Day1 : TWS Bash Blaze Challenge
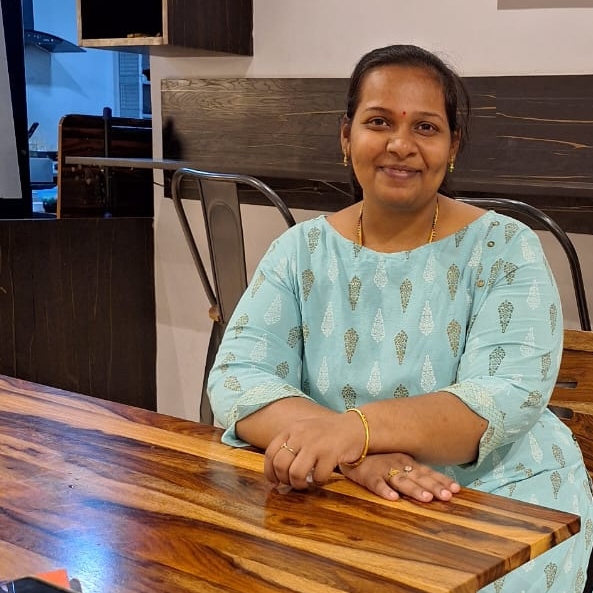
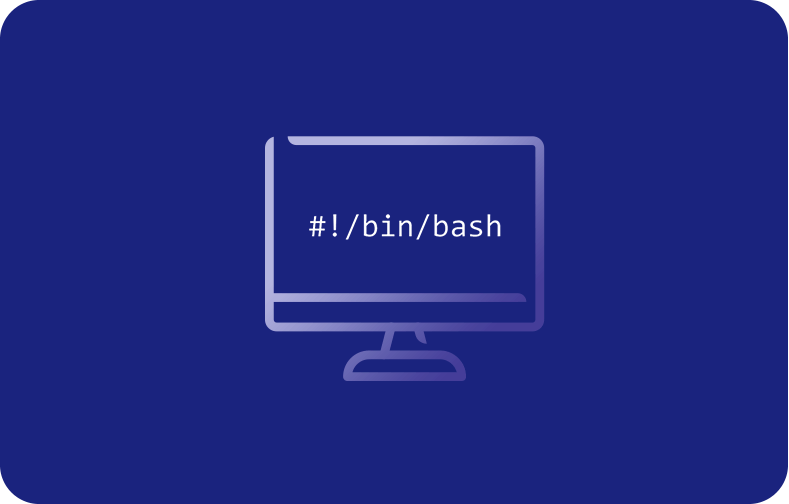
Bash scripting:
A bash script is a file containing a sequence of commands that are executed by the bash program line by line. It allows you to perform a series of actions, such as navigating to a specific directory, creating a folder, and launching a process using the command line.
By saving these commands in a script, you can repeat the same sequence of steps multiple times and execute them by running the script. Bash is one of the most commonly used Unix/Linux shells and is the default shell in many Linux distributions.
TASK1:Comments
Comments help you keep track of what your script is doing. They are useful because they allow you to leave internal notes throughout your script to remind you of things like what the next command will do or its expected output. You’re essentially annotating your scripts.
In Bash, we can identify comments using the # symbol. Every line that starts with the # sign is for our reference only. In Bash, anything after the # is not interpreted or executed.
# Say hello to the world
echo "Hello world!"
TASK2:Echo
The echo command is used to display messages on the terminal. This is a bash command that is mostly used in shell scripts to output status to a file.
#!/bin/bash
#echo is used to display messages
echo "welcome to the shell scripting"
welcome to the shell scripting
TASK3:Variables
Variables let you store data. You can use variables to read, access, and manipulate data throughout your script.
Note that:
The variable name cannot contain spaces, nor can there be spaces on either side of the equals sign.
Variable names are case-sensitive.
Use descriptive names that reflect the purpose of the variable.
Avoid using reserved keywords, such as
if
,then
,else
,fi
, and so on as variable names.
Let’s start by creating a variable called variable1
that has the string hello
stored inside it
#declaring variable
variable1=hello
echo $variable1
variable2=bash
echo $variable2
hello
bash
TASK4:Using variables
Now that you have declared variables, let's use them to perform a simple task. Create a bash script that takes two variables (numbers) as input and prints their sum using those variables.
#!/bin/bash
#using variables
echo 'Enter first number'
read a
echo 'Enter second number'
read b
result=$((a+b))
echo "Addition for two number i.e. $a and $b is:- $result"
TASK5:Using Built-in Variables
Built-in shell variables are predefined and are global variables that we can use in our script at any point in time. These are reserved shell variables and some of them may have a default value assigned by bash
. All built-in shell variables' names will be in uppercase.
some of the built-in variables are : BASH_VERSION, BASH, PWD, LANG, HOSTNAME, PATH and USER.
Your task is to create a bash script that utilizes at least three different built-in variables to display relevant information.
#!/bin/bash
#Description: Knowing about built-in shell variables
echo "My current bash path - $BASH"
echo "Bash version I am using - $BASH_VERSION"
echo "PID of bash I am running - $BASHPID"
echo "My home directory - $HOME"
echo "Where am I currently? - $PWD"
echo "My hostname - $HOSTNAME"
TASK6:Wildcards
Wildcard characters are used to define the pattern for searching or matching text on string data in the bash shell. Another common use of wildcard characters is to create regular expressions.
The three main wildcard characters are,
Star or Asterisk (*)
used to search for a particular character for zero or more times
Question mark (?):
used to search for a fixed number of characters where each question mark indicates each character
Square brackets ([]):
used to match with the characters of a defined range or a group of characters.
Thank you, for reading the article.
Happy Learning🙂
Subscribe to my newsletter
Read articles from Siri Chandana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
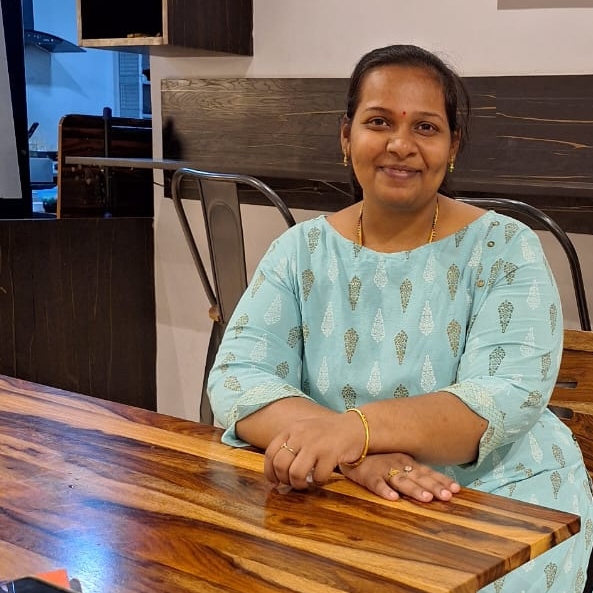