Luhn Algorithm Breakdown for Beginners

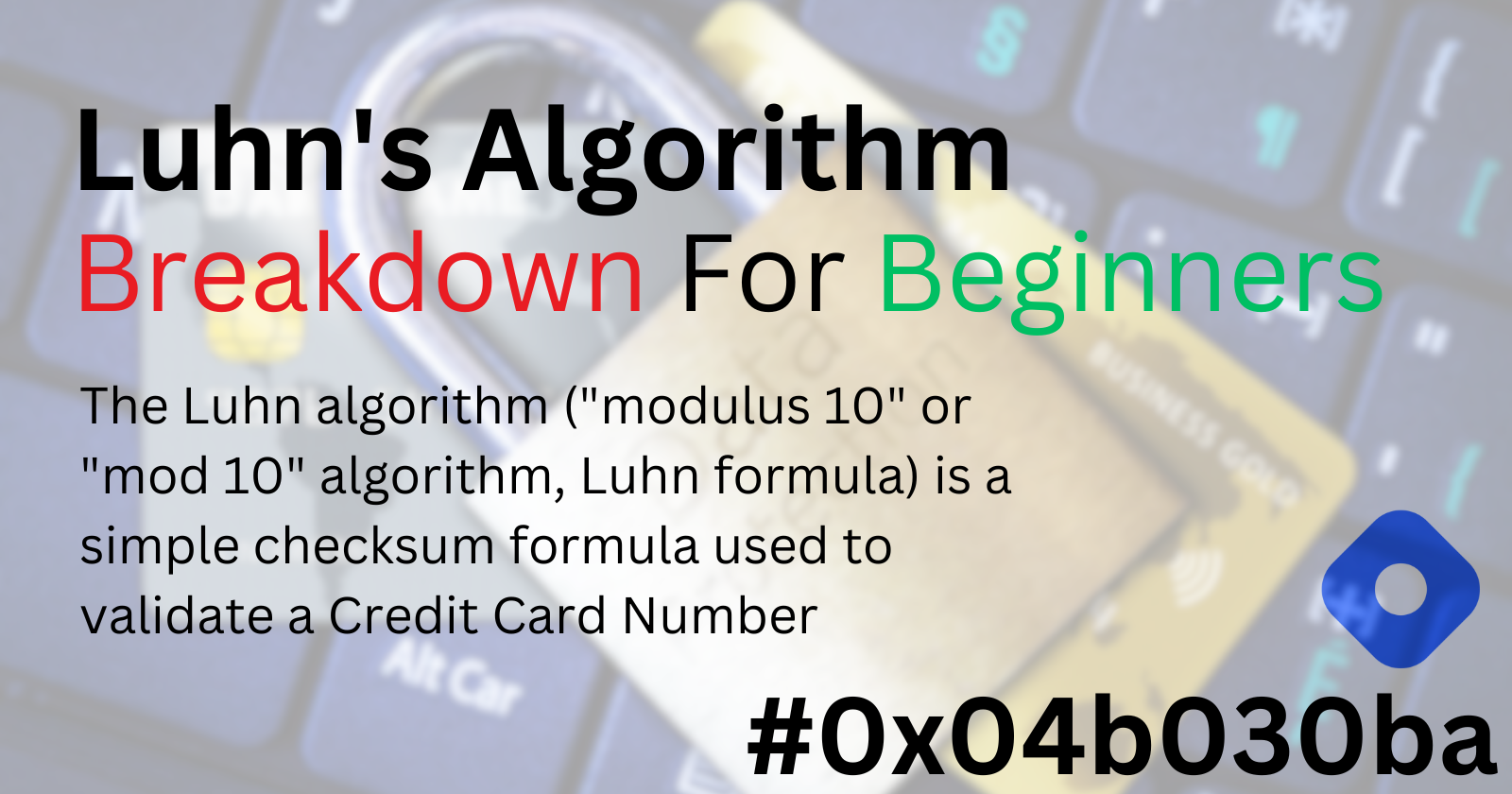
What is Luhn's Algorithm?
The Luhn algorithm, also known as the Luhn formula or modulus 10 algorithm, is a simple checksum formula used to validate a variety of identification numbers, such as credit card numbers, social security numbers, and more. It was created by Hans Peter Luhn in 1954 and is widely used to help catch errors in data entry and to prevent accidental transcription errors.
