Python Interview Q/A

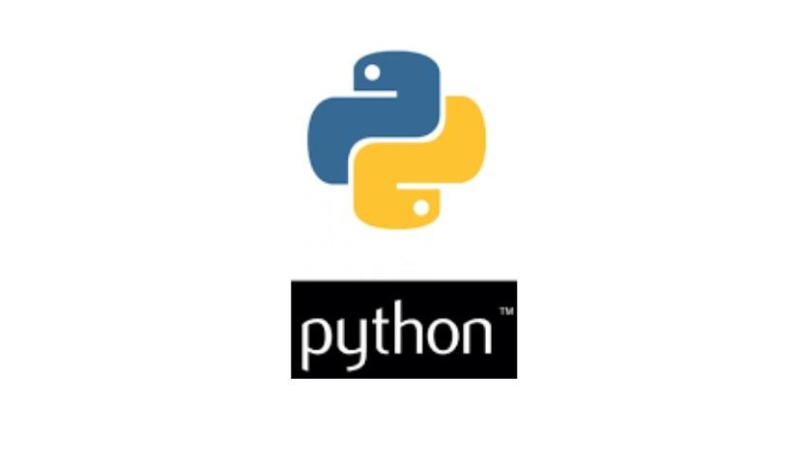
Basics of Python:
What is Python?
Python is a general-purpose interpreted, interactive, object-oriented, and high-level programming language used for various purposes, including web development, software development, mathematics operations, and more.Why should we use Python?
Python is easy to read, learn, and write. It supports multiple programming paradigms. Python is cross-platform. It has a vast standard library.
What are the applications of Python?
Web and Internet Development Scientific and Numeric Computing Education Desktop GUIs Software Development Business Applications
Functions and Modules:
How do you define functions in Python?
Functions in Python are defined using the def keyword, followed by the function name and parameters. The function body contains the code to be executed when the function is called.How do you import modules in Python?
Modules in Python are imported using the import keyword followed by the module name. For example, import math imports the math module.
Exception Handling:
What are the exceptions in Python? How do you handle them?
Exceptions in Python are errors that occur during program execution. They can be handled using try, except, else, and finally blocks. The try block contains code that may raise an exception, and the except block handles the exception if it occurs.How do you raise custom exceptions in Python?
Custom exceptions can be raised using the raise keyword followed by an exception class. You can create custom exception classes by inheriting them from the built-in Exception class.
Lists and Dictionaries:
How do you add and remove elements from a list in Python?
Elements can be added to a list using the append() method, and removed using methods like pop(), remove(), or del.How do you remove duplicates from a list in Python?
You can remove duplicates from a list by converting it to a set and then back to a list, assets automatically eliminate duplicates.How do you remove an item from a dictionary in Python?
You can remove an item from a dictionary using the pop() method or the del statement by specifying the key to be removed.
String Manipulation:
How do you split a string into a list of substrings?
You can split a string into a list of substrings using the split() method or regular expressions.How do you convert a string to uppercase and lowercase in Python?
Strings can be converted to uppercase using the upper() method and to lowercase using the lower() method.
Advanced Concepts:
How do you use list comprehensions in Python?
List comprehensions provide a concise way to create lists based on existing lists, sequences, or other iterables. They use a compact syntax to filter and transform elements.How do you work with regular expressions (RegEx) in Python?
Regular expressions are used to match patterns in strings. Python's re-module provides functions for working with regular expressions, including match(), search(), and sub().How do you perform API requests using the Requests library?
The Requests library in Python is used to make HTTP requests to web services and APIs. You can send GET, POST, PUT, DELETE, etc., requests and handle the responses.
These questions cover a wide range of Python topics, from the basics to more advanced concepts, and can help you prepare for Python interviews.
Object-Oriented Programming (OOP):
What is object-oriented programming (OOP)?
Object-oriented programming is a programming paradigm that organizes code into objects. Objects are instances of classes, which are blueprints for creating objects. OOP emphasizes encapsulation, inheritance, and polymorphism.What are Python classes and objects?
In Python, a class is a blueprint that defines the properties and behaviors of objects. An object is an instance of a class and represents a specific entity.
How do you create an object in Python? An object is created by calling the class as if it were a function. The class acts as a constructor, initializing the object and returning it.
Polymorphism and Inheritance:
What is polymorphism in Python?
Polymorphism is the ability of an object to take on multiple forms or have multiple behaviors. In Python, it is achieved through method overriding and method overloading.What is method overriding in Python?
Method overriding in Python allows a subclass to provide a specific implementation of a method that is already defined in its superclass. The subclass method overrides the behavior of the superclass method.What is inheritance in Python?
Inheritance is a mechanism in Python where a new class inherits properties and behaviors from an existing class. The existing class is called the superclass or parent class, and the new class is the subclass or child class.
File Handling:
How do you open and close files in Python?
Files can be opened using the open() function and closed using the close() method or by using the with statement, which automatically closes the file when done.What is the difference between read() and readline() methods for file reading? The read() method reads the entire file contents as a single string, while the readline() method reads one line at a time and returns a string.
Iterators and Generators:
What is an iterator in Python?
An iterator is an object that represents a stream of data and can be iterated (looped) over. It is used to traverse through elements in a sequence.What is a generator in Python?
A generator is a special type of iterator that generates values on the fly rather than storing them in memory. It uses the yield keyword to produce values one at a time.
Decorators:
What are decorators in Python?
Decorators are a powerful and flexible way to modify or enhance the behavior of functions or methods without changing their code. They are often used for tasks like logging, authentication, and memoization.How do you define and use decorators in Python?
Decorators are defined as functions and can be applied to other functions using the @ symbol before the function definition. They are typically used to wrap or modify the behavior of the target function.
Concurrency and Threading:
What is the Global Interpreter Lock (GIL) in Python?
The Global Interpreter Lock (GIL) is a mutex that allows only one thread to execute in the CPython interpreter at a time. It can limit the parallelism of multi-threaded Python programs.How can you achieve concurrency in Python?
Concurrency can be achieved in Python using multiple processes (multiprocessing) or threads (threading). Libraries like multiprocessing and threading are commonly used for this purpose.
These additional Python interview questions cover more advanced topics, including OOP, file handling, iterators, generators, decorators, and concurrency. Make sure to study these topics thoroughly to prepare for Python interviews effectively.
Subscribe to my newsletter
Read articles from Amrutha D directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amrutha D
Amrutha D
Gen AI Video Creator | Gen AIOps | AIOps Cloud DevOps Engineer | Let's Connect & share Technical knowledge & grow together in Technologies Everyday..