes more about:
Do you have people with the right coding skills?
Do you have the budget?
How long do you have?
While building a data driven mapping solution is not particularly complex, every additional feature adds time and cost and needs to be maintained. I've been in some discussions where the customer had a fantastic vision, but not the means to execute.
The alternative is to buy an off-the-shelf GIS (Geographic Information System) such as ArcGIS or similar and learn how to use it. These types of products have rich functionality and are often extensible - but you need to evaluate whether they have the features you need and integrate with your existing systems or data.
A balanced sweet spot seems to be in map generation vs. consumption. If you have professional cartographers, mining engineers and so on that spend all day working with this data, then they probably need professional tools. But if the data they generate is displayed and consumed by hundreds, thousands or millions of others then this is where Bing/Google/Azure maps come into their own with high scale and low cost.
Which platform should I choose?
OK, you have decided to build. Should you choose Google Maps, Bing Maps, Azure Maps or other?
My 2c - It really doesn't matter. All three work pretty much the same way and have the same basic features and development approach.
The HTML & Javascript below is for Azure Maps along with the resulting web page.
<html lang="en">
<head>
<!-- Add references to the Atlas Map control JavaScript and CSS files. -->
<link href="https://atlas.microsoft.com/sdk/javascript/mapcontrol/3/atlas.min.css" rel="stylesheet" />
<script src="https://atlas.microsoft.com/sdk/javascript/mapcontrol/3/atlas.min.js"></script>
<script>
var map;
function GetMap() {
//Initialize a map instance.
map = new atlas.Map('myMap', {center: [144.95, -37.8], zoom: 12,
authOptions: {
authType: 'subscriptionKey',
subscriptionKey: 'ReplaceWithYourKey'
}
});
}
</script>
</head>
<body onload="GetMap()">
<div id='myMap' style='width: 100vw; height: 100vh;'></div>
</body>
</html>
This code and image is for Bing Maps:
<html>
<body>
<div id='myMap' style='width: 100vw; height: 100vh;'></div>
<script type='text/javascript'>
function loadMapScenario() {
var map = new Microsoft.Maps.Map(document.getElementById('myMap'), { });
map.setView({
center: new Microsoft.Maps.Location(-37.8,144.95),
zoom: 12
});
}
</script>
<script type='text/javascript' src='https://www.bing.com/api/maps/mapcontrol?key=ReplaceWithYourKey&callback=loadMapScenario' async defer></script>
</body>
</html>
This final code block and website is using Google Maps:
<html>
<head>
<script src="https://polyfill.io/v3/polyfill.min.js?features=default"></script>
<script src="https://maps.googleapis.com/maps/api/js?key=ReplaceWithYourKey&callback=initMap&libraries=&v=weekly" defer></script>
<script>
function initMap() {
const myLatLng = {
lat: -37.8, lng: 144.95
};
const map = new google.maps.Map(document.getElementById("gmp-map"), {
zoom: 12, center: myLatLng, fullscreenControl: false,
zoomControl: true, streetViewControl: false
});
}
</script>
</head>
<body>
<div id="gmp-map"></div>
</body>
</html>
In each case :
The javascript library for the map engine is loaded.
A map object is created with longitude, latitude and other parameters.
A <div> is created in the HTML to display the map.
As we add more advanced elements later on we will see that the basic approach of markers and layers is fairly similar between all three platforms.
So - what should you choose given they all work similarly? I'd suggest starting with the one whose visual aesthetics you like best OR the one best integrated with your current cloud provider. Start overlaying your data and see how it looks and performs. In the early stages, it is very quick to move to one of the other providers (see the similarity of each code snip above) if you find you need to switch.
My personal experiences :
Google Maps tends to be attractive and fast but can get very cluttered with restaurants, shops and other data taking away from what I am trying to display.
Azure Maps are well integrated into the rest of the Azure ecosystem and support Azure AD authentication, but can be slower and the richness of the map data can be lacking in places.
Bing Maps sits between the other two. The map data is richer than Azure Maps but not as cluttered as Google. However, it is not as well integrated into the rest of the Azure/Microsoft ecosystem.
What about OpenStreetMap?
OpenStreetMap is another mapping choice that has all the same types of capabilities as Azure/Google/Bing. The key difference is that I would describe OpenStreetMap as an open ecosystem rather than a platform. The map tiles themselves are freely available, but you need to either host them yourself or find a hoster. Similarly, several different APIs can be used to display and manipulate them. This provides significant flexibility but also means there is no single canonical approach.
Having said that though, this series will be about the back-end geospatial data capture, storage and processing, all of which will work just as well with an OpenStreetMap front end.
What next?
Next, we will walk through a full application and associated architecture here: Building map based apps - The Camino
Subscribe to my newsletter
Read articles from AndrewC directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
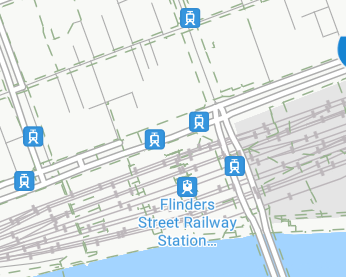