Today I'll show you how can we implement Lunh's Algorithm in Python for validating Credit Card numbers. The Python script we are gonna code will take a credit card number as input, process it, and check whether it's valid or not. Let's break it down step by step:
sum_odd = 0
sum_even = 0
- We will start by declaring two integer variables
sum_odd
andsum_even
having value 0. These variables will be used to keep track of the sums of odd and even digits in the credit card number.
card_number = input("Card number? ")
- After declaring our variables we will prompt the user to enter a credit card number.
card_number = card_number.replace("-", "")
card_number = card_number.replace(" ", "")
card_number = str(card_number)
- Using the replace() method we will remove any hyphens and white spaces from the user's entered Credit Card number as it is a string.
card_number = card_number[::-1]
- Using the reverse slicing technique we will reverse the credit card number to make it easier to process the digits from right to left.
for i in card_number[::2]:
sum_odd += int(i)
- Now with a
For Loop
we will iterate through the odd-indexed digits of the reversed card number (every other digit starting from the right) and add their values to thesum_odd
variable.
for i in card_number[1::2]:
i = int(i) * 2
if i >= 10:
sum_even += (1 + (i % 10))
else:
sum_even += i
After looping through the odd indexes, now we will iterate through the even-indexed digits of the reversed card number and perform some checks:
We will multiply each digit by 2.
If the result is greater than or equal to 10, we will add the digits of that result to the
sum_even
. For example, if the result is 16, we will add 1 and 6.If the result is less than 10, we will simply add it to
sum_even
.
total = sum_odd + sum_even
- Now we will calculate the total sum of the odd and even digits.
if total % 10 == 0:
print("VALID")
else:
print("INVALID")
- Now in the ultimate(final) step, we make sure to check that the total sum is divisible by 10. If it is, the program will print "VALID," indicating that the credit card number is valid. If not, it will print "INVALID."
Combined Code
sum_odd = 0
sum_even = 0
card_number = input("Card number? ")
card_number = card_number.replace("-","")
card_number = card_number.replace(" ","")
card_number = str(card_number)
card_number = card_number[::-1]
for i in card_number[::2]:
sum_odd += int(i)
for i in card_number[1::2]:
i =int(i) *2
if i>=10:
sum_even += (1 + (i % 10))
else:
sum_even += i
total = sum_odd+sum_even
if total%10==0:
print("VALID")
else:
print("INVALID")
An example in simple terms:
Remove Spaces and Hyphens:
Original Number: "4556 4243 2183 5962"
After Removing Spaces and Hyphens: "4556424321835962"
Reverse the Number:
- Reversed Number: "2695833214326654"
Separate Odd and Even Digits:
Odd Digits: 2, 9, 8, 3, 2, 4, 6
Even Digits: 6, 5, 4, 1, 3, 6, 5
Double the Even Digits (Digits at Even Positions):
- Doubled Even Digits: 12, 8, 6, 2, 6, 12, 10
Sum the Digits of Doubled Even Digits Greater Than or Equal to 10:
12 becomes 1 + 2 = 3
12 becomes 1 + 2 = 3
10 becomes 1 + 0 = 1
Updated Doubled Even Digits: 3, 8, 6, 2, 6, 3, 1
Sum All Digits (Odd Digits + Updated Even Digits):
Sum of Odd Digits: 2 + 9 + 8 + 3 + 2 + 4 + 6 = 34
Sum of Updated Even Digits: 3 + 8 + 6 + 2 + 6 + 3 + 1 = 29
Calculate the Total Sum (Sum of Odd and Updated Even Digits):
- Total Sum: 34 (Sum of Odd) + 29 (Sum of Updated Even) = 63
Check if the Total Sum is Divisible by 10:
- 63 % 10 = 3 (remainder)
Example Image:
The remainder from the above calculation is 3 which is != 0 so this "4556 4243 2183 5962" is not a VALID number (INVALID).
Note: Full code is available on my GitHub repository.
Tip: If you want the script keep to asking the user for credit card numbers you can enclose the whole code inside a while loop with condition True i-e while True:
and at the end of the loop reset the four variables to zero and empty strings then the script will process the card numbers and will tell the user whether each one is valid or not based on the Luhn algorithm for credit card validation.
#reset
card_number = ""
i = ""
sum_odd = 0
sum_even = 0
Subscribe to my newsletter
Read articles from Madah Ge directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
